The difference between MyBatis and MyBatisPlus_Shi is Null’s blog-CSDN blog
1. Basic operations of MyBatis-Plus (BaseMapper)
Note : This test is run in springboot environment
Create a new UserMaper.java interface and inherit the BaseMapper of mybatis-plus :
public interface UserMapper extends BaseMapper<User> {
}
Automatically inject UserMapper in tests.
@Autowired
private UserMapper userMapper;
1.1 Insert operation
@Test
public void testInsert(){
User user = new User();
user.setUserName("ray");
user.setName("雷东宸");
user.setAge(18);
user.setMail("[email protected]");
user.setPassword("123456");
int result = this.userMapper.insert(user); // 数据库受影响的行数
System.out.println("result => " + result);
}

1.2 Update operation
@Test
public void testUpdateById(){
User user = new User();
user.setId(7L); // 根据id跟新,L表示long类型
user.setAge(19); // 更新的字段
int result = this.userMapper.updateById(user);
System.out.println("result => " + result);
}

1.3 Delete operation
@Test
public void testDeleteByMap(){
Map<String, Object> map = new HashMap<>();
map.put("user_name", "ray");
map.put("password", "123456");
// 根据map删除数据,不同条件之间是and关系
this.userMapper.deleteByMap(map);
}

1.4 Search operation
@Test
public void testSelectBatchIds(){
this.userMapper.selectBatchIds(Arrays.asList(2L,3L,4L));
}

2. Commonly used advanced operations of MyBatis-Plus (Wrapper)
Basic database data (the following operations are based on this data):
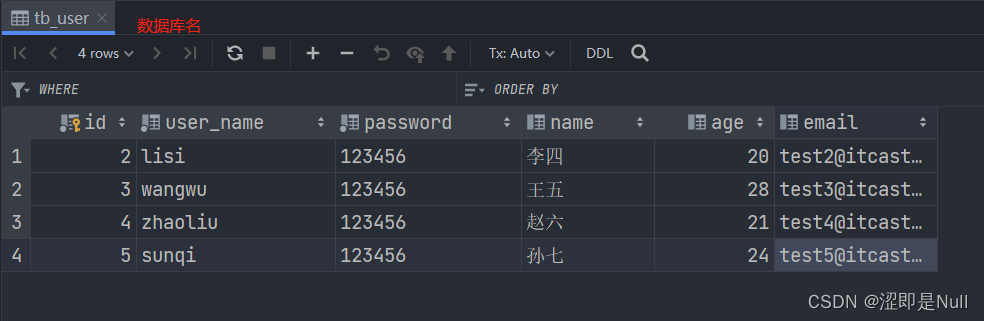
2.1 Related notes on dao entity classes
@Data
@TableName("tb_user")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String userName;
@TableField(select = false)
private String password;
private String name;
private Integer age;
@TableField(value = "email")
private String mail;
@TableField(exist = false)
private String address;
}
Here, we can see that mybatis-plus provides me with many convenient annotations:
@TableName ("tb_user"): Because in mybatis-plus, the default database table name found is "database name.entity name". However, our database table name tb_user is inconsistent with it, so we need to re-specify the table name in the database.
@TableId (type=IdType.AUTO): If type=IdType.AUTO, it means that the id will increase automatically.
@TableField (select=false): Indicates that the field value will not be returned when querying, and is used to protect the user's password information.
@TableField(value="email"): Because mail does not match the email field stored in our database (not the conventional camel case naming), direct query will cause the query to fail. Can be respecified using the value field.
@TableField(exist=false):假如该字段我们后期可能会插入数据库,但目前数据库还不存在该信息,那么我们就可以使用exist字段先不查询该字段以防报错。
2.2 DB策略配置
springboot开发中,在Application.properties可以添加如下配置:
2.2.1 idType(全局id自增长)
实体中id自增长(替代@TableId注解):
mybatis-plus.global-config.db-config.id-type=auto
这样在上面的实体类User中,当我们需要很多自增长的字段时,我们就不需要一个一个去配置@TableId(type=IdType.AUTO)。
2.2.2 tablePrefix(全局表名前缀)
映射数据库表名(替代@TableName注解):
mybatis-plus.global-config.db-config.table-prefix=tb_
我们数据库中的表为tb_User,那么我们加一个前缀 tb_ 就可以实现自动映射。
2.3 条件构造器wrapper(重要!!!)
条件构造器wrapper就是用来封装CRUD方法参数条件的一个接口,它的继承关系如下:
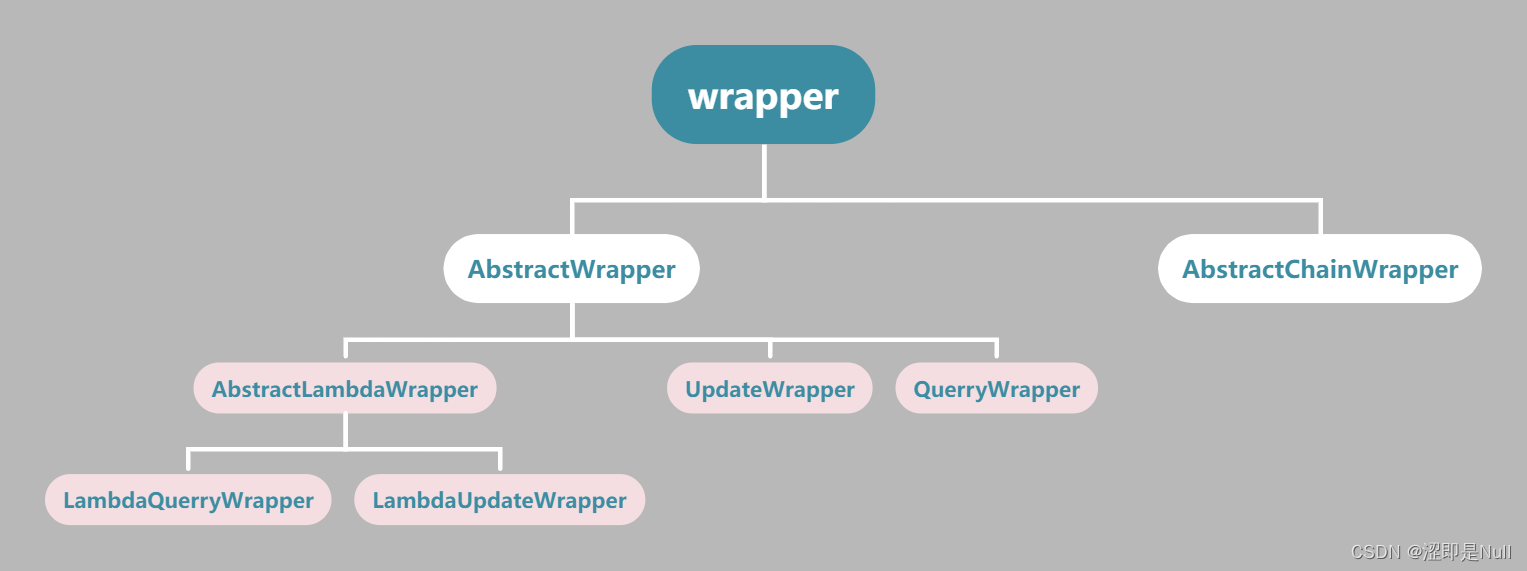
当然也可以打开源码包查看:
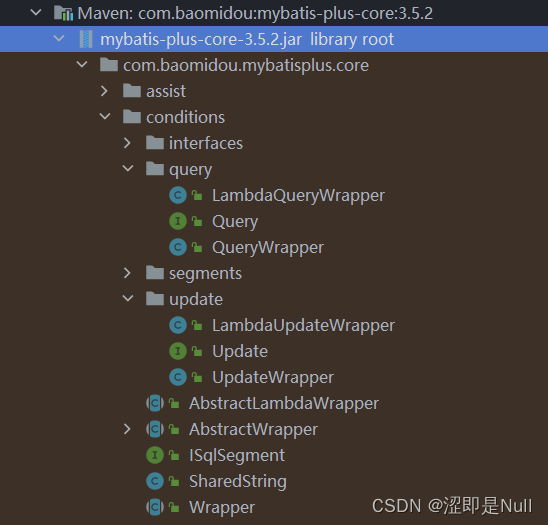
说明: QueryWrapper(LambdaQueryWrapper) 和 UpdateWrapper(LambdaUpdateWrapper) 的父类用于生成 sql的 where 条件, entity 属性也用于生成 sql 的 where 条件。
注意: entity 生成的 where 条件与使用各个 api 生成 的 where 条件没有任何关联行为 。
2.3.1 allEq
利用条件构造器QuerryWrapper中的allEq去查找数据库对象,allEq方法中封装的时map对象。
用法:
allEq(Map<R, V> params)
allEq(Map<R, V> params, boolean null2IsNull)
allEq(boolean condition, Map<R, V> params, boolean null2IsNull)
测试代码:
@Test
public void testAllEq() {
Map<String, Object> params = new HashMap<>();
params.put("name", "李四");
params.put("age", "20");
params.put("password", null);
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.allEq(params, false); // 第二个参数false表示null值不作为查询条件
List<User> users = this.userMapper.selectList(wrapper);
for (User user: users) {
System.out.println(user);
}
}
测试结果:

2.3.2 基本的比较操作
利用条件构造器的比较方法操作数据库。
eq
等于 =
ne 不等于 <>
gt 大于 >
ge 大于等于 >=
lt 小于 <
le 小于等于 <=
between BETWEEN 值1 AND 值2
notBetween NOT BETWEEN 值1 AND 值2
in 字段 IN (value.get(0), value.get(1), ...)
notIn
字段 NOT IN (v0, v1, ...)
测试代码举例:
@Test
public void testEq() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("password", "123456") // 密码等于 123456
.ge("age", 20) // 年龄大于等于20
.in("name", "李四", "王五", "赵六"); // 在李四,王五,赵六中去查找
List<User> users = this.userMapper.selectList(wrapper);
for (User user : users) {
System.out.println(user);
}
}
运行结果:
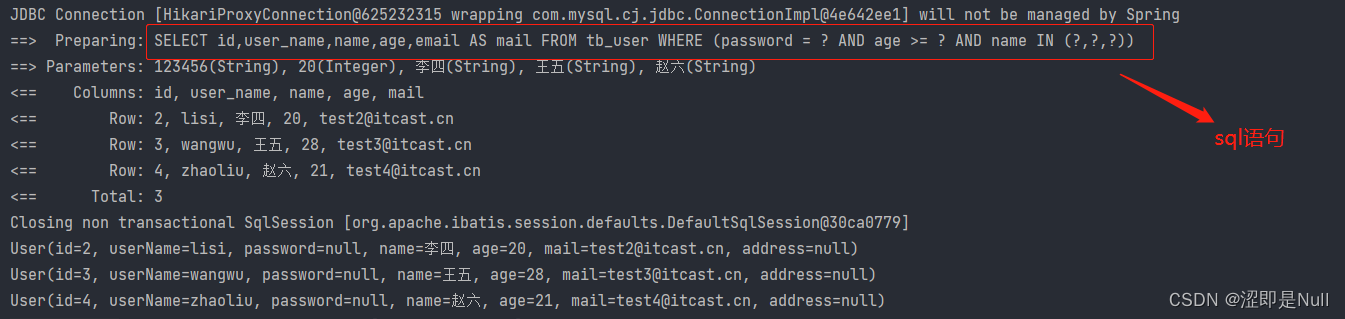
2.3.3 模糊查询
模糊查询操作数据库
like LIKE '%值%' 例: like("name", "王") ---> name like '%王%'
notLike NOT LIKE '%值%' 例: notLike("name", "王") ---> name not like '%王%'
likeLeft LIKE '%值' 例: likeLeft("name", "王") ---> name like '%王'
likeRight LIKE '值%' 例: likeRight("name", "王") ---> name like '王%'
测试代码:
@Test
public void testWrapper() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.like("name", "李"); //Parameters: %李%(String)
List<User> users = this.userMapper.selectList(wrapper);
for (User user : users) {
System.out.println(user);
}
}
运行结果:

2.3.4 排序
对从数据库中的数据排序。
orderBy 排序:ORDER BY 字段, ... 例: orderBy(true, true, "id", "name") ---> order by id ASC,name ASC
orderByAsc 排序:ORDER BY 字段, ... ASC 例: orderByAsc("id", "name") ---> order by id ASC,name ASC
orderByDesc 排序:ORDER BY 字段, ... DESC 例: orderByDesc("id", "name") ---> order by id DESC,name DESC
测试代码:
@Test
public void testWrapper() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.orderByDesc("age"); // 按照年龄倒叙排序
List<User> users = this.userMapper.selectList(wrapper);
for (User user : users) {
System.out.println(user);
}
}
运行结果:
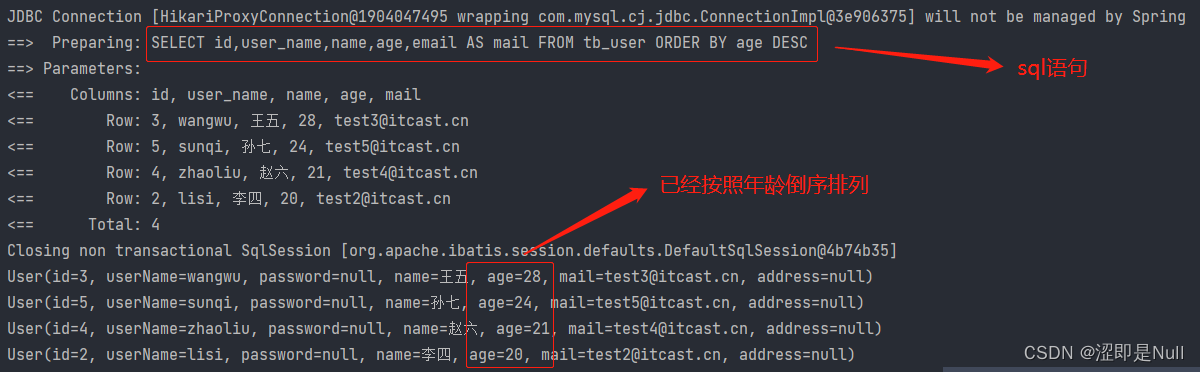
2.3.5 逻辑查询
or 拼接 OR 主动调用 or 表示紧接着下一个方法不是用 and 连接!(不调用 or 则默认为使用 and 连接)
and AND 嵌套 例: and(i -> i.eq("name", "李白").ne("status", "活着")) ---> and (name = '李白' and status <> '活着')
测试代码:
@Test
public void testWrapper() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name","李四").or().eq("age", 24); // 查找 姓名是李四 或者 年龄是24
List<User> users = this.userMapper.selectList(wrapper);
for (User user : users) {
System.out.println(user);
}
}
运行结果:
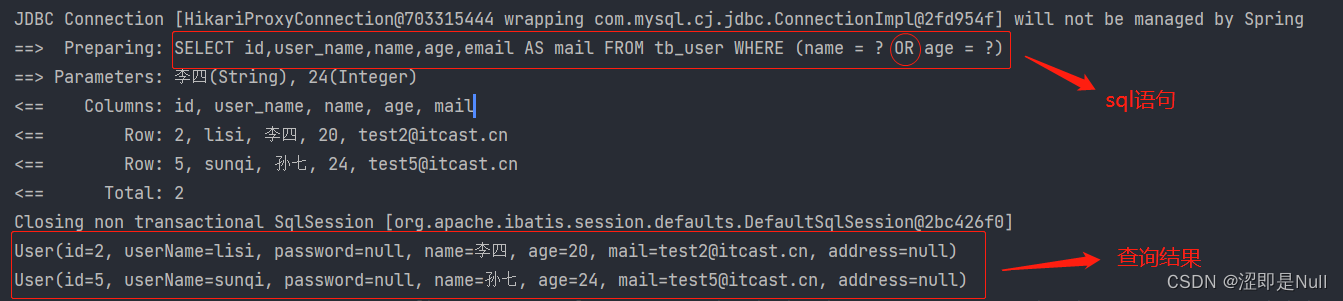
2.3.6 select
在MP查询中,默认查询所有的字段,如果有需要也可以通过select方法进行指定字段。
测试代码:
@Test
public void testWrapper() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name", "李四")
.or()
.eq("age", 24)
.select("id", "name", "age");
List<User> users = this.userMapper.selectList(wrapper);
for (User user : users) {
System.out.println(user);
}
}
运行结果:
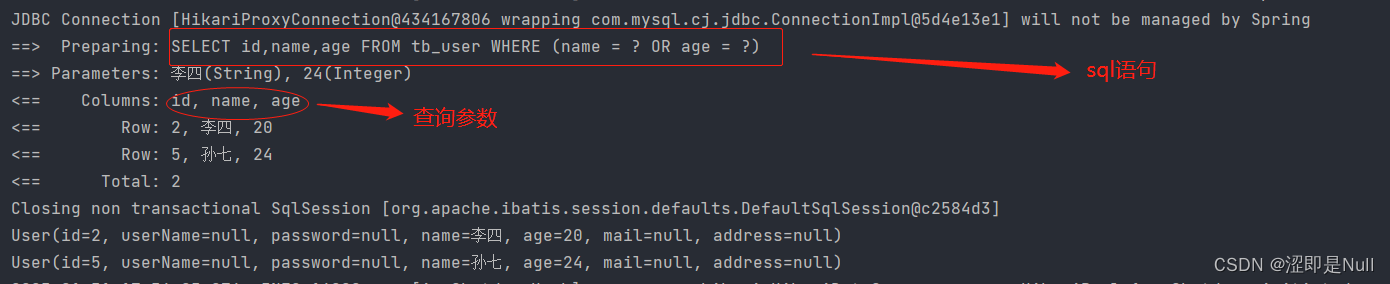
2.4 分页查询(IPage)
基于 MyBatis 的物理分页 ,开发者无需关心具体操作,配置好插件之后,写分页等同于普通 List 查询。
/**
* 根据 entity 条件,查询全部记录(并翻页)
*
* @param page 分页查询条件(可以为 RowBounds.DEFAULT)
* @param queryWrapper 实体对象封装操作类(可以为 null)
*/
IPage selectPage(IPage page, @Param(Constants.WRAPPER) Wrapper queryWrapper);
2.4.1 编写分页插件
这段代码可以固定的就这么写。
@Configuration
@MapperScan("com.example.mybatisplus.mapper") // 设置mapper接口的扫描包
public class MybatisPlusConfig {
@Bean // 配置分页插件
public MybatisPlusInterceptor paginationInnerInterceptor(){
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
// PaginationInnerInterceptor为分页拦截器
interceptor.addInnerInterceptor(new PaginationInnerInterceptor(DbType.MYSQL));
return interceptor;
}
}
2.4.2 编写测试方法
@Test
public void testSelectPage() {
Page<User> page = new Page<>(2, 2); // 查询第二页,每页显示2条数据
QueryWrapper<User> wrapper = new QueryWrapper<>();
// 设置查询条件
wrapper.like("email", "itcast");
IPage<User> iPage = this.userMapper.selectPage(page, wrapper);
System.out.println("数据总条数:" + iPage.getTotal());
System.out.println("数据总页数:" + iPage.getPages());
System.out.println("当前页数:" + iPage.getCurrent());
List<User> records = iPage.getRecords();
for (User record : records) {
System.out.println(record);
}
}
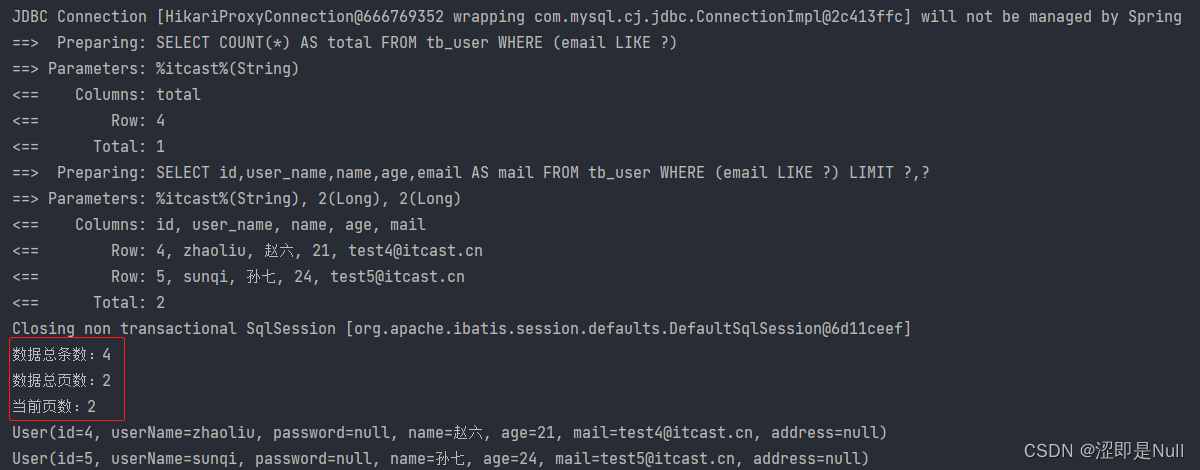