This article will show you how to use OpenCV to draw straight, square, circular and elliptical objects using the Go programming language (Golang) and the GoCV package.
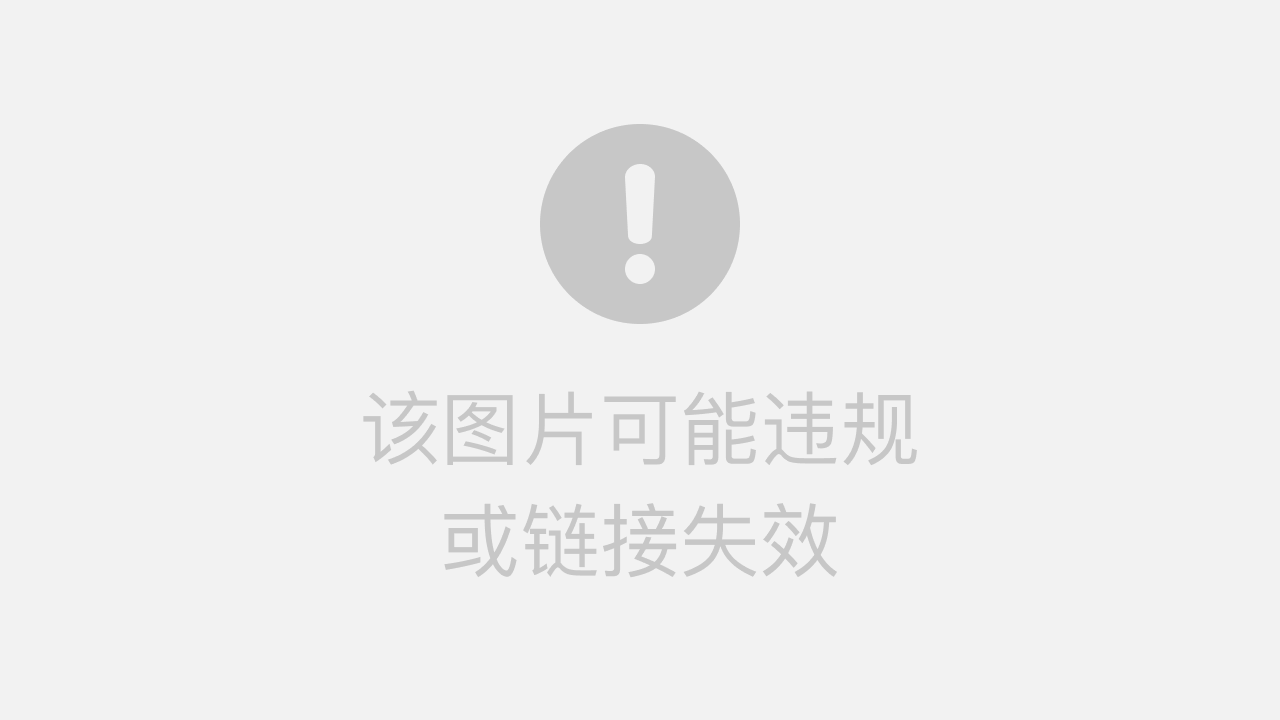
OpenCV is a programming function library mainly used for real-time computer vision. Originally developed by Intel, then supported by Willow Garage and Itseez. This library is cross-platform and available for free under the open source Apache 2 license.
In addition to drawing objects, OpenCV can also be used for face detection, motion tracking, etc.
GoCV is a package for the Golang language and supports the latest versions of Go and OpenCV v4.5.4 on Linux, macOS and Windows. GoCV makes the Go language a "first-class" client compatible with the latest developments in the OpenCV ecosystem.
GoCV gives golang programmers access to the OpenCV4 computer vision library.
Require
Install Golang (make sure the target operating system matches your computer)
Install OpenCV
Install OpenCV
For instructions on installing OpenCV, you can open the documentation on the GoCV website at: https://gocv.io/
macOS installation: https://gocv.io/getting-started/macos/
Install on Linux: https://gocv.io/getting-started/linux/
Windows installer: https://gocv.io/getting-started/windows/
Or you can visit the official website here (https://opencv.org/). We use MacOs operating system.
create project
First, let's create a new project using the following command:
mkdir belajar-gocv
cd belajar-gocv
Then create a module using the following command:
go mod init belajar-gocv
Open the project using another IDE or editor, such as VSCode. Create a file called main.go in the root directory . Next we will create a function that draws objects.
package main
func main() {
// buat canvas
}
Installation library
Installation package GoCV
go get -u -d gocv.io/x/gocv
Draw objects using OpenCV
In addition to improving the image quality of images or videos, OpenCV can also be used to draw and write objects.
Create canvas
Open main.go and change/add the following code in the main function:
package main
import "gocv.io/x/gocv"
func main() {
// membuat window baru
window := gocv.NewWindow("Belajar Gambar Objek")
defer window.Close()
// membuat canvas
width := 640
height := 480
canvas := gocv.NewMatWithSize(width, height, gocv.MatTypeCV8UC3)
// loop
for {
// tampilkan canvas di window
window.IMShow(canvas)
// wait key to press
if window.WaitKey(10) >= 0 {
break
}
}
}
Try running the above code using the following command:
go run main.go
A black blank window appears
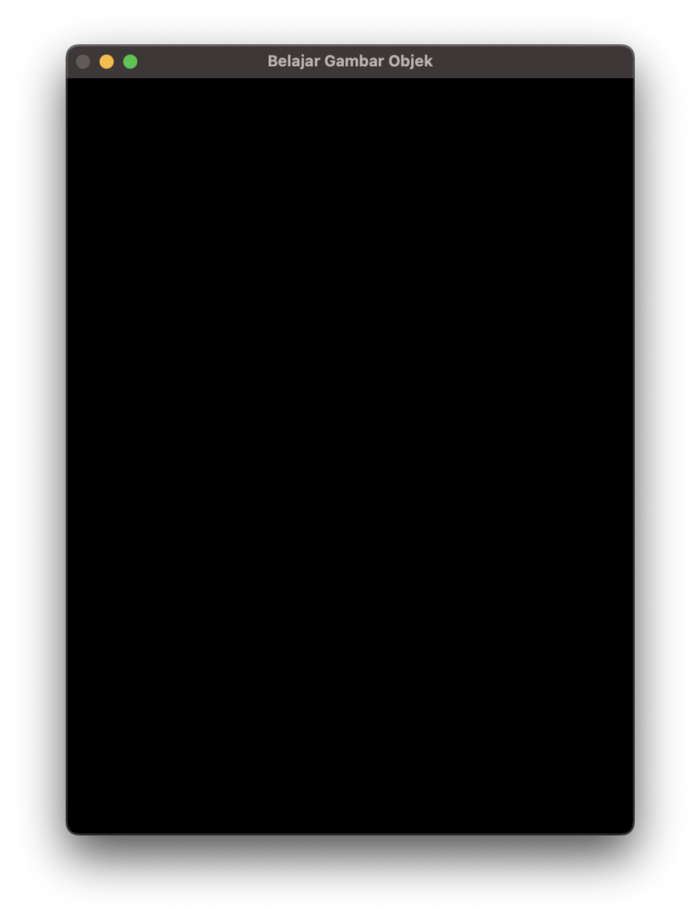
new window
Press the esc key on your keyboard to close the window.
make color
First we prepare the colors we want to use on the object. Open main.go and add the following lines after creating the canvas
// membuat canvas
...
// membuat warna
red := color.RGBA{255, 0, 0, 0}
green := color.RGBA{0, 255, 0, 0}
blue := color.RGBA{0, 0, 255, 0}
purple := color.RGBA{106, 90, 205, 0}
// loop
...
draw line
After successfully creating the canvas, next we try to draw the canvas with lines. Before drawing lines, we must understand the functions and properties used to draw lines. The function structure and attributes used to draw lines are as follows:
gocv.Line(img *gocv.Mat, pt1 image.Point, pt2 image.Point, c color.RGBA, thickness int)
img = is used to specify the location where the line is drawn using a memory address reference
pt1 = (x1, y1) creates the starting coordinates of the first point of the line
pt2 = (x2, y2) End coordinates create the end point of the straight line
c = (color) a value between 0-255 used to create RGBA colors
thickness=line width
Open main.go and add the following lines:
// membuat canvas
...
// membuat warna
...
// membuat garis
gocv.Line(&canvas, image.Pt(100, 150), image.Pt(300, 400), red, 1)
// loop
...
Try running the above code using the following command:
go run main.go
So the result will be like this:
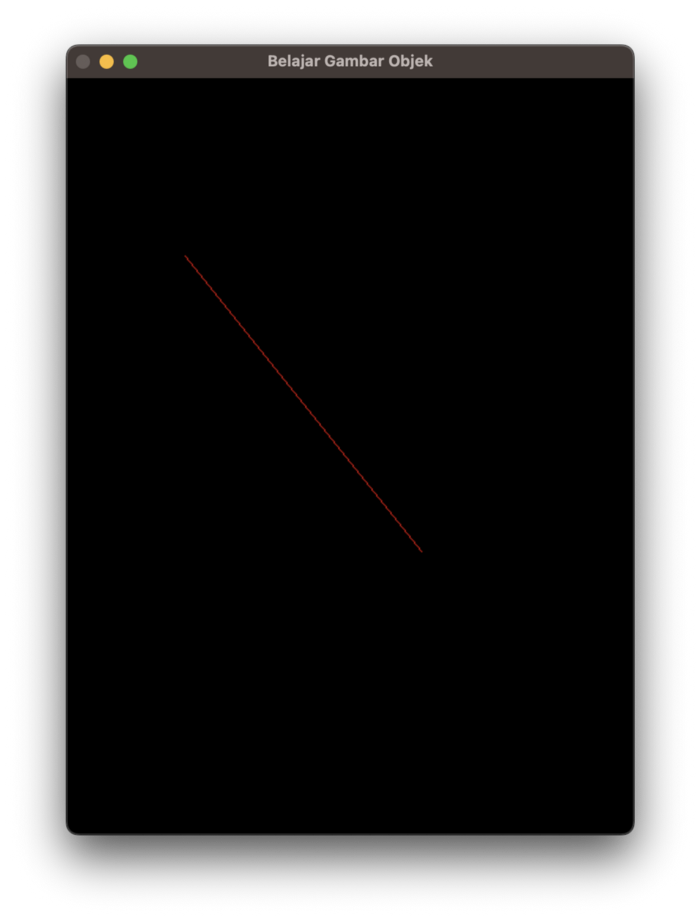
Create a square
The second object to create is a square.
gocv.Rectangle(img *gocv.Mat, r image.Rectangle, c color.RGBA, thickness int)
img: used to specify where the rectangle will be drawn by referencing its memory address
r = (x0, y0, x1, y1): x0 and y0 are the coordinates of the upper left corner of the square, x1 and y1 are the coordinates of the lower right corner of the square
c = (color): a value between 0-255 used to create RGBA colors
thickness: line width
Open main.go and add the following lines:
// membuat canvas
...
// membuat warna
...
// membuat garis
...
// membuat persegi
gocv.Rectangle(&canvas, image.Rect(150, 200, 350, 400), green, 2)
// loop
...
Try running the above code using the following command:
go run main.go
So the result will be like this:
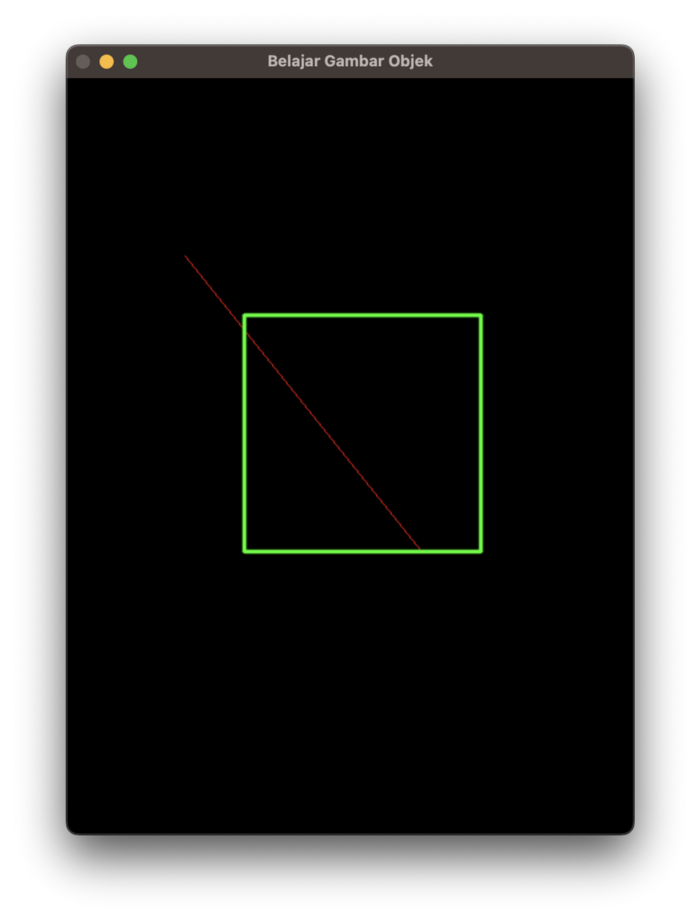
create a circle
gocv.Circle(img *gocv.Mat, center image.Point, radius int, c color.RGBA, thickness int)
img = is used to specify the location where the circle is drawn using its memory address reference
center = (x, y) represents the center of the circle on the x and y axes
radius = represents the radius (area) of a circle
c = (color) a value between 0-255 used to create RGBA colors
thickness = line width (-1 means fill all spaces)
Open main.go and add the following lines:
// membuat canvas
...
// membuat warna
...
// membuat garis
...
// membuat persegi
...
// membuat lingkaran
gocv.Circle(&canvas, image.Pt(220, 250), 80, blue, -1)
// loop
...
Try running the above code using the following command:
go run main.go
So the result will be like this:
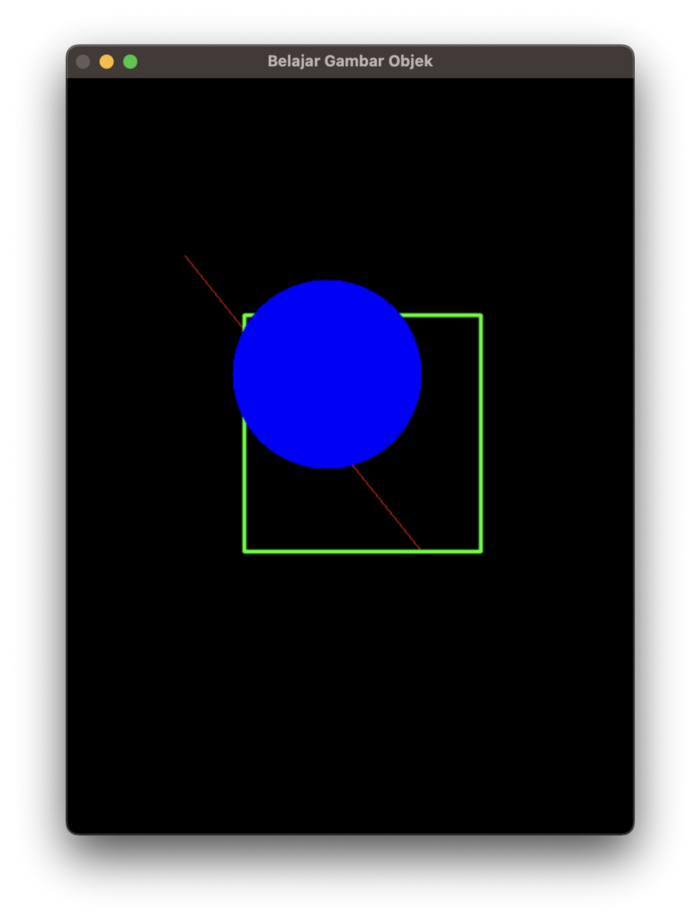
Create an ellipse
gocv.Ellipse(img *gocv.Mat, center image.Point, axes image.Point, angle float64, startAngle float64, endAngle float64, c color.RGBA, thickness int)
center = (x, y) coordinates of the center point of the ellipse
axes = (minorAxes, majorAxes) = length of minor and major axes
angel = rotation, the rotation angle of the ellipse is calculated counterclockwise
startAngle = starting angle (clockwise number)
endAngel = end angle (clockwise number)
c = (color) a value between 0-255 used to create RGBA colors
thickness = line width
Open main.go and add the following lines:
// membuat canvas
...
// membuat warna
...
// membuat garis
...
// membuat persegi
...
// membuat lingkaran
...
// membuat ellips
gocv.Ellipse(&canvas, image.Pt(300, 500), image.Pt(100, 50), 45, 130, 270, purple, 4)
// loop
...
Try running the above code using the following command:
go run main.go
So the result will be like this:
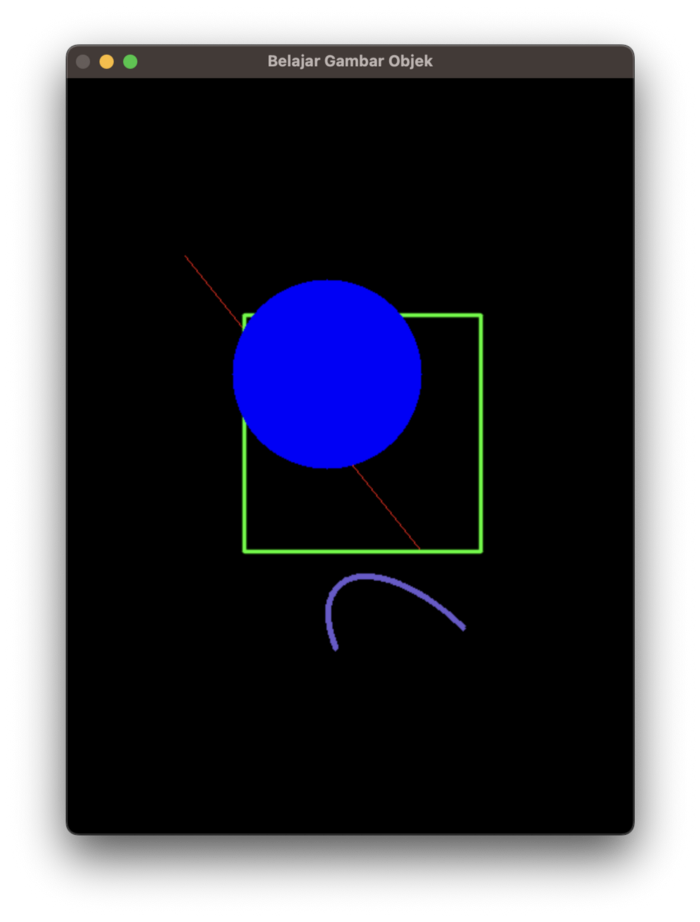
source code
https://github.com/apriliantocecep/belajar-gocv
☆ END ☆
If you see this, it means you like this article, please forward it and like it. Search "uncle_pn" on WeChat. Welcome to add the editor's WeChat "woshicver". A high-quality blog post will be updated in the circle of friends every day.
↓ Scan the QR code to add editor↓