Preface
I have nothing to do on weekends. I watched the video explaining the string-related algorithms online in July. I received a lot of money. I followed the video explanation and made a few notes for future review and review. I am also happy to share it with you. I won’t talk too much about the polite words on the road like how important strings are in algorithms. Let’s go straight to the notes.
1. String
- java: String is a built-in type and cannot be changed. (If you need to change, consider: StringBuffer, StringBuilder, char[], etc.)
2. Classification
Related question types related to strings usually include the following aspects:
- Conceptual understanding: lexicographical order
- Simple operations: insert, delete characters, rotate
- Rule judgment (whether Roman numeral conversion is a legal integer or floating point number)
- Number operations (large number addition, binary addition)
- sort, exchange
- Character Count: Anagrams
- Matching (regular expression, full string matching, KMP, period judgment)
- Dynamic programming (LCS, edit distance, longest palindrome substring)
- Search (word transformation, permutation and combination)
3. Examples
1. Exchange: Sort a string containing only 01, and exchange the positions of any two numbers. How many exchanges are needed at least?
Idea: Sweep from both ends to the middle. During the sweeping process, if you encounter a 1 on the left, it will exchange positions with the 0 encountered on the right, and it will end when there is a subscript on the left. The specific code is as follows:
1 public static void main(String[] strs) {
2 int count = 0;
3 int[] arrays = new int[] {
0, 0, 1, 1, 1, 0, 1, 0, 0, 1};
4 int left = 0;
5 int right = arrays.length - 1;
6 while (true) {
7 while (arrays[left] == 0) {
8 left++;
9 }
10 while (arrays[right] == 1) {
11 right--;
12 }
13 if (left >= right) {
14 break;
15 } else {
16 int temp = arrays[left];
17 arrays[left] = arrays[right];
18 arrays[right] = temp;
19 count++;
20 }
21 }
22 Logger.println("交换次数:" + count);
23 for (int array : arrays) {
24 Logger.print(array + ", ");
25 }
26 }
For the sake of clarity, the string output after the number of exchanges and sorting is as follows:
交换次数:3
0, 0, 0, 0, 0, 1, 1, 1, 1, 1,
2. String replacement and copying: delete all a’s in a string and copy all b’s (the character array is large enough)
Idea: See the code comments for detailed ideas
1 public static void main(String[] strs) {
2 char[] input = new char[]{
'a', 'b', 'c', 'd', 'a', 'f', 'a', 'b', 'c', 'd', 'b', 'b', 'a', 'b'};
3 char[] chars = new char[50];
4 for (int j = 0; j < input.length; j++) {
5 chars[j] = input[j];
6 }
7 Logger.println("操作前:");
8 for (char c:chars
9 ) {
10 Logger.print(c + ", ");
11 }
12 int n = 0;
13 int countB = 0;
14 // 1、删除a,用n当做新下标,循环遍历数组,凡是不是a的元素都放到新下标的位置,由于新n增长慢,老下标i增长快,所以元素不会被覆盖。
15 // 并且在删除a时顺便记录b的数量,以便下一步复制b时可以提前确定数组最终的最大的下标。
16 for (int i = 0; chars[i] != '\u0000' && i < chars.length; i++) {
17 if (chars[i] != 'a') {
18 chars[n++] = chars[i];
19 }
20 if (chars[i] == 'b') {
21 countB++;
22 }
23 }
24
25 // 2、复制b,由于在第一步中就已经知道了字符串中b的个数,这里就能确定最终字符串的最大下标,从最打下表开始倒着复制原字符串,碰到b时复制即可。
26 int newMaxIndex = n + countB - 1;
27 for (int k = n - 1; k >= 0; k--) {
28 chars[newMaxIndex--] = chars[k];
29 if (chars[k] == 'b') {
30 chars[newMaxIndex--] = chars[k];
31 }
32 }
33
34 Logger.println("\n操作后:");
35 for (char c:chars
36 ) {
37 Logger.print(c + ", ");
38 }
39 }
3. Exchange asterisks: If a string only contains * and numbers, please put all * at the beginning.
For example: 1 * 2 * 4 * 3 => * * * 1 2 4 3
- Option 1: Operate backwards, starting from the largest subscript and traversing forward. When encountering elements other than *, add them to the "new" subscript. After the traversal is completed, j represents the number of *, and then 0-j Just assign the value to *. (After the operation, the relative position of the numbers remains unchanged) The code is as follows:
1 public static void main(String[] strs) {
2 char[] chars = new char[]{
'1', '*', '4', '3', '*', '5', '*'};
3 // 方案一(操作后,数字的相对位置不变)
4 // 倒着操作:从最大下标开始向前遍历,遇到非*号的元素则加入"新"下标中,遍历完毕后,j即代表*号的个数,然后将0-j赋值为*即可。
5 int j = chars.length - 1;
6 for (int i = j; i >= 0; i--) {
7 if (chars[i] != '*') {
8 chars[j--] = chars[i];
9 }
10 }
11 while (j >= 0) {
12 chars[j--] = '*';
13 }
14 for (char c:chars
15 ) {
16 Logger.print(c + ", ");
17 }
18 }
The output is as follows:
*, *, *, 1, 4, 3, 5,
- Option 2 (after the operation, the relative position of the array will change) quick sort division, according to the loop invariant (the condition is true after each step of the loop): For example, [0...i-1] in this question is *, [i...j-1 ] is a number, [j...n-1] has not been detected. During the loop, as i and j increase, this condition remains unchanged. The code is as follows:
1 public static void main(String[] strs) {
2 char[] chars = new char[]{
'1', '*', '4', '3', '*', '5', '*'};
3 // 方案二(操作后,数组的相对位置会变)
4 // 快排划分,根据循环不变式(每一步循环之后条件都成立):如本题[0..i-1]是*,[i..j-1]是数字,[j...n-1]未探测,循环时,随着i和j增加,维护此条件依然不变
5 for (int i = 0, j = 0; j < chars.length; ++j) {
6 if (chars[j] == '*') {
7 char temp = chars[i];
8 chars[i] = chars[j];
9 chars[j] = temp;
10 i++;
11 }
12 }
13 for (char c:chars
14 ) {
15 Logger.print(c + ", ");
16 }
17 }
The output is as follows:
*, *, *, 3, 1, 5, 4,
4. Word Flip
例如:I am a student =》 student a am I
Ideas:
1. First flip the entire string: such as: I am a student => tneduts a ma I
2. Determine each word through the spaces, and then flip each word
code show as below:
1 public static void main(String[] strs) {
2 String input = "I am a student";
3 char[] chars = input.toCharArray();
4 int i = 0;
5 int j = chars.length - 1;
6 while (i < j) {
7 swap(chars, i++, j--);
8 }
9 int front = 1;
10 int tail = 0;
11 while (front < chars.length) {
12 if (chars[front] == ' ') {
13 int frontTemp = front - 1;
14 while (tail < frontTemp) {
15 swap(chars, tail++, frontTemp--);
16 }
17 tail = front + 1;
18 }
19 front++;
20 }
21 for (char c:chars
22 ) {
23 Logger.print(c);
24 }
25 }
26
27 public static void swap(char[] chars, int index1, int index2) {
28 char temp = chars[index1];
29 chars[index1] = chars[index2];
30 chars[index2] = temp;
31 }
The output is as follows:
student a am I
5. Substring anagram: Given two strings a and b, ask whether b is a substring anagram of a.
For example: a=hello. b=lel, lle, ello are all true; b=elo is false
Ideas:
-
- 1. First, you need to understand whether two strings are anagrams:
-
- Sort the two strings according to the same rules. If they are equal after sorting, they are anagrams.
- Count the letters that appear in the two strings. If the same letter in the two strings appears the same number of times, it is an anagram.
-
- 2. Then traverse from the first element of the parent string. Each time it traverses an element, the position from the current element to the length of the substring is used as an interval and compared with the substring to see if it is an anagram.
The last question combines some of the skills used in the previous questions. It is quite interesting. I will not post the code here, but also stimulate everyone's hands-on ability. Interested children's shoes may wish to try to write.
Finally, I will share an analysis of the answers to the interview questions.
Due to the large amount of content and limited space, the information has been organized into PDF documents. If you need the complete document with answers to the most comprehensive Android intermediate and advanced interview questions in 2023, you can scan the QR code to get it! ! !
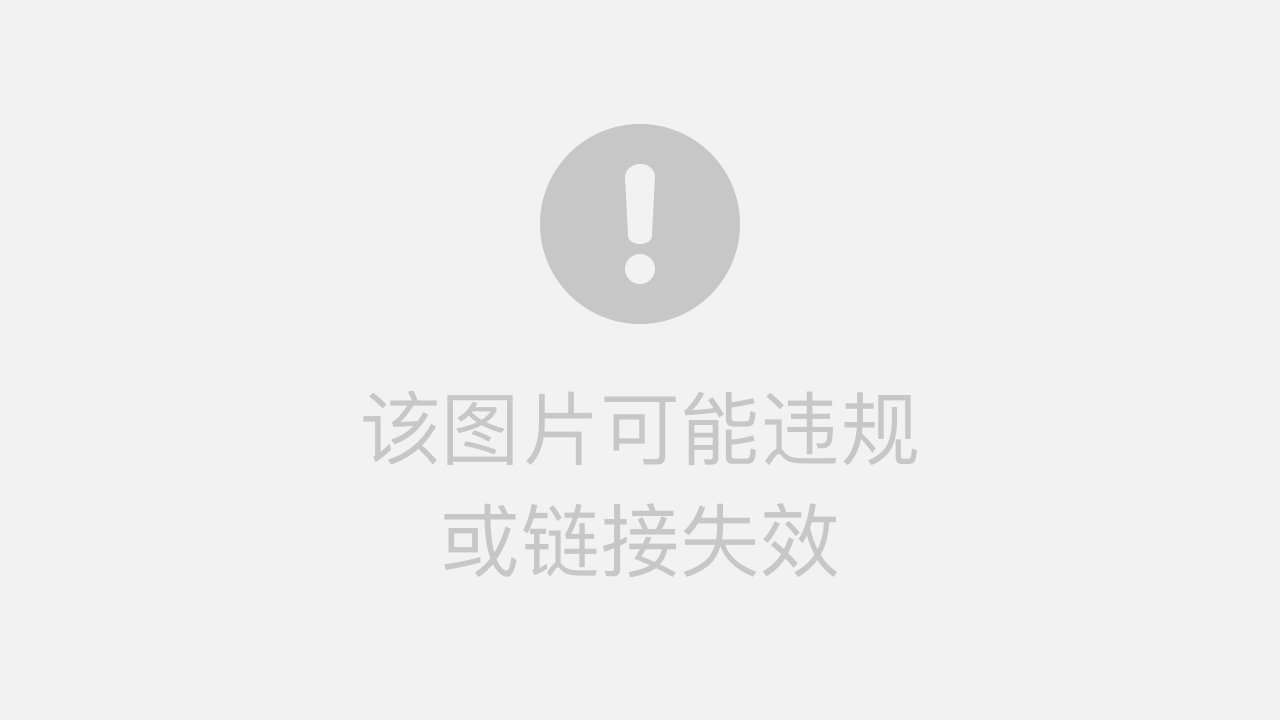
Table of contents
Chapter 1 Java
- Java basics
- Java Collections
- Java multithreading
- Java virtual machine
Chapter 2 Android
- Related to the four major components of Android
- Android asynchronous tasks and message mechanism
- Android UI drawing related
- Android performance tuning related
- IPC in Android
- Android system SDK related
- Third-party framework analysis
- Comprehensive technology
- Data structure
- Design Patterns
- computer network
- Kotlin aspect
Chapter 3 Audio and video development high-frequency interview questions
- Why can a huge original video be encoded into a very small video? What is the technology involved?
- How to optimize the instant start of live broadcast?
- What is the most important role of histogram in image processing?
- What are the methods of digital image filtering?
- What features can be extracted from images?
- What are the criteria for measuring the quality of image reconstruction? How to calculate?
Chapter 4 Flutter high-frequency interview questions
- Dart part
- Flutter part
Chapter 5 Algorithm High Frequency Interview Questions
- How to find prime numbers efficiently
- How to use binary search algorithm
- How to efficiently solve rainwater problems
- How to remove duplicate elements from sorted array
- How to perform modular exponentiation efficiently
- How to find the longest palindrome substring
Chapter 6 Andrio Framework
- Analysis of system startup process interview questions
- Analysis of Binder interview questions
- Analysis of Handler interview questions
- Analysis of AMS interview questions