topic description
Please write a sequence detection module to detect whether the input signal a satisfies the 01110001 sequence, and when the signal satisfies the sequence, an indication signal match is given.
The interface signal diagram of the module is as follows:
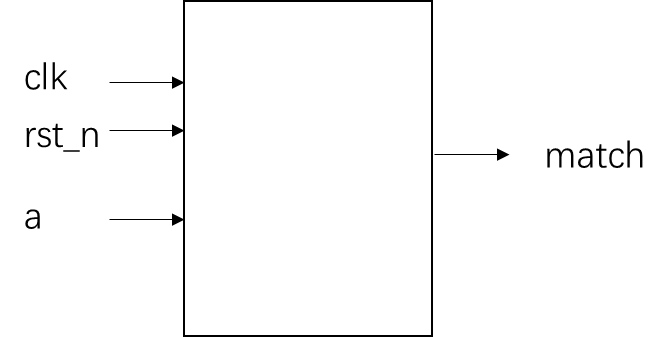
The timing diagram of the module is as follows:

Please use Verilog HDL to achieve the above functions
Enter a description:
clk: system clock signal
rst_n: asynchronous reset signal, active low
a: single-bit signal, data to be detected
Output description:
match: When the input signal a satisfies the target sequence, the signal is 1, and the signal is 0 at other times
problem solving ideas
To determine that the serial sequence is equal to the target sequence, it is first necessary to convert the serial sequence into a number equal to the bit width of the target sequence;
So our idea is to define a shift register to receive serial input data, and then use the shift register to compare with the target sequence. The specific implementation code is as follows:
`timescale 1ns/1ns
module sequence_detect(
input clk,
input rst_n,
input a,
output reg match
);
parameter GOAL_SER = 8'b01110001 ;
reg [7:0] sft_reg ;
always@(posedge clk or negedge rst_n)begin
if(~rst_n)begin
sft_reg <= 8'hFF ; // 复位值给全1
end else begin
sft_reg <= {sft_reg[6:0],a};
end
end
always@(posedge clk or negedge rst_n)begin
if(~rst_n)begin
match <= 1'd0 ;
end else if(sft_reg == GOAL_SER ) begin
match <= 1'b1 ;
end else begin
match <= 1'd0 ;
end
end
endmodule
Pay attention to a detail here, because the highest bit of the target sequence is 0, and our shift register shifts from low to high;
If the reset is all 0, then if the first sequence after reset is 111001x, the target sequence is actually not satisfied at this time
But it will be misjudged to be satisfied.