Table of contents
2. Stack simulation implementation
2. The simulated stack is presented in the form of an (integer) array
2.5 Get the number of effective elements in the stack
2.6 Get the top element of the stack
2.7 Complete code implementation
1. The concept of stack
(1) Stack : A special linear table that only allows insertion and deletion of elements at one fixed end . The end where data insertion and deletion operations are performed is called the top of the stack, and the other end is called the bottom of the stack. The data elements in the stack follow the principle of LIFO ( Last In First Out ).(2) Push stack: The insertion operation of the stack is called push / push / push, and the incoming data is at the top of the stack .(3) Popping the stack: The deletion operation of the stack is called popping the stack. The output data is on the top of the stack .
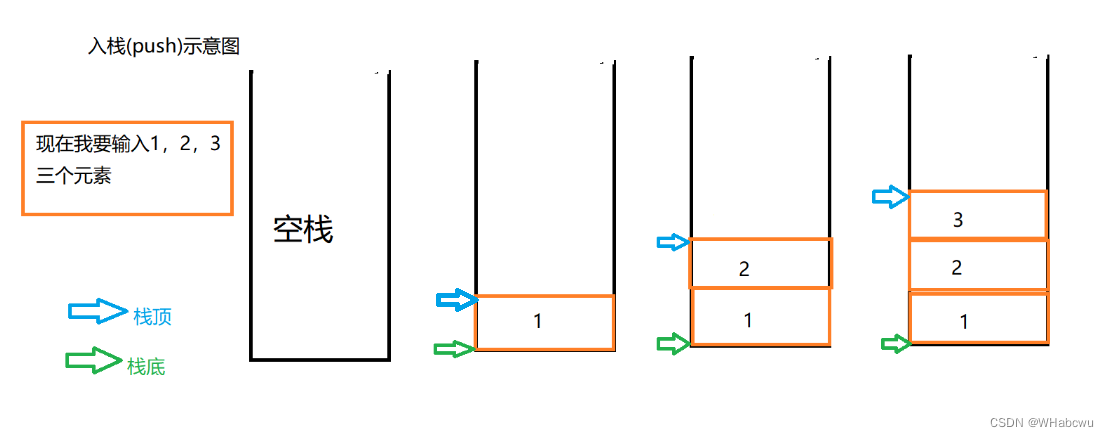
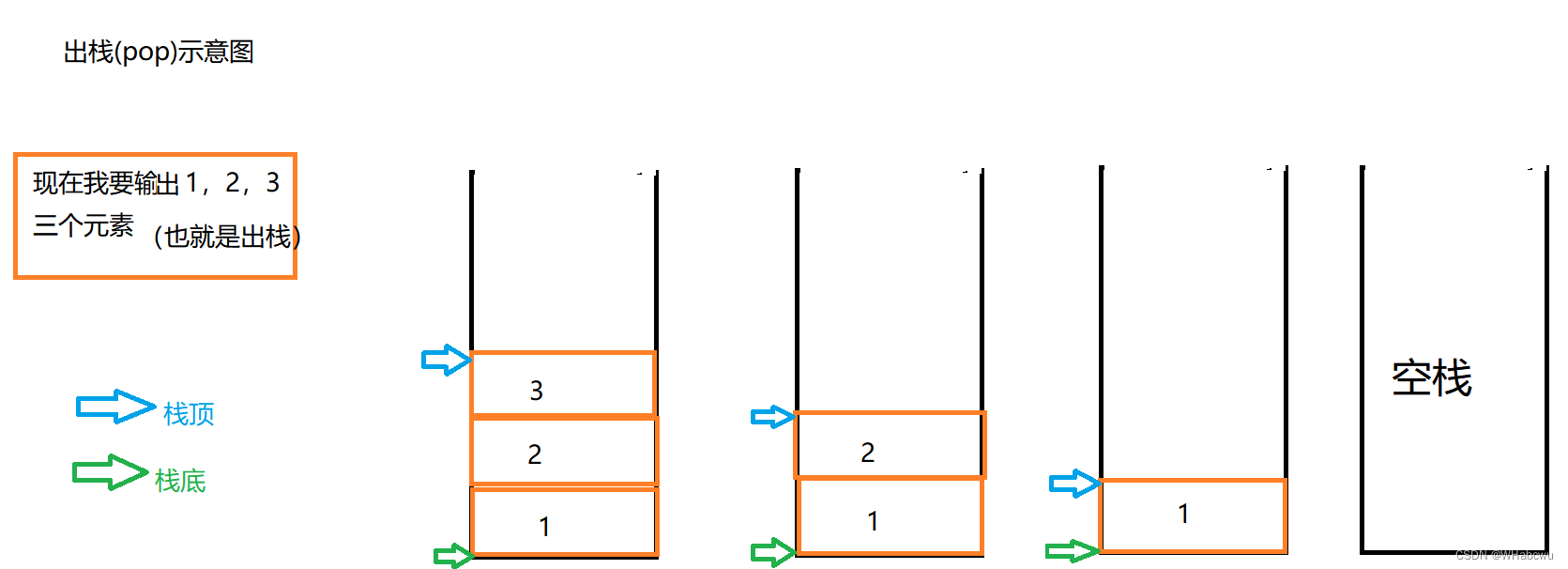
2. Stack simulation implementation
1. Stack method
2. The simulated stack is presented in the form of an (integer) array
2.1 Stack creation
public class MyStack {
public int[] arr;
public int size;
public MyStack() {
this.arr = new int[10];
}
}
2.2 push stack
(1) First, judge whether the existing stack is full, and if it is full, it needs to be expanded
Expansion:
private void ensureCapacity(){
if(size==arr.length)
{
arr= Arrays.copyOf(arr,size*2);
}
}
(2) Add to the array
public int push(int x){
ensureCapacity();
arr[size++]=x;
return x;
}
2.3 Is the stack empty?
public boolean empty(){
return 0 == size;
}
2.4 pop out
(1) First of all, it is necessary to judge whether the stack is empty. If it is empty, we need to throw an exception
Customize an exception as EmptyException as follows:
public class EmptyException extends RuntimeException{
public EmptyException() {
}
public EmptyException(String message) {
super(message);
}
}
(2) Legal pop-up
public int pop() {
if(empty()) {
throw new EmptyException("栈是空的!");
}
return arr[--size];
}
2.5 Get the number of effective elements in the stack
public int size(){
return size;
}
2.6 Get the top element of the stack
public int peek(){
if(empty()) {
throw new EmptyException("栈是空的!");
}
return arr[size-1];
}
2.7 Complete code implementation
import java.util.Arrays;
public class MyStack {
public int[] arr;
public int size;
public MyStack() {
this.arr = new int[10];
}
private void ensureCapacity(){
if(size==arr.length)
{
arr= Arrays.copyOf(arr,size*2);
}
}
public int push(int x){
ensureCapacity();
arr[size++]=x;
return x;
}
public boolean empty(){
return 0 == size;
}
public int pop() {
if(empty()) {
throw new EmptyException("栈是空的!");
}
return arr[--size];
}
public int size(){
return size;
}
public int peek(){
if(empty()) {
throw new EmptyException("栈是空的!");
}
return arr[size-1];
}
}
EmptyException
public class EmptyException extends RuntimeException{
public EmptyException() {
}
public EmptyException(String message) {
super(message);
}
}
3. Stack usage of linked list
(1) Singly linked list:
Insert from the head (push), delete from the head (pop)
The time complexity is O(1);
(2) Doubly linked list:
Can be inserted from the head (push) and deleted from the head (pop)
You can also insert from the end (push) and delete from the end (pop)
The time complexity is O(1);
LinkedList comes with its own implementation method:
public static void main(String[] args) {
LinkedList<Integer> linkedList = new LinkedList<>();
linkedList.push(1);
linkedList.push(2);
linkedList.push(3);
linkedList.push(4);
System.out.println(linkedList.peek());
System.out.println(linkedList.pop());
System.out.println(linkedList.pop());
}
operation result:
The above is my personal sharing, if you have any questions, welcome to discuss! ! !
I've seen this, why don't you pay attention and give a free like