Magician-Web3 is a blockchain development kit. It consists of two functions. One is to scan the blockchain and monitor transactions as needed by the developer. The other is some secondary packaging of web3j, which can reduce the workload of developers in some common scenarios. It plans to support three chains, ETH (BSC, POLYGAN, etc.), SOL and TRON, and has already completed the support for ETH.
Since then, the positioning of Magician has changed. It is no longer a framework in the web field, but a toolkit for Java development, covering the two fields of blockchain and web development. We can review the existing all components.
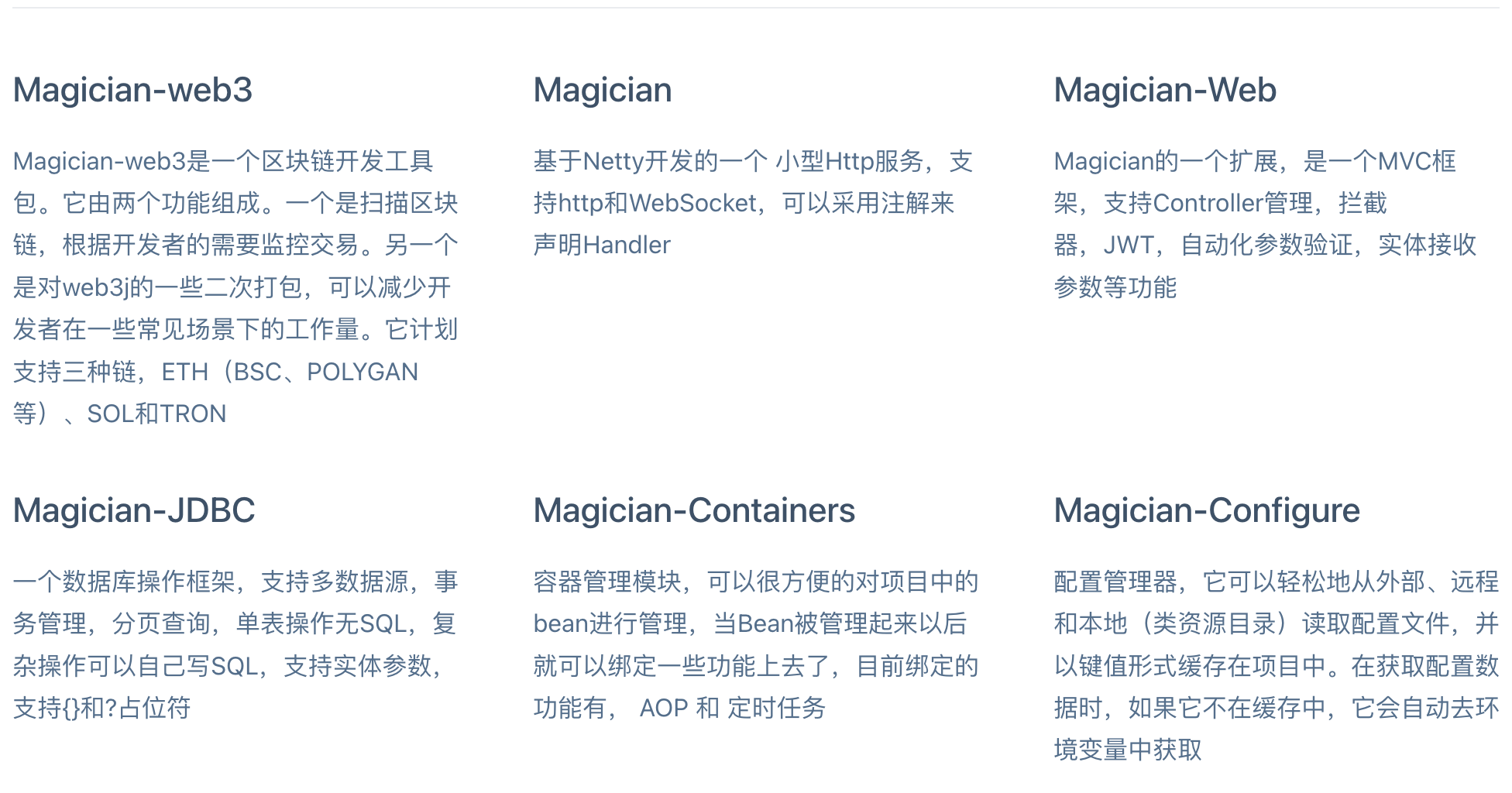
These components do not have strong dependencies, and some do not even have any dependencies, such as Magician-Web3, Magician, Magician-JDBC, and Magician-Configure, which can be used independently without any dependencies between them.
-
If you just need to develop a block scanning + storage operation, then the combination of Magician-Web3 + Magician-JDBC is just right for you.
-
If you just want to provide a simple http interface and websocket service to the outside world, then Magician can just satisfy you.
-
If you have a non-web project, but just have a need to put data into the database, then Magician-JDBC can help you.
Magician's positioning is not web development, but a toolkit for Java development! If you need a function, but other people's solutions are too heavy, then I may be able to help you.
Magician-Web3
Having said so much, let's go back to the introduction of Magician-Web3. There are two functions mentioned above, one is to scan the block, monitor the dynamics of the account address or contract, and the other is the secondary encapsulation of Web3j.
Sweep block + monitor
Create a listener first, pay attention to the comments in the code
/**
* 创建一个类,实现 EthMonitorEvent接口 即可
*/
public class EventDemo implements EthMonitorEvent {
/**
* 筛选条件,如果遇到了符合条件的交易,会自动触发 call方法
* 这些条件都是 并且的关系,必须要同时满足才行
* 如果不想根据某个条件筛选,直接不给那个条件设置值就好了
* 这个方法如果不实现,或者返回null, 那么就代表监听任意交易
*/
@Override
public EthMonitorFilter ethMonitorFilter() {
return EthMonitorFilter.builder()
.setFromAddress("0x131231249813d334C58f2757037F68E2963C4crc") // 筛选 fromAddress 发送的交易
.setToAddress("0x552115849813d334C58f2757037F68E2963C4c5e") // 筛选 toAddress 或 合约地址 收到的交易
.setMinValue(BigInteger.valueOf(1)) // 筛选发送的主链币数量 >= minValue 的交易
.setMaxValue(BigInteger.valueOf(10)) // 筛选发送的主链币数量 <= maxValue 的交易
.setFunctionCode("0xasdas123"); // 筛选调用合约内 某方法 的交易
}
/**
* 如果遇到了符合上面条件的交易,就会触发这个方法
* transactionModel.getEthTransactionModel() 是一个交易对象,内部包含hash,value,from,to 等 所有的数据
*/
@Override
public void call(TransactionModel transactionModel) {
String template = "EventOne 扫描到了, hash:{0}, from:{1}, to: {2}, input: {3}";
template = template.replace("{0}", transactionModel.getEthTransactionModel().getBlockHash());
template = template.replace("{1}", transactionModel.getEthTransactionModel().getFrom());
template = template.replace("{2}", transactionModel.getEthTransactionModel().getTo());
template = template.replace("{3}", transactionModel.getEthTransactionModel().getInput());
System.out.println(template);
}
}
Then start a scanning task, pay attention to the comments in the code
// 初始化线程池,核心线程数必须 >= 扫块的任务数量,建议等于扫块的任务数量
EventThreadPool.init(1);
// 开启一个扫块任务,如果你想扫描多个链,那么直接拷贝这段代码,并修改配置即可
MagicianBlockchainScan.create()
.setRpcUrl("https://data-seed-prebsc-1-s1.binance.org:8545/") // 节点的RPC地址
.setChainType(ChainType.ETH) // 要扫描的链(如果设置成ETH,那么可以扫描BSC, POLYGAN 等其他任意 以太坊标准的链)
.setScanPeriod(5000) // 每轮扫描的间隔
.setScanSize(1000) // 每轮扫描的块数
.setBeginBlockNumber(BigInteger.valueOf(24318610)) // 从哪个块高开始扫描
.addEthMonitorEvent(new EventOne()) // 添加 监听事件
.addEthMonitorEvent(new EventTwo()) // 添加 监听事件
.addEthMonitorEvent(new EventThree()) // 添加 监听事件
.start();
Web3j secondary packaging
Main chain currency query and transfer
String privateKey = ""; // 私钥
Web3j web3j = Web3j.build(new HttpService("https://data-seed-prebsc-1-s1.binance.org:8545/")); // 链的RPC地址
// 这种方式是单例的
EthHelper ethHelper = MagicianWeb3.getEthBuilder().getEth(web3j, privateKey);
// 如果你想创建多个EthHelper对象,可以用这种方式
EthHelper ethHelper = EthHelper.builder(web3j, privateKey);
// 余额查询
BigInteger balance = ethHelper.balanceOf(fromAddress);
// 转账
TransactionReceipt transactionReceipt = ethHelper.transfer(
toAddress,
BigDecimal.valueOf(1),
Convert.Unit.ETHER
);
InputData codec
// 这种方式是单例的
EthAbiCodec ethAbiCodec = MagicianWeb3.getEthBuilder().getEthAbiCodec();
// 如果你想创建多个EthAbiCodec对象,可以直接new
EthAbiCodec ethAbiCodec = new EthAbiCodec();
// 编码
String inputData = ethAbiCodec.getInputData(
"transfer", // 方法名
new Address(toAddress), // 参数1
new Uint256(new BigInteger("1000000000000000000")) // 参数2,如果还有其他参数,可以继续传入下一个
);
// 解码
List<Type> result = ethAbiCodec.decoderInputData(
"0x" + inputData.substring(10), // 去除方法签名的inputData
new TypeReference<Address>() {}, // 被编码的方法的参数1 类型
new TypeReference<Uint256>() {} // 被编码的方法的参数2 类型, 如果还有其他参数,可以继续传入下一个
);
for(Type type : result){
System.out.println(type.getValue());
}
// 获取方法签名,其实就是inputData的前十位
String functionCode = ethAbiCodec.getFunAbiCode(
"transfer", // 方法名
new Address(toAddress), // 参数1,值随意传,反正我们要的方法签名,不是完整的inputData
new Uint256(new BigInteger("1000000000000000000")) // 参数2,值随意传,反正我们要的方法签名,不是完整的inputData,如果还有其他参数,可以继续传入下一个
);
Contract query and write
String privateKey = ""; // 私钥
Web3j web3j = Web3j.build(new HttpService("https://data-seed-prebsc-1-s1.binance.org:8545/")); // 链的RPC地址
// 这种方式是单例的
EthContract ethContract = MagicianWeb3.getEthBuilder().getEthContract(web3j, fromAddressPrivateKey);
// 如果你想创建多个EthContract对象,可以用这种方式
EthContract ethContract = EthContract.builder(web3j, privateKey);
EthAbiCodec ethAbiCodec = MagicianWeb3.getEthBuilder().getEthAbiCodec();
// 查询
List<Type> result = ethContract.select(
contractAddress, // 合约地址
ethAbiCodec.getInputData(
"balanceOf", // 要调用的方法名称
new Address(toAddress) // 方法的参数,如果有多个,可以继续传入下一个参数
), // 要调用的方法的inputData
new TypeReference<Uint256>() {} // 方法的返回类型,如果有多个返回值,可以继续传入下一个参数
);
// 往合约里写入数据
// gasPrice,gasLimit 两个参数,如果想用默认值可以不传,或者传null
// 如果不传的话,两个参数都必须不传,要传就一起传, 如果设置为null的话,可以一个为null,一个有值
SendResultModel sendResultModel = ethContract.sendRawTransaction(
fromAddress, // 调用者的地址
contractAddress, // 合约地址
new BigInteger("1200000"), // gasPrice,如果想用默认值 可以直接传null,或者不传这个参数
new BigInteger("800000"), // gasLimit,如果想用默认值 可以直接传null,或者不传这个参数
ethAbiCodec.getInputData(
"transfer", // 要调用的方法名称
new Address(toAddress), // 方法的参数,如果有多个,可以继续传入下一个参数
new Uint256(new BigInteger("1000000000000000000")) // 方法的参数,如果有多个,可以继续传入下一个参数
) // 要调用的方法的inputData
);
sendResultModel.getEthSendTransaction(); // 发送交易后的结果
sendResultModel.getEthGetTransactionReceipt(); // 交易成功上链后的结
If you are interested in Magician, you can visit the official website: https://magician-io.com