1. What is the software development process and personnel division?
software development process
How is a piece of software developed?
demand analysis
You must first know the target group of the software, the user group, what functions it has, and what effect it wants to achieve.
It is necessary to obtain requirements specifications and product prototypes.
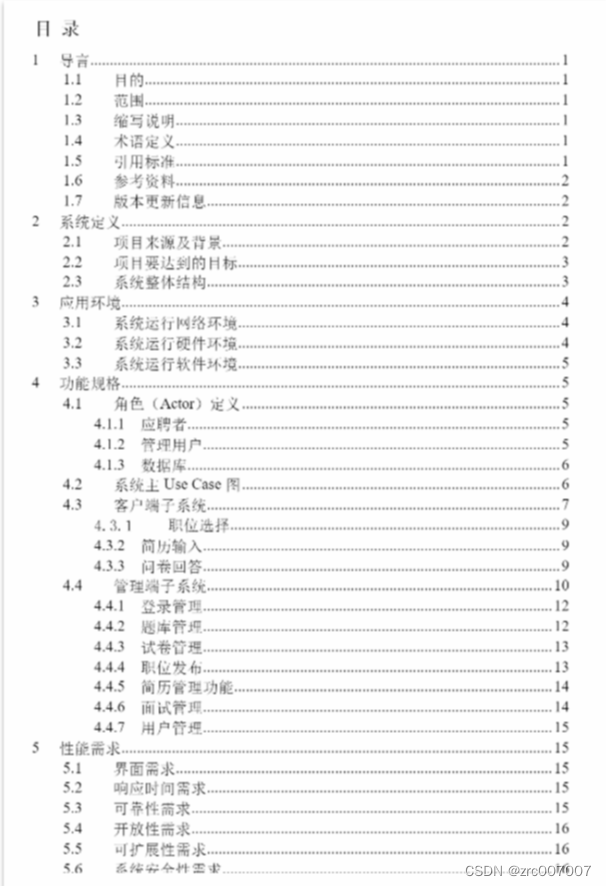
Among them, the front-end and back-end engineers should pay attention to the product prototype .
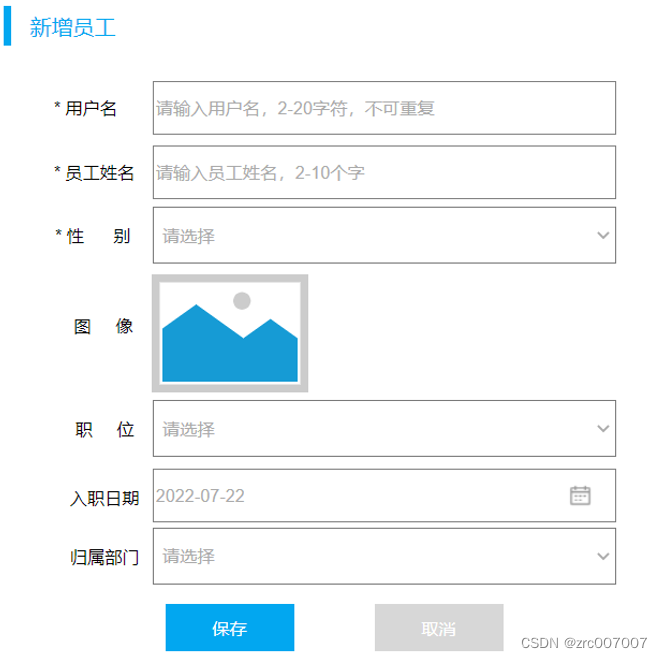
Look at this product prototype, isn't it ugly? To optimize, it depends on the UI (designer).
design
Including UI design, database design, interface design, etc.
UI design is that the prototype interface is so ugly that it cannot be made like this.
Database design is to design the structure of storing data.
Interface design is to design front-end and back-end interaction rules.
coding
Just write code.
Including project code, unit tests.
This code writing is to write according to the prototype and interface documents.
Some people will say, "Test directly with the page!"
Generally at this time, the front-end page is not seen, so joint debugging is not possible, so it is still a unit test.
test
Produce test cases, test reports, etc.
Testing includes stress testing, performance testing, etc.
For example, it took a few seconds to find the data.
For example, can the concurrency of 1k per second be resisted?
There is no problem with the test, the project is ok, and it is delivered for use.
Online operation and maintenance
Software environment installation and configuration.
Build the software operating environment.
Role division
project manager
Responsible for the overall project division and progress control.
Project management software may be used, such as Zen Tao and ones.
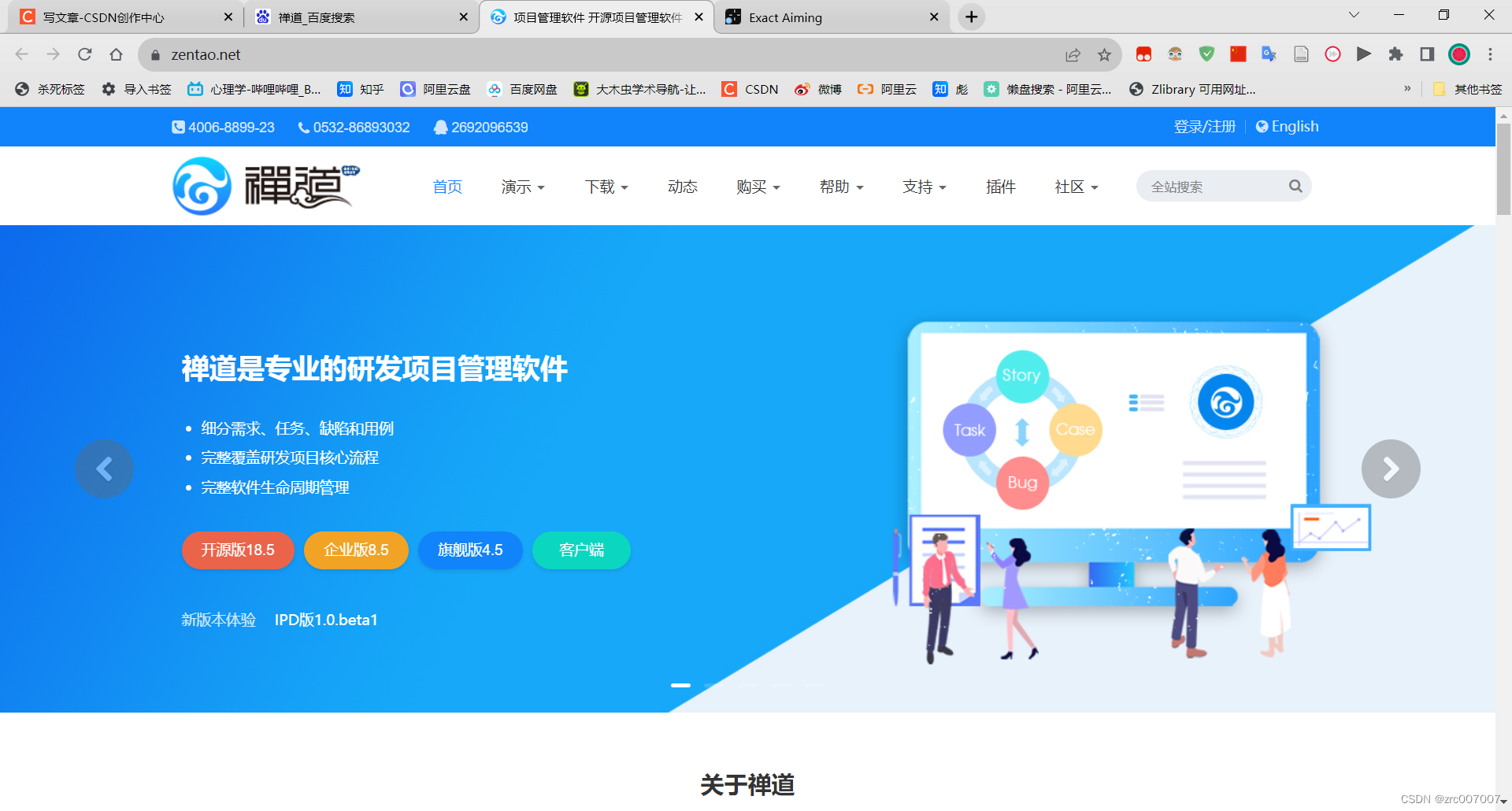
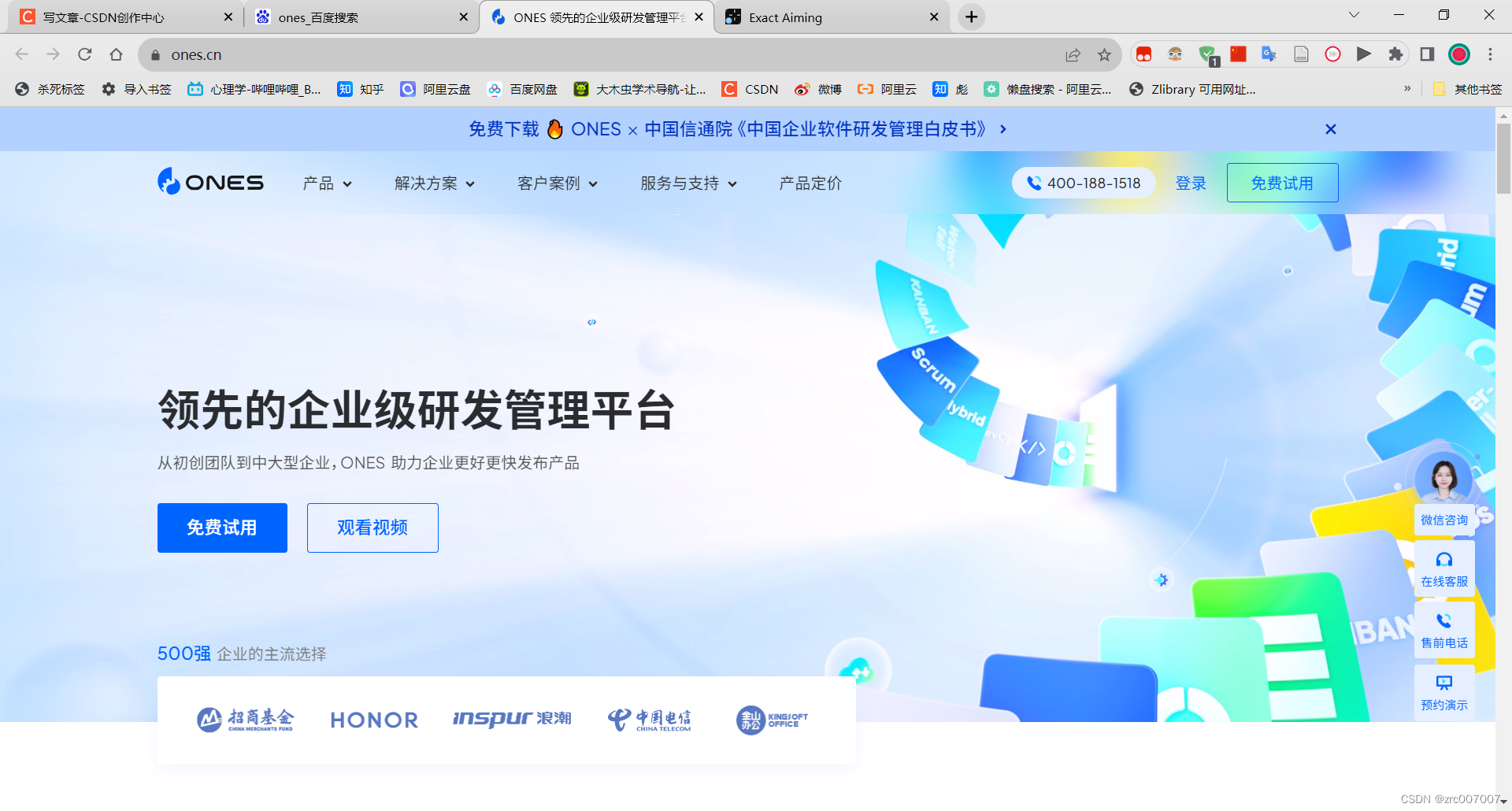
In addition to the project manager, the second most arrogant:
product manager
Conduct demand research and analysis , and output demand research documents and product prototypes .
I have more dealings with the leaders above, but it's not that leaders are better than leaders, it's just a job title. It means that I make a demand, and you come to realize it. It is a request, so generally be a little more arrogant.
(The product manager who produces the demand is the natural enemy of the programmer)
UI designer
Output the interface effect diagram according to the product prototype.
It doesn't have to be Miss Sister's.
architect
The overall structure design of the project, that is, the construction of the overall structure of the project, as well as the selection of technology.
For example, whether to choose microservices or monolithic architecture, what technology to use, what modules to design,
And some thorny issues are all architects to do.
Architects don't have to be great, there are good ones as well as good ones. Some large companies have groups, including architect groups. What’s inside is not necessarily what you can admire to the top of the mountain~
Development Engineer
Code.
Well, here comes the hard-working coder.
Test Engineer
Write test cases and output test reports.
Operation and Maintenance Engineer
Build the software environment and run the project.
other
There may be other refinement directions in the company, such as DBA, that is, database administrator.
They just write SQL statements.
Special case
Ideally, there are all the positions above. But when you enter the company, you will find that there are always a few full-time positions that are lacking, and these subdivided jobs are done part-time by other people.
minimum configuration
There are two types of people. One type of person is a programmer who writes codes, and is responsible for development, testing, operation and maintenance, and even architecture. There may be UI, or the UI may be outsourced.
The second type of people also serve as project managers and product managers. This configuration has advantages and disadvantages compared to dividing two people. The advantage is that he or she is technically savvy and you can go chat with him. (Some product managers can’t realize the function because they are not good at technology. You can search and identify the color of the phone case to fight , and experience the love and hatred between programmers and products.) The downside is that they dare not hate him. First, he is knowledgeable. Second, he is the leader, how dare you hate him? [manual funny]
2. What modules are there in the backend project? What's in each module?
There are 4 modules in total, namely the parent module sky-take-out, the entity module sky-pojo, the public resource module sky-common, and the business code module sky-server.
Parent module sky-take-out
The parent project manages dependency versions, aggregates other modules, and realizes unified management of modules.
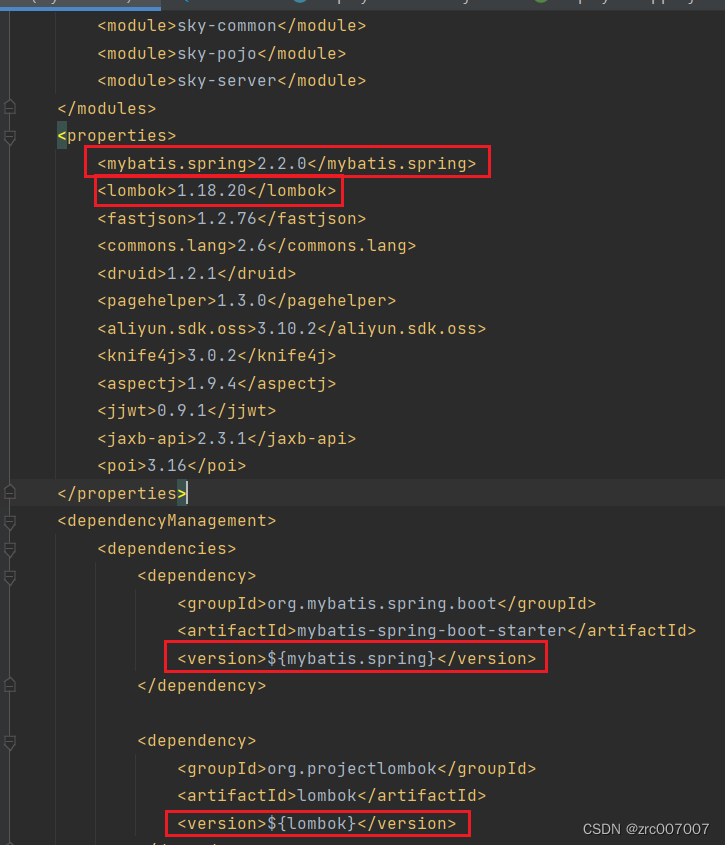
Entity module sky-pojo
Including the following 3 types:
Entity class POJO or Entity. Ensure that the attribute names correspond to the field names of the database table one-to-one.
The data transfer object DTO encapsulates the request parameters passed from the front end.
The view object VO encapsulates the response data that the front end needs to receive.
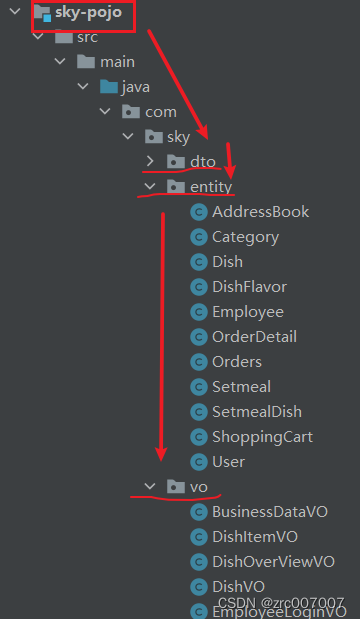
Common resource module sky-common
Store public resources, such as tool classes, constant classes, enumeration classes, exception classes, etc.
Business code module sky-server
Store business code, such as the code of the presentation layer, business layer, and persistence layer in the three-tier architecture.
3. Describe clearly what the login process is like.
As shown below:
4. What are reverse proxy and load balancing?
reverse proxy
The front end sends the request to nginx, and nginx sends the request to the backend server.
load balancing
One of the 3 benefits of reverse proxy. Details below.
3 Benefits of Reverse Proxy
1. Improve access speed
Because nginx can store access data cache, for example, if there are 10 identical accesses, nginx can return the cache to the front end, and only need to return to the back end once instead of accessing the back end 10 times.
2. Perform load balancing
When there is a server cluster, the front end sends it to nginx, and nginx selects a deployment strategy to selectively deploy servers, which is called load balancing.
3. Ensure the security of back-end services
Directly exposing the back-end port has the risk of being attacked; while only exposing nginx, nginx will indirectly call the server, which ensures the security of the back-end service.
Code
In nginx, in nginx.conf under the conf folder:
server {
listen 80;
server_name localhost;
....
}
It means that nginx is listening on port 80.
Then look below:
server {
listen 80;
server_name localhost;
location / {
root html/sky;
index index.html index.htm;
...
}
}
The location tells the program that if you access the written front-end pages, they will be placed in the html/sky folder. Take a look, indeed.
Read on:
server {
listen 80;
server_name localhost;
...
# 反向代理,处理管理端发送的请求
location /api/ {
proxy_pass http://localhost:8080/admin/;
#proxy_pass http://webservers/admin/;
}
...
}
The code here means that as long as the resource under the api path is accessed, it will be automatically reverse-proxyed to the localhost:8080/admin/ path.
Continue to load balancing :
server {
listen 80;
server_name localhost;
...
# 反向代理,处理用户端发送的请求
location /user/ {
proxy_pass http://webservers/user/;
}
upstream webservers{
server 127.0.0.1:8080 weight=90 ;
server 127.0.0.1:8088 weight=10 ;
}
}
The above code is the resource of the reverse proxy/user path. Proxy to the path under webservers. This webservers can be used as an alias.
Then look at this webservers, load balancing is used here. For the path of 127.0.0.1:8080, assign a weight of 90; for the path of 127.0.0.1:8088, assign a weight of 10.
For load balancing, there are different strategies worth talking about.
Different Strategies for Load Balancing
polling
default method. That is, one person once.
If the above is polling, it is written as follows:
upstream webservers{
server 127.0.0.1:8080;
server 127.0.0.1:8088;
}
weight
Weight method, the default is 1, the higher the weight, the more client requests are allocated. The weight can be regarded as the probability assigned to the address. For example, the following experiment:
weight experiment
In the configuration file of nginx, the inside of the nginx.conf file is as follows:
upstream testservers {
server 127.0.0.1:8080 weight=4;
server 127.0.0.1:8081 weight=1;
}
server {
listen 80;
server_name localhost;
... # 省略其他配置
# 自定义反向代理
location /testPort/ {
proxy_pass http://testservers/test/;
}
...
}
It can be seen that when we set access to resources under /testPort, it will automatically jump to the path under /test resources under 8080 and 8081. The weights of ports 8080 and 8081 are 4 and 1 respectively.
Write a test controller class on the backend:
package com.sky.controller.admin;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.time.LocalDateTime;
@RestController
@RequestMapping("/test")
@Slf4j
@Api(tags = "负载均衡接口使用测试")
public class TestPortController {
@Value("${server.port}")
private String port;
@ApiOperation("测试端口")
@GetMapping("/port")
public String port() {
return port + " " + LocalDateTime.now();
}
}
As you can see, the port number and access time will be returned.
Add the release code to the interceptor (com.sky.interceptor.JwtTokenAdminInterceptor):
if (1==1) {return true;}
The default port is 8080, start it. Then create a startup class and set the port to the new 8081 with the system property parameter. Then start.
Then start nginx and start testing:
Enter the address (you can think about why you lost it like this)
http://localhost/testPort/port
Look at the result:
Basically a 4:1 odds.
Verification succeeded.
5. What is the difference between Swagger and yapi?
yapi is an online project interface management tool that requires networking;
Swagger is a locally deployed interface management tool.