Table of contents
Hill sort
concept
Hill sort is a kind of insertion sort, which is an optimization of direct insertion sort. Its characteristic lies in grouping and sorting.
Algorithm ideas
Hill sorting is named after its designer Hill, who analyzed the efficiency of insertion sorting and came to the following conclusions:
1. In the worst case, when the sequence to be sorted is in reverse order, it takes O(n^2) time
2. In the best case, when the sequence to be sorted is in order, it takes O(n) time
So Hill thought: If the sequence to be sorted can be pre-sorted first, so that the sequence to be sorted is close to order, and then an insertion sort is performed on the sequence. Therefore, the time complexity of direct insertion sorting is O(n) at this time , so it is only necessary to control the time complexity of the pre-sorting stage to be less than O(n^2) , then the overall time complexity is lower than that of insertion sorting .
So how should the specific pre-sorting be done so that the time complexity can meet the requirements?
1. First select an integer gap smaller than n (generally, n/2 is used as gap) as the first increment, and then group all elements whose distance is gap into a group, and perform insertion sort on each group
2. Repeat step 1 until the gap is equal to 1 and stop. At this time, the entire sequence is divided into one group, and a direct insertion sort is performed, and the sorting is completed.
You may be wondering why the gap is from large to small?
Because the larger the gap, the faster the data moves and takes less time; the smaller the gap, the slower the data moves and takes more time. Make the gap larger in the early stage, so that the data can be quickly moved to the vicinity of its corresponding position, reducing the number of times of movement.
animation
Let's take an example:
First, the gap is 5. At this time, the elements with a distance of 5 are divided into one group (a total of five groups, each with two elements), and then each group is inserted and sorted separately.
The gap is halved to 2. At this time, the elements with a distance of 2 are divided into one group (a total of two groups with five elements in each group), and then each group is inserted and sorted separately.
The gap is halved again to 1. At this time, all elements are divided into one group, and it is inserted and sorted, and the insertion sort is completed.
In this example, the first two trips are the pre-sorting of Hill sorting, and the last trip is the insertion sorting of Hill sorting
code show as below
public static void shellSort(int[] a){
int gal = a.length;
while(gal>1) {
int j = 0;
gal/=2; //特别之处gal 分组排序 5 3 1.。。
//核心
for (int i = gal; i < a.length; i++) {
j = i-gal;
if(a[j] > a[i]) {
int tmp = a[j];
a[j] = a[i];
a[i] = tmp;
}
}
}
}
Complexity Analysis
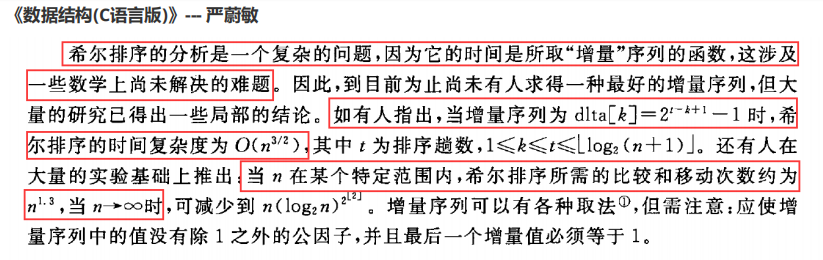
"Data Structure - Description with Object-Oriented Method and C++ " --- Yin Renkun
Time complexity n^1.3 -- n^1.5 Can't tell. It is related to the number of gaps in each group.
Space complexity O(1)
Stability Unstable When there are several identical numbers, the relative position will change after sorting
time complexity test
Next, let's try to test it with a lot of data.
int[] a = new int[10_0000]; //100,000 data test
1. The orderArray function is implemented to generate a basic ordered sequence, that is, arranged from small to large.
public static void orderArray(int[] a) {
for (int i = 0; i < a.length; i++) {
a[i] = i;
}
}
2. The notOrderArray function generates a reverse sequence, that is, arranged from largest to smallest.
public static void notOrderArray(int[] a) {
for (int i = 0; i < a.length; i++) {
a[i] = a.length-i;
}
}
3. The randomArray function generates a random unordered array.
public static void randomArray(int[] a) {
Random random = new Random();
for (int i = 0; i < a.length; i++) {
a[i] = random.nextInt(10_0000);
}
}
4. The testInsertSort function tests the return value of System.currentTimeMillis() in milliseconds .
public static void testInsertSort(int[] a){
int[] tmpArray = Arrays.copyOf(a,a.length);
long startTime = System.currentTimeMillis(); //注意用long接收
shellSort(tmpArray);
long endTime = System.currentTimeMillis(); //返回单位是毫秒
System.out.println("直接插入排序耗时:"+(endTime-startTime));
}
5. Main function call execution
public static void main(String[] args) {
int[] a = new int[10_0000];
//有序
System.out.println("基本有序数列");
orderArray(a);
testInsertSort(a);
//倒序
System.out.println("逆序数列");
notOrderArray(a);
testInsertSort(a);
//随机乱序
System.out.println("无序数列");
randomArray(a);
testInsertSort(a);
}
operation result
Both Hill sort and direct insertion sort are insertion sorts, and Hill sort is an optimization of direct insertion sort. Compare the test results of the direct insertion sort above .
It can be seen that Hill sort is indeed much faster. And time-consuming and stable.
full code
import java.util.Random;
public class sort {
public static void main(String[] args) {
int[] a = new int[10_0000];
//有序
System.out.println("基本有序数列");
orderArray(a);
testInsertSort(a);
//无序
System.out.println("逆序数列");
notOrderArray(a);
testInsertSort(a);
//乱序
System.out.println("无序数列");
randomArray(a);
testInsertSort(a);
}
//希尔排序 是插入排序的优化
//时间复杂度 n^1.3 -- n^1.5 说不准 与每次分组的个数有关
//空间复杂度 O(1)
//稳定性 不稳定 当有几个相同的数字时,排序后相对位置会改变
public static void shellSort(int[] a){
int gal = a.length;
while(gal>1) {
int j = 0;
gal/=2; //特别之处gal 分组排序 5 3 1.。。
//核心
for (int i = gal; i < a.length; i++) {
j = i-gal;
if(a[j] > a[i]) {
int tmp = a[j];
a[j] = a[i];
a[i] = tmp;
}
}
}
}
//生成有序数组 从小到大排列
public static void orderArray(int[] a) {
for (int i = 0; i < a.length; i++) {
a[i] = i;
}
}
//n无序 其实就是从大到小排列
public static void notOrderArray(int[] a) {
for (int i = 0; i < a.length; i++) {
a[i] = a.length-i;
}
}
//乱序 随机生成序列
public static void randomArray(int[] a) {
Random random = new Random();
for (int i = 0; i < a.length; i++) {
a[i] = random.nextInt(10_0000);
}
}
//大量数据测试
public static void testInsertSort(int[] a){
int[] tmpArray = Arrays.copyOf(a,a.length);
long startTime = System.currentTimeMillis(); //注意用long接收
shellSort(tmpArray);
long endTime = System.currentTimeMillis();
System.out.println("希尔排序耗时:"+(endTime-startTime));
}
}