The previous article recorded the content related to front-end authentication. This note mainly records the content related to the request header, including a request message analysis of HTTP, fastapi setting cookie and parameters, fastapi setting request header parameters, etc.
HTTP Request
The HTTP request message is divided into three parts: request line, request header and request body:
request line
The request line has three parts: request method, request address, and protocol version. Generally, a request is sent through the request line
request header
The request header is generally a number of attributes, all of which are key-value pairs. The server obtains the basic information of the client through the request header.
request body
Everyone is familiar with the request body, which is the data sent by the client to the server.
Cookie parameters
Like the previous setting of path parameters and query parameters, you can use the methods in fastapi to define cookie parameters.
But before defining cookie parameters, cookie parameters are required to test. We know from the previous chapter that cookies are responded to by the server to the front end, so we need to send a cookie to the front end first.
@app05.get("/stu05/setcookie")
def get_setcookie(response:Response):
response.set_cookie("cookie1","cookie111111111111")
response.set_cookie("cookie2","cookie222222222222")
response.set_cookie("cookie3", "cookie333333333333")
return {
"result":"设置cookie成功"}
Here, the response method of fastapi is used to send a set_cookie to the front-end. After the front-end receives the response, there is a cookie. The specific operation and detailed application will be described in detail in a chapter later. Now it is mainly to test the received cookie parameters.
Then we visit a link at random, and let's create hello world by default! You will find that the cookie already exists.
Define cookie parameters
@app05.get("/stu05/getcookie")
def stu05_read_cookie(
cookie1:Optional[str] = Cookie(None),
cookie2:Optional[str] = Cookie(None)
):
return {
"cookie1":cookie1,"cookie2":cookie2} # 定义Cookie参数需要使用Cookie类,否则就是查询参数
With the cookie, you can receive the cookie parameter through the fastapi method, declare a cookie parameter with the cookie, and then return the received cookie parameter! Since there is already a cookie, you can find that the request has been completed by testing directly in the api document!
Header parameters
Before that, let's briefly understand the difference between header and headers:
The header corresponds to the body, which is the header part of the request or response, and is a plurality of text lines separated by CRLF.
There are two consecutive CRLFs after the header, and the part after that is the body. Usually GET has no body.
headers refers to multiple lines of text in the header paragraph.headers is the content in the header.
@app05.get("/stu05/getheader")
def stu05_read_header(
user_agent:Optional[str] = Header(None)
):
return {
"User-agent":user_agent}
Like other parameters, you can declare a Header parameter with the Header class of fastapi. The above user_agent is a Header parameter. It will also be parsed as a query parameter without the Header class declaration.
automatic conversion
Most standard headers are '-'
separated by a "hyphen", that is, a minus sign, eg user-agent
, but such variables are invalid in Python. Therefore, you cannot use the exact same writing method when defining parameters in python. In this case, FastAPI Header
will convert the characters of the parameter name from underscores ( _
) to hyphens ( -
) by default to extract and record headers
;
At the same time, HTTP headers
are case-insensitive, so they can be declared using standard Python style, that is, can be used as usual in Python code user_agent
, without the need to capitalize the first letter as User_Agent
or similar;
Of course, FastAPI also provides the function of disabling underscore conversion, that is, it can be set under the corresponding parameters convert_underscores=False
, as follows, but some HTTP proxies and servers do not allow the use of underscores headers
, so it may cause the situation that the information cannot be received, so the default is True
can;
user_agent:Optional[str] = Header(None,convert_underscores=False)
Duplicate headers
In practice, it is entirely possible to receive duplicate headers. That is, a parameter under the same header has multiple values.
For this case, use a list directly in the type declaration to define these cases.
@app05.get("/stu05/getheaders")
def stu05_read_headers(
paramlist:Optional[List[str]] = Header(None)
):
return {
"paramlist":paramlist}
The above code declares a
List
typeHeader
parameter, and then Postman can more directly display what the repeated Headers mean, as follows:
source code
# -*- coding: utf-8 -*-
# @Time: 2022/11/28 18:27
# @Author: MinChess
# @File: stu05.py
# @Software: PyCharm
from fastapi import APIRouter,Response,Cookie,Header
from pydantic import BaseModel
from typing import Optional,List
app05 = APIRouter()
@app05.get("/stu05/setcookie")
def get_setcookie(response:Response):
response.set_cookie("cookie1","cookie111111111111")
response.set_cookie("cookie2","cookie222222222222")
response.set_cookie("cookie3", "cookie333333333333")
return {
"result":"设置cookie成功"}
@app05.get("/stu05/getcookie")
def stu05_read_cookie(
cookie1:Optional[str] = Cookie(None),
cookie2:Optional[str] = Cookie(None)
):
'''
:param cookie1:
:param cookie2:
:return:
'''
return {
"cookie1":cookie1,"cookie2":cookie2} # 定义Cookie参数需要使用Cookie类,否则就是查询参数
@app05.get("/stu05/getheader")
def stu05_read_header(
user_agent:Optional[str] = Header(None)
):
return {
"User-agent":user_agent}
@app05.get("/stu05/getheaders")
def stu05_read_headers(
paramlist:Optional[List[str]] = Header(None)
):
return {
"paramlist":paramlist}
Thanks for reading!
Blog link: FastAPI from entry to actual combat (9) - setting Cookie and Header parameters
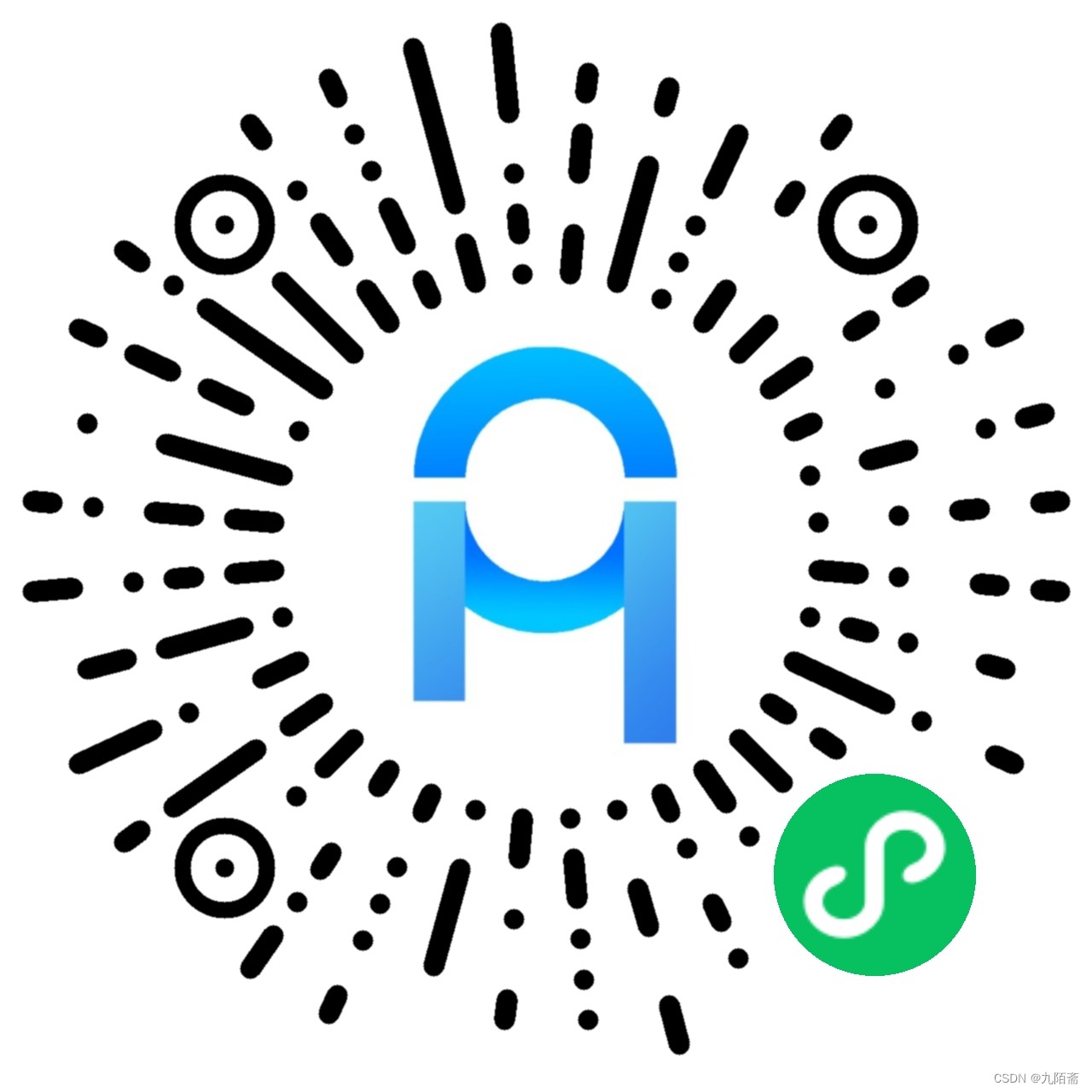