This article mainly records the data request of the form and related content such as uploading files of different sizes, uploading multiple files, and obtaining file information.
form request
@app07.post("/stu07/form")
def stu07_form(
param1: str = Form(),
param2: str = Form(),
param3: int = Form()
):
return {
"param1": param1,
"param2": param2,
"param3": param3
}
Consistent with the path parameters and query parameters, use the built-in Form method of fastapi to declare;
Upload a bytes file
@app07.post("/stu07/files")
def stu07_files(
file: bytes = File()
):
return {
"files_size": len(file)
}
Upload files are uploaded in the form of Form, and the File in the above code is also inherited from Form;
If the parameter type of the path operation function is declared as
bytes
, FastAPI will read and receive the file content in bytes.This method stores all the contents of the file in memory and is suitable for small files.
Upload files using UploadFile
@app07.post("/stu07/uploadfile/")
def stu07_uploadfile(
file: UploadFile
):
return {
"文件名": file.filename,
"内容类型": file.content_type
}
UploadFile
Compared withbytes
, it has more advantages; it is more suitable for processing large files such as images, videos, binary files, etc., and the advantage is that it does not take up all the memory;You
UploadFile
can also use attributes to get related information directly:
filename
: upload file name string (str), for example,myimage.jpg
;content_type
: content-type (MIME-type/media-type) string (str), eg,image/jpeg
;file
:SpooledTemporaryFile
(file-like
object). In fact, it isPython
a file that can be passed directly to otherfile-like
functions or support libraries that expect objects.UploadFile supports the following async methods , which (use internally
SpooledTemporaryFile
) call the corresponding file methods.
write(data)
:data (str 或 bytes)
write to the file;read(size)
(size (int))
: Read the file content by the specified number of bytes or characters ;seek(offset)
: move tooffset (int)
the position of the file byte;
- For example,
await myfile.seek(0)
move to the beginning of the file;await myfile.read()
This method is especially useful when you need to read the read content again after executing ;close()
: Close the file.Note :
The above methods are all
async
methods and should be used with "await";For example, in
async
the path operation function, you need to read the content in the following waycontents = await myfile.read()
In ordinary
def
path operation functions, you can directly accessUploadFile.file
contents = myfile.file.read()
Optional file upload
@app07.post("/stu07/optionalfile")
def stu07_optional_file(
file: Optional[bytes] = File(None)
):
if not file:
return {
"message": "未上传文件"}
else:
return {
"filesize": len(file)}
Also use Optional or Union to set it;
set metadata
@app07.post("/stu07/uploadfile/metadata/")
def stu07_uploadfile_metadata(
file: UploadFile = File(None, description="一个UploadFile文件")
):
if not file:
return {
"message": "未上传文件"}
else:
return {
"filename": file.filename}
This part of the content is consistent with the path parameters and query parameters, and can be set according to the requirements;
Upload multiple files
@app07.post("/stu07/fileslist")
def stu07_files_list(
byteslist: List[bytes] = File(...),
uploadfilelist: List[UploadFile] = File(...)
):
return {
"bytes_files_size": [len(file) for file in byteslist],
"upload_filesname": [file.filename for file in uploadfilelist]
}
To upload multiple files, set the corresponding parameter to
List
type;
Upload form parameters and files at the same time
@app07.post("/stu07/form_file/")
def stu07_form_file(
file: UploadFile,
form: str = Form()
):
return {
"filename": file.filename,
"form": form
}
Mixed upload, that is, declare different parameters in the corresponding path function;
source code
# -*- coding: utf-8 -*-
# @Time: 2022/11/30 18:09
# @Author: MinChess
# @File: stu07.py
# @Software: PyCharm
from fastapi import APIRouter, Form, File, UploadFile
from typing import List, Optional
from fastapi.encoders import jsonable_encoder
from pydantic import BaseModel
from datetime import datetime
app07 = APIRouter()
# 一个form请求
@app07.post("/stu07/form")
def stu07_form(
param1: str = Form(),
param2: str = Form(),
param3: int = Form()
):
return {
"param1": param1,
"param2": param2,
"param3": param3
}
# 上传一个bytes类型的文件
@app07.post("/stu07/files")
def stu07_files(
file: bytes = File()
):
return {
"files_size": len(file)
}
# 使用UploadFile
@app07.post("/stu07/uploadfile/")
def stu07_uploadfile(
file: UploadFile
):
return {
"文件名": file.filename,
"内容类型": file.content_type
}
# 可选文件上传
@app07.post("/stu07/optionalfile")
def stu07_optional_file(
file: Optional[bytes] = File(None)
):
if not file:
return {
"message": "未上传文件"}
else:
return {
"filesize": len(file)}
# 设置UploadFile元数据
@app07.post("/stu07/uploadfile/metadata/")
def stu07_uploadfile_metadata(
file: UploadFile = File(None, description="一个UploadFile文件")
):
if not file:
return {
"message": "未上传文件"}
else:
return {
"filename": file.filename}
# 多文件上传
@app07.post("/stu07/fileslist")
def stu07_files_list(
byteslist: List[bytes] = File(...),
uploadfilelist: List[UploadFile] = File(...)
):
return {
"bytes_files_size": [len(file) for file in byteslist],
"upload_filesname": [file.filename for file in uploadfilelist]
}
# 同时上传表单和文件
@app07.post("/stu07/form_file/")
def stu07_form_file(
file: UploadFile,
form: str = Form()
):
return {
"filename": file.filename,
"form": form
}
Thanks for reading!
Jiumozhai address: https://blog.jiumoz.com/archives/fastapi-cong-ru-men-dao-shi-zhan-biao-dan-qing-qiu-yu-shang-chuan-wen-jian
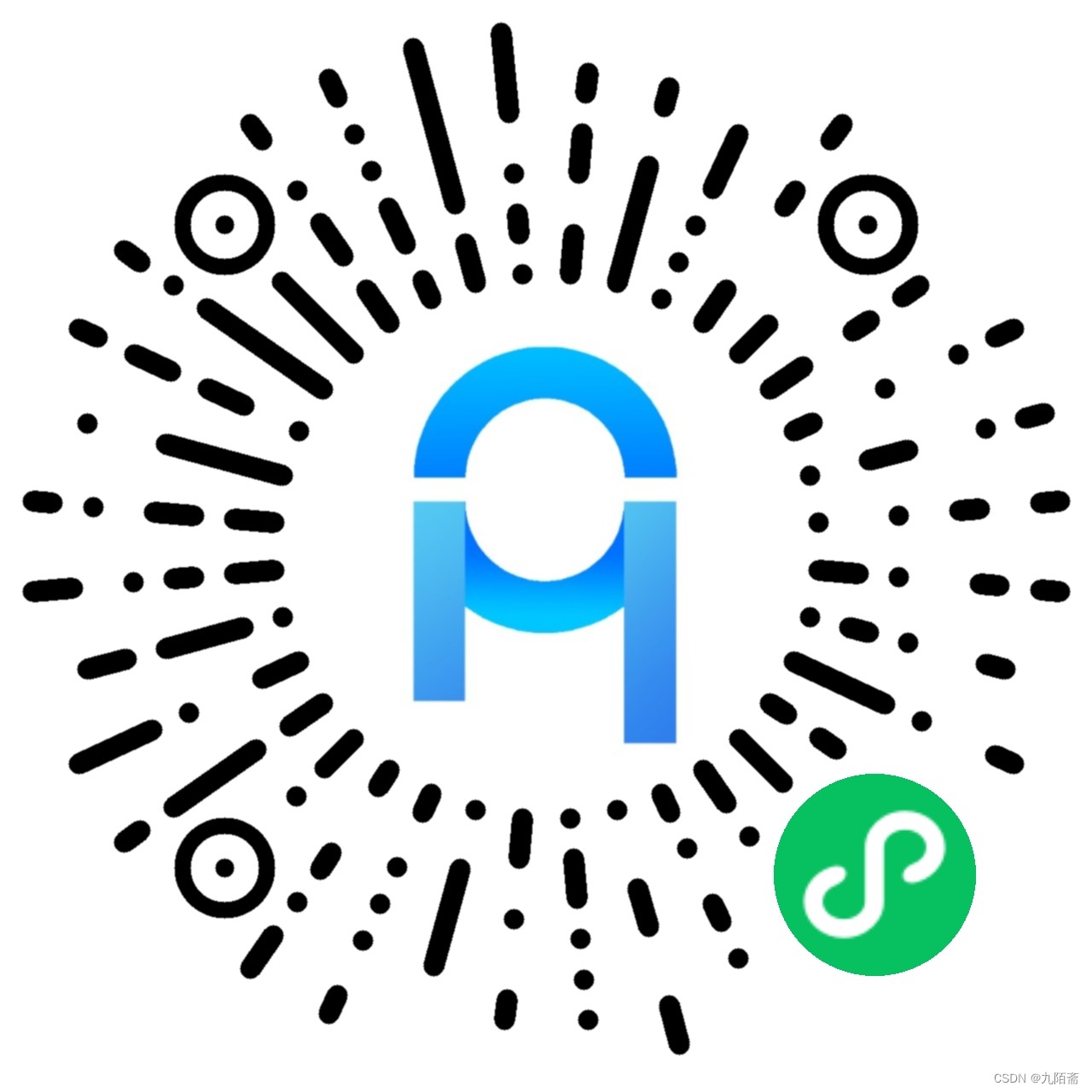