Premise: To sign an unsigned apk, you can first check whether the apk is signed
keytool -printcert -jarfile your_app.apk
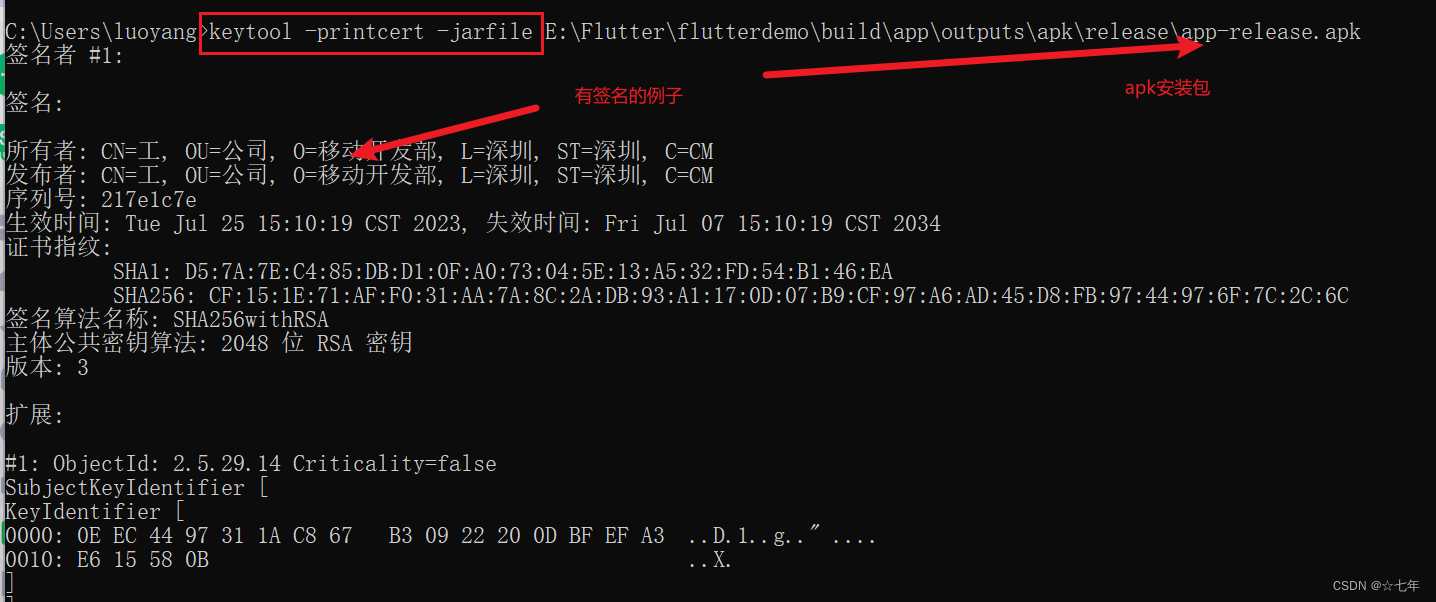
Method 1. Manual signature
1. Generate a key file, select a file directory, and enter the following command on the cmd command line
keytool -genkey -v -keystore test.keystore -alias YYY -keyalg RSA -validity 4000
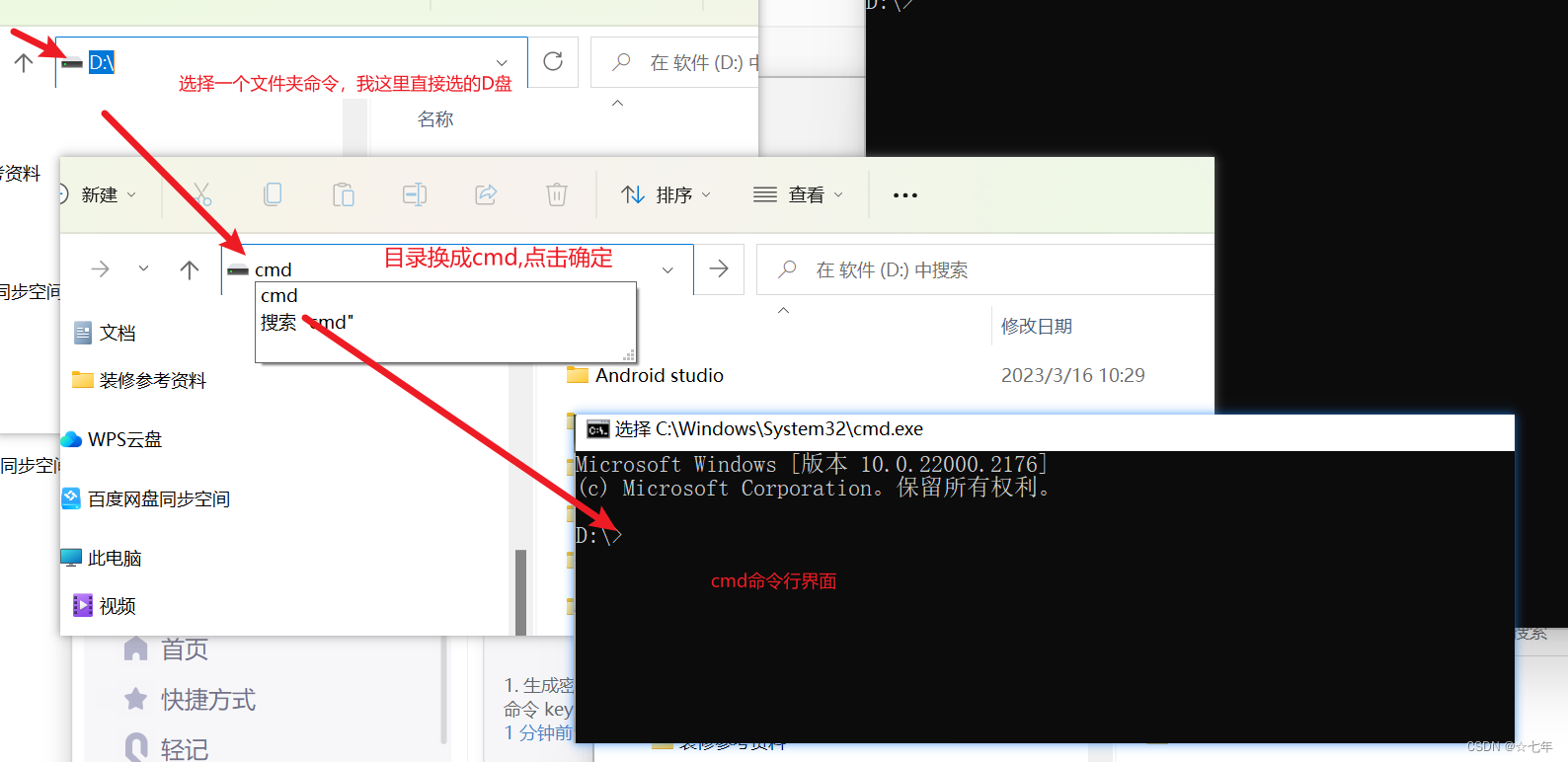
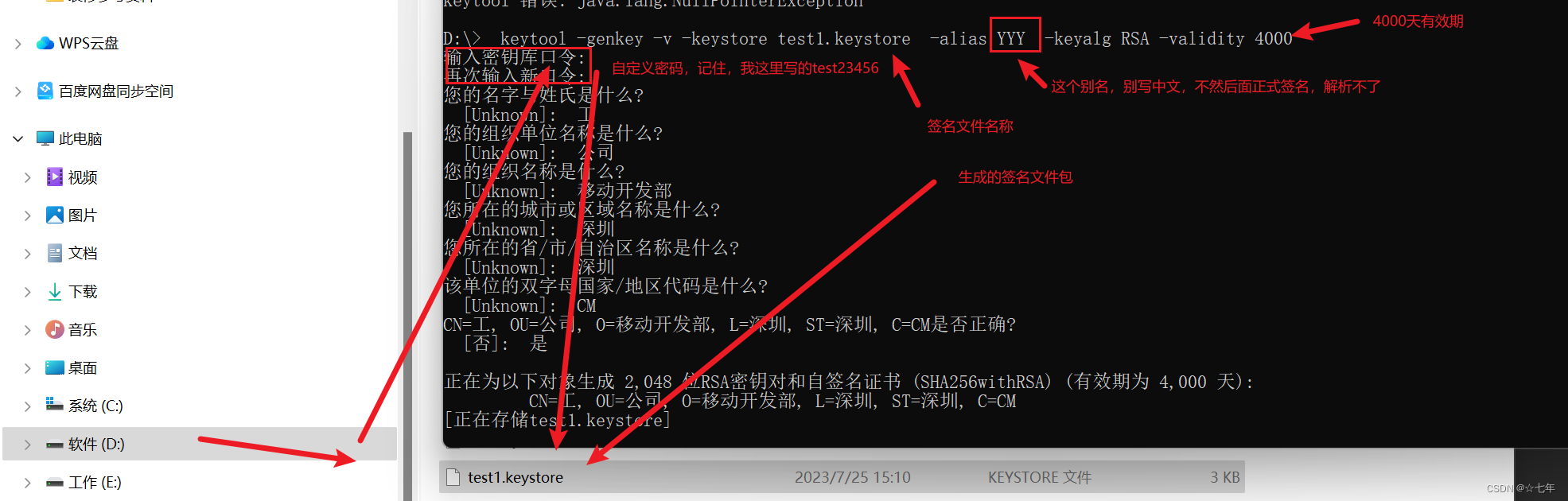
2. View certificate information
keytool -list -v -keystore test.keystore
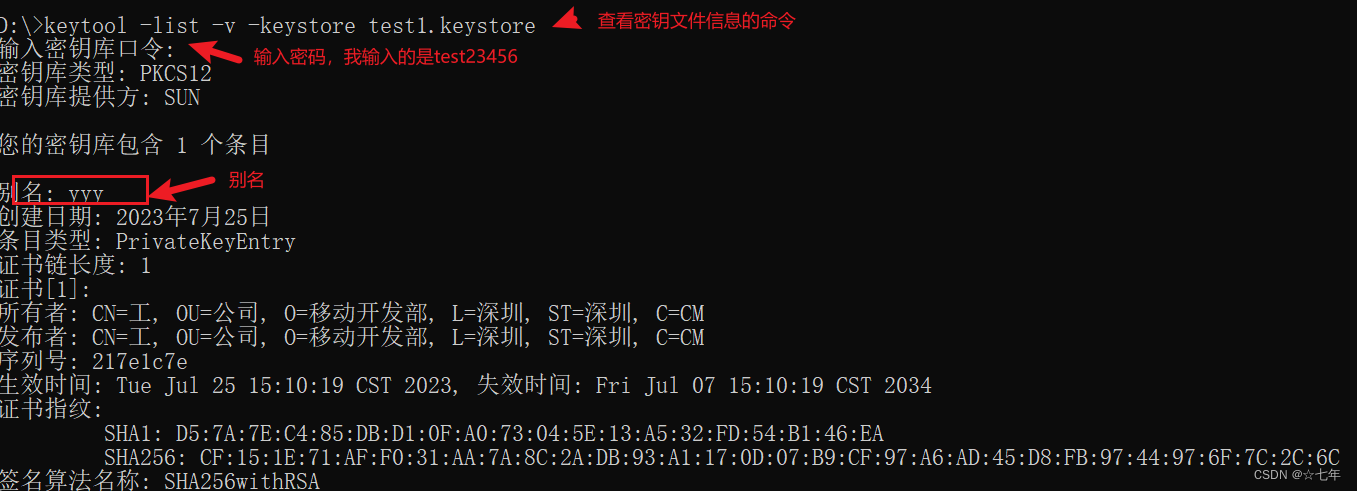
3. Start signing
jarsigner -verbose -keystore E:\xxx\test.keystore -signedjar
xxxx签名后的xxx.apk D:\xxx\未签名的xxx.apk testalias
如下 :jarsigner -verbose -keystore D:\test1.keystore -signedjar flutter_demo.apk
E:\Flutter\flutterdemo\build\app\outputs\flutter-apk\app-release.apk YYY
Method 2. Configure automatic signature
The following takes the Android of the Flutter project as an example
To configure APK signing in your Flutter project, you can follow these steps:
1. Create a sign folder in the root directory of the Android project, and copy your keystore file (.keystore) to this directory.
2. Create a file named `key.properties`
Make sure that the keystore file name specified in `key.properties` is the same as the key file name, add the following content to the file and replace it with the real value:
```properties
storePassword=your_store_password
keyPassword=your_key_password
keyAlias=your_key_alias
storeFile=your_keystore_file.jks
# If you are building different build types (for example, debug and release) and need to provide different configurations, you can create the following configurations:
# storePassword.debug=debug_store_password
# keyPassword.debug=debug_key_password
# keyAlias.debug=debug_key_alias
# storeFile.debug=debug_keystore_file.jks
#
# storePassword.release=release_store_password
# keyPassword.release=release_key_password
# keyAlias.release=release_key_alias
# storeFile.release=release_keystore_file.jks
- `your_store_password`: Password for the keystore file.
- `your_key_password`: The password for the key.
- `your_key_alias`: The alias for the key.
- `your_keystore_file.jks`: The name of the keystore file.
If you need to provide different configurations for different build types (for example, debug and release), uncomment the corresponding lines and provide appropriate values for each build type.
3. In the `android/app/build.gradle` file of the Flutter project, find the `android` block, and add the following code in it:
```groovy
//Declaration to obtain the signature configuration configuration file path
def signProperties = rootProject.file("sign/keystore.properties")
def props = new Properties()
props.load(new FileInputStream(signProperties))
def file = file(props['storeFile'])
android {
signingConfigs {
release {
//Sign if the keystore file and configuration file exist, otherwise do not sign
if (file.exists() && signProperties.exists()) {
keyAlias props['keyAlias']
keyPassword props['keyPassword']
storeFile file
storePassword props['storePassword']
}
}
}
// ...
buildTypes {
release {
// obfuscation
minifyEnabled true
//Remove useless res files
shrinkResources true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
//Use signature file
signingConfig signingConfigs.release
}
}
}
```
This will read the value you configured in `key.properties` and apply the signing configuration in the `release` build type.
Note, make sure to replace `keyAlias`, `keyPassword`, `storeFile` and `storePassword` with your own actual values.
After completing these steps, your Flutter project will have APK signing configured.
When building release builds, the Android build system will sign with the specified keystore and password.
4. Compile signed apk
#You can generate a signed APK file by running the `flutter build apk` command,
It will be located under `build/app/outputs/flutter-apk/app-release.apk` path (for release build type).
#If it is a native Android project, you can find build/build in Gradle and compile the signed apk
Note: 1. Before using the APK signature, please ensure the security of the keystore file and related passwords, and back up important files and password information to prevent loss or disclosure. 2. After the compilation is successful, you can go back to the beginning of the article to verify whether the signature is successful.
Refer to the packaging signature after decompilation: apk decompilation and repackaging process_apk decompilation and repackaging_☆Seven Years Blog-CSDN Blog