Computer Vision
Histogram, Gaussian Filter, Histogram Equalization
1. Histogram
1. Basic principles
How it works:
A histogram is an accurate graphical representation of the distribution of numerical data. It is an estimate of the probability distribution of a continuous variable (quantitative variable) and was first introduced by Karl Pearson. It is a type of bar graph.
To build a histogram, the first step is to segment the range of values, that is, divide the entire range of values into a series of intervals, and then count how many values are in each interval. The values are usually specified as consecutive, non-overlapping variable intervals. The intervals must be adjacent and usually (but not necessarily) of equal size.
Functions:
1. Display the status of quality fluctuations;
2. Intuitively transmit information about the quality status of the process;
3. After people have studied the fluctuation status of quality data, they can grasp the status of the process, so as to determine where to concentrate their efforts Quality improvement work.
2. Related code
from numpy import array
from PIL import Image
#from matplotlib.pyplot import *
from pylab import *
#import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif'] = 'SimHei'
plt.rcParams['axes.unicode_minus'] = False
im = array(Image.open('E:/Pythonproject/2021.png').convert('L')) # 打开图像,并转成灰度图像
print(im)
figure()
subplot(121)
gray()
contour(im, origin='image') # 画图
axis('equal') # 自动调整比例
axis('on') # 去除x、y轴上的刻度
title(u'原图轮廓')
subplot(122)
hist(im.flatten(), 128)
print(im.flatten())
title(u'直方图')
plt.xlim([0, 300])
plt.ylim([0, 11000])
show()
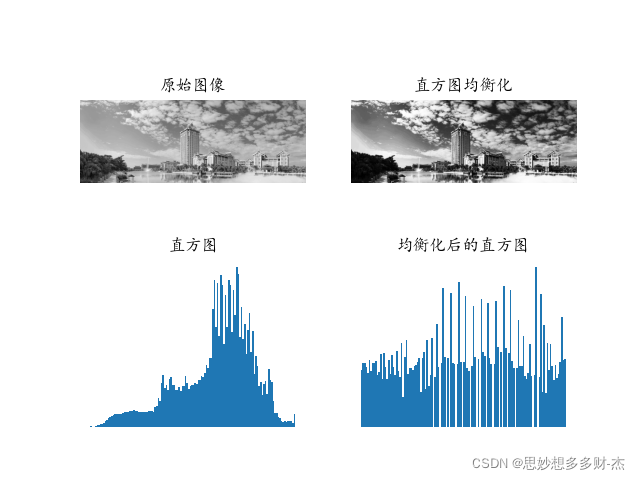
3. Results display
2. Gaussian filter
1. Basic principles
The Gaussian filter is a linear smoothing filter, which has a good suppression effect on the noise that obeys the normal distribution. In actual scenes, we usually assume that the noise contained in the image is Gaussian white noise, so in the preprocessing part of many practical applications, Gaussian filtering is used to suppress noise.
Gaussian filtering, like mean filtering, uses a mask and image for convolution. The difference is that the template coefficients of the mean filter are all the same, which is 1. The template coefficient of the Gaussian filter, as the distance from the center of the template increases, the coefficient decreases (obeys the two-dimensional Gaussian distribution). Therefore, compared with the mean filter, the Gaussian filter blurs the image less and can better maintain the overall details of the image.
2. Related code
import cv2
import matplotlib.pyplot as plt
from matplotlib.pyplot import title
import matplotlib
im = cv2.imread("E:/Pythonproject/2021.png")
# 高斯滤波
img_Guassian = cv2.GaussianBlur(im, (5, 5), 0)
plt.subplot(121)
plt.imshow(im)
# 设置字体为楷体
matplotlib.rcParams['font.sans-serif'] = ["KaiTi"]
title(u'原图')
plt.subplot(122)
plt.imshow(img_Guassian)
title(u'高斯滤波后')
plt.show()
3. Results display
3. Histogram equalization
1. Basic principles
Histogram Equalization, also known as histogram flattening, essentially stretches the image nonlinearly and redistributes the pixel values of the image so that the number of pixel values in a certain gray scale range is roughly equal. In this way, the contrast of the peak part in the middle of the original histogram is enhanced, while the contrast of the bottom parts on both sides is reduced, and the histogram of the output image is a flat segmented histogram: if the segment value of the output data is small, there will be Roughly categorized visuals.
Histogram equalization is to modify the randomly distributed image histogram into a uniformly distributed histogram. The basic idea is to do a certain mapping transformation on the pixel gray level of the original image, so that the probability density of the transformed image gray level is uniformly distributed. This means that the dynamic range of the gray scale of the image has been increased and the contrast of the image has been improved.
2. Related code
from PIL import Image
from pylab import *
from PCV.tools import imtools
import matplotlib.pyplot as plt
import matplotlib
# 设置字体为楷体
matplotlib.rcParams['font.sans-serif'] = ["KaiTi"]
# 打开图像,并转成灰度图像
im = array(Image.open('E:/Pythonproject/2021.png').convert('L'))
im2, cdf = imtools.histeq(im)
figure()
subplot(2, 2, 1)
axis('off')
gray()
title(u'原始图像')
imshow(im)
subplot(2, 2, 2)
axis('off')
title(u'直方图均衡化')
imshow(im2)
subplot(2, 2, 3)
axis('off')
title(u'直方图')
hist(im.flatten(), 128, density=True)
subplot(2, 2, 4)
axis('off')
title(u'均衡化后的直方图')
hist(im2.flatten(), 128, density=True)
plt.show()