Introduction
As the strongest UI framework in history, Flutter has many rich UI components. For the actual combat of the Douyin project, this article briefly introduces the usage of ListView, which is the most used basic component.
Constructor
ListView({
super.key,
super.scrollDirection,//滑动方向
super.reverse,//列表反向
super.controller, //控制器
super.primary,
super.physics, //系数
super.shrinkWrap,//item总长度相关
super.padding,//内边距
this.itemExtent,
this.prototypeItem,
bool addAutomaticKeepAlives = true,//自动保存页面状态
..................
List<Widget> children = const <Widget>[], //item集合
int? semanticChildCount,
})
Introduction to common attributes
Common attributes are shown in the table below
Attributes | type | overview |
---|---|---|
scrollDirection | Axis | Axis.horizontal: horizontal list, Axis.vertical: vertical list |
padding | EdgeInsetsGeometry | Padding |
reverse | bool | Components in reverse order |
children | List | list element |
shrinkWrap | bool | Whether to determine the total height based on the item height |
basic use
- ListView.builder
ListView.builder(
itemBuilder: (BuildContext context, int index) {
RouteBean bean = RouteData.getElements()[index];
return itemWidget(bean); //每个item的布局
},
itemCount: RouteData.getElements().length
)
//自定义Item布局
Widget itemWidget(bean){
return Padding(padding: const EdgeInsets.only(
top:10,left: 20,right: 20),child: Container(
constraints: const BoxConstraints(maxWidth: double.infinity,maxHeight: 60),
child: TextButton(
style:ButtonStyle(backgroundColor: MaterialStatePropertyAll(Theme.of(context).primaryColor)) ,
child: Text(
bean.name,
style: const TextStyle(fontSize: 20,color: Colors.white),
),
onPressed: ()=>Navigator.pushNamed(context, bean.route,arguments:bean.name),
)
),);
ListView.builder is generally used when the number of items is uncertain, or a list of data obtained from the network. Display the list by customizing the Item layout.
The effect is as follows:
- ListView.separated
Compared with ListView.builder, ListView.separated has a separatorBuilder attribute, which can directly add a custom dividing line to each Item in the list. Due to the large amount of code, only part of the code is shown here.
ListView.separated(
itemBuilder: (BuildContext context, int index) {
RouteBean bean = RouteData.getElements()[index];
return itemWidget(bean)
},
separatorBuilder: (context, index) { //自定义分割线
return const Divider(
thickness: 10, // 高度 10
color: Color(0xFFF80D05),
);
},
The effect is as follows:
3.ListView(children: [],) . This is suitable for situations where the number of items is fixed and will not increase later. This scenario is rarely used.
add fixed head
We all know that in Android, if you want to add a header to the list, it is not easy to implement, especially the early ListView, which does not support adding headers at all, and it was not supported until the official RecyclewView later. But for FLutter, adding header components is very easy.
ListView.separated(
itemBuilder: (BuildContext context, int index) {
RouteBean bean = RouteData.getElements()[index];
if(index == 0){
return Center(child: Text("我是ListView头部",style:
TextStyle(fontWeight: FontWeight.bold,fontSize: 20),),);
}else{
return itemWidget(bean);
};
},
separatorBuilder: (context, index) {
return const Divider(
thickness: 10,
color: Color(0xFFF80D05),
);
},
The effect is as follows:
We added an if judgment to the item construction place of the ListView. If the subscript is equal to 0, return a header component, otherwise return the item layout. It seems that there is no problem in doing so, but in fact there will be one missing subscript as 0 base component option, we can add a Column component when the subscript is 0 to solve this problem.
ListView.separated(
itemBuilder: (BuildContext context, int index) {
RouteBean bean = RouteData.getElements()[index];
if(index == 0){
return Column(
children: [
Center(child: Text("我是ListView头部",style:
TextStyle(fontWeight: FontWeight.bold,fontSize: 20),),),
SizedBox(width: double.infinity,child: itemWidget(bean))
],
);
}else{
return itemWidget(bean);
};
}
Column() is a vertically arranged component, and the internal components are arranged from top to bottom. Similar to linear layout. The Center() component child is displayed in the center, and the SizedBox() is used to fix the size of the component. More basic components will not be described in detail here, and students can view the webpage by themselves.
error-prone
During development, if you need to use ListView in Column to display the page, there will be a problem that the page cannot be displayed. This is because the ListView cannot be used to measure the height directly in the Column, and an Expend component needs to be added outside the ListView to solve this problem. Interested readers can try it out.
Summarize
ListView is a frequently used basic component in Flutter, and readers should memorize common attributes. To deepen the understanding of ListView through source code analysis, this article only introduces simple usage. In order to be used in the actual combat of the following projects.
In the future, Flutter will be used to develop a vibrato-like project. Interested students can click to follow!
at last
If you want to become an architect or want to break through the 20-30K salary range, then don't be limited to coding and business, but you must be able to select models, expand, and improve programming thinking. In addition, a good career plan is also very important, and the habit of learning is very important, but the most important thing is to be able to persevere. Any plan that cannot be implemented consistently is empty talk.
If you have no direction, here is a set of "Advanced Notes on Eight Android Modules" written by a senior architect of Ali, to help you organize the messy, scattered and fragmented knowledge systematically, so that you can systematically and efficiently master various knowledge points of Android development.
Compared with the fragmented content we usually read, the knowledge points of this note are more systematic, easier to understand and remember, and are arranged strictly according to the knowledge system.
Full set of video materials:
1. Interview collection
2. Source code analysis collection
3. The collection of open source frameworks
welcomes everyone to support with one click and three links. If you need the information in the article, just click the CSDN official certification WeChat card at the end of the article to get it for free↓↓↓
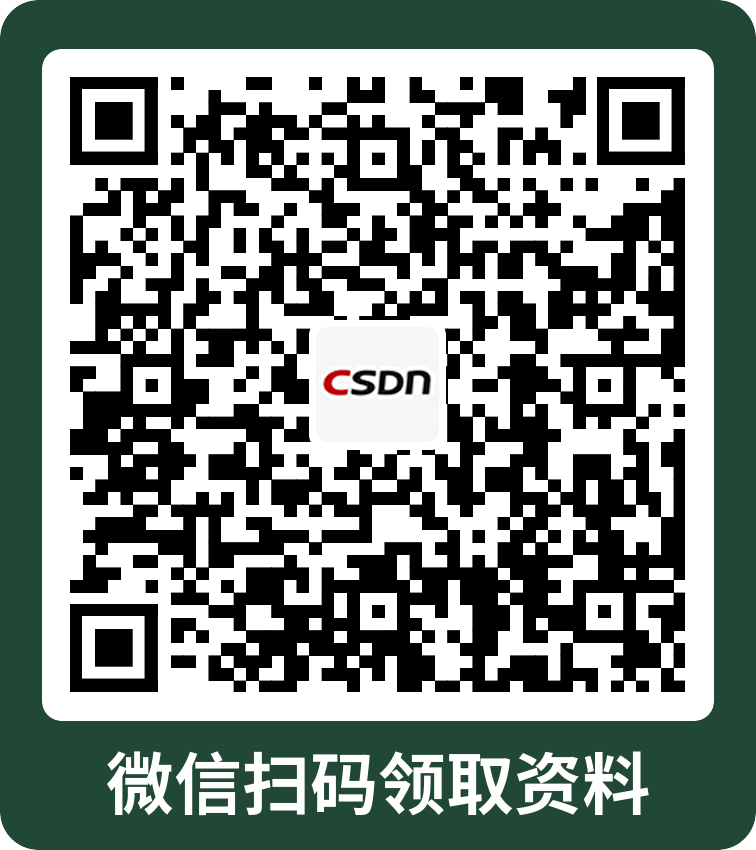