foreword
For Android developers, it is far from enough to master basic application development skills. Whether at work or during an interview, having a solid knowledge of performance optimization is critical to improving your application experience. Android performance optimization mainly covers the following aspects: startup optimization, rendering optimization, memory optimization, network optimization, freeze detection and optimization, power consumption optimization, installation package size optimization, and security issues. The following is a summary of Android performance optimization based on the experience sharing of many bigwigs on the Internet.
There are many solutions for Android performance optimization. During the development process, the following optimizations are mainly considered:
- layout optimization
- drawing optimization
- Memory Leak Optimization
- Response speed optimization
- Listview optimization
- Bitmap optimization
- thread optimization
Next, we briefly introduce the optimization scheme from these aspects.
layout optimization
Everyone must know that there are many layouts in Android, such as Linerlayout, RelativeLayout, etc. Layout optimization is to reduce the layout file level. If the level is reduced, the program will draw much faster, so it can improve performance.
In the layout code, what layout to use basically follows the following rules:
- If both LinearLayout and RelativeLayout can be used in the layout, then LinearLayout is used, because the functions of RelativeLayout are more complicated, and its layout process requires more CPU time.
- If the layout needs to be done in a nested way. In this case, it is recommended to use RelativeLayout, because the nesting of ViewGroup is equivalent to increasing the layout level, which will also reduce the performance of the program.
3. Use or tags and ViewStub to extract the layout of the common part of the layout, which can improve the efficiency of layout initialization.
drawing optimization
Drawing optimization is not to do a lot of operations in the view's onDraw method.
First, do not create new objects in the onDraw method, because the onDraw method may be called frequently, which will generate a large number of temporary files, resulting in excessive memory usage and reduced program execution efficiency.
Second, do not do time-consuming operations as much as possible, and a large number of loops will also take up CPU time
Memory Leak Optimization
Memory leak optimization In other words, what situations may cause memory leaks, I believe everyone is clear, because this is also a classic interview question for beginners. There are mainly the following situations:
- Do not declare static variables in Activity, so that the Activity cannot be completely destroyed and released
- The memory leak with the singleton design pattern, the static nature of the singleton design pattern will make its life cycle as long as the life cycle of the application, which means that if an object is no longer in use, and the singleton object is still holding a reference to the object, then the GC will not be able to recycle the object, resulting in a memory leak. So when using singleton mode, the incoming context should use ApplicationContext
- Memory leaks caused by static instances created by non-static inner classes
- For memory leaks caused by Handler, do not use non-static anonymous inner classes to refer to Hanlder in Activity, for example:
public class MainActivity extends AppCompatActivity {
private Handler mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
loadData();
}
private void loadData(){
Message message = Message.obtain();
mHandler.sendMessage(message);
}
}
In this way, the hanlder will hold a reference to the Activity, and the handler runs in a Looper thread, and the Looper thread polls to process messages in the message queue . Suppose we process 10 messages, and when it reaches the 6th message, the user exits and destroys the current Activity.
Response speed optimization
The core idea of response speed optimization is to avoid time-consuming operations in the main thread. Android stipulates that if the Activity fails to respond to screen touch events or keyboard input events within 5 seconds, ANR will occur. If the BroadcastReceiver is 10 seconds, the Service time is 20 seconds. Of course, this is a small probability event. If it is not reflected within the corresponding time, ANR will occur. When there is a time-consuming operation, a thread can be opened separately for operation.
listview optimization
I believe everyone is familiar with listview optimization. It is also a classic interview question. I won’t go into details here. It mainly includes reusing views. First, judge whether the view is empty.
Bitmap optimization
In fact, the idea is also very simple, that is to use BitmapFactory.Options to load the picture of the required size. Here it is assumed that the image is displayed through ImageView. In many cases, ImageView is not as large as the original size of the image. At this time, the entire image is loaded and then set to imageView. This is obviously unnecessary, because ImageView has no way to display the original image. Through BitmapFactory.Options, the reduced image can be loaded according to a certain sampling rate, and the reduced image can be displayed in ImageView, which will reduce memory usage and avoid OOM to a certain extent, and improve the performance of Bitmap loading.
thread optimization
The idea of thread optimization is to use a thread pool to avoid a large number of Threads in the program. The thread pool can reuse internal threads, thereby avoiding the performance overhead caused by the creation and destruction of threads. At the same time, the thread pool can effectively control the maximum number of concurrency of the thread pool, and avoid the occurrence of blocking phenomenon caused by a large number of threads preempting each other for system resources. Therefore, in actual development, we should try to use the thread pool instead of creating a Thread object every time.
Android performance optimization
theoretical aspects
There are many knowledge points involved in Android performance optimization. In addition to the common solutions mentioned above, the underlying principles are also worthy of our in-depth discussion. In addition, there are performance monitoring and the use of tools. Based on my Android development experience, I have compiled the underlying principles of these performance optimizations, solutions to various problems, and knowledge outlines into materials. Hope to help you guys.
Summary of content: "Android Performance Optimization Practical Chapter"; 360° all-round performance optimization.
Content features: clear organization, including graphic representation, which is easier to understand.
Due to the large content of the article and the limited space, the information has been organized into a PDF document. If you need "Android Performance Optimization in Practice" and 360° all-round performance tuning complete document, you can scan the card below to get it!
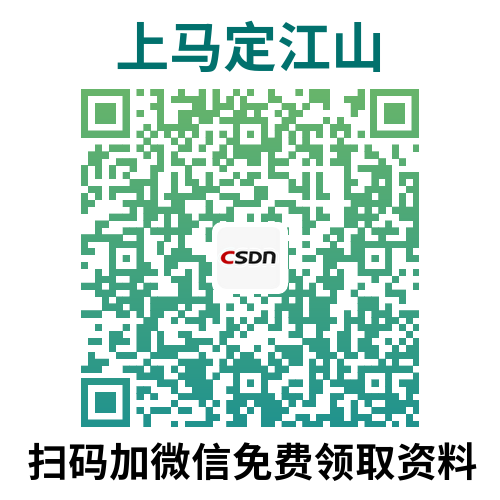