1. Experimental purpose and requirements
Understand and master the concept, storage structure and operation requirements of linear tables, and experience the characteristics of sequential and chain storage structures; according to the different requirements of operations, choose an appropriate storage structure, design and implement algorithms, and analyze the time complexity and space complexity of the algorithms. By realizing the algorithm design of various operations on the linear table, the purpose of mastering the research method, algorithm design and analysis method of the data structure is achieved.
Proficiency in using JAVA language to realize data structure design and algorithm design, and understand various errors that occur during program operation. Not only must master the basic skills of editing, compiling and running programs in integrated development environments such as MyEclipse, but also master program debugging techniques such as setting breakpoints and single-step running, so as to find errors in time and adopt different methods to deal with different errors.
2. Experimental topics
1. Compare equals equals(Object obj)
2. Return the sublist SinglyList<T> subList(begin, end)
3. Experimental methods and steps (requirements analysis, algorithm design ideas, flowcharts, etc.)
First create a singly linked list node class, which requires a data field to store data, and an address field to refer to subsequent nodes.
Then create a singly linked list class and add a head node. Realize basic functions such as inserting and deleting elements, and the method of returning the sub-list is: first create an empty single-linked list, and create a pointer to point to the head node of the new linked list, create another pointer to point to the current linked list, move to begin and start copying data to the new linked list until end, and finally return to the new linked list.
Applying deep copy to copy a new singly linked list, the equals method compares whether the two singly linked lists are equal, sets p and q to point to the next node of the head node of the two linked lists respectively, and traverses to compare whether the data fields of the two nodes are equal. The Sublist method takes out the sublist by inserting, first builds a new singly linked list, p points to the head pointer to traverse, sets the beginning and end of the sublist as begin and end, and finally inserts the begin to end data obtained by p into the new sublist singly linked list.
4. The original record of the experiment (source program, data structure, etc.)
package unit2;
public class Node<T>
{
public T data;
public Node<T>next;
public Node(T data,Node<T>next)
{
this.data = data;
this.next = next;
}
public Node()
{
this(null,null);
}
public StringtoString()
{
return this.data.toString();
}
}
package unit2;
public class SinglyList<T extends Comparable<?super T>> extends Object
{
public Node<T> head;
public SinglyList()
{
this.head=new Node<T>();
}
public SinglyList(T[] values) //Construct a singly linked list, the values array provides elements
{
this();
Node<T> rear=this.head; //rear points to the last node
for(int i=0;i<values.length;i++)
{
rear.next=new Node<T>(values[i],null);
rear=rear.next; //rear points to the new chain end node
}
}
public boolean isEmpty() //Determine whether the singly linked list is empty
{
return this.head.next==null;//Return the description string of all elements in the singly linked list, in the form of "(,)". Override the toString() method in the Object class
}
public T get(int i)
{
Node<T>p=this.head.next;
for (int j=0;p!=null&&j<i;j++)
p=p.next;
return(i>=0&&p!=null)?p.data:null;
}
public void set(int i,T x)
{
if(x==null)
throw newNullPointerException("x==null");//抛出空对象异常
Node<T>p=this.head.next;
for(int j=0;p!=null&&j<i;j++)//遍历寻找第i个结点(p)
p=p.next;
if(i>=0&&p!=null)
p.data=x;//p指向第i个结点
else throw newIndexOutOfBoundsException(i+"");//抛出序号越界异常
}
public StringtoString()
{
String str=this.getClass().getName()+"(";
for(Node<T> p=this.head.next; p!=null; p=p.next)//p遍历单链表
{
str += p.data.toString();
if(p.next!=null)
str += ",";
}
return str+")";
}
public int size() //返回链表长度
{
int i=0;
for(Node<T> p=this.head.next;p!=null;p=p.next)//p遍历单链表
i++;
return i;
}
publicNode<T>insert(int i,T x)
{
if(x==null)
throw newNullPointerException("x==null");
Node<T> front=this.head; //front指向头结点
for(int j=0;front.next!=null&&j<i;j++)//寻找第i个结点或者第i-1个结点
front=front.next;
front.next=new Node<T>(x,front.next);//front之后插入为x的结点
return front.next;
}
public Node<T>insert(T x)
{
return insert(Integer.MAX_VALUE,x);
}
public T remove(int i)
{
Node<T> front=this.head;
for(int j=0;front.next!=null&&j<i;j++)
front=front.next;
if(i>0 && front.next!=null);//后继存在就删除
{
T old = front.next.data;
front.next= front.next.next;
return old;
}
}
public void clear()
{
this.head.next=null;
}
publicNode<T>search (T key)
{
for(Node<T> p=this.head.next;p!=null;p=p.next)
if(key.equals(p.data))
return p;
return null;
}
public boolean contains(T key)
{
return this.search(key)!=null;
}
publicSinglyList(SinglyList<T> list)//深拷贝
{
this();
Node<T> rear=this.head;
for(Node<T> p=list.head.next;p!=null;p=p.next)
{
rear.next=new Node<T>(p.data,null);
rear=rear.next;
}
}
//判断
public boolean equals(Object obj)//比较两条单链表是否相等
{
if(obj==this)
return true;
if(!(obj instanceofSinglyList<?>))
return false;
Node<T> p=this.head.next;
Node<T> q=((SinglyList<T>)obj).head.next;
while(p!=null&&q!=null&&p.data.equals(q.data))
{
p=p.next;
q=q.next;
}
return p==null&&q==null;
}
publicSinglyList<T> subList(int begin,int end)//取出子表
{
SinglyList<T> sublist = newSinglyList<T>();
Node<T> p=this.head;
if(end>=this.size())
throw newjava.lang.IndexOutOfBoundsException();
else
for(int j=0;p!=null&&j<this.size();j++)
{
p=p.next;
while(j>=begin-1&&j<end)
{
sublist.insert(p.data);//尾插入
break;
}
}
return sublist;
}
public static void main(String args[])
{
//String[] str1= {"a","b","c"};
//SinglyList l1= new SinglyList(str1);
//System.out.println(l1.toString());
Integer[]values={8,7,2,6,3}; //自动将int包装成Integer实例
SinglyList<Integer> list1 = new SinglyList<Integer>(values);
list1.insert(1,10); //调用insert(i,x),单链表插入
list1.insert(2); //调用insert(x),单链表尾插入,将int包装成Integer实例
System.out.println("list1="+list1.toString());
System.out.println("sublist="+list1.subList(2,4).toString());
SinglyList<Integer>list2 = new SinglyList<Integer>(list1); //深拷贝
System.out.print("list2="+list2.toString());
System.out.println(",list1.equals(list2)?"+list1.equals(list2));
//System.out.println("sublist="+list1.subList(2,4).toString());
}
}
五、实验结果及分析(计算过程与结果、数据曲线、图表等)
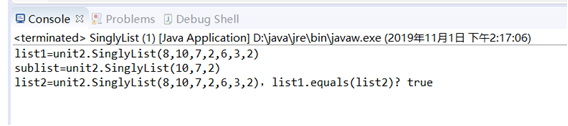