Whether it is going to school or going to work, attendance will be counted, and some schools or companies will punish those who miss their cards too many times (for example, more than three times) per month. Some companies also stipulate that grassroots employees are required to submit logs on working days, managers are required to submit weekly or monthly reports, and those who submit less are required to be punished. It will be a time-consuming and boring job if the company HR processes the personnel log or missing card data one by one.
This article provides methods to automatically handle missing attendance and logs. No need to install Python, no need to learn Python syntax, as long as you can create a new folder on the computer, click on the file to realize the statistical output of attendance and log missing list. Next let's take a look at the implementation steps.
1. Effect display
1 to achieve the effect
First look at the implementation effect.
The general implementation steps are as follows:
Step 1: Create a new "monthly card missing data processing" folder in the D drive (it has been fixed in the code, and this folder must be created).
Step 2: Put the exe file and original data file for processing missing attendance into the folder created in step1.
Step 3: Click the exe file, and the csv result file will be automatically displayed. The specific format is as follows:
2 Raw data template
The original data file needs to be "judging whether to submit the log 2.xlsx". The original data used in this article is as follows (the table header needs to be named as follows):
Note: If you need the original data of this article and the exe file that you can run directly to get the result, you can reply "missing card" in this official account, and you can get it for free.
Among them, the person who fills in the report refers to the name of the student or employee. If the department is a student, you can fill in a certain class. The filling time refers to the time when the diary is filled, and the date refers to the actual date of the diary. If it is attendance punching, fill in the actual punching date for both dates. If it is attendance punching, the column of work completed today can be left blank.
If you want to store the annual data of employees' card punching in the original file, but want to count the missing card data of a certain month. Just put the month you want to count in the first line of the date, and the code has already filtered the data sub-boxes of the same year and month according to the first line of the date. If you need to set a scheduled task and send a scheduled email of the running result to the relevant personnel, you can private message me in the official account.
2. Detailed code explanation
For some friends who know Python, if they have individual needs, they can fine-tune the code to achieve their own needs. Next, the code to realize the above functions will be described in detail.
1 import library
First import the libraries that need to be loaded in this article. If you have not installed some libraries and an error is reported when running the code, you can use the pip method to install them in Anaconda Prompt.
# -*- coding: UTF-8 -*-
'''
代码用途 :处理缺卡数据
作者 :阿黎逸阳
公众号 : 阿黎逸阳的代码
'''
import os
import calendar
import numpy as np
import pandas as pd
from datetime import datetime
from xlrd import xldate_as_tuple
from chinese_calendar import is_workday
from chinese_calendar import is_holiday
from chinese_calendar import get_holiday_detail
This article applies to os, calendar, numpy, pandas, datetime, xlrd, chinese_calendar libraries.
The os library can set where the file is read from.
The calendar and chinese_calendar libraries are date processing libraries.
The numpy and pandas libraries work with data frames.
The xlrd and datetime libraries deal with time.
2 Define the time processing function
Then use the functions in the xlrd and datetime libraries to define the time processing function, and convert the time stamp or the time with hours, minutes and seconds into a time containing only the year, month, and day.
def num_to_date(x):
'''
日期处理函数
把时间戳或带时分秒的时间转换成只含年月日的时间
'''
try:
x1 = datetime(*xldate_as_tuple(x, 0)).strftime('%Y-%m-%d')
except:
x1 = datetime.date(x).strftime('%Y-%m-%d')
return x1
The purpose of defining the uniform time of the year, month, and day is to facilitate the operation of subsequent codes.
3 Read the data and adjust the date format
Then read the data, and apply the time processing function defined in the second section to process the time and date.
#读取数据
os.chdir(r'D:\每月缺卡数据处理')
date = pd.read_excel('判断是否提交日志2.xlsx', sheet_name='Sheet1')
#调整日期格式
date['填报时间'] = date['填报时间'].apply(num_to_date)
date['日期'] = date['日期'].apply(num_to_date)
The original part of the data is as follows:
the part of the data obtained by calling the time processing function is as follows:
4 Calculate the number of working days
Then take out the first value of the date column of the data frame to obtain the year and month information to be counted. Get the number of working days in the month according to the year and month information.
#取出想看缺卡信息的年月
y_m1 = date['日期'][0][0:7]
def sele_ym(x, y_m=y_m1):
'''
判断数据框中的日期是否为某月
'''
if x.find(y_m)>=0:
return True
#找出这一个月中的工作日,求出工作日的天数
days = calendar.Calendar().itermonthdates(int(y_m1.split('-')[0]), int(y_m1.split('-')[1]))
mth_nwkdays = [] #非工作日
mth_wkdays = [] #工作日
mth_days = [] #全部日期
for day in days:
if str(day).find(y_m1)>=0:
#print(str(day))
mth_days.append(str(day))
if is_workday(day)>0:
mth_wkdays.append(str(day))
else:
mth_nwkdays.append(str(day))
work_days = len(mth_wkdays) #工作日天数
Compare the number of working days with the actual number of days that the employee punches in or writes a log this month. If the actual value is less than the theoretical value, it means that the employee is out of card or asked for leave. Since most of the employees clock in or write logs normally, manual inspection of employees with missing cards at this time has greatly reduced the scope of investigation. If you have special code needs and need help, you can private message me in the official account.
5 Get the missing card list
Finally, the function is called to obtain the missing card list, mainly to compare each filling date with the actual working date.
#定义获取缺卡信息的函数
def stat_dail_short(date, y_m1, work_days):
'''
date:存储日志的数据大表
y_m1:月份
work_days:该月的工作天数
'''
qk_file = []
date_m = date[date['日期'].apply(sele_ym)==True]
for i in set(date_m['填报人']):
sub_date = date_m[date_m['填报人'] == i]
if len(sub_date['日期'])<work_days:
qk = str(set(sub_date['填报人'])) + str(set(sub_date['部门'])) + '缺了'+ str((work_days-len(sub_date['日期']))) + '次卡' + ';缺卡日期为:'+ str(set(mth_wkdays)^set(sub_date['日期']))
qk_file.append(qk)
print(set(sub_date['填报人']), set(sub_date['部门']), '缺了%d次卡'%(work_days-len(sub_date['日期'])), ';缺卡日期为:', set(mth_wkdays)^set(sub_date['日期']),sep='')
qk_file_1 = pd.DataFrame(qk_file)
qk_file_1.columns = ['缺卡信息']
qk_file_1.to_csv(y_m1+' 缺卡名单'+'.csv', encoding='gbk')
#调用函数获取缺卡名单
stat_dail_short(date, y_m1, work_days)
got the answer:
{
'张继科'}{
'体育部'}缺了5次卡;缺卡日期为:{
'2022-04-11', '2022-04-29', '2022-04-22', '2022-04-18', '2022-04-21'}
{
'杨紫'}{
'历劫部'}缺了1次卡;缺卡日期为:{
'2022-04-20'}
{
'刘诗雯'}{
'体育部'}缺了2次卡;缺卡日期为:{
'2022-04-18', '2022-04-28'}
The data in the result is spliced and displayed by the person who filled in the report, the reporting department, the number of missing cards, and the specific date of missing cards. It will be stored in the specified folder in the form of csv. If you need to separate information such as name, department, number of missing cards, etc., you can sort them out according to specific conditions in excel, or adjust the code to achieve it.
For the exe file generation method at the beginning of this article, you can refer to the article Pinstaller (Python is packaged into an exe file). I have been reporting errors in the process of generating exe. Later, I saw a method on the Internet that said to run pip uninstall matplotlib in cmd first, and then run the statement to generate exe, and no error will be reported. According to the online method, it was really successful. Although I didn't understand the principle, thank you very much! If you did not report an error when packaging, it is still not recommended to delete the matplotlib library.
So far, the fully automatic processing of monthly card shortage data and the output of card shortage personnel information have been explained. Let's share it with those who need it around you.
You may be interested in:
Draw Pikachu with Python
Draw a word cloud map
with Python Draw 520 eternal heart beats with Python Python face recognition - you are the only one
in my eyes With sound and text) Use the py2neo library in Python to operate neo4j and build a relationship map Python romantic confession source code collection (love, rose, photo wall, confession under the stars)
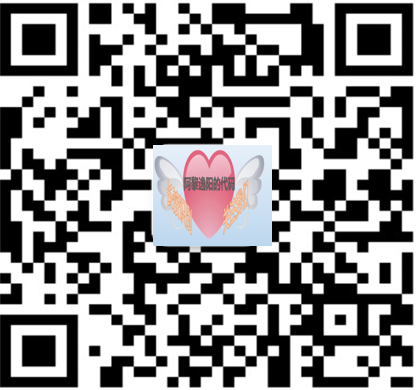