Article Directory
Interview question 08.01. Three-step question
Click to view: three-step question
Three step question. A child is going up the stairs. The stairs have n steps. The child can go up 1 step, 2 steps or 3 steps at a time. Implement a method that counts how many ways the child can go up the stairs. The result can be very large, you need to modulo 1000000007 on the result.
Example 1:
Input: n = 3
Output: 4
Explanation: There are four ways to move
Example 2:
Input: n = 5
Output: 13
topic analysis
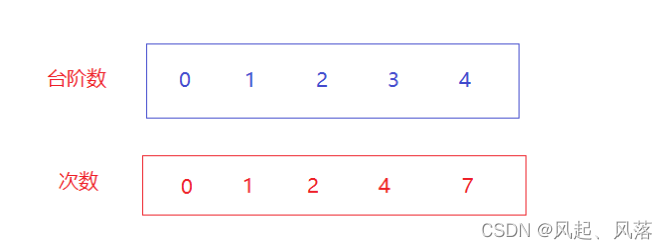
When n==1,
it can only go from 0 to 1, that is, 0->1, so there is only one method
When n==2
, it can go from 0->2, there is one way
to go from 1->2, and there is only one way from 0 to 1, and only one step is needed from 1 to 2, so there are two ways to
finally 1+ 1, there are 2 methods
When n==3,
there is 1 method from 0->3
From 1->3, because there is only 1 method from 0->1, and only one step is needed from 1 to 3, so there is 1 method
from 2->3 , because 0->2 has 2 methods, and 2 to 3 only needs to add one step, so there are 2 methods, and
finally 1+1+2, there are 4 methods in total
When n==4
, because at most 3 steps are taken at a time, 0->4 is not valid.
From 1->4, because there is 1 method for 0->1, and 1 to 4 only needs to add one step, so there is 1 method
from 2->4, because 0->2 has 2 methods, and 2 to 4 only needs to add one step, so there are 2 methods
from 3->4, because 0->3 has 3 methods, and 3 to 4 only Need to add one step, so there are 3 methods,
finally 1+2+3, a total of 7 methods
state transition equation
Ending at position i
dp[i] represents how many ways there are when reaching position i
State transition equation
Divide the problem with the state of position i, the latest step
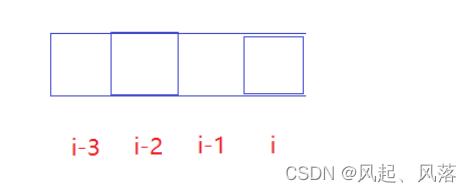
dp[i] is considered in three cases,
from i-1 position to i position is dp[i-1]
from i-2 position to i position is dp[i-2]
from i-3 position to i position is dp [i-3]
dp[i]= dp[i-1]+dp[i-2]+dp[i-3]
full code
class Solution {
public:
int waysToStep(int n) {
if(n==1||n==2)
{
return n;
}
if(n==3)
{
return 4;
}
vector<int>dp(n+1);
dp[1]=1;
dp[2]=2;
dp[3]=4;
int i=0;
for(i=4;i<=n;i++)
{
dp[i]=( (dp[i-1]+dp[i-2])%1000000007+dp[i-3])%1000000007;
}
return dp[n];
}
};
When calculating the state transition equation, you cannot add the three together and then take the modulus, otherwise an error will be reported.
When adding dp[i-1] and dp[i-2], you need to take the modulus, and then use dp[i-3 ] and take the modulus when adding
746. Climb Stairs with Minimum Cost
Click to view: Climb stairs with minimum cost
You are given an integer array cost, where cost[i] is the cost to climb up the i-th step of the stairs. Once you pay this fee, you can choose to climb a flight of stairs or two.
You can choose to start climbing the stairs from the step with subscript 0 or subscript 1.
Please calculate and return the minimum cost to reach the top of the stairs.
Example 1:
Input: cost = [10,15,20]
Output: 15
Explanation: You will start from the step with subscript 1.
Pay 15 and go up two steps to the top of the stairs.
The total cost is 15.
topic analysis
Starting from the subscript 0, you can spend 10 yuan to the subscript 1, or you can go to the subscript 2,
but the subscript 2 is not the roof, because if it is the roof, the minimum cost should be is 10, not 15,
so the roof is the next to the last element of the cost array
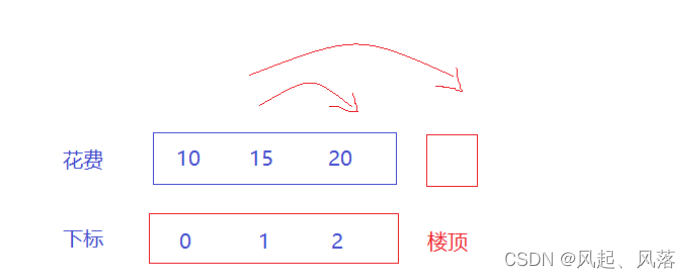
Starting from the position with subscript 1, you can go to the position with subscript 2, or you can go to the top of the building
state transition equation
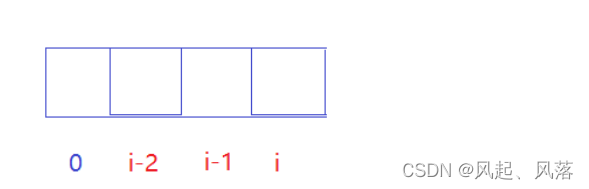
dp[i] represents the minimum cost when reaching position i
, and the minimum cost of position i is obtained by combining the minimum cost of position i-1 and the minimum cost of position i-2
dp[i] can be divided into
1. First reach position i-1, pay cost[i-1], take one step
dp[i-1] represents the minimum cost to reach position i-1, and cost[i-1] represents the cost required for position i-1, that
is dp[i-1]+cost[i-1]
2. First reach position i-2, pay cost[i-2], take two steps
dp[i-2] represents the minimum cost to reach position i-2, cost[i-2] represents the cost required for position i-2
That is, dp[i-2]+cost[i-2]
Dynamic transfer equation
dp[i]= min(dp[i-1]+cost[i-1],dp[i-2]+cost[i-2]);
full code
class Solution {
public:
int minCostClimbingStairs(vector<int>& cost) {
vector<int>dp(cost.size()+1,0);
dp[0]=0;
dp[1]=0;
int i=0;
for(i=2;i<cost.size()+1;i++)
{
dp[i]=min(dp[i-1]+cost[i-1],dp[i-2]+cost[i-2]);
}
return dp[cost.size()];
}
};
For the state transition equation, the positions with subscript 0 and subscript 1 cannot be used, which will cause cross-border.
In the question, you can choose to start climbing stairs from position 0 or 1, which means that the two positions are free,
that is, dp[0 ] =0 ,dp[1]=0
91. Decoding method
Click to view: decoding method
A message containing the letters AZ is encoded by the following mapping:
'A' -> "1"
'B' -> "2"
'Z' -> "26"
To decode an encoded message, all numbers must be based on the above mapping method, reverse mapping back to letters (there may be more than one method). For example, "11106" can be mapped as:
"AAJF", grouping messages as (1 1 10 6)
"KJF", grouping messages as (11 10 6)
Note that messages cannot be grouped as (1 11 06) because " 06" cannot be mapped to "F" because "6" and "06" are not equivalent in the mapping.
Given a non-empty string s containing only numbers, please count and return the total number of decoding methods.
The question data guarantees that the answer must be a 32-bit integer.
Example 1:
Input: s = "12"
Output: 2
Explanation: It can be decoded as "AB" (1 2) or "L" (12).
Example 3:
Input: s = "06"
Output: 0
Explanation: "06" cannot be mapped to "F" because of leading zeros ("6" and "06" are not equivalent).
topic analysis
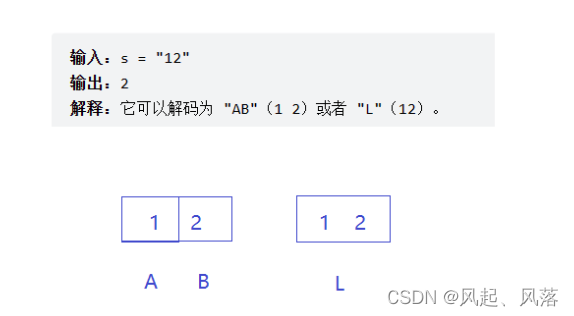
If it is divided into 1 and 2, it corresponds to A and B respectively.
If it is regarded as a whole, it corresponds to L
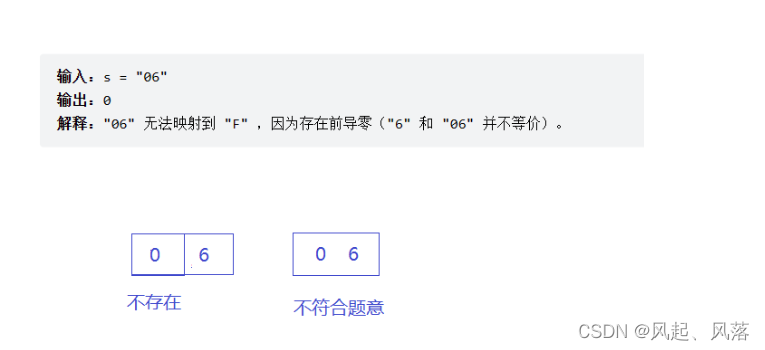
If it is divided into 0 and 6, then 0 has no corresponding letter
If it is regarded as a whole, no leading 0 is allowed to indicate
state transition equation
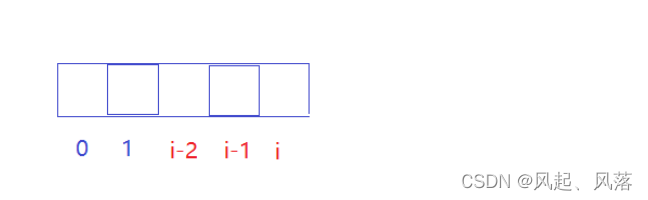
dp[i] indicates the total number of decoding methods when the i position is the end
Case 1: Let the number at position i be decoded separately
The number decoded separately needs to be 1-9, so there will be success/failure
If the decoding is successful, the number corresponding to position i is between 1-9, which is equivalent to adding a character after all the decoding schemes at positions 0 to i-1, and the
overall decoded number is the number ending at position i-1 That is, dp[i-1]
If the decoding fails, all of them fail, and the decoding number is 0,
such as: 60 is calculated separately, 6 is F, and 0 does not have a corresponding number, so there is no decoding success
Case 2: Let the number at position i and the number at position i-1 be combined to decode
If the decoding is successful, the combined number is between 10-26, which is equivalent to adding a character after all the decoding schemes at positions 0 to i-2, and the
overall decoding number is the number ending with i-2, that is, dp [i-2]
If the decoding fails, all fail, and the decoding number is 0
dp[i]=dp[i-1]+dp[i-2]
dp[i-1] and dp[i-2] will only be added when the decoding is successful, otherwise it will be 0
full code
class Solution {
public:
int numDecodings(string s) {
vector<int>dp(s.size());
int i=0;
//初始化
if(s[0]!='0')
{
dp[0]=1;
}
else
{
dp[0]=0;
}
//有可能s字符串只有一个数字
if(s.size()==1)
{
return dp[0];
}
if(s[0]!='0'&&s[1]!='0')
{
dp[1]++;
}
//因为s[0]存的是字符,所以选哟减去'0',从而获取数字
int sum=(s[0]-'0')*10+(s[1]-'0');
if(sum>=10&&sum<=26)
{
dp[1]++;
}
for(i=2;i<s.size();i++)
{
//说明可以单独编码成功
if(s[i]!='0')
{
dp[i]+=dp[i-1];
}
//说明可以结合编码成功
int sum=(s[i-1]-'0')*10+(s[i]-'0');
if(sum>=10&&sum<=26)
{
dp[i]+=dp[i-2];
}
}
return dp[s.size()-1];
}
};
Initialize
dp[0] means there is only one number.
If the number is between 1-9, the decoding is successful and 1 is returned.
If the number is 0, the decoding fails and 0 is returned.
dp[1] means that two numbers
can be divided into two numbers that can be decoded separately and combined to decode
If the decoding is successful alone, the decoding number + 1, otherwise it is 0,
if the combination is successful, the decoding number + 1, otherwise it is 0,
so there are 0 1 2 three cases