@reqeustbody
There are two ways to receive the request body in json format, one is java entity class, which will convert the request body in json format into entity class
public R adminbook(@RequestBody Project project) {
System.out.println(project);
return R.ok().data("data",project);
}
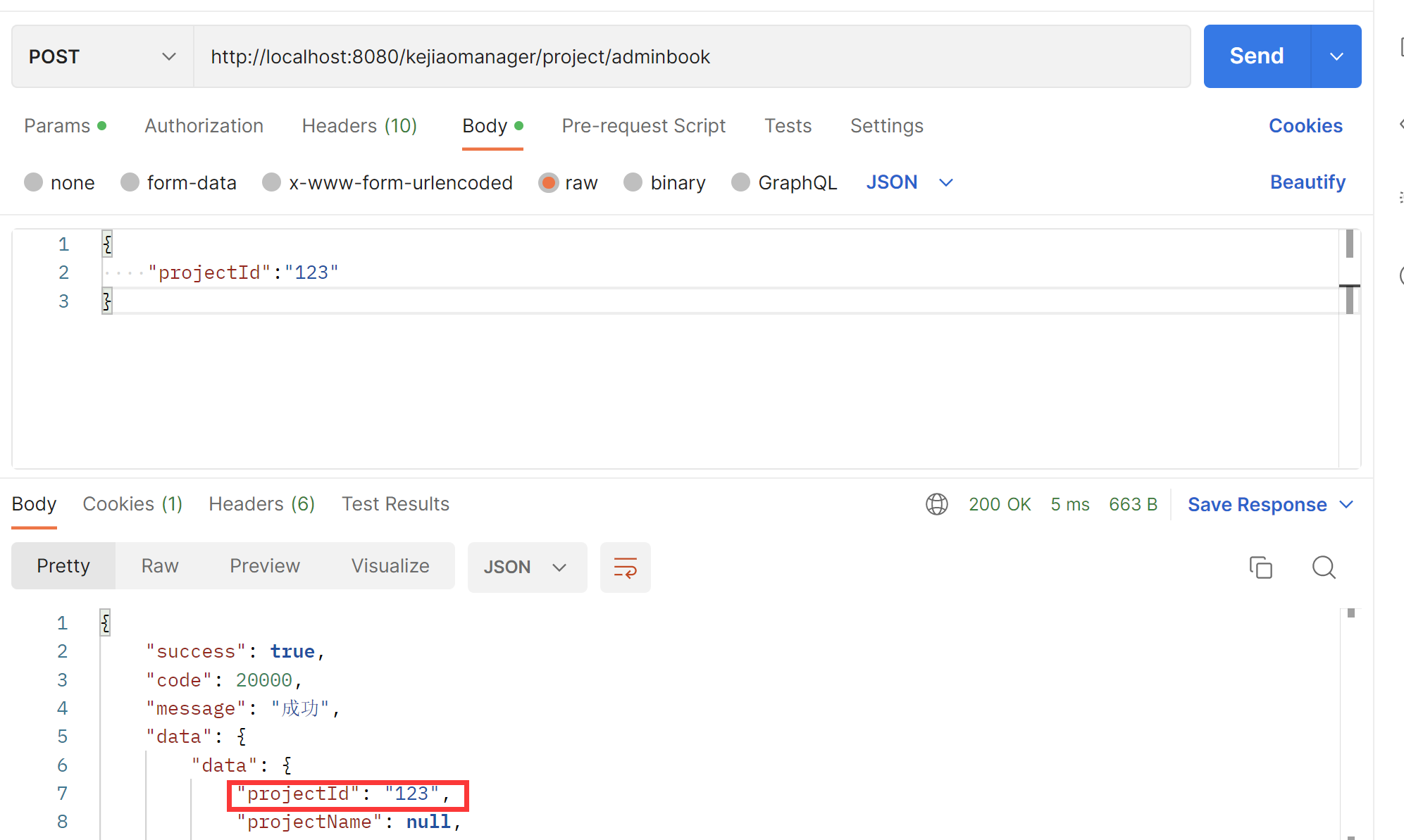
If there is no parameter corresponding to the entity class in the request body, each attribute in the entity class is empty.
The second way is Map, which will convert the json format in the request body into a map object, as follows
public R adminbook(@RequestBody Map map) {
//遍历map
Iterator<Map.Entry<String, String>> entires = map.entrySet().iterator();
while (entires.hasNext()) {
Map.Entry<String, String> entry = entires.next();
String key = entry.getKey();
String value = entry.getValue();
System.out.println(key + ":" + value);
}
return null;
}
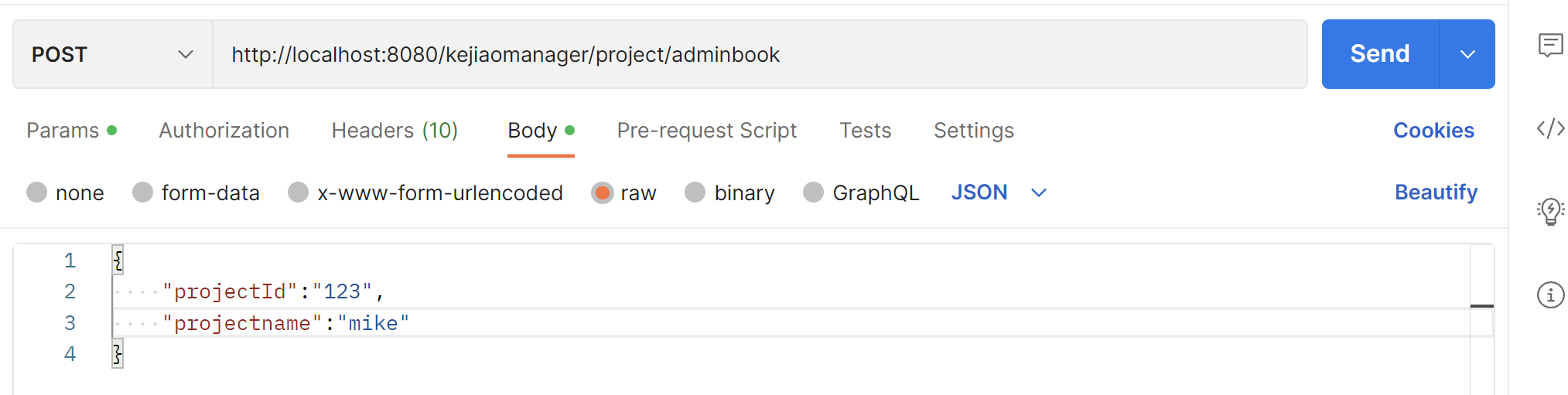
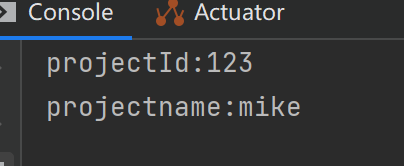
Note: Requests of form-data type cannot be received, and an error will be reported, as follows
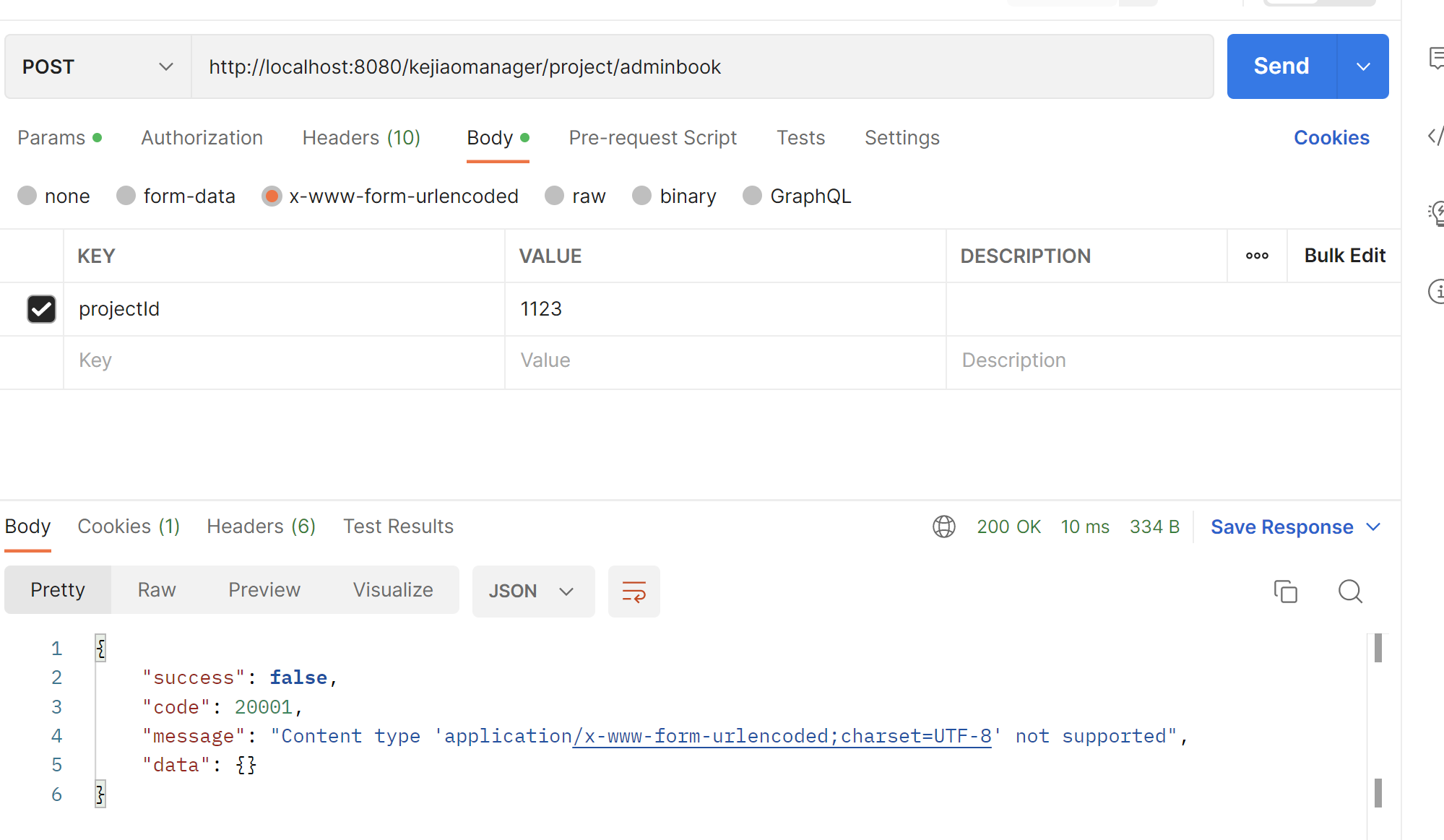
Write string directly, you can receive form format data (request header is application/x-www-form-urlencoded ),
@postmapping("/adminbook")
public R adminbook(String projectPlanDescTeacher,
String projectId,
String projectStatus) {
System.out.println(projectId);
System.out.println(projectPlanDescTeacher);
System.out.println(projectStatus);
return null;
}
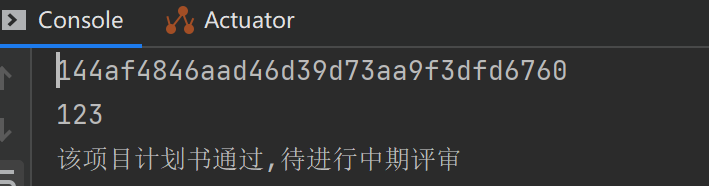
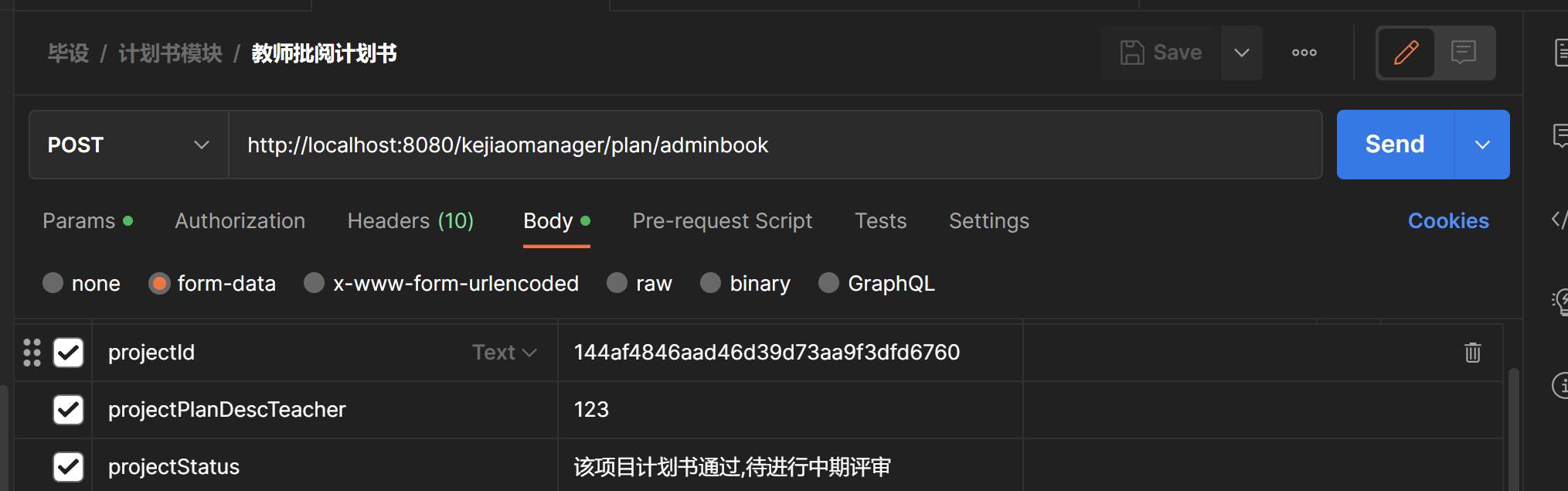
can receive the request path carried directly
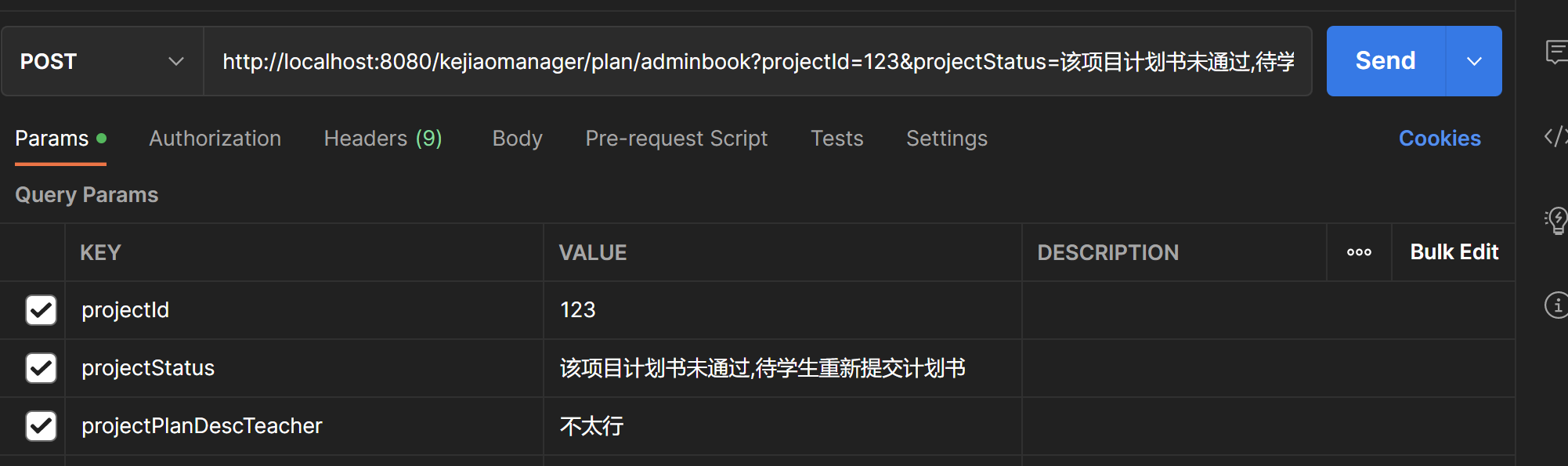
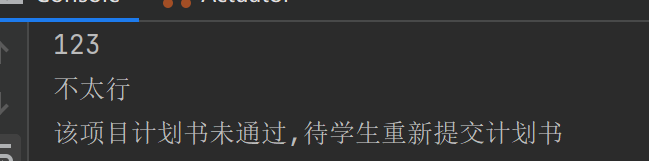
@RequestParam
Can receive form-data format
public R info(@RequestParam String u_name, @RequestParam String u_pwd, @RequestParam String roleName) {
System.out.println(u_name);
System.out.println(u_pwd);
System.out.println(roleName);
// userService.adduser(new User(u_name, u_pwd), roleName);
return R.ok();
}
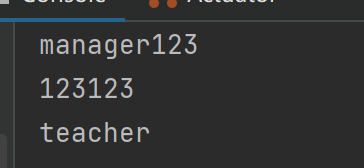
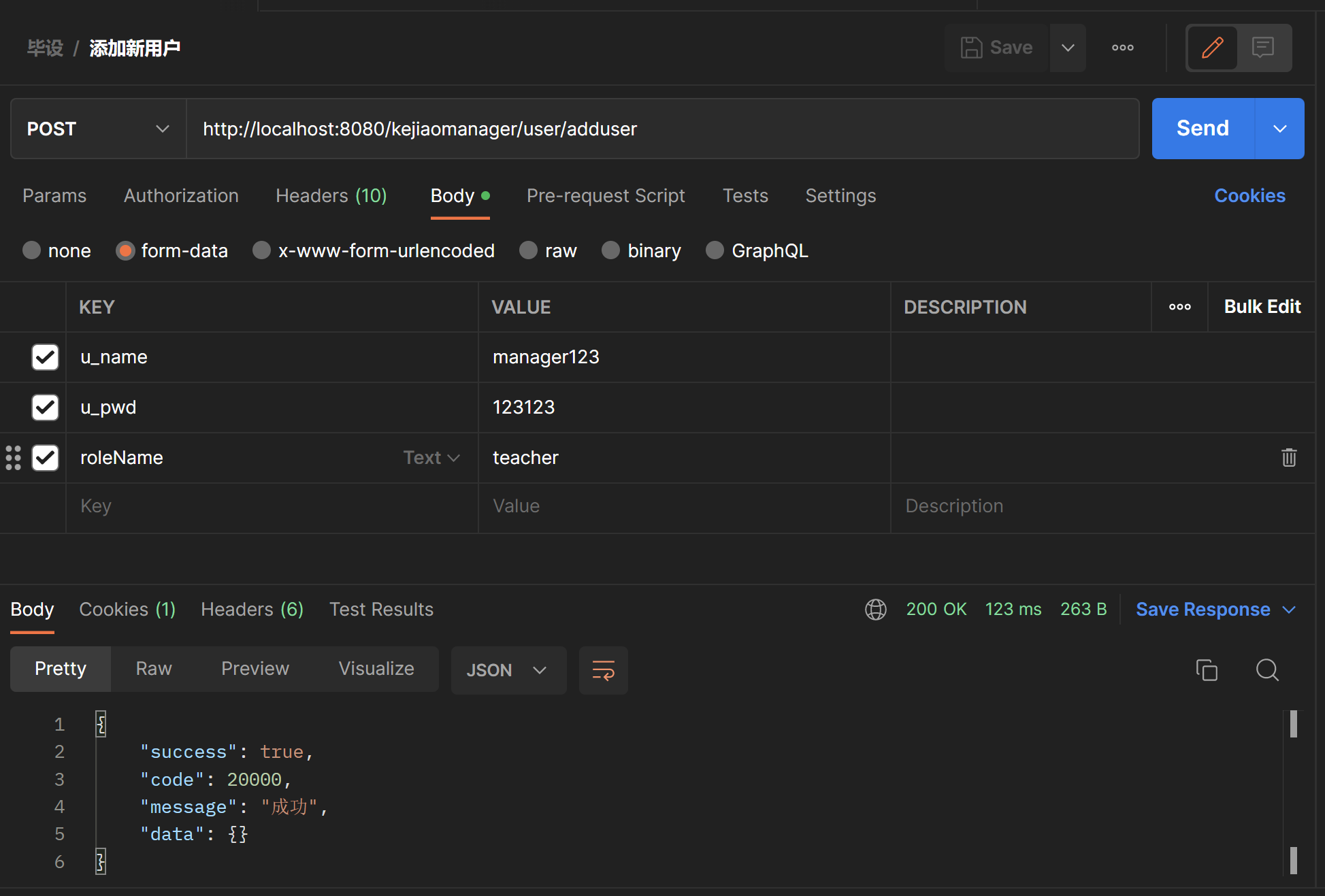
Data in form-urlencoded format can be accepted
@PostMapping("/adduser")
public R info(@RequestParam String u_name, @RequestParam String u_pwd, @RequestParam String roleName) {
System.out.println(u_name);
System.out.println(u_pwd);
System.out.println(roleName);
// userService.adduser(new User(u_name, u_pwd), roleName);
return R.ok();
}
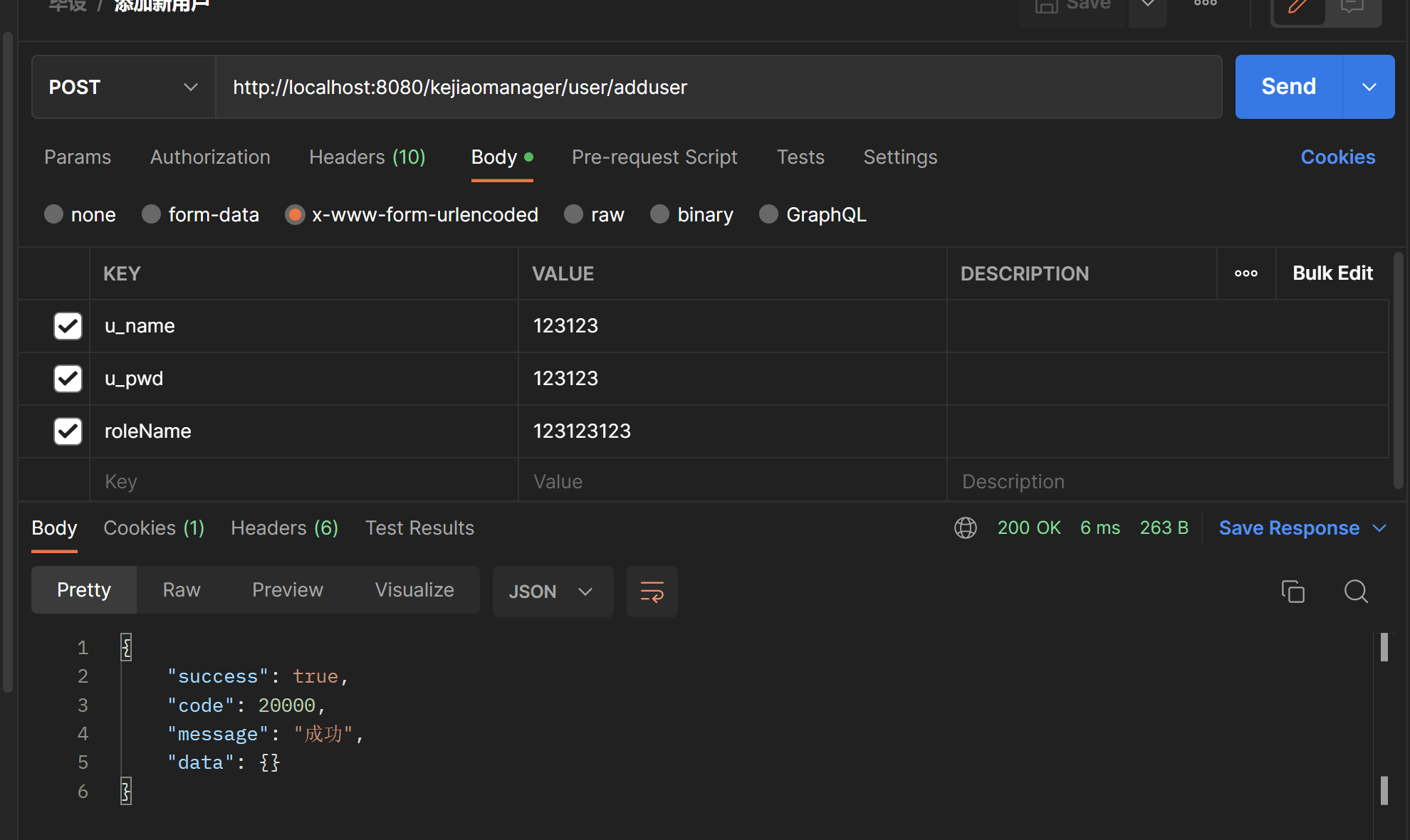
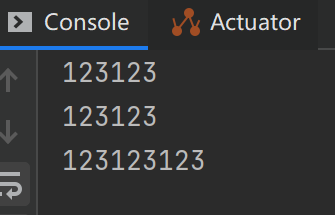
You can also receive parameters in the request header
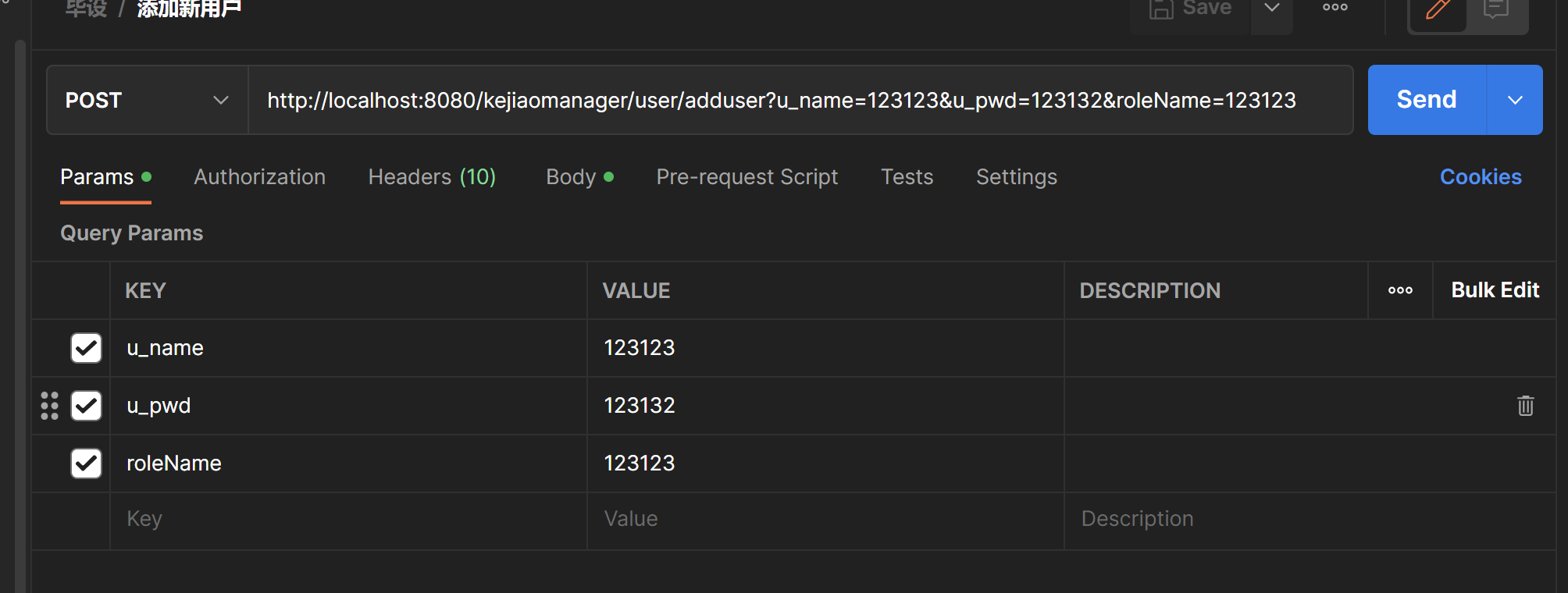