Import matplotlib third-party library
In addition, in matplotlib, we can only input the y-axis, that is, we can also output only one array, and x is not a necessary condition. And you can also use the plt.xticks() function to set the label of the x-axis.
import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif'] = ['SimHei'] # 可以正常显示中文
plt.rcParams['axes.unicode_minus']=False #用来正常显示负号
plt.tight_layout() # 为了正常显示一些图表被遮盖或者不完全显示的问题
import random
import numpy as np
Understand axes, figure, plot and corresponding functions
The difference between axis, axes, and figure
Simply put, axis is the coordinate axis, axes is an added sub-area when drawing , and figure is a canvas for drawing pictures . The specific distinction is shown in the figure.
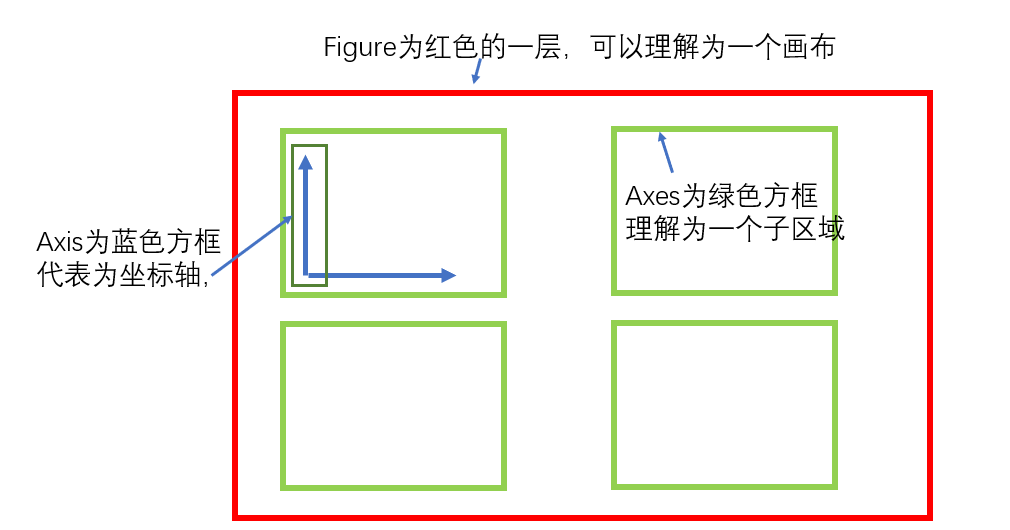
The difference between ax, figure, and plot
As shown above, ax generally represents an axes area. It is a submodule on the figure, but generally when using plt.plot(), it is used to obtain the current axes.
If figure is a sentence, ax is one of the elements such as flowers. That is, the figure contains ax.
Plot() is the most basic drawing function, and we generally add horizontal and vertical coordinate data to this drawing function.
Ax and figure are generally generated by the following three methods. Specifically as shown in the code.
# ax代码生成一般主要有以下三种方法
fig = plt.figure()
ax = fig.add_subplot(2, 2, 1)
ax = plt.subplot(2, 2, 1)
fig, ax = plt.subplots(2, 2) # 此时的ax为一个列表,List[list[axes]]
# fig生成一般有以下两种方法
fig = plt.figure()
fig, ax = plt.subplots(2, 2) # 此时的ax为一个列表,List[list[axes]]
ax_1 = ax[0,0]
ax_2 = ax[0,1]
x = np.random.randn(10)*10
y = np.random.randn(10)*10
ax_1.plot(x, y)
ax_2.plot(x, y)
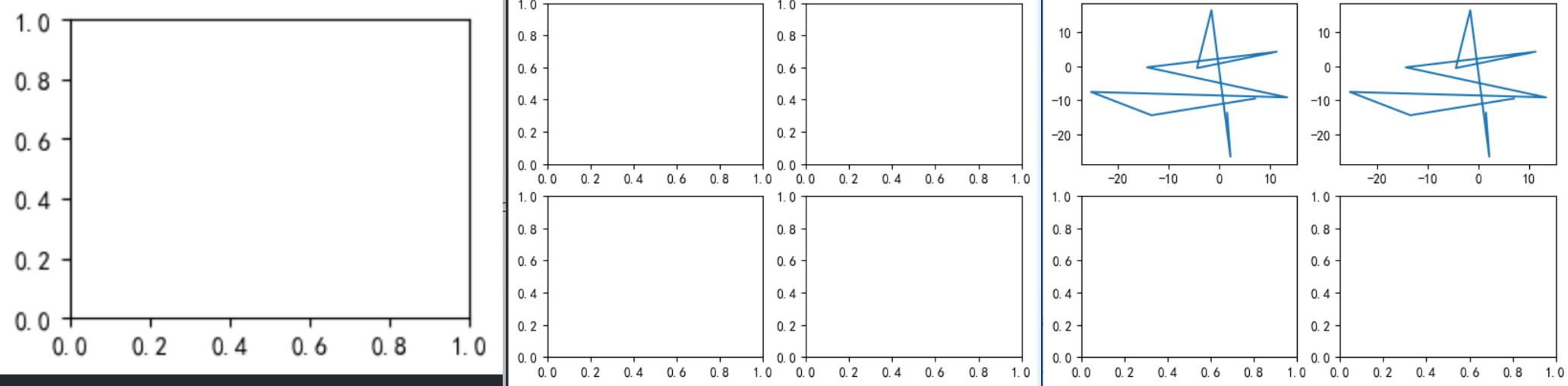
plt.subplots() function (generate figure and axes list objects)
The plt.subplots function specifically generates a figure canvas and multiple axes subregions.
fig, ax = plt.subplots(2,2)# 这里代表的是生成一共有四个axes的figure,即为一个画布上有四个对象,而且ax为第一个对象
# 2,2,1 代表将整个图像窗口分为两行两列,而且当前位置为1,
# 此时的ax为可以选择ax[0,0][0,1][1,0][1,1],但没有办法加入plt.subplots(2,2,1)
ax_2 = fig.add_subplot(2,2,1)
plt.subplot() function (generate axes object)
The words here are mainly to add the axes area. My personal understanding is to directly call the existing figure object to directly operate on the figure. If there is no fig = plt.figure(), a figure object will be automatically generated.
# fig = plt.figure()
ax = plt.subplot(2,2,1)
ax.plot(x,y)
plt.figure() function (generate figure object)
plt.figure() is to generate a figure, the parameters are name, figsize(), etc. as shown in the figure.
figure(name=int|str, figsize(width, height)),
if the name is the same, it will be drawn in the same axes object. If the name is different, redraw a figure object.
figsize mainly controls the size of the figure, and width is the width , height is height
fig = plt.figure(1, figsize(10,8))
x = np.random.randn(10)*10
y = np.random.randn(10)*10
plt.plot(x, y)
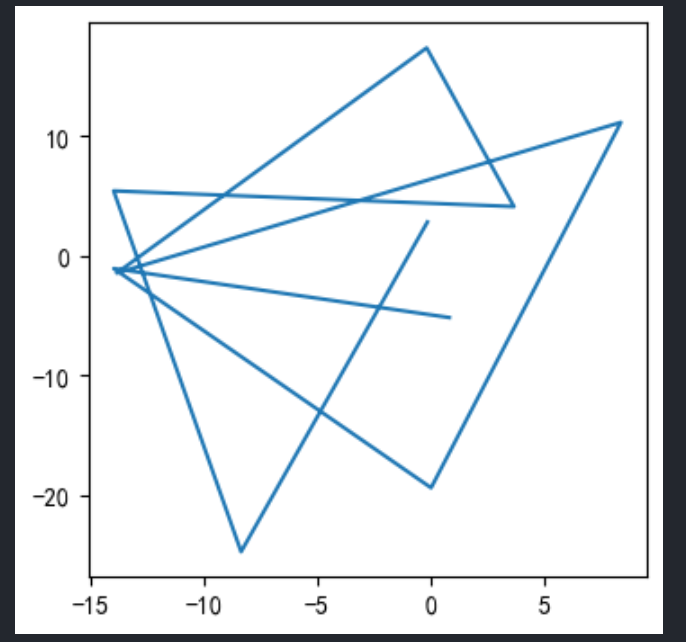
fig.add_subplot() function (personally thinks the most convenient and best)
The words here are generally placed on the premise that the figure has been generated, the effect will be much better, and I personally recommend this method.
fig = plt.figure()
ax = fig.add_subplot(2,2,1)
ax.plot(x,y)
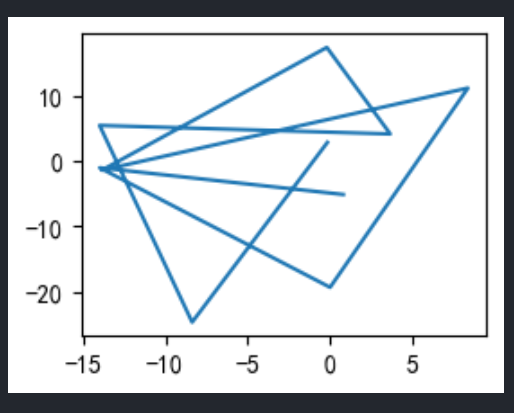
The plt.plot() function and the lines, point types, and colors corresponding to the drawn image
plt.plot(color='', linestyle='', marker='',markerfacecolor='', markersize='')
'''
color代表线段颜色
linestyle代表线段的类型
marker代表线段上的对应点的标识
markerfacecolor为标记(marker)的颜色
markersize为标记(marker)的尺寸
里面都需用''引号
'''
The main purpose is to draw a simple line graph , and the parameters are generally (x, y), which is the data of the x-axis and the data of the y-axis. In addition, there are some special legends that need to be explained and introduced later, such as drawing a straight line. Dashed lines, and shapes that show coordinates of points, etc.
This topic mainly introduces to draw the lines, point types and colors corresponding to the image.
plot(x, y, 'fmt')
fmt is mainly divided into three parameters, namely color, marker (point type), line style (linestyle)
plot(x, y, color='blue', marker='o', linestyle='-')
The color is mainly as follows, and the color can also be expressed in hexadecimal of #0000
'b' blue, 'g' green, 'r' red, 'c' cyan, '
k' black, 'w' white, 'm' magenta, 'y' yellow
对于其中点的形状主要如下所示。
'.' 为圆点, ',' 为pixel marker, 'o'为空心圆点, '^'为上三角形,
'v'为下三角形,'<'为左三角形,'>'为右三角形,'+'为十字标记,'x'为x标记
'D'为菱形标记,'*'Wie星形标记,'d'为瘦菱形标记,'|'为垂直线标记
对于linestyle主要有以下格式
'-'为实线,'--'为虚线,'-.'为点画线,即为一个点一个线交替,
':'为点线,即为全部由点构成
plt.show()函数
plt.show()函数比较简单,即为呈现所有的figure的图像。一般我们绘制出图片之后即可进行plt.show()调用之后进行可视化呈现。
plt.legend()函数
主要是运用调用pyplot函数的图例进行,我们这里理解的图例为如下图所示,简单应用如下,而且loc默认会选择最合适的位置进行放置。
x = np.random.randn(10)*10
y = np.random.randn(10)*10
plt.figure(num=1, figsize=(4,4))
plt.plot(x)
plt.plot(y,'r-^')
plt.legend(['one','two']) # 这里参考具体当前figure里面有几条线就用几个图例
plt.show()
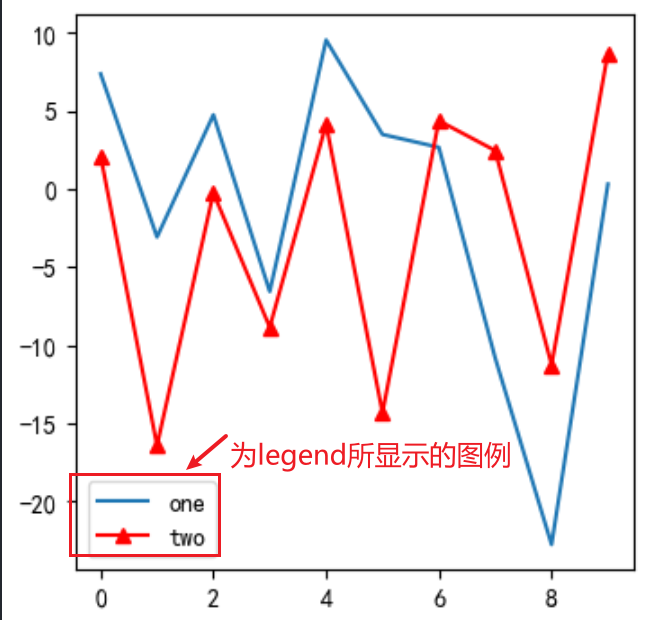
常见具体参数如下
plt.legend(loc='upper center') 这里的uppe是偏上方的意思,即为一般lower为偏下方,具体如下所示。一般默认是用0,即为选择最优的位置放置。
0: ‘best' 1: ‘upper right' 2: ‘upper left' 3: ‘lower left'
4: ‘lower right' 5: ‘right' 6: ‘center left' 7: ‘center right'
8: ‘lower center' 9: ‘upper center' 10: ‘center'
plt.legend(fontsize=int|float)为设置图例大小的数值
plt.legend(frameon=False)为去除图例边框,即为修改图例边框。
plt.legend(edgecolor='blue')设置图例边框颜色。
plt.legend(facecolor='blue')设置图例背景颜色,如果没有边框即为参数无效。
plt.axis()函数
plt.axis([xmin, xmax, ymin, ymax])参数具体可以放置四个数值,即为xmin,xmax,ymin,ymax,即为横纵坐标的最小值和最大值。
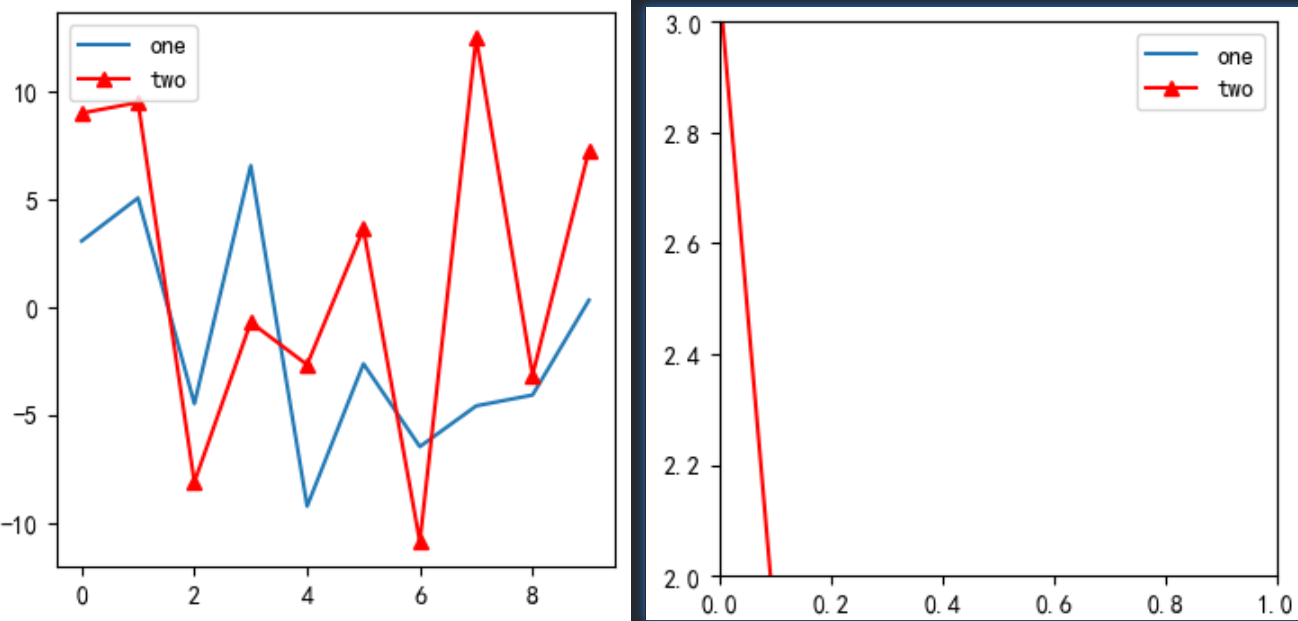
其他参数具体如下。
'on'显示坐标轴和坐标轴标签,
'off'为不显示坐标轴和坐标轴标签
具体效果如下图所示。很明显能够看出差距,而且我们可以设置具体
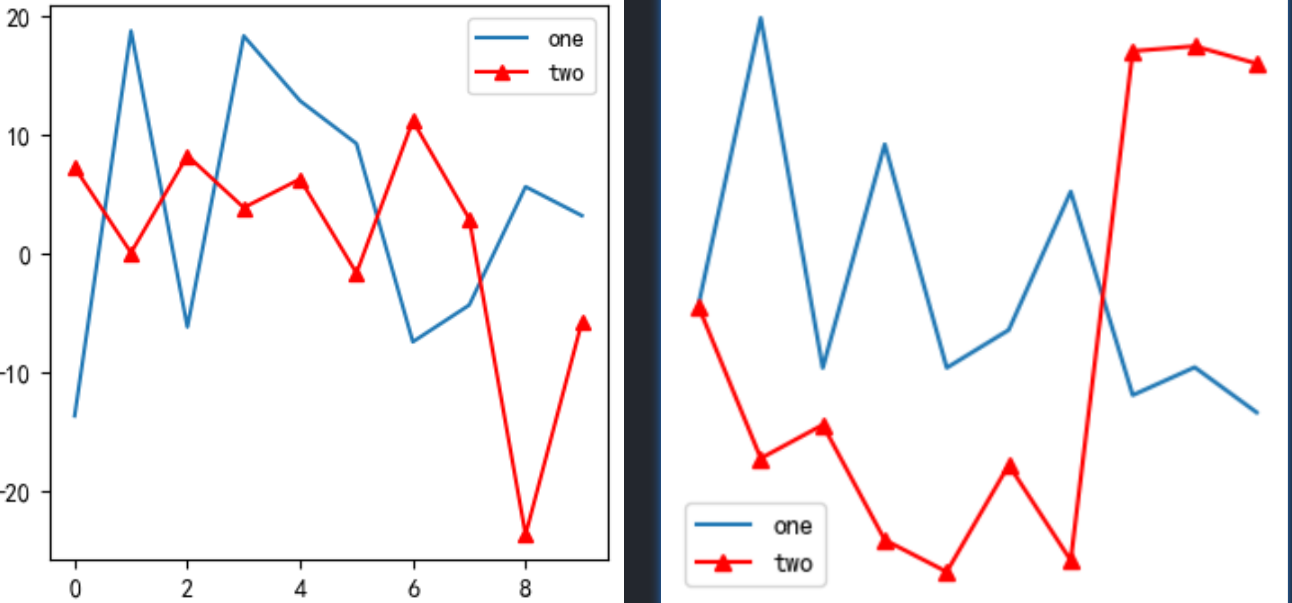
设置坐标轴刻度函数(xlim(), ylim()函数)
xlim(0,10)使得x坐标轴从0-10范围内可视化。
ylim(0,10)使得y坐标轴从0-10范围内可视化。
设置坐标轴的label(xlabel(), ylabel())函数
一般比较常用的是设置label的标签,即为我们在x,y轴上看到的说明或者汉字,常见的有以下参数,value,fontdict,loc。
loc:'left','right', 'center'
value:需要的文字
fontdict:设置字典样式,具体例子为如下fontdict={'fontsize': 10, 'fontstyle': 'oblique', 'color': 'red', 'fontfamily': 'fantasy'}
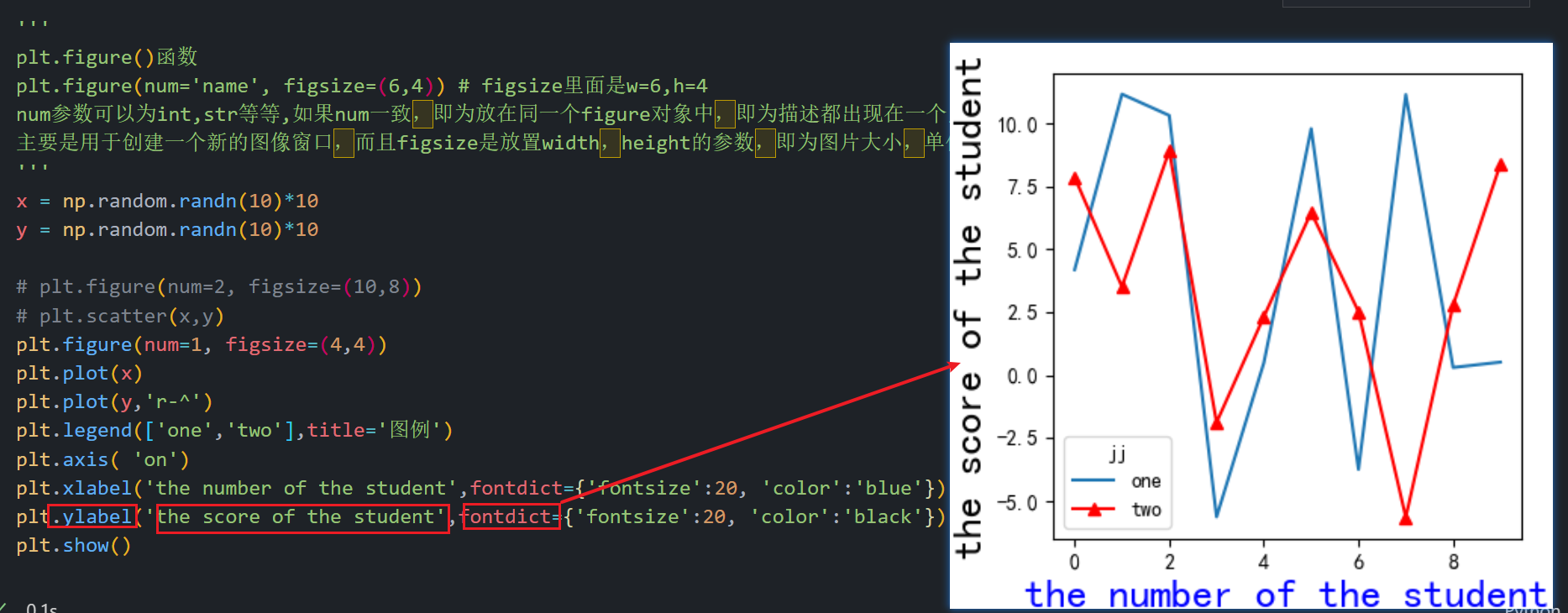
mathplotlib设置中文,负号正常显示
plt.rcParams['font.sans-serif'] = ['SimHei'] # 可以正常显示中文
plt.rcParams['axes.unicode_minus']=False #用来正常显示负号
plt.tight_layout() # 为了正常显示一些图表被遮盖或者不完全显示的问题
mathplotlib自定义坐标轴间隔
from matplotlib.pyplot import MultipleLocator
figure = plt.figure()
ax = figure.add_suplot(221)
number = 0.3 # 这里的话适用于所有的坐标轴间隔,我这里设置默认为10
x_major_locator=MultipleLocator(number)
# matplotlib 关于使用 MultipleLocator 自定义刻度间隔
ax.xaxis.set_major_locator(x_major_locator) # 这两个为设置坐标轴的间隔是否,而且为自己手动调整,需要导入MultipleLocator
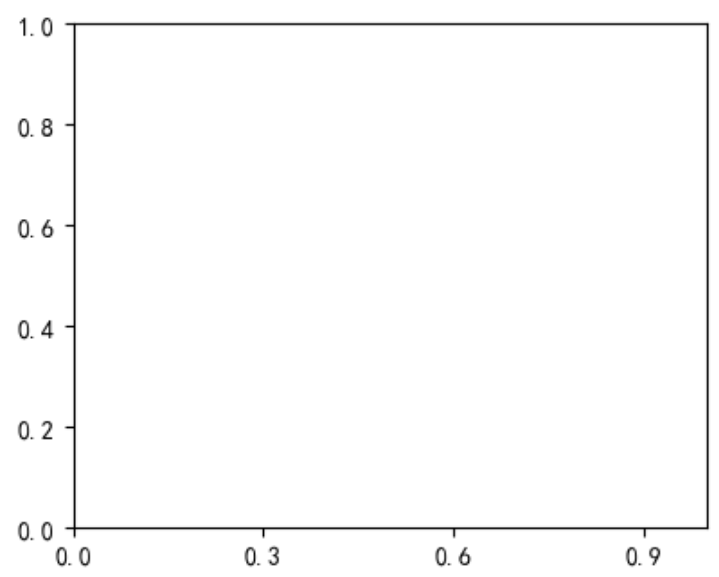
在figure和ax对象加入标题titile
plt.title('xxx')
ax.set_title('xxx')