Summary: The export declare const X: Y syntax is used to declare a constant variable of a specified type in an Angular application and export it for use in other files.
This article is shared from Huawei Cloud Community " About the usage of export declare const XY in Angular applications ", author: Jerry Wang.
When doing Angular development of Spartacus recently, I encountered the following TypeScript code:
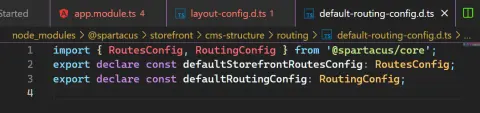
I don't understand the usage of declare inside, so I checked some information on the Internet to learn.
In Angular applications, export declare const X: Y means to declare a constant X and export it so that other modules can use it. Here X is the variable name and Y is the type. The export keyword is used to indicate that the constant can be imported and used in other modules, and the declare keyword indicates that the constant is defined elsewhere and does not need to be assigned a specific value. This is especially useful in TypeScript because it lets you define a type without an actual value.
In TypeScript, the declare keyword is used to tell the TypeScript compiler that a variable, constant or function has been defined elsewhere. This is useful when interacting with JavaScript libraries, because you can declare JavaScript library variables, constants, or functions in TypeScript without providing them with an actual TypeScript implementation.
For example, suppose you use a JavaScript library called myLibrary that provides a function called myFunction globally. You can declare this function in TypeScript using the declare keyword:
declare function myFunction(): void;
Now, we can call myFunction() in TypeScript code without compiling errors.
In TypeScript and Angular applications, the export keyword is used to export variables, constants, functions, interfaces or classes so that other modules can import and use them. This is the core concept of the TypeScript module system and the basis for code separation and reuse.
For example, you might have a module called constants.ts that exports a constant called API_URL:
export const API_URL = 'https://api.example.com';
You can then import and use the API_URL constant in other TypeScript modules:
import { API_URL } from './constants';
console.log(API_URL); // 输出 'https://api.example.com'
Here is an example explaining export declare const X: Y semantics in a more detailed manner:
// constants.ts 文件
export declare const API_URL: string;
export declare const MAX_ITEMS: number;
export declare const ENABLE_FEATURE: boolean;
// 使用常量的文件
import { API_URL, MAX_ITEMS, ENABLE_FEATURE } from './constants';
console.log(API_URL); // 输出:定义的 API_URL 值
console.log(MAX_ITEMS); // 输出:定义的 MAX_ITEMS 值
console.log(ENABLE_FEATURE); // 输出:定义的 ENABLE_FEATURE 值
In the above example, we defined several constant variables in the constants.ts file, namely API_URL, MAX_ITEMS and ENABLE_FEATURE. These constant variables are declared as exported, so they can be used in other files.
As a further example, suppose we have an application that needs to use the URL of an API as a constant. We can declare and export a constant variable named API_URL of type string in the constants.ts file as follows:
export declare const API_URL: string;
Then, import that constant variable and use it in other files:
import { API_URL } from './constants';
console.log(API_URL); // 输出:定义的 API_URL 值
In this way, we can uniformly define the URL of the API as a constant and reuse it throughout the application. If you need to change the URL of the API, you can just update the value of the constant in the constants.ts file instead of changing it one by one throughout the application.
In addition to constant variables of string type, the export declare const X: Y syntax is also applicable to other types of constant variables. Here are some examples of other types of constant variables:
export declare const PI: number; // 数字类型常量
export declare const COLORS: string[]; // 字符串数组类型常量
export declare const SETTINGS: {
theme: string;
enableNotifications: boolean;
}; // 对象类型常量
The specific semantics of these constant variables are similar to the above example, but the types are different. Depending on the needs of the application, we can use different types to define constant variables.
Summarize
The export declare const X: Y syntax is used to declare a constant variable of a specified type in an Angular application and export it for use in other files. In this way, we can define and manage constants in the application and ensure their consistency and maintainability throughout the application. This syntax is very useful when defining constant variables of different types such as strings, numbers, arrays, objects, etc., and can be used flexibly according to the needs of the application.
Click to follow and learn about Huawei Cloud's fresh technologies for the first time~