Refer to the official document: Python upload SDK-common file upload , get playback URL
This article only operates on uploading videos and obtaining a single video playback address. For more cases such as obtaining all video lists in batches, please refer to the official document directly: Media Management
1. Upload video: return a videoId parameter after the upload is completed, and then use this videoId to obtain the video playback address
from voduploadsdk.AliyunVodUtils import *
from voduploadsdk.AliyunVodUploader import AliyunVodUploader
from voduploadsdk.UploadVideoRequest import UploadVideoRequest
import re
filePath = "F:/Download/新增红色视频/丹心映红六盘山——夜宿单家集探寻“红色密码”.mp4"
# print(filename.rindex('/'))
# print(filename[filename.rindex('/')+1: filename.index('.')])
filename = filePath[filePath.rindex('/')+1: filePath.index('.')]
# 测试上传本地音视频
def testUploadLocalVideo(accessKeyId, accessKeySecret, filePath, storageLocation=None):
try:
# 可以指定上传脚本部署的ECS区域。如果ECS区域和视频点播存储区域相同,则自动使用内网上传,上传更快且更省公网流量。
# ecsRegionId ="cn-shanghai"
# uploader = AliyunVodUploader(accessKeyId, accessKeySecret, ecsRegionId)
# 不指定上传脚本部署的ECS区域。
uploader = AliyunVodUploader(accessKeyId, accessKeySecret)
uploadVideoRequest = UploadVideoRequest(filePath, filename)
# 可以设置视频封面,如果是本地或网络图片可使用UploadImageRequest上传图片到视频点播,获取到ImageURL
# ImageURL示例:https://example.com/sample-****.jpg
# uploadVideoRequest.setCoverURL('<your Image URL>')
# 标签
# uploadVideoRequest.setTags('tag1,tag2')
if storageLocation:
uploadVideoRequest.setStorageLocation(storageLocation)
videoId = uploader.uploadLocalVideo(uploadVideoRequest)
print("file: %s, videoId: %s" % (uploadVideoRequest.filePath, videoId))
except AliyunVodException as e:
print(e)
testUploadLocalVideo('yourAccessKeyID', "yourAccessKeySecret", filePath)
Replace yourAccessKeyID and yourAccessSecret in the code with the AccessKeyID and AccessKeySecret of your Alibaba Cloud main account or the AccessKeyID and AccessKeySecret of the RAM access control user
2. Obtain the video playback address according to the videoId: the parameter PlayURL in the return is the playback address
Official parameter description document: GetPlayInfo interface description
import json
import traceback
from aliyunsdkcore.client import AcsClient
def init_vod_client(accessKeyId, accessKeySecret):
regionId = 'cn-shanghai' # 点播服务接入地域
connectTimeout = 3 # 连接超时,单位为秒
return AcsClient(accessKeyId, accessKeySecret, regionId, auto_retry=True, max_retry_time=3, timeout=connectTimeout)
from aliyunsdkvod.request.v20170321 import GetPlayInfoRequest
def get_play_info(clt, videoId):
request = GetPlayInfoRequest.GetPlayInfoRequest()
request.set_accept_format('JSON')
request.set_VideoId(videoId)
request.set_AuthTimeout(3600*5) # 设置播放地址的有效时间。单位:秒。
response = json.loads(clt.do_action_with_exception(request))
return response
try:
clt = init_vod_client('yourAccessKeyID', "yourAccessKeySecret")
playInfo = get_play_info(clt, '4aa4f9c0b73c71ed80110675a0ec0102') # 第二个参数是videoID
print(json.dumps(playInfo, ensure_ascii=False, indent=4))
except Exception as e:
print(e)
print(traceback.format_exc())
Replace yourAccessKeyID and yourAccessSecret in the code with the AccessKeyID and AccessKeySecret of your Alibaba Cloud main account or the AccessKeyID and AccessKeySecret of the RAM access control user
Note: If an error is reported in operations such as uploading or obtaining addresses, it may be because the RAM user used does not have permission, just search for the user and add the corresponding permission
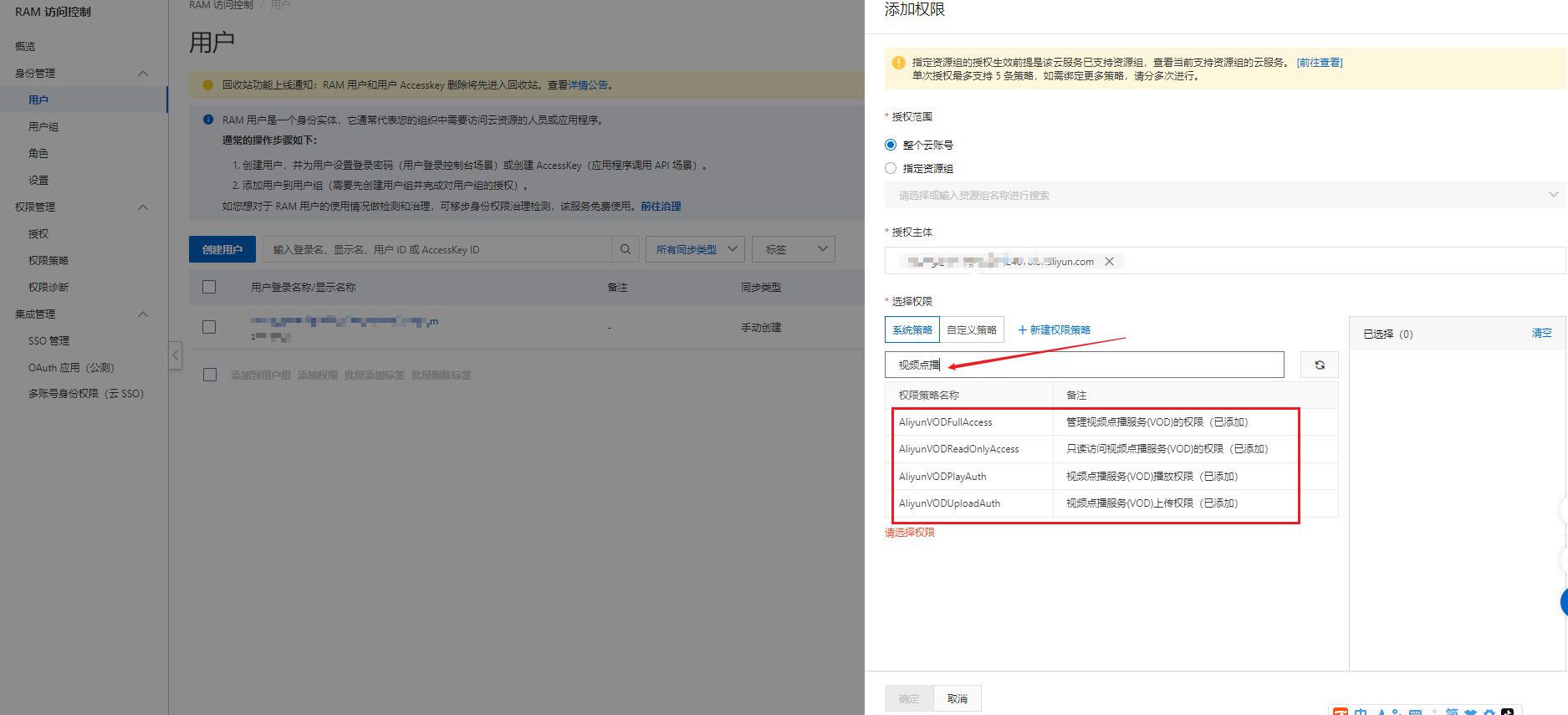
3. You can also use sts to get token and access
1. Obtain the AccessKeyID and AccessKeySecret from the user created in the RAM access control, and obtain the ARN from the created role (the method of obtaining these parameters is explained in detail in the previously published article, so I won’t go into details here)
2. Obtain SecurityToken, AccessKeyID and AccessKeySecret of STS through AccessKeyID, AccessKeySecret and ARN, which are used for subsequent access to identify the interface of picture text
Refer to the official api: STS SDK overview , security token acquisition (please fill in the necessary parameters in the API interface and copy the code)
from aliyunsdkcore.client import AcsClient
from aliyunsdkcore.acs_exception.exceptions import ClientException
from aliyunsdkcore.acs_exception.exceptions import ServerException
from aliyunsdkcore.auth.credentials import AccessKeyCredential
from aliyunsdkcore.auth.credentials import StsTokenCredential
from aliyunsdksts.request.v20150401.AssumeRoleRequest import AssumeRoleRequest
credentials = AccessKeyCredential('yourAccessKeyID', 'yourAccessSecret')
# use STS Token
# credentials = StsTokenCredential('<your-access-key-id>', '<your-access-key-secret>', '<your-sts-token>')
client = AcsClient(region_id='cn-shanghai', credential=credentials)
request = AssumeRoleRequest()
request.set_accept_format('json')
policy_text = '{"Version": "1","Statement": [{"Action": "vod:*","Resource": "*","Effect": "Allow"}]}' # 策略
request.set_RoleArn("yourRoleArn") # 在RAM访问控制中的角色里获取ARN
request.set_RoleSessionName("client") # 任意写
request.set_Policy(policy_text)
response = client.do_action_with_exception(request)
# python2: print(response)
print(str(response, encoding='utf-8'))
Please replace yourAccessKeyID, yourAccessKeySecret and yourRoleArn in the above code with the values obtained in the first step
2. Use the SecurityToken request interface to obtain data
import json
import traceback
from aliyunsdkcore.client import AcsClient
from aliyunsdkcore.auth.credentials import StsTokenCredential
from aliyunsdkvod.request.v20170321 import GetPlayInfoRequest
from aliyunsdkvod.request.v20170321 import CreateUploadVideoRequest
def init_vod_client(accessKeyId, accessKeySecret, securityToken):
regionId = 'cn-shanghai' # 点播服务接入地域
connectTimeout = 3 # 连接超时,单位为秒
credential = StsTokenCredential(accessKeyId, accessKeySecret, securityToken)
return AcsClient(region_id=regionId, auto_retry=True, max_retry_time=3, timeout=connectTimeout, credential=credential)
def get_play_info(clt, videoId):
request = GetPlayInfoRequest.GetPlayInfoRequest()
request.set_accept_format('JSON')
request.set_VideoId(videoId)
request.set_AuthTimeout(3600*5)
response = json.loads(clt.do_action_with_exception(request))
return response
try:
clt = init_vod_client('yourAccessKeyID', 'yourAccessSecret', "yourSecurityToken")
playInfo = get_play_info(clt, '20b87bd0b73e71ed801d0674a2ce0102') # 第二个参数是videoID
print(json.dumps(playInfo, ensure_ascii=False, indent=4))
except Exception as e:
print(e)
print(traceback.format_exc())
Replace yourAccessKeyID, yourAccessKeySecret, and yourSecurityToken in the above code with the SecurityToken, AccessKeyId, and AccessKeySecret returned by the code in the third and second small steps