Minesweeper
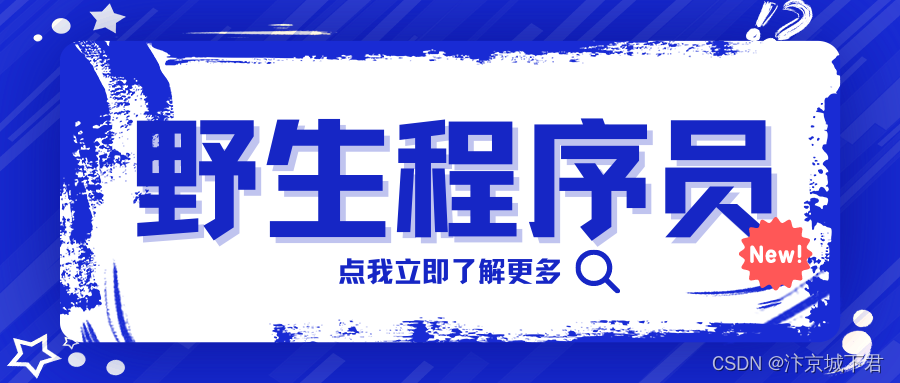
development process
- Preparation
- Show results
Preparation:
game.h a header file –> declare the function
test.c as the main file
game.c as the function implementation file
Show results
Development idea
-
menu interface
-
Game interface printing (two-dimensional array)
-
random spawn of thunder
-
Demining (and judging the number of mines around)
-
victory condition
The general idea is like this, let's analyze it step by step
menu interface
void menu()
{
printf("*********************\n");
printf("*** 1.play 0.exit ***\n");
printf("*********************\n");
}
here we have to keep going Receive user input, when it is 1, enter the game, when it is 0, exit the program
directly think of conditional statement judgment, here we use switch
Continuous : After the user finishes the game, the menu can be printed again for the user to choose
This operation is of course the core part of the project, placed inmain() functioninside
int main()
{
int input = 0;
//设置随机数 后面会讲
srand((unsigned int)time(NULL));
do
{
menu();
printf("请输入:>");
scanf("%d", &input);
switch (input)
{
case 1:
game();
break;
case 0:
printf("退出游戏");
break;
default:
printf("输入有误!!!");
break;
}
} while (input);
return 0;
}
Printing of the game interface
Of course, a two-dimensional array is used here, but we have to consider whether it can achieve the effect of uninformed demining if only one array is used.
Of course, an array is not possible, so we have to createtwo arrays,
one is real , and the other is shown to the user
char mine[ROWS][COLS] = {
0 };//存放布置好的雷的信息
char show[ROWS][COLS] = {
0 };//存放排查出雷的信息
Here we have to think about it first. If the array is 9×9, when it is on the edge, it willout of bounds
We can create an array of 11×11, of which we do not use the outermost, just set as the default value
#define ROW 9
#define COL 9
#define ROWS ROW+2
#define COLS COL+2
Initialization of the map
//mine 数组在没有布置雷的时候全为 0
InitBoard(mine, ROWS, COLS, '0');
//show 数组在没有排查雷的时候全为 *
InitBoard(show, ROWS, COLS, '*');
Our InitBoard function is:
void InitBoard(char board[ROWS][COLS], int rows, int cols, char set)
{
int i = 0, j = 0;
for (i = 0; i < rows; i++)
{
for (j = 0; j < cols; j++)
{
board[i][j] = set;
}
}
}
printing of maps
void DisplayBoard(char board[ROWS][COLS], int row, int col)
{
int i = 0, j = 0;
printf("-----------扫雷游戏--------------\n");
//打印上面0~9注释
for (j = 0; j <= col; j++)
{
printf("%d ", j);
}
printf("\n");
for (i = 1; i <= row; i++)
{
printf("%d ", i); //打印竖着1~9的注释
for (j = 1; j <= col; j++)
{
printf("%c ", board[i][j]);
}
printf("\n");
}
printf("-----------扫雷游戏--------------\n");
}
random spawn of thunder
It is easy for us to think that it is to generate random numbers and change the element values of its array.
That's itsrand() function
//设置随机数的起始
srand((unsigned int)time(NULL));
Randomly generated function:
We define EASY_COUNT (the number of mines) in the header file
void SetMine(char board[ROWS][COLS], int row, int col)
{
int count = EASY_COUNT;
//横纵坐标1~9
while (count)
{
int d = rand() % row + 1;
int c = rand() % col + 1;
//随机生成十个雷,雷:1
if (board[d][c] == '0')
{
board[d][c] = '1';
count--;
}
}
}
Minesweeper and determine victory conditions
Minesweeper
Let the user enter a coordinate and judge its value
- If the coordinate in the show array is *, the coordinate has been cleared
- If the coordinate in the mine array is 1, it will fail to be bombed by mine
- Otherwise, determine how many mines there are in the mine array and display the number at the coordinates of the show array
Determine whether to repeatedly enter coordinates
if (show[x][y] != '*')
{
printf("该坐标已经排查过了,请重新输入:>\n");
}
The failed code is very simple, just a judgment
if (mine[x][y] == '1') //是雷
{
printf("被炸死咯\n");
DisplayBoard(mine, ROW, COL);
break;
}
The function of judging how many mines are around is
int get_mine_count(char board[ROWS][COLS], int x, int y)
{
return (board[x - 1][y] +
board[x - 1][y - 1] +
board[x - 1][y + 1] +
board[x][y + 1] +
board[x][y - 1] +
board[x + 1][y - 1] +
board[x + 1][y] +
board[x + 1][y + 1]- 8*'0');
}
The function of the whole operation is
void FindMine(char mine[ROW][COL], char show[ROW][COL], int row, int col)
{
int x = 0, y = 0;
int a = 0, b = 0;
int win = 0;
while (win<row*col-EASY_COUNT)//判断胜利条件,win小于整体-雷数
{
printf("请输入排查的坐标:>\n");
scanf("%d %d", &a, &b);
x = a + 1;
y = b + 1;
if (x >= 1 && x <= row && y >= 1 && y <= col)
{
if (show[x][y] != '*')
{
printf("该坐标已经排查过了,请重新输入:>\n");
}
else
{
if (mine[x][y] == '1') //是雷
{
printf("被炸死咯\n");
DisplayBoard(mine, ROW, COL);
break;
}
else //不是雷 '0'-'0'=0 '1'-'0'=1 字符->整型
// ASCII码值分别为 '1':49 '0':48
{
int count = get_mine_count(mine, x, y);//统计该坐标周围有几颗雷
show[x][y] = count + '0';//转换成数字字符
//printf("%c\n", count + '0');
DisplayBoard(show, ROW, COL);
win++;
}
}
}
else
{
printf("输入坐标错误,请重新输入:>\n");
}
}
if (win == row * col - EASY_COUNT)
{
printf("恭喜你,扫雷成功!!!\n");
DisplayBoard(mine, ROW, COL);
}
}
code integration
test.c
#include "game.h"
void menu()
{
printf("*********************\n");
printf("*** 1.play 0.exit ***\n");
printf("*********************\n");
}
void game()
{
char mine[ROWS][COLS] = {
0 };//存放布置好的雷的信息
char show[ROWS][COLS] = {
0 };//存放排查出雷的信息
//初始化数组的内容为指定内容
//mine 数组在没有布置雷的时候全为 0
InitBoard(mine, ROWS, COLS, '0');
//show 数组在没有排查雷的时候全为 *
InitBoard(show, ROWS, COLS, '*');
//DisplayBoard(mine,ROW, COL);
//设置雷
SetMine(mine, ROW, COL);
//展示
DisplayBoard(show, ROW, COL);
//排雷
FindMine(mine,show,ROW,COL);
}
int main()
{
int input = 0;
//设置随机数
srand((unsigned int)time(NULL));
do
{
menu();
printf("请输入:>");
scanf("%d", &input);
switch (input)
{
case 1:
game();
break;
case 0:
printf("退出游戏");
break;
default:
printf("输入有误!!!");
break;
}
} while (input);
return 0;
}
game.c
#include "game.h"
void InitBoard(char board[ROWS][COLS], int rows, int cols, char set)
{
int i = 0, j = 0;
for (i = 0; i < rows; i++)
{
for (j = 0; j < cols; j++)
{
board[i][j] = set;
}
}
}
void DisplayBoard(char board[ROWS][COLS], int row, int col)
{
int i = 0, j = 0;
printf("-----------扫雷游戏--------------\n");
for (j = 0; j <= col; j++)
{
printf("%d ", j);
}
printf("\n");
for (i = 1; i <= row; i++)
{
printf("%d ", i);
for (j = 1; j <= col; j++)
{
printf("%c ", board[i][j]);
}
printf("\n");
}
printf("-----------扫雷游戏--------------\n");
}
void SetMine(char board[ROWS][COLS], int row, int col)
{
int count = EASY_COUNT;
//横纵坐标1~9
while (count)
{
int d = rand() % row + 1;
int c = rand() % col + 1;
//随机生成十个雷,雷:1
if (board[d][c] == '0')
{
board[d][c] = '1';
count--;
}
}
}
int get_mine_count(char board[ROWS][COLS], int x, int y)
{
return (board[x - 1][y] +
board[x - 1][y - 1] +
board[x - 1][y + 1] +
board[x][y + 1] +
board[x][y - 1] +
board[x + 1][y - 1] +
board[x + 1][y] +
board[x + 1][y + 1]- 8*'0');
}
void FindMine(char mine[ROW][COL], char show[ROW][COL], int row, int col)
{
int x = 0, y = 0;
int a = 0, b = 0;
int win = 0;
while (win<row*col-EASY_COUNT)//判断胜利条件,win小于整体-雷数
{
printf("请输入排查的坐标:>\n");
scanf("%d %d", &a, &b);
x = a + 1;
y = b + 1;
if (x >= 1 && x <= row && y >= 1 && y <= col)
{
if (show[x][y] != '*')
{
printf("该坐标已经排查过了,请重新输入:>\n");
}
else
{
if (mine[x][y] == '1') //是雷
{
printf("被炸死咯\n");
DisplayBoard(mine, ROW, COL);
break;
}
else //不是雷 '0'-'0'=0 '1'-'0'=1 字符->整型
// ASCII码值分别为 '1':49 '0':48
{
int count = get_mine_count(mine, x, y);//统计该坐标周围有几颗雷
show[x][y] = count + '0';//转换成数字字符
//printf("%c\n", count + '0');
DisplayBoard(show, ROW, COL);
win++;
}
}
}
else
{
printf("输入坐标错误,请重新输入:>\n");
}
}
if (win == row * col - EASY_COUNT)
{
printf("恭喜你,扫雷成功!!!\n");
DisplayBoard(mine, ROW, COL);
}
}
game.h
#pragma once
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
#define ROW 9
#define COL 9
#define ROWS ROW+2
#define COLS COL+2
#define EASY_COUNT 10 //雷
void InitBoard(char board[ROWS][COLS], int rows, int cols, char set);
void DisplayBoard(char board[ROWS][COLS], int row, int col);
void SetMine(char board[ROWS][COLS],int row,int col);
void FindMine(char mine[ROW][COL],char show[ROW][COL], int row, int col);