This lecture is mainly
- C# data types
- type conversion
- operator
- expression
C# data types
In C#, variables are divided into the following types:
- Value types
- Reference types
- Pointer types
Value types
Value type variables can be assigned directly to a value. They are derived from the class System.ValueType.
Value types contain data directly. For example, int, char, and float, which store numbers, characters, and floating point numbers respectively. When you declare an int type, the system allocates memory to store the value.
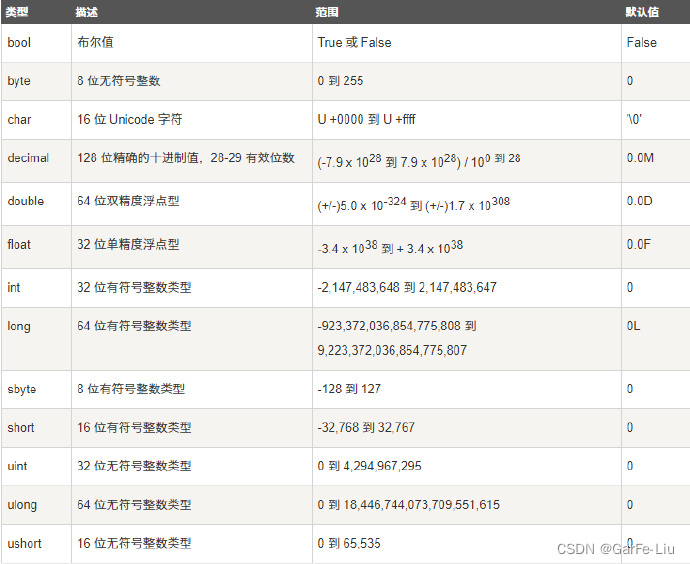
To get the exact size of a type or a variable on a particular platform, use the sizeof method. The expression sizeof(type) yields the storage size in bytes of the stored object or type. The following example obtains the storage size of int type on any machine:
using System;
namespace DataTypeApplication
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Size of int: {0}", sizeof(int));
Console.ReadLine();
}
}
}
When the above code is compiled and executed, it produces the following result:
Size of int: 4
Reference types
Reference types do not contain the actual data stored in the variable, but they contain a reference to the variable.
In other words, they refer to a memory location. A reference type can point to a single memory location when using multiple variables. If the data at a memory location is changed by one variable, the other variables automatically reflect this change in value. The built-in reference types are: object, dynamic, and string.
Object (Object) type
The Object (Object) type is the ultimate base class for all data types in the C# Common Type System (CTS). Object is an alias for the System.Object class. So the Object (Object) type can be assigned the value of any other type (value type, reference type, predefined type or user-defined type). However, before assigning a value, a type conversion is required.
When a value type is converted to an object type, it is called
boxing
; on the other hand, when an object type is converted to a value type, it is called
unboxing
.
object obj;
obj = 100; // this is boxing
int num = (int)obj; //this is unboxing
Dynamic type
You can store any type of value in a dynamic data type variable. Type checking of these variables happens at runtime.
Syntax for declaring dynamic types:
dynamic = value;
For example:
dynamic d = 20;
Dynamic typing is similar to object typing, but type checking for object type variables happens at compile time, whereas type checking for dynamic type variables happens at runtime.
Popularize the following compile-time/run-time:
Compile time and runtime are used in software development to describe two different phases of software development. The source code needs to be compiled into a program that the machine can recognize. This compilation process is called compile time. Users can run compiled programs, and the process of program execution is called runtime
.
String (String) type
String (String) type allows you to assign any string value to the variable. The String (String) type is an alias for the System.String class. It is derived from the Object (Object) type. Values of type String can be assigned in two forms: quotes and @quotes.
For example:
String str = "runoob.com";
An @quoted string:
@"runoob.com";
C# string can be preceded by @ (called "verbatim string") to treat escape characters (\) as ordinary characters, such as:
string str = @"C:\Windows";
Equivalent to:
string str = "C:\\Windows";
@ Any newline can be used in the string, and newline characters and indentation spaces are counted within the length of the string.
string str = @"
";
User-defined reference types are: class, interface or delegate. We will discuss these types in later chapters.
Pointer types
A variable of pointer type stores another type of memory address. Pointers in C# have the same functionality as pointers in C or C++.
The syntax for declaring a pointer type is:
type* identifier;
For example:
char* cptr;
int* iptr;
type conversion
Type casting is basically type casting, or converting data from one type to another. In C#, type casting comes in two flavors:
- Implicit Type Conversions − These conversions are the default conversions in C# that are performed in a safe manner and do not result in loss of data. For example, converting from a small integer type to a large integer type, and from a derived class to a base class.
- Explicit Type Conversions - Explicit type conversions, i.e. casts. Explicit conversions require a casting operator, and casting causes data loss.
C# type conversion method
C# provides the following built-in type conversion methods:
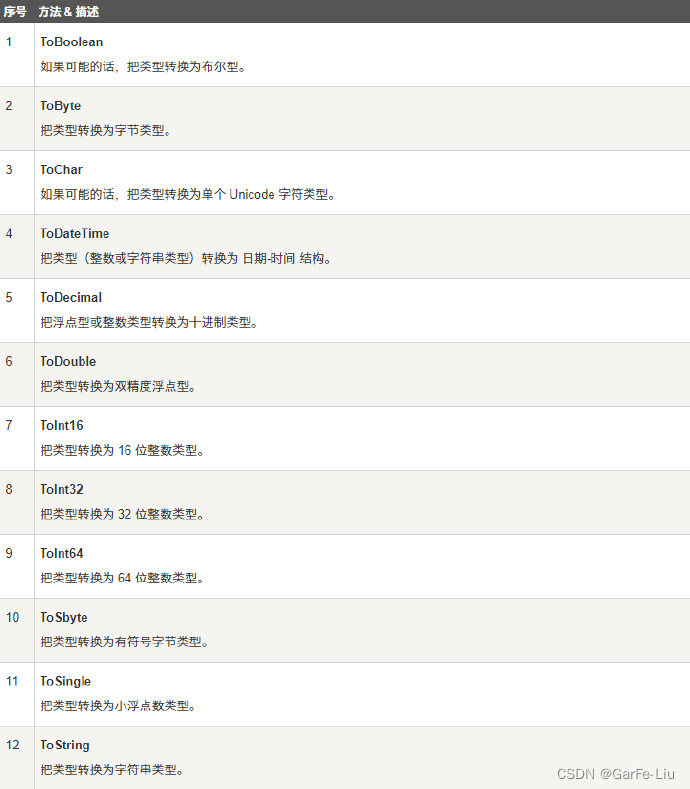
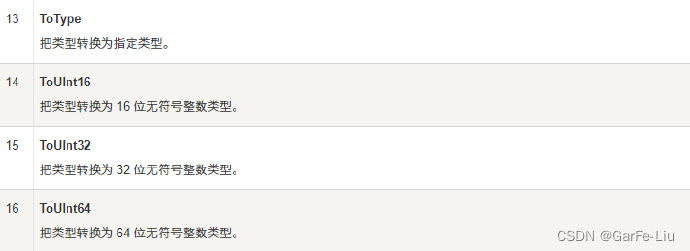
operator
An operator is a symbol that tells the compiler to perform a specific mathematical or logical operation. C# has a wealth of built-in operators, categorized as follows:
- arithmetic operator
- relational operator
- Logical Operators
- bitwise operator
- assignment operator
- other operators
arithmetic operator
The following table shows all arithmetic operators supported by C#. Assuming variable A has a value of 10 and variable B has a value of 20, then:
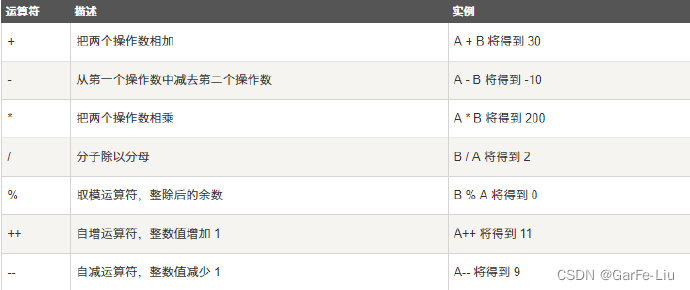
Note on usage of self-increment and self-decrement:
c =
a++
: assign a to c first, and then perform self-increment operation on a.
c =
++a
: first perform self-increment operation on a, and then assign a to c.
c =
a--
: first assign a to c, and then perform a self-decrement operation on a.
c =
--a
: first decrement a, and then assign a to c.
using System;
namespace OperatorsAppl
{
class Program
{
static void Main(string[] args)
{
int a = 1;
int b;
// a++ 先赋值再进行自增运算
b = a++;
Console.WriteLine("a = {0}", a);
Console.WriteLine("b = {0}", b);
Console.ReadLine();
// ++a 先进行自增运算再赋值
a = 1; // 重新初始化 a
b = ++a;
Console.WriteLine("a = {0}", a);
Console.WriteLine("b = {0}", b);
Console.ReadLine();
// a-- 先赋值再进行自减运算
a = 1; // 重新初始化 a
b= a--;
Console.WriteLine("a = {0}", a);
Console.WriteLine("b = {0}", b);
Console.ReadLine();
// --a 先进行自减运算再赋值
a = 1; // 重新初始化 a
b= --a;
Console.WriteLine("a = {0}", a);
Console.WriteLine("b = {0}", b);
Console.ReadLine();
}
}
}
Execute the above program, the output is:
a = 2
b = 1
a = 2
b = 2
a = 0
b = 1
a = 0
b = 0
relational operator
The following table shows all relational operators supported by C#. Assuming variable A has a value of 10 and variable B has a value of 20, then:
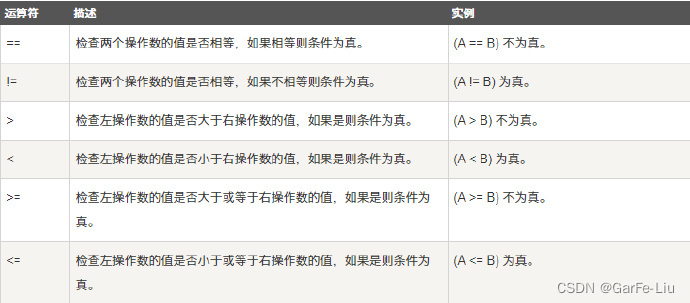
Logical Operators
The following table shows all logical operators supported by C#. Assuming variable A is Boolean true and variable B is Boolean false, then:
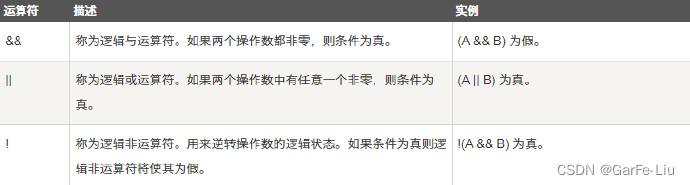
bitwise operator
Bitwise operators operate on bits and perform operations bit by bit. The truth tables for &, |, and ^ are as follows:
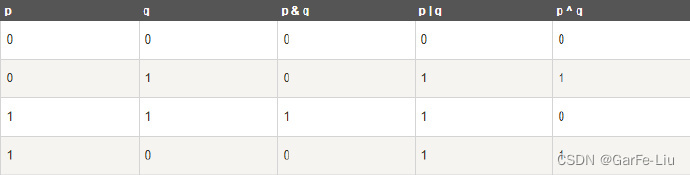
Suppose if A = 60, and B = 13, now represented in binary format, they look like this:
A = 0011 1100
B = 0000 1101
-----------------
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~A = 1100 0011
The following table lists the bitwise operators supported by C#. Assuming variable A has a value of 60 and variable B has a value of 13, then:
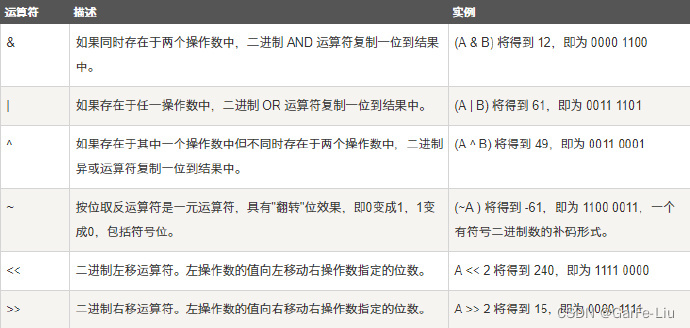
assignment operator
The following table lists the assignment operators supported by C#:
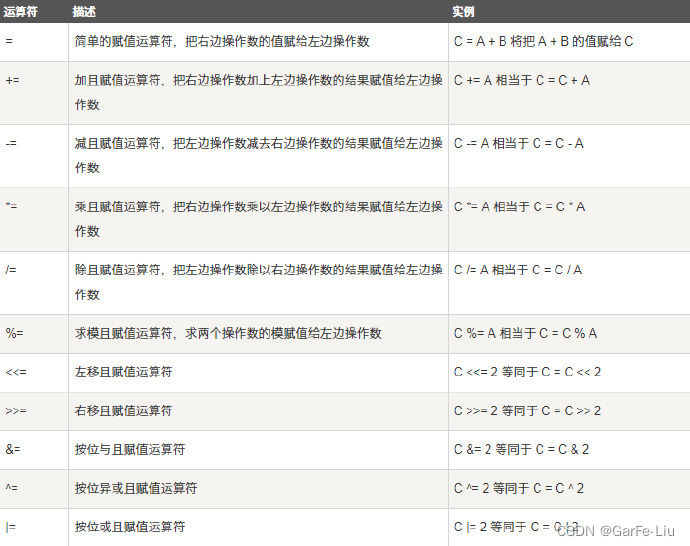
other operators
The following table lists some other important operators supported by C#, including sizeof, typeof, and ?:.
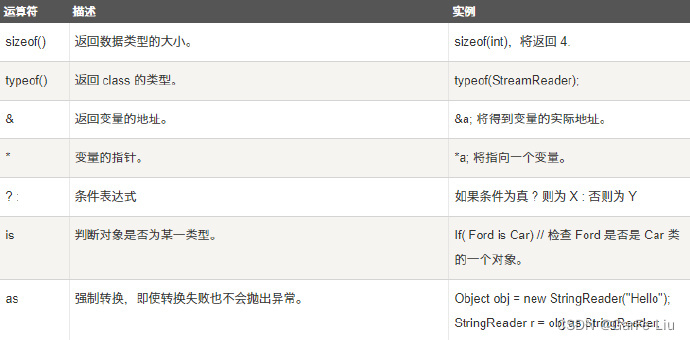
Operator precedence in C#
The precedence of operators determines the combination of terms in an expression. This affects how an expression is evaluated. Some operators have higher precedence than others, for example, the multiplication and division operators have higher precedence than the addition and subtraction operators.
For example x = 7 + 3 * 2, here, x is assigned a value of 13, not 20, because the operator * has a higher priority than +, so the multiplication 3*2 is calculated first, and then 7 is added.
The following table lists the operators in descending order of operator precedence, with operators with higher precedence appearing above the table and operators with lower precedence appearing below the table. In expressions, operators with higher precedence are evaluated first.
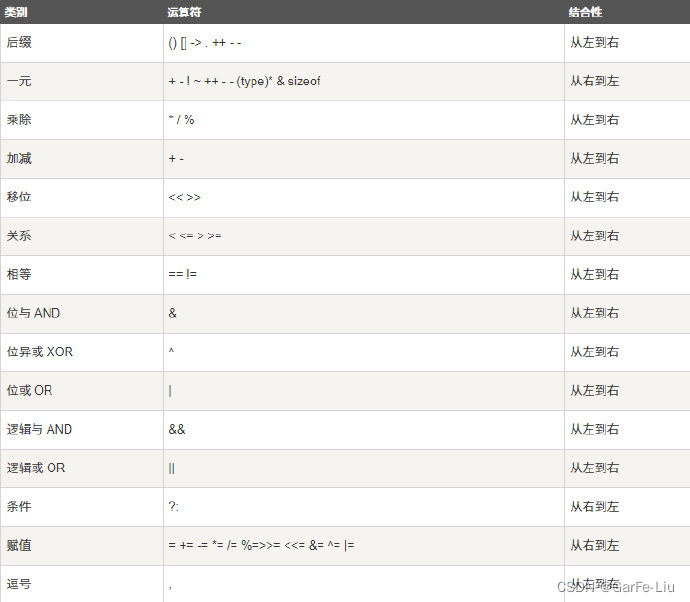
Special explanation:
&, |, ^ are not only used for bit operations, but also for logic operations, corresponding to AND, OR, and XOR respectively.
^ Operator
The binary ^ operator is predefined for integral types and bool. For integral types, ^ computes the bitwise exclusive-or of its operands. For bool operands, ^ computes the logical exclusive-or of its operands;
that is, the result is true if and only if one of its operands is true.
Console.WriteLine(true ^ false); // return true
Console.WriteLine(false ^ false); // 返回 false
Console.WriteLine(true ^ true); // returns false
| Operator The
binary | operator is predefined for integral types and bool. For integral types, | computes the bitwise OR of its operands. For bool operands, | computes the logical OR of its operands;
that is, the result is false if and only if both of its operands are false.
Console.WriteLine(true | false); // return true
Console.WriteLine(false | false); // 返回 false
& Operator
The binary & operator is predefined for integral types and bool. For integral types, & computes the logical bitwise AND of its operands. For bool operands, & computes the logical AND of its operands;
that is, the result is true if and only if both of its operands are true.
Console.WriteLine(true & false); // returns false
Console.WriteLine(true & true); // return true
The operation results of & and | are exactly the same as && and ||, but the performance of && and || is better
. Because both && and || check the value of the first operand, if the result can already be judged, the second operand is not processed at all.
for example:
bool a = true;
boolean b = false;
bool c = a || b;
When checking the first operand a, c has already been found to be true, so there is no need to process the second operand b.
regular expression
A regular expression
is a pattern that matches input text. The .Net framework provides a regular expression engine that allows this kind of matching. Patterns consist of one or more characters, operators, and structures.
define regular expressions
The various categories of characters, operators, and constructs used to define regular expressions are listed below.
- character escape
- character class
- location point
- group structure
- qualifier
- backreference construct
- Alternate configuration
- replace
- Miscellaneous construction
character escape
A backslash character (\) in a regular expression indicates that the character following it is special or should be interpreted literally.
The following table lists escape characters:
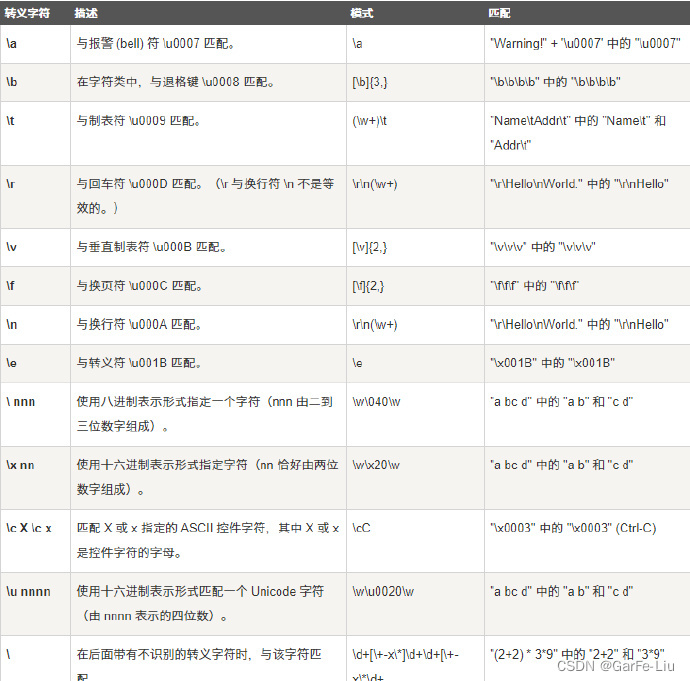
character class
A character class matches any one character in a set of characters.
The following table lists the character classes:
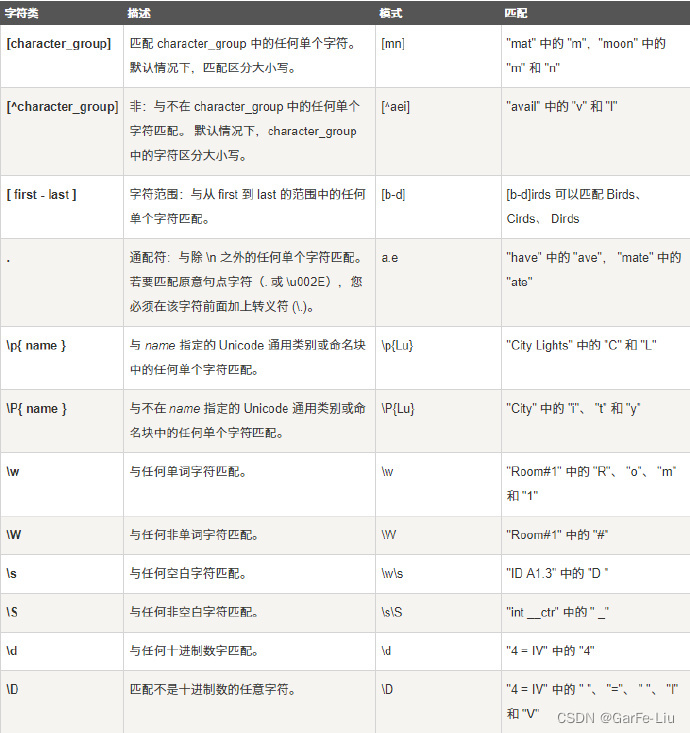
location point
Anchors or atomic zero-width assertions cause the match to succeed or fail depending on the current position in the string, but they do not cause the engine to advance or consume characters in the string.
The following table lists the anchor points:
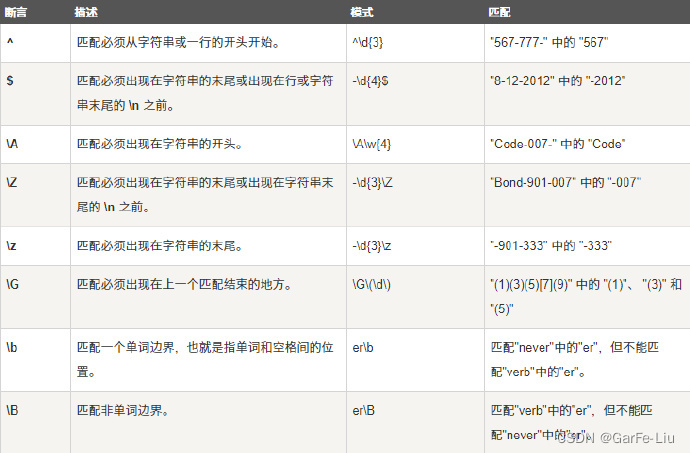
group structure
Grouping constructs describe subexpressions of regular expressions and are typically used to capture substrings of the input string.
The following table lists the grouping constructs:
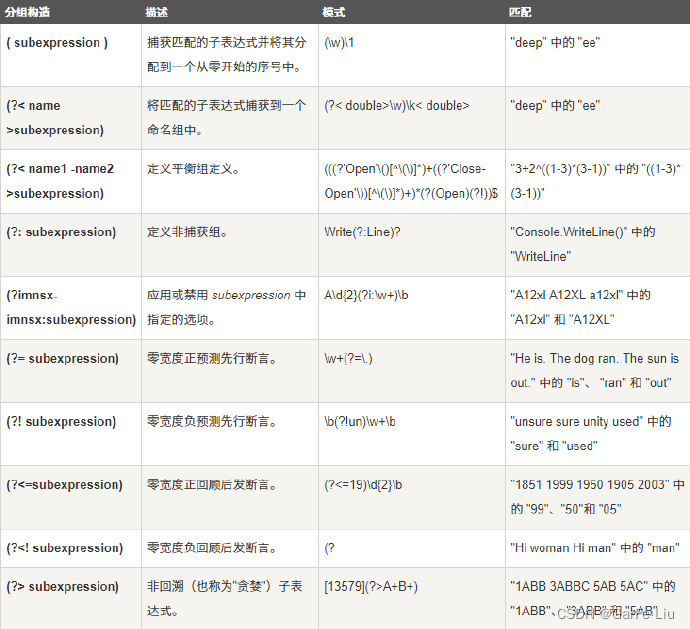