1. Introduction to python gui (graphical) module:
Tkinter: is the simplest graphical module of python, with only 14 components in total
Pyqt: is the most complex and widely used graphics for python
Wx: It is a graphic centered in python, and the learning structure is very clear
Pywin: It is a module under python windows, camera control (opencv), often used in plug-in production
Second, the installation of the wx module:
1 |
C:\Users\Administrator> pip install wxpython |
3. Graphical introduction
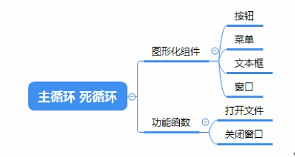
……

4. Introduction of main components of wx
1. frame ( window )
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
参数: parent = None #父元素,假如为None,代表顶级窗口 id = None #组件的标识,唯一,假如id为-1代表系统分配id title = None #窗口组件的名称 pos = None #组件的位置,就是组件左上角点距离父组件或者桌面左和上的距离 size = None #组件的尺寸,宽高 style = None #组件的样式 name = None #组件的名称,也是用来标识组件的,但是用于传值 |
2. TextCtrl (text box)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
参数: parent = None #父元素,假如为None,代表顶级窗口 id = None #组件的标识,唯一,假如id为-1代表系统分配id value = None #文本框当中的内容 GetValue #获取文本框的值 SetValue #设置文本框的值 pos = None #组件的位置,就是组件左上角点距离父组件或者桌面左和上的距离 size = None #组件的尺寸,宽高 style = None #组件的样式 validator = None #验证 name = None #组件的名称,也是用来标识组件的,但是用于传值 |
3. Button (button)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
参数: parent = None #父元素,假如为None,代表顶级窗口 id = None #组件的标识,唯一,假如id为-1代表系统分配id lable = None #按钮的标签 pos = None #组件的位置,就是组件左上角点距离父组件或者桌面左和上的距离 size = None #组件的尺寸,宽高 style = None #组件的样式 validator = None #验证 name = None #组件的名称,也是用来标识组件的,但是用于传值 |
The parameters of other components are similar
4. Create window base code
1 2 3 4 5 |
基本创建窗口代码说明:<br><br> import wx #引入wx模块<br> app = wx.App() #实例化一个主循环<br> frame = wx.Frame( None ) #实例化一个窗口<br> frame.Show() #调用窗口展示功能<br> app.MainLoop() #启动主循环 |
The effect is as follows:
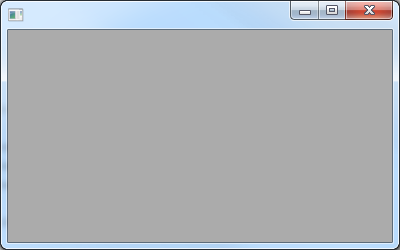
Five, Gui write a simple example
Implement the following GUI interface, enter the address of the text file in the upper text box, and click the "Open" button to display the content of the text file in the lower text box.
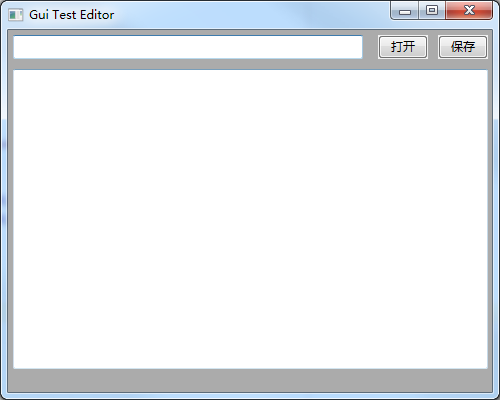
1. Graphical writing
1 2 3 4 5 6 7 8 |
import wx app = wx.App() frame = wx.Frame( None ,title = "Gui Test Editor" ,pos = ( 1000 , 200 ),size = ( 500 , 400 )) path_text = wx.TextCtrl(frame,pos = ( 5 , 5 ),size = ( 350 , 24 )) open_button = wx.Button(frame,label = "打开" ,pos = ( 370 , 5 ),size = ( 50 , 24 )) save_button = wx.Button(frame,label = "保存" ,pos = ( 430 , 5 ),size = ( 50 , 24 ))content_text = wx.TextCtrl(frame,pos = ( 5 , 39 ),size = ( 475 , 300 ),style = wx.TE_MULTILINE) # wx.TE_MULTILINE可以实现换行功能,若不加此功能文本文档显示为一行显示frame.Show() app.MainLoop() |
2. Event binding
1 2 3 4 5 6 7 8 9 10 11 |
1 、定义事件函数 事件函数有且只有一个参数,叫event def openfile(event): path = path_text.GetValue() with open (path, "r" ,encoding = "utf-8" ) as f: #encoding 设置文件打开时指定为utf8编码,避免写文件时出现编码错误 content_text.SetValue(f.read()) 2 、绑定出发事件的条件和组件 open_button.Bind(wx.EVT_BUTTON,openfile) |
3. Complete code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
#coding:utf-8 import wx def openfile(event): # 定义打开文件事件 path = path_text.GetValue() with open (path, "r" ,encoding = "utf-8" ) as f: # encoding参数是为了在打开文件时将编码转为utf8 content_text.SetValue(f.read()) app = wx.App() frame = wx.Frame( None ,title = "Gui Test Editor" ,pos = ( 1000 , 200 ),size = ( 500 , 400 )) path_text = wx.TextCtrl(frame,pos = ( 5 , 5 ),size = ( 350 , 24 )) open_button = wx.Button(frame,label = "打开" ,pos = ( 370 , 5 ),size = ( 50 , 24 )) open_button.Bind(wx.EVT_BUTTON,openfile) # 绑定打开文件事件到open_button按钮上 save_button = wx.Button(frame,label = "保存" ,pos = ( 430 , 5 ),size = ( 50 , 24 )) content_text = wx.TextCtrl(frame,pos = ( 5 , 39 ),size = ( 475 , 300 ),style = wx.TE_MULTILINE) # wx.TE_MULTILINE可以实现以滚动条方式多行显示文本,若不加此功能文本文档显示为一行 frame.Show() app.MainLoop() |
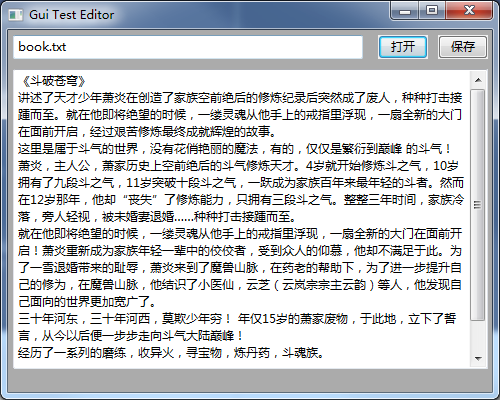
6. Sizer
According to the GUI code above, there is a flaw. Since each of our components has a fixed size, when the frame is stretched, the corresponding components will not be stretched accordingly, which will affect the user experience.
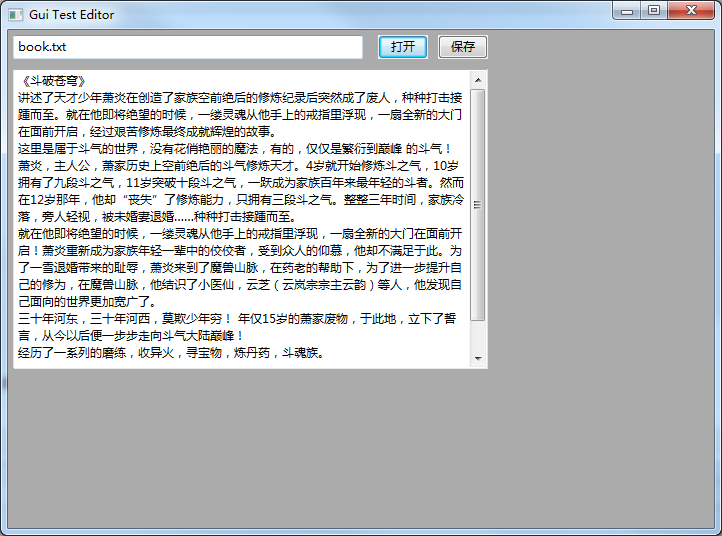
In order to solve the above problem, we can use the sizer for layout, which is similar to the CSS style of HTML.
1. BoxSizer (sizer)
- The sizer acts on the canvas (panel)
- default horizontal layout
- Vertical layout can be adjusted
- in relative proportion
2. Steps
- Instantiate sizers (can be multiple)
- Add components to different sizers
- Set parameters such as relative proportion, fill style and direction, border, etc.
- set master sizer
3. Rewrite the above code through the sizer
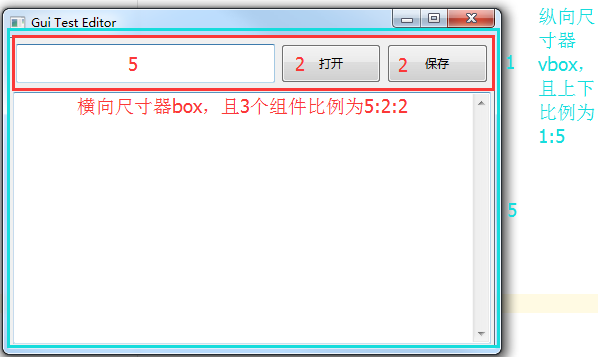
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
#coding:utf-8 import wx def openfile(event): # 定义打开文件事件 path = path_text.GetValue() with open (path, "r" ,encoding = "utf-8" ) as f: # encoding参数是为了在打开文件时将编码转为utf8 content_text.SetValue(f.read()) app = wx.App() frame = wx.Frame( None ,title = "Gui Test Editor" ,pos = ( 1000 , 200 ),size = ( 500 , 400 )) panel = wx.Panel(frame) path_text = wx.TextCtrl(panel) open_button = wx.Button(panel,label = "打开" ) open_button.Bind(wx.EVT_BUTTON,openfile) # 绑定打开文件事件到open_button按钮上 save_button = wx.Button(panel,label = "保存" ) content_text = wx.TextCtrl(panel,style = wx.TE_MULTILINE) # wx.TE_MULTILINE可以实现以滚动条方式多行显示文本,若不加此功能文本文档显示为一行 box = wx.BoxSizer() # 不带参数表示默认实例化一个水平尺寸器 box.Add(path_text,proportion = 5 ,flag = wx.EXPAND|wx. ALL ,border = 3 ) # 添加组件 #proportion:相对比例 #flag:填充的样式和方向,wx.EXPAND为完整填充,wx.ALL为填充的方向 #border:边框 box.Add(open_button,proportion = 2 ,flag = wx.EXPAND|wx. ALL ,border = 3 ) # 添加组件 box.Add(save_button,proportion = 2 ,flag = wx.EXPAND|wx. ALL ,border = 3 ) # 添加组件 v_box = wx.BoxSizer(wx.VERTICAL) # wx.VERTICAL参数表示实例化一个垂直尺寸器 v_box.Add(box,proportion = 1 ,flag = wx.EXPAND|wx. ALL ,border = 3 ) # 添加组件 v_box.Add(content_text,proportion = 5 ,flag = wx.EXPAND|wx. ALL ,border = 3 ) # 添加组件 panel.SetSizer(v_box) # 设置主尺寸器 frame.Show() app.MainLoop() |
Layout through the sizer, no matter how the wide body is stretched, the internal components will change proportionally.
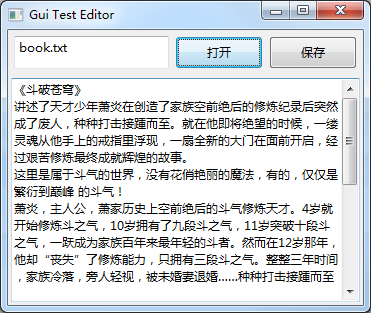