brief introduction
Recently, I encountered the situation of using the Properties class to read and write configuration files for IO in my work, so I became interested in this kind. After studying online, I come here to summarize. Let's first look at some source code
public class Properties extends Hashtable<Object,Object> {
/**
* use serialVersionUID from JDK 1.1.X for interoperability
*/
@java.io.Serial
private static final long serialVersionUID = 4112578634029874840L;
private static final Unsafe UNSAFE = Unsafe.getUnsafe();
You can see that this class inherits from HashTable<k,v>, and of course implements the Map<k,v> interface, so it can be used as a Map, and it is also a double-column collection, where k and v are characters string type. Let's take an example to demonstrate the collection properties of Properties
@Test
void contextLoads() {
Properties prop = new Properties();
prop.put("name","Superman");
prop.put("age",18);
System.out.println(prop.toString());
prop.clear();
System.out.println(prop.toString());
}
Let's look at the output
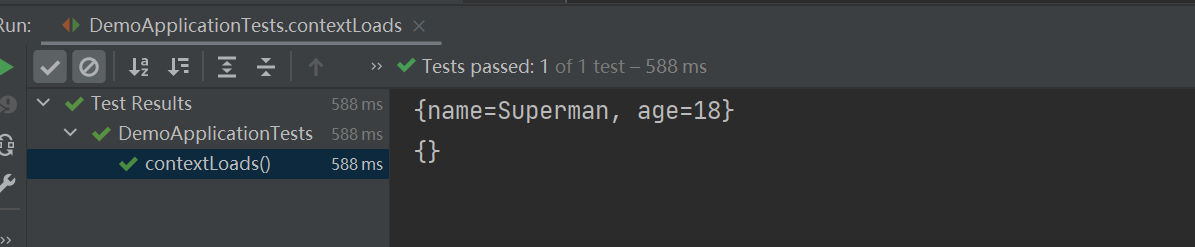
You can see that the collection is stored in the form of key-value. The most commonly used Properties scene is definitely the .properties configuration file. Let's see if this class can read the configuration file.
@Test
void contextLoads() {
File file = new File("src/main/resources/demo.properties");
File file1 = new File("src/main/resources/demo1.yml");
Properties properties = new Properties();
try(FileInputStream fileInputStream = new FileInputStream(file);
InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream, "UTF-8"))
{
properties.load(inputStreamReader);//读取输入字符流
System.out.println(properties);
System.out.println(properties.getProperty("name"));
}catch (FileNotFoundException | UnsupportedEncodingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
The two configuration files are as follows:
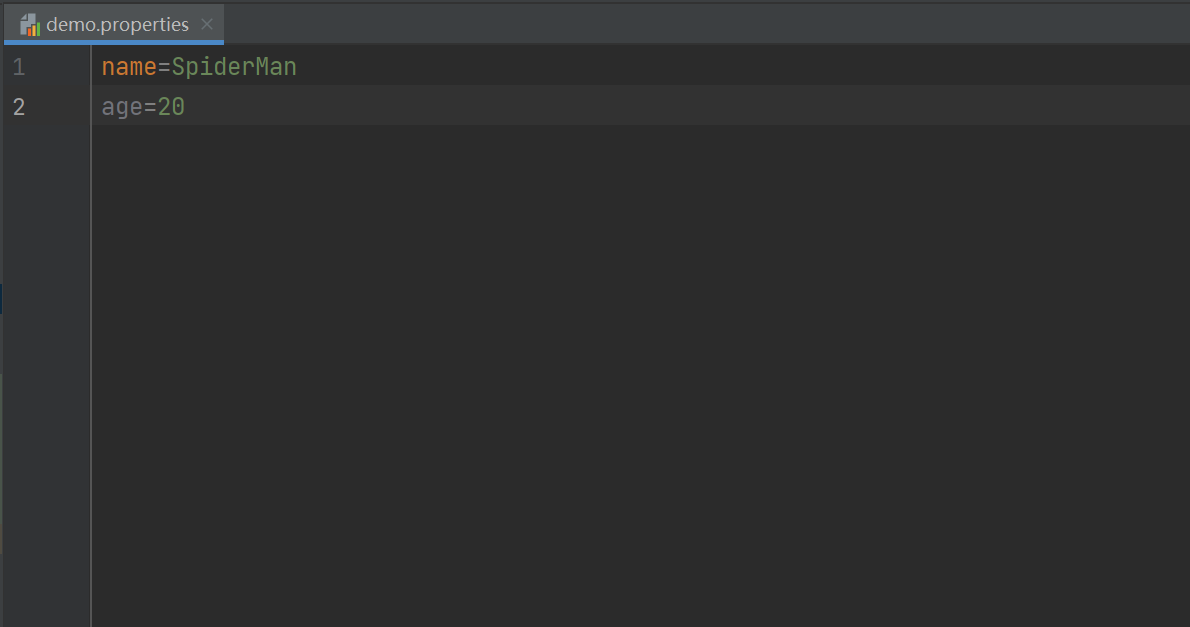
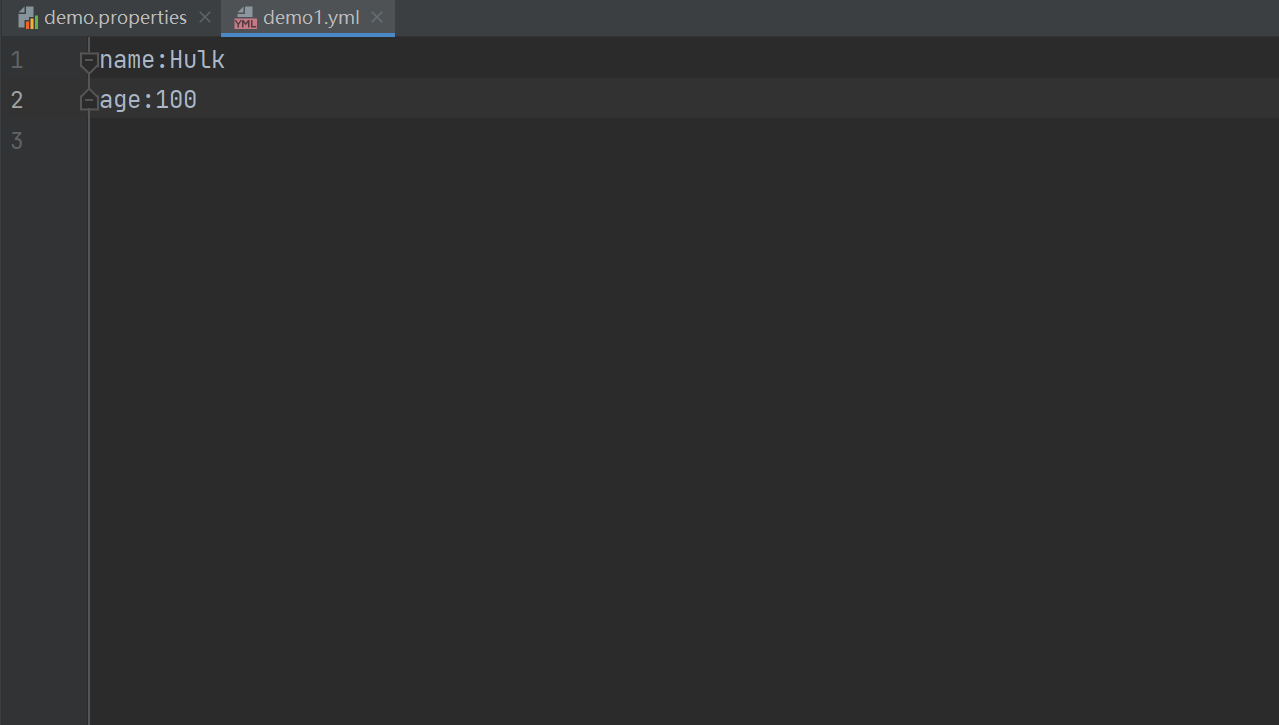
Let's take a look at the results of reading the two configuration files separately
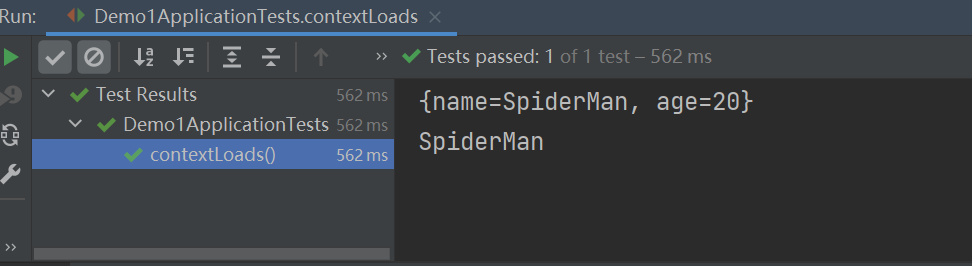
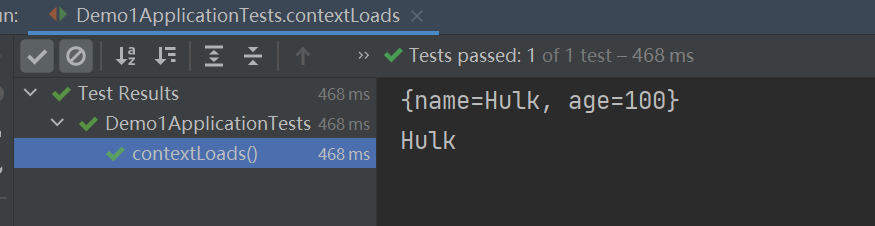
We can see that the load method parameter can be passed to the input character stream Reader reader, and can also be passed to the input byte stream InputStream inputStream. are all readable. Let's look at another method of this class, the replace method
File file = new File("src/main/resources/demo.properties");
File file1 = new File("src/main/resources/demo1.yml");
Properties properties = new Properties();
try(FileInputStream fileInputStream = new FileInputStream(file);
InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream, "UTF-8"))
{
properties.load(inputStreamReader);
System.out.println(properties);
System.out.println(properties.getProperty("name"));
properties.replace("name","SpiderMan","yang");
System.out.println(properties);
} catch (FileNotFoundException | UnsupportedEncodingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
Let's look at the results:
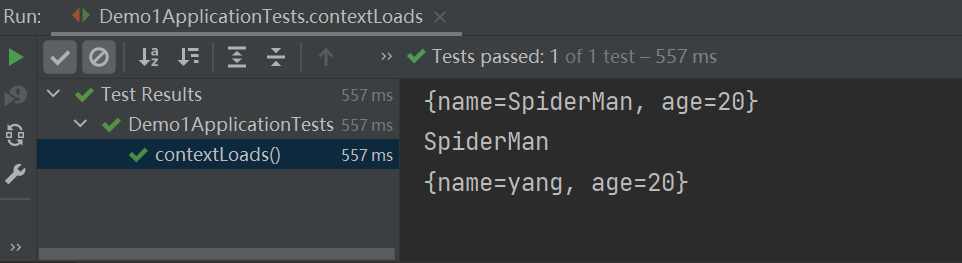
It can be seen that the properties in the Properties collection have changed, but if we want to modify the configuration file, we will not only read, but also write to the hard disk. Is it possible? We add the following code:
try {
final FileOutputStream fileOutputStream = new FileOutputStream(file);
properties.store(fileOutputStream,"change");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
look at the results
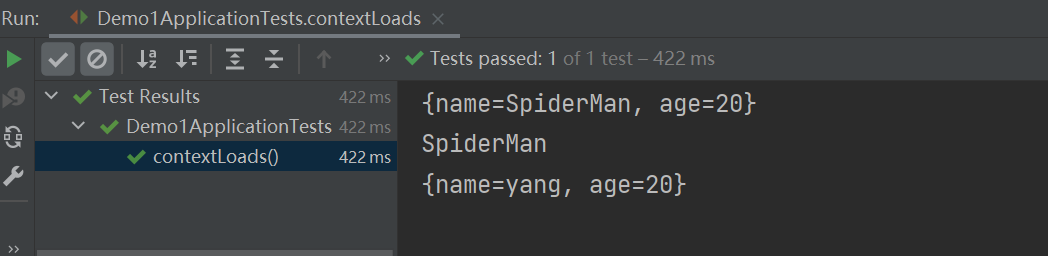
The output has not changed, look at the source files in resources
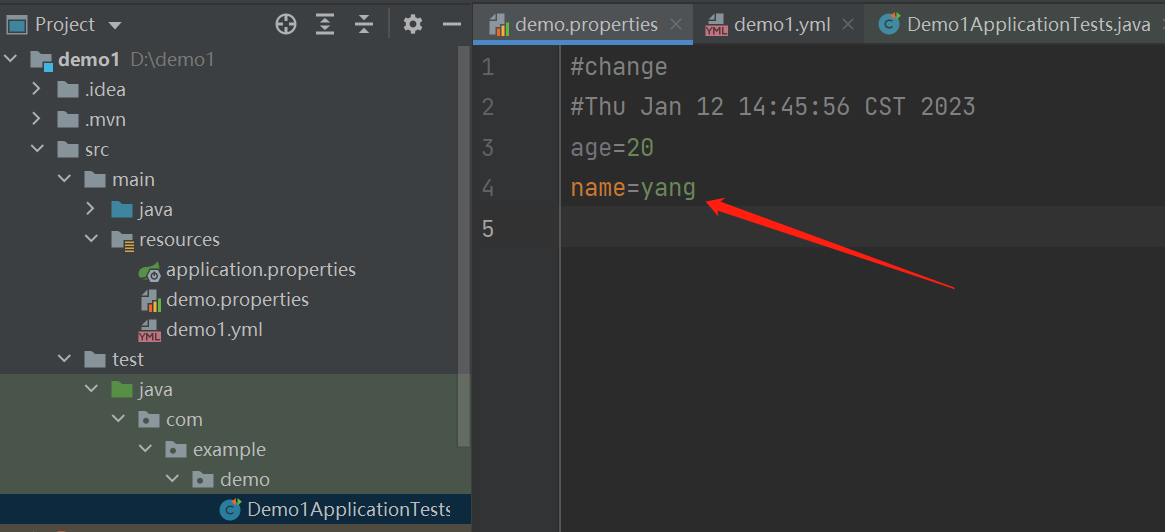
You can see that it has been modified, and there is also a comment when the modification is made. The Properties class actually has many other applications, basically all about configuration files. For example, we can use the getProperties method of System to obtain all the parameter information of the current JVM, and print it out to the console. The relevant example code is as follows:
@SpringBootTest
public class JVMPropertiesTest {
@Test
void testDemo(){
Properties properties = System.getProperties();
properties.list(System.out);
}
}
Related display information:
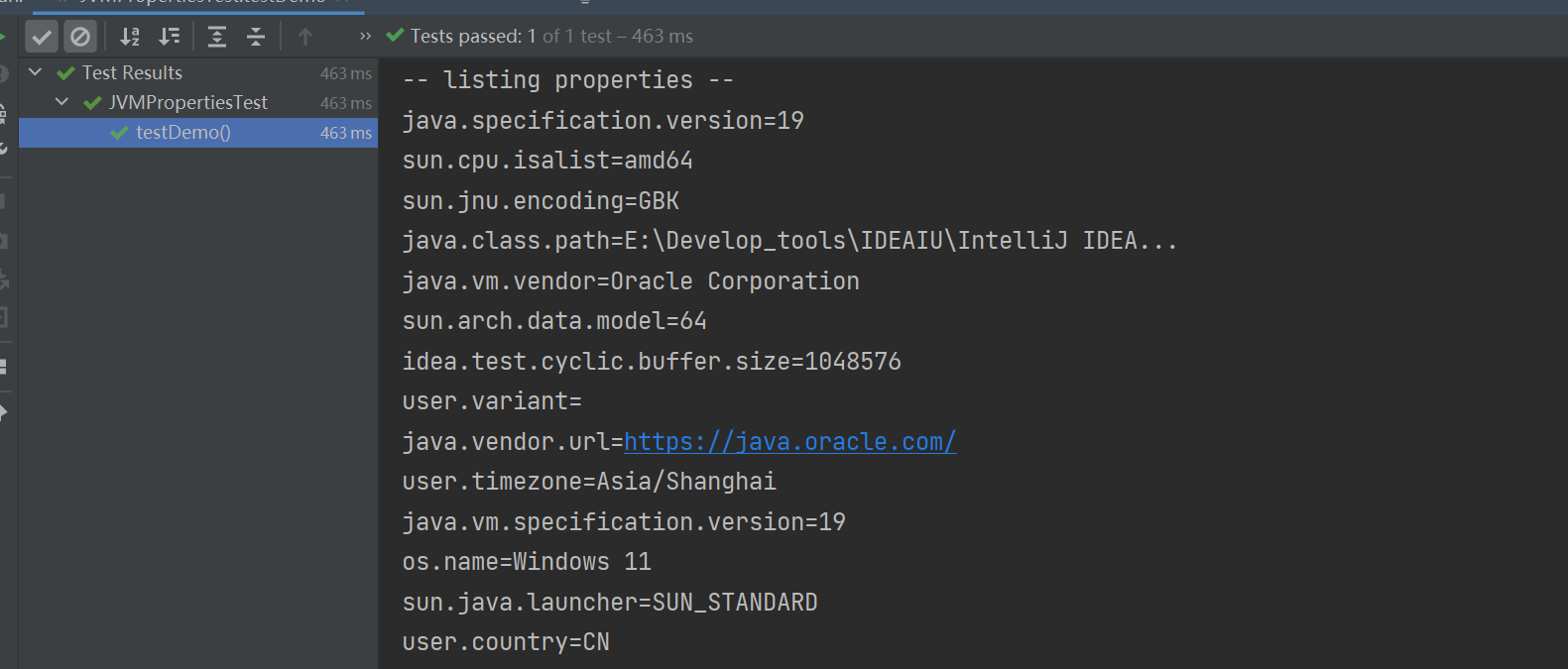
There are still many parameters that are not cut out. We can use the stream to output to the document for easy viewing.
The above is what I have learned about this class so far, and I will share it with you.