Preface: In project development, it is often necessary to display some text in different styles, such as: bolding new lines, adding image link adjustments, etc. At this time, most of them use Spannable that comes with Android. If there are fewer special style fonts, Spannable or html is still possible. However, if the client needs to support complex styles of copywriting, Spannable is not very flexible. In this case, many friends will think of using rich text HTML, that is, using WebView, but WebView consumes more performance and is not suitable for use in the message table. , and then everyone will think of using MarkDown, but Android's native support for MarkDown is not very good, so everyone generally imports libraries to do it.
The better libraries packaged online are: RichText and Markwon
RichText:https://github.com/zzhoujay/RichText
Rich text parser under Android platform
streaming operation
low invasive
Less dependencies, only disklrucache and support v4
Support Html and Markdown format text
Support image click and long press events
Link click event and long press event
Supports setting images during loading and loading errors
Support click callback for custom hyperlinks
Support for correcting image width and height
Support GIF pictures
Support Base64 encoding, local pictures and Assets catalog pictures
Self-supporting custom image loader, image loader
Support memory and disk double buffering
Support for custom Html parsers has been added
Effect:
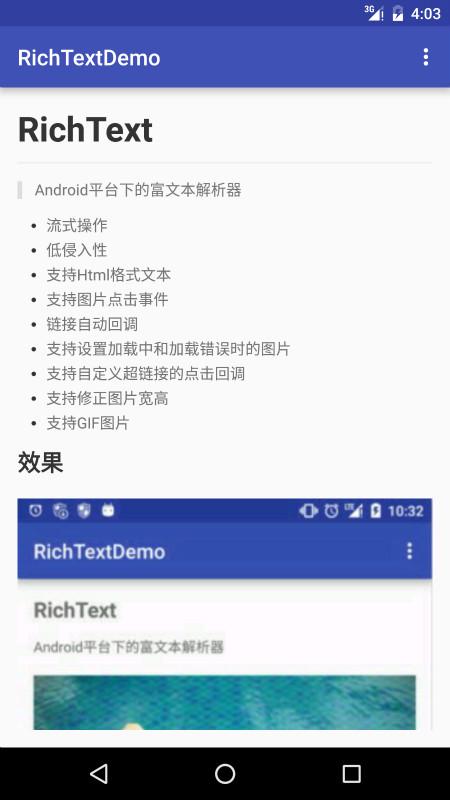
Use: method referenced in gradle:
implementation 'com.zzhoujay.richtext:richtext:3.0.7' //RichText资源库
Code used in:
Because it is rich text, there will be a lot of content, so a scroll bar has to be added (I won’t show it here)
<TextView
android:id="@+id/goods_details"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
Java code:
RichText.initCacheDir(GoodDetail.this); //设置缓存目录,不设置会报错
String goods_details_content = goodsList.getString("goods_details"); //后台获取到的数据资源
RichText.from(goods_details_content)
.bind(GoodDetail.this)
.showBorder(false)
.size(ImageHolder.MATCH_PARENT, ImageHolder.WRAP_CONTENT)
.into(goods_details);
@Override
protected void onDestroy() {
super.onDestroy();
//结束时清空内容
RichText.clear(GoodDetail.this);
}
2. Use: Markwon : https://github.com/noties/Markwon
A relatively new library, which has been updated:
Markwon is a markdown library for Android. It parses markdown following commonmark-spec with the help of amazing commonmark-java library and renders result as Android-native Spannables. No HTML is involved as an intermediate step. No WebView is required. It's extremely fast, feature-rich and extensible.
It gives ability to display markdown in all TextView widgets (TextView, Button, Switch, CheckBox, etc), Toasts and all other places that accept Spanned content. Library provides reasonable defaults to display style of a markdown content but also gives all the means to tweak the appearance if desired. All markdown features listed in commonmark-spec are supported (including support for inlined/block HTML code, markdown tables, images and syntax highlight).
Markwon comes with a sample application. It is a collection of library usages that comes with search and source code for each code sample.
Since version 4.2.0 Markwon comes with an editor to highlight markdown input as user types (for example in EditText).
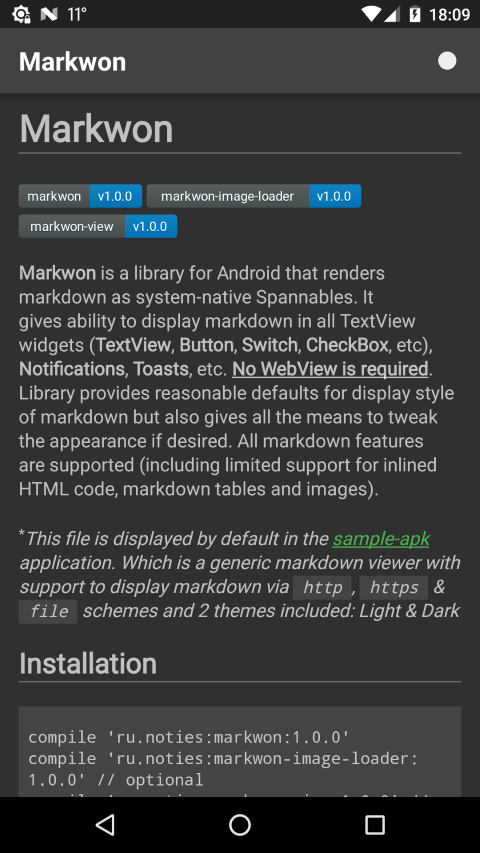
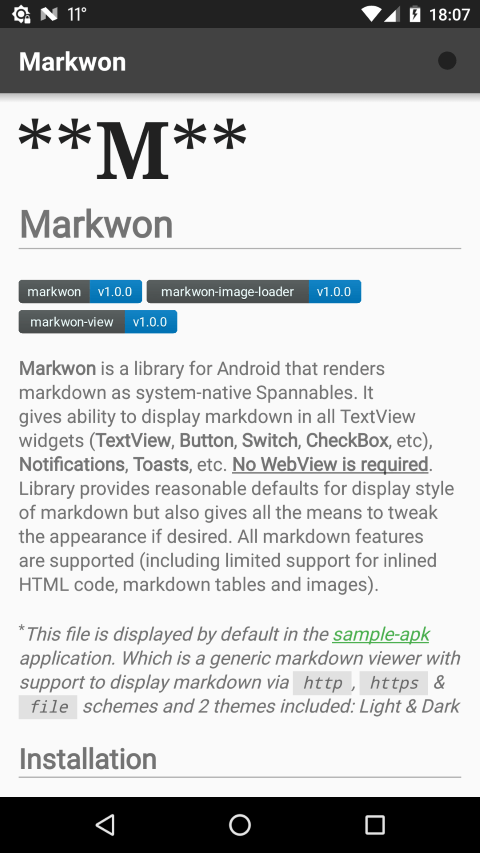
Use: import library:
implementation "io.noties.markwon:core:4.6.2"
implementation 'io.noties.markwon:image:4.6.2'
implementation 'io.noties.markwon:image-glide:4.6.2'
Android uses demo code kotlin writing method:
val content = "
\n" +
"\n" +
"1. 第一项:\n" +
" - 第一项嵌套的第一个元素\n" +
" - 第一项嵌套的第二个元素\n" +
"\n" +
"这是一个链接 [菜鸟教程](https://www.runoob.com)\n"
val markwon = Markwon.builder(this)
.usePlugin(GlideImagesPlugin.create(this))
.usePlugin(GlideImagesPlugin.create(Glide.with(this)))
.usePlugin(GlideImagesPlugin.create(object : GlideImagesPlugin.GlideStore {
override fun cancel(target: Target<*>) {
Glide.with(this@TestActivity).clear(target);
}
override fun load(drawable: AsyncDrawable): RequestBuilder<Drawable> {
return Glide.with(this@TestActivity).load(drawable.destination);
}
}))
.build()
markwon.setMarkdown(textView, content)
The above is a demo of displaying markdown rich text. If you want to display html, it is also possible, but you need to add the corresponding html dependency library.