1. What is Spring Security? Core functions?
Spring Security is a security framework based on the Spring framework, which provides a complete security solution, including authentication, authorization, attack protection and other functions.
Its core functions include:
Authentication: Provides a variety of authentication methods, such as form authentication, HTTP Basic authentication, OAuth2 authentication, etc., and can be integrated with various authentication mechanisms.
Authorization: Provides a variety of authorization methods, such as role authorization, expression-based authorization, etc., which can authorize different resources in the application.
Attack protection: Provides a variety of protection mechanisms, such as cross-site request forgery (CSRF) protection, injection attack protection, etc.
Session management: Provides session management mechanisms, such as token management, concurrency control, etc.
Monitoring and management: Provides monitoring and management mechanisms, such as access logging, auditing, etc.
Spring Security implements the above functions by configuring security rules and filter chains, and can easily provide security and protection mechanisms for Spring applications.
2. The principle of Spring Security?
Spring Security is a security authentication and authorization framework based on the Spring framework, which provides a comprehensive security solution that can protect all key parts of a web application.
The core principle of Spring Security is the interceptor (Filter). Spring Security will add a set of custom filters to the filter chain of the web application, which can implement authentication and authorization functions. When a user requests a resource, Spring Security intercepts the request and uses the configured authentication mechanism to authenticate the user. If authentication is successful, Spring Security authorizes the user to access the requested resource.
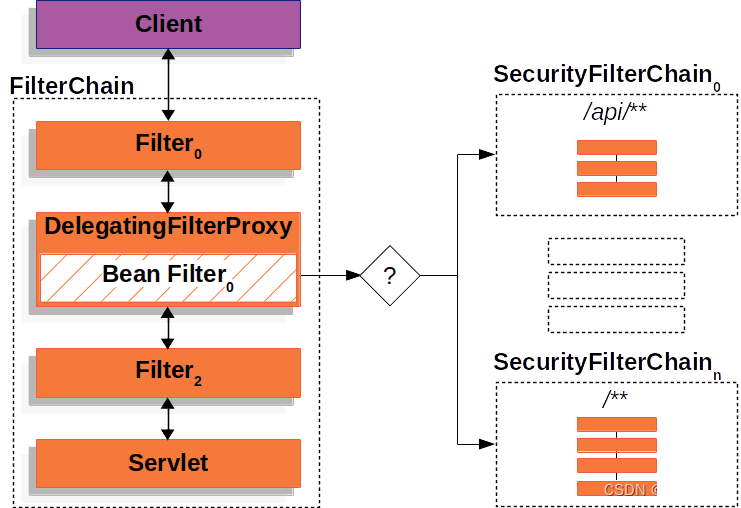
The specific working principle of Spring Security is as follows:
1. A user requests a protected resource of the web application.
2. Spring Security intercepts the request and tries to obtain the user's authentication information.
3. If the user is not authenticated, Spring Security will show the user a login page and ask the user to provide valid credentials (username and password).
4. Once the user provides valid credentials, Spring Security will verify these credentials and create an authenticated security context (SecurityContext) object.
5. The security context object contains authenticated user information, including user name, role and authorization information.
6. In the next request, Spring Security will use the authenticated security context object to determine whether the user has the right to access the protected resource.
7. If the user has permission to access the resource, Spring Security will allow the user to access the resource, otherwise an error message will be returned.
3. What are the ways to control the access permissions requested?
In Spring Security, the following methods can be used to control request access permissions:
permitAll() : Allows all users to access the request without any authentication.
denyAll() : Deny all users access to the request.
anonymous() : Allow anonymous users to access the request.
authenticated() : Requires the user to be authenticated, but does not require the user to have any particular role.
hasRole(String role) : Requires the user to have a specific role to access the request.
hasAnyRole(String...roles) : Requires the user to have at least one of several roles to access the request.
hasAuthority(String authority) : Requires the user to have a specific authority to access the request.
hasAnyAuthority(String... authorities) : Requires the user to have at least one of several authorities to access the request.
These methods can be applied to Spring Security configuration classes or used in Spring Security annotations.
4. Is there any difference between hasRole and hasAuthority?
In Spring Security, both hasRole and hasAuthority can be used to control user access, but they have some subtle differences.
The hasRole method is based on role access control. It checks if the user has the specified roles and those roles are prefixed with "ROLE_" (eg "ROLE_ADMIN").
The hasAuthority method performs access control based on permissions. It checks if the user has the specified permissions and those permissions are not prefixed.
Therefore, using the hasRole method requires prefixing the user's role name with "ROLE_", while using the hasAuthority method does not.
For example, assuming a user has a role of "ADMIN" and a permission of "VIEW_REPORTS", the user's access to pages can be controlled using:
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/reports/**").hasAuthority("VIEW_REPORTS")
In this example, only users with the "ROLE_ADMIN" role can access pages under the /admin/ path, while users with "VIEW_REPORTS" permissions can access pages under the /reports/ path.
5. How to encrypt the password?
Encrypting passwords in Spring Security usually uses a password encoder (PasswordEncoder). The role of PasswordEncoder is to encrypt plaintext passwords into ciphertext passwords for easy storage and verification. Spring Security provides a variety of common password encoders, such as BCryptPasswordEncoder, SCryptPasswordEncoder, StandardPasswordEncoder, etc.
Taking BCryptPasswordEncoder as an example, the steps are as follows:
1. Add the dependency of BCryptPasswordEncoder in the pom.xml file:
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-crypto</artifactId>
<version>5.6.1</version>
</dependency>
2. Inject BCryptPasswordEncoder in the Spring configuration file:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
// ...
}
3. Call the passwordEncoder.encode() method to encrypt the password where the password is used, such as encrypting the password when registering:
@Service
public class UserServiceImpl implements UserService {
@Autowired
private PasswordEncoder passwordEncoder;
@Override
public User register(User user) {
String encodedPassword = passwordEncoder.encode(user.getPassword());
user.setPassword(encodedPassword);
// ...
return user;
}
// ...
}
The above are the steps to use BCryptPasswordEncoder to encrypt the password. The steps for using other password encoders are similar, just replace BCryptPasswordEncoder with the corresponding password encoder.
6. Spring Security's authentication mode process based on username and password?
The requested username and password can be obtained from HttpServletRequest through form login, basic authentication, and digital authentication. The data source strategies used for authentication include memory, database, ldap, and custom.
The process of intercepting unauthorized requests and redirecting to the login page:
When a user accesses a resource that requires authorization, Spring Security checks whether the user is authenticated (that is, logged in), and redirects to the login page if not logged in.
When redirected to the login page, the user is required to enter a username and password for authentication.
Form login process:
The user enters the username and password on the login page and submits the form.
Spring Security's UsernamePasswordAuthenticationFilter intercepts the request submitted by the form and encapsulates the username and password into an Authentication object.
After the AuthenticationManager receives the Authentication object, it will query the user information according to the username and password, and encapsulate the user information into a UserDetails object.
If the user information is queried, the UserDetails object will be encapsulated into an authenticated Authentication object and returned. If the user information cannot be queried, a corresponding exception will be thrown.
After successful authentication, the user will be redirected to the previously accessed resource. If the previously accessed resources require specific roles or permissions to access, an authorization process is also required.
The authentication process of Spring Security can be roughly divided into two processes, the first is the process of user login authentication, and then the authorization process when users access protected resources. During the authentication process, the user needs to provide a user name and password. Spring Security encapsulates the user name and password into an Authentication object through the UsernamePasswordAuthenticationFilter and submits it to the AuthenticationManager for authentication. If the authentication is successful, the authentication result will be stored in the SecurityContextHolder. During the authorization process, Spring Security checks whether the user has permission to access the protected resource, and if not, redirects to the login page for authentication.
Intercept unauthorized requests and redirect to login page
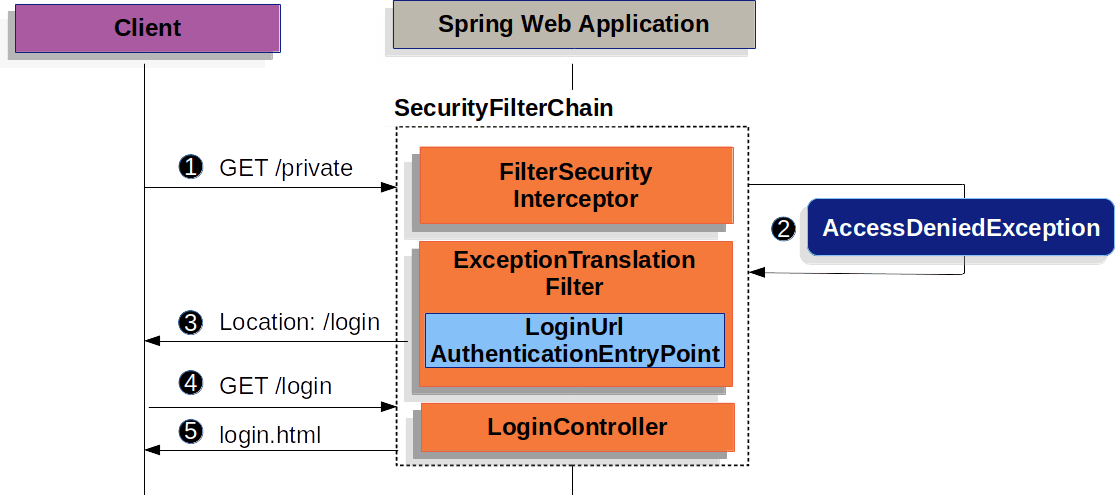
Form login process, account password authentication
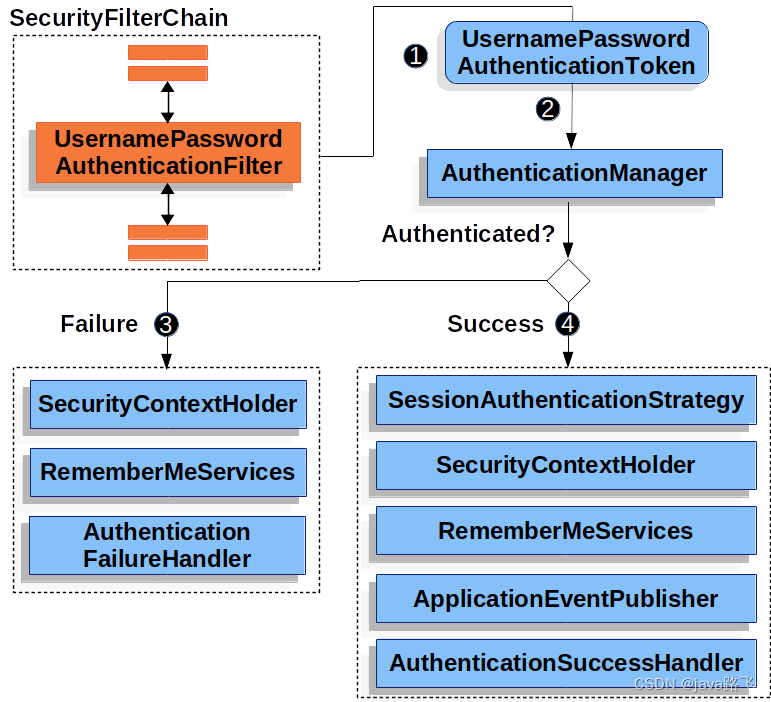