contour detection
cv2.findContours(image, mode, method)
-
mode: Contour retrieval mode
- RETR_EXTERNAL: Only retrieve outer contours
- RETR_LIST: Retrieve all contours and save them in a linked list
- RETR_CCOMP: Retrieve all contours and organize them into two layers, the top layer is the outer boundary of each part, and the second layer is the boundary of the cavity
- RETR_TREE: Retrieve all contours, and reconstruct the entire hierarchy of nested contours
-
method: contour approximation method
- CHAIN_APPROX_NONE: outputs contours as reeman chaincodes, all other methods output polygons (sequences of vertices)
- CHAIN_APPROX_SIMPLE: Compresses horizontal, vertical and oblique parts, i.e. the function keeps only their end parts
1. Read the image
import cv2
import matplotlib.pyplot as plt
image = cv2.imread('6.png')
# 灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
res = cv2.cvtColor(thresh, cv2.COLOR_BGR2RGB)
plt.imshow(res);
2. Find contour information
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_NONE)
3. Draw the outline
draw all contours
draw_img = image.copy()
# 传入绘制的底图, 绘制内容, 轮廓索引, 颜色, 厚度
res = cv2.drawContours(draw_img, contours, -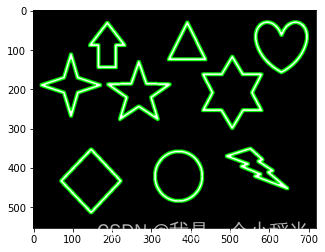
1, (0, 255, 0), 2)
# BGR转RGB
res = cv2.cvtColor(res, cv2.COLOR_BGR2RGB)
plt.imshow(res);
Draw an index (inner or outer contour of an image)
draw_img = image.copy()
# 传入绘制的底图, 绘制内容, 轮廓索引, 颜色, 厚度
res = cv2.drawContours(draw_img, contours, 1, (0, 255, 0), 2)
# BGR转RGB
res = cv2.cvtColor(res, cv2.COLOR_BGR2RGB)
plt.imshow(res);
draw_img = image.copy()
# 传入绘制的底图, 绘制内容, 轮廓索引, 颜色, 厚度
res = cv2.drawContours(draw_img, contours, 0, (0, 255, 0), 2)
# BGR转RGB
res = cv2.cvtColor(res, cv2.COLOR_BGR2RGB)
plt.imshow(res);