- 1. Introduction to TypeScript
- 2. Summary of TypeScript syntax
-
- 1. TypeScript—variable type declaration
- 2. TypeScript—basic data types
- 3. TypeScript—Array Type
- 4. TypeScript—Union Types
- 5. TypeScript—Map object
- 6. TypeScript—Tuples
- 7. TypeScript—Interface
- 8. TypeScript—Classes
- 9. TypeScript—Functions
- 10. TypeScript—Type Inference and Type Assertions
- 11. TypeScript — Enum
- 12. TypeScript — Generics
- 13. TypeScript—string literal type
- 14. TypeScript—namespace
- 15. TypeScript—type declaration file
This article is my summary of TS. Although TypeScript is similar to JavaScript syntax, there are still great differences in the details of their use. The purpose of writing this article is to facilitate your own reference and review in the future. It also applies to people who want to switch from JS to TS and some people who have zero TS foundation. It is quite long, so it is recommended to use a PC to read and consult. If this blog is helpful to you, thank you for a like. If not, then just for me, just write it for yourself.
1. Introduction to TypeScript
Although some people may think that this part of the introduction is unnecessary, I think it is still necessary to write briefly. Since we want to use it frequently in the future, of course we have to start by getting to know it! Scroll down if you don't want to see it.
Summary :
TypeScript is 微软
a free and open-source programming language developed by. TypeScript is a superset of JavaScript, supports the ECMAScript 6 standard, extends the syntax of JavaScript, and solves some shortcomings of JS. So existing JavaScript code can work with TypeScript without any modification, and TypeScript provides compile-time static type checking through type annotations. The design goal of TypeScript is to develop large-scale applications, which can be compiled into pure JavaScript, and the compiled JavaScript can run on any browser. In other words, the writing is written in TS, but the final compilation is still JS. But it should be noted that TS is not intended to replace JS, it is built on top of JS.
TypeScript added features :
type annotations and compile-time type checking, type inference, interfaces, enumerations, Mixin, generic programming, tuples, Await, classes, modules, arrow syntax for lambda functions, optional parameters, and default parameters.
Learning environment setup :
1. Download the latest version of Node.js and install it
2. Open cmd and use npm to install typescript
npm install -g typescript
After TypeScript is installed globally, we can use the tsc command anywhere, and the tsc command is responsible for compiling TypeScript files into JavaScript files.
TypeScript usage example:
The first way is to compile the TypeScript code through the global tsc command
and create a directory:
mkdir ts-practice
cd ts-practice
We create a test.ts file and write a piece of code inside:
export enum TokenType {
ACCESS = 'accessToken',
REFRESH = 'refreshToken'
}
Run the tsc command test:
tsc test.ts
After running, you will get a compiled file of test.js.
Another way to use it is the engineering compilation scheme , which involves more configuration and attention points, which will be explained in detail in the next blog.
2. Summary of TypeScript syntax
The following is a summary of the basic grammar, which involves grammar such as variable declaration, basic type, object type, tuple, enumeration, interface, class, function, generic, literal type, type assertion, type protection, etc.
1. TypeScript—variable type declaration
TypeScript is a superset of JavaScript. Like JavaScript, variables can be declared using var
, let
, and const
three keywords.
Naming rules for TypeScript variables: Variable names can contain numbers and letters. Except for 下划线 _
and 美元 $ 符号
, no other special characters, including spaces, are allowed. Variable names cannot start with a number.
Variable type declaration is a very important feature of ts, through which the data type of the variable in the current ts can be specified. After specifying the type, when assigning a value to the variable, the TS compiler will automatically check whether it conforms to the type declaration, assign the value if it conforms, and report an error if it does not conform. In short, the type declaration sets the type for the variable so that the variable can only store a certain type of value.
The syntax of a type declaration:
//1.声明变量的类型,但没有初始值,变量值会设置为 undefined:
let 变量名 : 类型 ;
//2.声明变量的类型及初始值:
let 变量名 : 类型 = 值 ;
//函数参数类型和返回值类型声明
function(参数名1 : 类型 ,参数名2 : 类型):返回值类型{
···
}
2. TypeScript—basic data types
There are two types of JavaScript: 基础数据类型
and对象类型
(1) Boolean value type
Boolean value is the most basic data type. In TypeScript, use to boolean
define the Boolean value type.
let isDone: boolean = false;
Note that 构造函数 Boolean
the object created by using is not a Boolean value, and the compilation will report an error :
let isMale: boolean = new Boolean(1);
In fact, new Boolean() returns one Boolean 对象
. We need to change the boolean type declaration to Boolean type declaration so that no error will be reported:
let isMale: Boolean = new Boolean(1);
Or call Boolean()
the function to return a boolean type, so that no error will be reported when writing:
let isMale: boolean = Boolean(1);
(2) Numerical types
Use to number
define numeric types, which can be used to represent integers and fractions.
let binaryNumber: number = 0b1010; // 二进制
let octalNumber: number = 0o744; // 八进制
let decNumber: number = 6; // 十进制
let hexNumber: number = 0xf00d; // 十六进制
(3) String type
Use to string
define a string type, a series of characters, use single quotes ' or double quotes " to represent the string type. Backticks ` to define multi-line text and embedded expressions.
let myName: string = '害恶细君';
let myAge: number = 20;
// 模板字符串,这其实也是ES6的语法
let sentence: string = `Hello, my name is ${
myName}.
I'll be ${
myAge + 1} years old next month.`;
Compilation result:
let myName = '害恶细君';
let myAge = 20;
// 模板字符串
let sentence = "Hello, my name is " + myName + ".\nI'll be " + (myAge + 1) + " years old next month.";
(4) Void type
JavaScript does not have the concept of void (Void). In TypeScript, you can use to void
represent a function without any return value. Can be used to identify the type of method return value, indicating that the method has no return value.
function alertName(): void {
alert('My name is haiexijun');
}
Declaring a variable of type void is useless because you can only assign it to undefined and null.
let unusable: void = undefined;
(5) Null and Undefined types
In TypeScript, you can use null
and undefined
to define these two primitive data types. Null means that the object value is missing, and undefined
is used to initialize the variable to an undefined value.
let u: undefined = undefined;
let n: null = null;
The difference from void is that undefined and null are subtypes of all types. That is to say, variables of type undefined can be assigned to variables of type number:
// 这样不会报错
let num: number = undefined;
// 这样也不会报错
let u: undefined;
let num: number = u;
A variable of type void cannot be assigned to a variable of type number, and an error will be reported when compiling the following:
let u: void;
let num: number = u;
(6) Any value type
Any value (Any) is used to indicate that any type is allowed to be assigned, and any
is used to represent any type. A variable declared as any can be assigned a value of any type.
If it is an ordinary type, it is not allowed to change the type during assignment, and the compilation will report an error:
let myFavoriteNumber: string = 'seven';
myFavoriteNumber = 7;
But if it is any type, it is allowed to be assigned any type.
let myFavoriteNumber: any = 'seven';
myFavoriteNumber = 7;
After declaring a variable as an arbitrary value, any operation on it will return an arbitrary value.
If a variable is declared without specifying its type, it will be recognized as any value type:
let something;
something = 'seven';
something = 7;
//等价于
let something: any;
something = 'seven';
something = 7;
3. TypeScript—Array Type
In TypeScript, array types can be defined in many ways, which is more flexible.
The easiest way is to use 类型 []
to represent arrays:
let fibonacci: number[] = [1, 1, 2, 3, 5];
Array items are not allowed to appear in other types, otherwise the compilation will report an error:
let fibonacci: number[] = [1, '1', 2, 3, 5];
The parameters of some methods of the array will also be restricted according to the type agreed upon when the array is defined:
let fibonacci: number[] = [1, 1, 2, 3, 5];
fibonacci.push('8');
In the above example, the push method only allows parameters of type number to be passed in, but a parameter of type "8" is passed, so the compilation will also report an error.
Representing arrays with array generics
We can also use array genericsArray<elemType>
to represent arrays:
let nums: Array<number> = [1, 1, 2, 3, 5];
About generics, you can explain in detail below. The main purpose here is to introduce that array generics can define arrays.
Using interfaces to represent arrays
Interfaces can also be used to describe arrays (interfaces will be explained in detail later):
interface NumberArray {
[index: number]: number;
}
let fibonacci: NumberArray = [1, 1, 2, 3, 5];
The above NumberArray means: as long as the type of the index is a number, then the type of the value must be a number. Although interfaces can also be used to describe arrays, we generally don't do this, because this method is much more complicated than the first two methods, just a little understanding.
Array-like
Array-like Object (Array-like Object) is not an array type, such asarguments
:
function sum() {
let args: number[] = arguments;
}
In the above example, arguments
it is actually a class array, which cannot be described by an ordinary array, but should be described by an interface:
function sum() {
let args: {
[index: number]: number;
length: number;
callee: Function;
} = arguments;
}
In this example, we not only constrain that when the type of the index is a number, the type of the value must be a number, but also constrain it to have two attributes of length and callee.
In fact, commonly used class arrays have their own interface definitions, such as IArguments
, NodeList
, HTMLCollection
and so on:
function sum() {
let args: IArguments = arguments;
}
Among them IArguments
is the type defined in TypeScript, which is actually:
interface IArguments {
[index: number]: any;
length: number;
callee: Function;
}
The application of any in an array
A more common practice is to use any to indicate that any type is allowed in the array:
let list: any[] = ['xcatliu', 25, {
website: 'http://www.baidu.com' }];
Creating an Array from an Array Object
We can alsonew Array()
create an array using an Array object. The constructor for an Array object accepts two values: A numeric value representing the size of the array. Initialized array list with elements separated by commas.
//指定数组初始化大小:
let arr_names:number[] = new Array(4)
arr_names[1]=12
//或者直接初始化时声明数组元素:
let sites:string[] = new Array("Google","csdn","haiexijun","Facebook")
Array Destructuring
We can also assign array elements to variables as follows:
let arr:number[] = [12,13]
let [x,y] = arr // 将数组的两个元素赋值给变量 x 和 y
console.log(x)
console.log(y)
Array iteration
We can use the for in statement to loop through the elements of an array:
let j:any;
let nums:number[] = [1001,1002,1003,1004]
//迭代打印
for(j in nums) {
console.log(nums[j])
}
Multidimensional arrays
The elements of one array can be another array, thus forming a multidimensional array. The simplest multidimensional array is a two-dimensional array, defined as follows:
let multiArr:number[][] = [[1,2,3],[23,24,25]]
console.log(multi[0][1])
//输出2
Using arrays in functions
Arrays can be passed as parameters to functions:
let sites:string[] = new Array("Google","CSDN","Taobao","haiexijun")
function alertSite(arr_sites:string[]) {
for(let i = 0;i<arr_sites.length;i++) {
console.log(arr_sites[i])
}
}
alertSite(sites);
//输出结果为
Google
CSDN
Taobao
haiexijun
Arrays can also be used as return values of functions:
function alertName():string[] {
return new Array("Google", "CSDN", "Taobao", "haiexijun");
}
let sites:string[] = alertName()
for(let i in sites) {
console.log(sites[i])
}
//输出结果为
Google
CSDN
Taobao
haiexijun
Summary of common array methods
method name | effect |
---|---|
concat() | Concatenates two or more arrays and returns the result. |
every() | Checks whether each element of the numeric element meets the condition. |
filter() | Detects numeric elements and returns an array of all elements matching the criteria. |
forEach() | The callback function is executed once for each element of the array. |
indexOf() | Searches for an element in an array and returns its position. If the search cannot be found, the return value is -1, which means there is no item. |
join() | Put all the elements of the array into a string. |
lastIndexOf() | Returns the position of the last occurrence of a specified string value, searching backwards and forwards at the specified position in a string. |
map() | Processes each element of the array with the specified function and returns the processed array. |
pop() | Removes the last element of the array and returns the removed element. |
push() | Adds one or more elements to the end of the array and returns the new length. |
reduce() | Computes an array element as a value (left to right). |
reduceRight() | Computes an array element as a value (right to left). |
reverse() | Reverses the order of the elements of an array. |
shift() | Remove and return the first element of the array. |
slice() | Select a portion of an array and return a new array. |
some() | Checks whether any element in the array element meets the specified condition. |
sort() | Sorts the elements of an array. |
splice() | Add or remove elements from an array. |
toString() | Convert an array to a string and return the result. |
unshift() | Adds one or more elements to the beginning of the array and returns the new length. |
The specific usage of these array methods is not clear, you can go to Baidu again, here is no code example, after all, those things are the content of js.
4. TypeScript—Union Types
Union types (Union Types) indicate that the value can be one of many types. The syntax is:类型1|类型2|类型3
let myFavoriteNumber: string | number;
myFavoriteNumber = 'seven';
myFavoriteNumber = 7;
myFavoriteNumber = true; //这里报错
The meaning of let myFavoriteNumber: string | number here is that the type of myFavoriteNumber is allowed to be string or number, but not other types.
When a variable of a union type is assigned a value, a type will be inferred according to the rules of type inference:
let myFavoriteNumber: string | number;
myFavoriteNumber = 'seven';
console.log(myFavoriteNumber.length); // 5
myFavoriteNumber = 7;
console.log(myFavoriteNumber.length); // 编译时报错 number类型没有length属性
In the above example, myFavoriteNumber in the second line is inferred to be a string, and no error will be reported when accessing its length property.
The myFavoriteNumber in the fourth line is inferred to be a number, and an error is reported when accessing its length property.
5. TypeScript—Map object
Map objects hold key-value pairs and are able to remember the original insertion order of the keys. Any value (object or primitive) can be used as a key or a value. Map is a new data structure introduced in ES6, you can refer to ES6 Map and Set.
Create a Map object and operate on it
TypeScript uses the Map type and the new keyword to create a Map:
//创建 Map对象
let myMap = new Map();
// 设置 Map 对象用set(key,value)方法
myMap.set("Google", 1);
myMap.set("CSDN", 2);
myMap.set("Taobao", 3);
// 获取键对应的值用get()方法
console.log(myMap.get(" CSDN")); //2
// 判断 Map 中是否包含键对应的值用has()方法
console.log(myMap.has("Taobao")); // true
console.log(myMap.has("Zhihu")); // false
// 返回 Map 对象键/值对的数量
console.log(nameSiteMapping.size); // 3
// 删除 CSDN
console.log(myMap.delete("CSDN")); // true
console.log(myMap);
// 移除 Map 对象的所有键/值对
myMap.clear();
console.log(myMap);
// 迭代 Map 中的 key
for (let key of myMap.keys()) {
console.log(key);
}
// 迭代 Map 中的 value
for (let value of myMap.values()) {
console.log(value);
}
// 迭代 Map 中的 key => value
for (let entry of myMap.entries()) {
console.log(entry[0], entry[1]);
}
6. TypeScript—Tuples
We know that the data types of the elements in the array are generally the same (arrays of any[] type can be different), if the data types of the stored elements are different, you need to use tuples.
Different types of elements are allowed to be stored in tuples, and tuples can be passed as parameters to functions.
Usage example: define a pair of tuples whose values are string and number:
//定义了一个有字符串和数字的的元组,下面是正确写法:
let tom: [string, number] = ['Tom', 25];
console.log(tom[1])
//当当定义了存2个值,却是3个值时就会编译报错,如下:
let tom: [string, number] = ['Tom', 25, 123];
console.log(tom[1])
//内容的类型和定义的顺序不一样,也会编译报错,如下
let tom: [string, number] = [ 25,'Tom'];
console.log(tom[1])
Tuple Operations
We can add new elements to or remove elements from a tuple using the following two functions:
push()
add an element to a tuple, and add at the end.
pop()
Removes an element (the last one) from the tuple and returns the removed element.
Destructuring tuples
We can also assign tuple elements to variables as follows:
let a =[10,"haiexijun"]
let [b,c] = a
console.log( b )
console.log( c )
7. TypeScript—Interface
An interface is a declaration of a series of abstract methods. It is a collection of method features. These methods should be abstract and need to be implemented by specific classes. Then third parties can use this group of abstract method calls to let specific classes execute specific methods. Methods. In object-oriented language, interface (Interfaces) is a very important concept. It is an abstraction of behavior, and how to act specifically needs to be implemented by classes (classes). Interfaces are generally capitalized. Some programming languages suggest that interface names be prefixed with I.
The TypeScript interface is defined as follows:
interface interface_name {
}
In the following example, we define an interface IPerson, and then define a variable customer whose type is IPerson.
customer implements the properties and methods of the interface IPerson.
interface IPerson {
firstName:string,
lastName:string,
sayHi: ()=>string
}
let customer:IPerson = {
firstName:"zhang",
lastName:"chao",
sayHi: ():string =>{
return "Hi there"}
}
console.log("Customer 对象 ")
console.log(customer.firstName)
console.log(customer.lastName)
console.log(customer.sayHi())
Union types and interfaces
interface RunOptions {
program:string;
commandline:string[]|string;
}
Interface and array
In the interface, we can set the index value and element of the array to different types, and the index value can be a number or a string.
Set the element to a string type, if other types are used, an error will be reported:
interface namelist {
[index:number]:string
}
// 类型一致,正确
let list2:namelist = ["Google","CSDN","Taobao"]
//类型不一致,错误。编译会报错
let list2:namelist = ["haiexijun",1,"Taobao"]
Interface inheritance
Interface inheritance means that an interface can extend itself through other interfaces. Typescript allows interfaces to inherit from multiple interfaces. Inheritance uses the keyword extends .
An example of a single inheritance interface:
interface Person {
name: string;
age: number;
}
let tom: Person = {
name: 'Tom',
age: 25
};
Example of multiple inheritance:
interface IParent1 {
v1:number
}
interface IParent2 {
v2:number
}
interface Child extends IParent1, IParent2 {
}
Optional properties of the interface
interface Person {
name: string;
age?: number;
}
//可以不给age属性赋值
let tom: Person = {
name: 'Tom'
};
Arbitrary attributes of interfaces
Sometimes we want an interface to allow arbitrary attributes, we can use the following methods:
interface Person {
name: string;
age?: number;
[propName: string]: any;
}
let tom: Person = {
name: 'Tom',
gender: 'male'
};
Use [propName: string] to define any property to take the value of string type.
It should be noted that once any attribute is defined, the type of both the definite attribute and the optional attribute must be a subset of its type
Read-only properties of interfaces
Sometimes we want some fields in an object to be assigned only when they are created, so we can use to readonly
define
interface Person {
readonly id: number;
name: string;
age?: number;
[propName: string]: any;
}
8. TypeScript—Classes
TypeScript is object-oriented JavaScript. A class describes the properties and methods common to the objects created. TypeScript supports all object-oriented features, such as classes, interfaces, etc.
ES6 syntax also has class
the concept of classes.
Usage of classes in ES6
Properties and methods
Use class
to defineconstructor
to define a constructor. When a new instance is generated by new, the constructor will be called automatically.
class Animal {
public name;
constructor(name) {
this.name = name;
}
sayHi() {
return `My name is ${
this.name}`;
}
}
let a = new Animal('害恶细君');
console.log(a.sayHi()); // My name is 害恶细君
Class inheritance
uses extends
the keywordsuper
the keyword to call the constructor and method of the parent class.
class Cat extends Animal {
constructor(name) {
super(name); // 调用父类的 constructor(name)
console.log(this.name);
}
sayHi() {
return 'csdn, ' + super.sayHi(); // 调用父类的 sayHi()
}
}
let c = new Cat('haiexijun'); // haiexijun
console.log(c.sayHi()); // csdn, My name is haiexijun
Accessors
Use getters and setters to change the assignment and read behavior of properties:
class Animal {
constructor(name) {
this.name = name;
}
get name() {
return 'Jack';
}
set name(value) {
console.log('setter: ' + value);
}
}
let a = new Animal('Kitty'); // setter: Kitty
a.name = 'Tom'; // setter: Tom
console.log(a.name); // Jack
Static methods
Methods decorated with static
the modifier
class Animal {
static isAnimal(a) {
return a instanceof Animal;
}
}
Static properties
You can use static
to define
class Animal {
static num = 42;
constructor() {
// ...
}
}
console.log(Animal.num); // 42
Usage of classes in TypeScript
TypeScript can use three access modifiers, which arepublic
, ,private
andprotected
.
Modifier | effect |
---|---|
public | The modified properties or methods are public and can be accessed anywhere. By default, all properties and methods are public |
private | The decorated property or method is private and cannot be accessed outside the class in which it is declared |
protected | The modified property or method is protected, it is similar to private, the difference is that it is also allowed to be accessed in subclasses |
class Animal {
public name;
public constructor(name) {
this.name = name;
}
}
let a = new Animal('Jack');
console.log(a.name); // Jack
a.name = 'Tom';
console.log(a.name); // Tom
In the example above, name is set to public, so direct access to the instance's name attribute is allowed.
Many times, we hope that some attributes cannot be accessed directly. At this time, private can be used:
class Animal {
private name;
public constructor(name) {
this.name = name;
}
}
let a = new Animal('Jack');
console.log(a.name);//编译报错
a.name = 'Tom';//编译报错
Attributes or methods modified with private are not allowed to be accessed in subclasses, so I won't demonstrate them here. If it is modified with protected, access is allowed in subclasses. When the constructor is modified as private, the class is not allowed to be inherited or instantiated. When the constructor is modified as protected, the class is only allowed to be inherited.
class type
class Animal {
name: string;
constructor(name: string) {
this.name = name;
}
sayHi(): string {
return `My name is ${
this.name}`;
}
}
let a: Animal = new Animal('Jack');
console.log(a.sayHi()); // My name is Jack
9. TypeScript—Functions
used tofunction
define functions
function sum(x, y) {
return x + y;
}
Function overloading
Overloading means that the method name is the same, but the parameters are different, and the return type can be the same or different.
Each overloaded method (or constructor) must have a unique list of parameter types.
//参数类型不同:
function disp(string):void;
function disp(number):void;
//参数数量不同:
function disp(n1:number):void;
function disp(x:number,y:number):void;
//参数类型顺序不同:
function disp(n1:number,s1:string):void;
function disp(s:string,n:number):void;
Then, it seems that there is nothing to add, and most of the others are not commonly used, so I won’t write them here. Just remember that any syntax that JS can use, TS can use.
10. TypeScript—Type Inference and Type Assertions
If no type is explicitly specified, TypeScript will infer a type according to the rules of type inference.
Type inference
Although the following code does not specify a type, it will report an error when compiling:
let myFavoriteNumber = 'seven';
myFavoriteNumber = 7;
//事实上等价于
let myFavoriteNumber: string = 'seven';
myFavoriteNumber = 7;
TypeScript will infer a type when there is no explicit specified type, which is type inference.
If there is no assignment at the time of definition, no matter whether there is an assignment afterwards, it will be inferred as any type and will not be type checked at all:
//下面就不会报错
let myFavoriteNumber;
myFavoriteNumber = 'seven';
myFavoriteNumber = 7;
Type Assertions
Type assertions can be used to manually specify the type of a value.
The syntax is:值 as 类型
or <类型>值
It is recommended that值 as 类型
this syntax uniformly when using type assertions. Because <类型>值
this
When TypeScript is not sure which type a variable of a joint type is, we can only access properties or methods common to all types of this joint type:
interface Cat {
name: string;
run(): void;
}
interface Fish {
name: string;
swim(): void;
}
function getName(animal: Cat | Fish) {
//只能访问name
return animal.name;
}
//而有时候,我们确实需要在还不确定类型的时候就访问其中一个类型特有的属性或方法
function isFish(animal: Cat | Fish) {
//获取 animal.swim 的时候会报错。
if (typeof animal.swim === 'function') {
return true;
}
return false;
}
//此时可以使用类型断言,将 animal 断言成 Fish,这样就可以解决访问 animal.swim 时报错的问题了。
function isFish(animal: Cat | Fish) {
if (typeof (animal as Fish).swim === 'function') {
return true;
}
return false;
}
11. TypeScript — Enum
The enumeration (Enum) type is used in scenarios where the value is limited to a certain range, for example, there are only seven days in a week, and the colors are limited to red, green, and blue.
Enumerations are defined using enum
the keyword :
enum Days {
Sun, Mon, Tue, Wed, Thu, Fri, Sat};
console.log(Days["Sun"]); // 0
console.log(Days[0]); // Sun
We can also manually assign values to the enumeration items , and the enumeration items that are not manually assigned will be incremented after the previous enumeration item:
enum Days {
Sun = 7, Mon = 1, Tue, Wed, Thu, Fri, Sat};
console.log(Days["Sun"]); // 7
console.log(Days["Mon"]); // 1
console.log(Days["Tue"]); // 2
console.log(Days["Sat"]); // 6
Of course, manually assigned enumeration items can also be decimals or negative numbers. In this case, the incremental step of subsequent items that are not manually assigned is still 1:
enum Days {
Sun = -7, Mon = 1.5, Tue, Wed, Thu, Fri, Sat};
console.log(Days["Tue"]); // 2
console.log(Days["Mon"]); // 1.5
I won't write other details, and I will talk about it when I use it.
12. TypeScript — Generics
Generic refers to a feature that does not pre-specify a specific type when defining a function, interface, or class, but specifies the type when it is used.
//首先,我们来实现一个函数 createArray,它可以创建一个指定长度的数组,同时将每一项都填充一个默认值:
function createArray(length: number, value: any): Array<any> {
let result = [];
for (let i = 0; i < length; i++) {
result[i] = value;
}
return result;
}
createArray(3, 'x'); // ['x', 'x', 'x']
In the above example, we used the array generic type mentioned earlier to define the type of the return value.
This code compiles without error, but an obvious flaw is that it does not accurately define the type of the return value. Array allows each item of the array to be of any type. But what we expect is that each item in the array should be the type of the input value. This is where generics come in handy:
function createArray<T>(length: number, value: T): Array<T> {
let result: T[] = [];
for (let i = 0; i < length; i++) {
result[i] = value;
}
return result;
}
createArray<string>(3, 'x'); // ['x', 'x', 'x']
Generics with multiple type parameters:
//定义了一个 swap 函数,用来交换输入的元组。
function swap<T, U>(tuple: [T, U]): [U, T] {
return [tuple[1], tuple[0]];
}
swap([7, 'seven']); // ['seven', 7]
13. TypeScript—string literal type
The string literal type is used to constrain the value to be only one of several strings. The string literal type is defined type
using , here is a simple example:
//使用 type 定了一个字符串字面量类型 EventNames,它只能取三种字符串中的一种。
type EventNames = 'click' | 'scroll' | 'mousemove';
function handleEvent(ele: Element, event: EventNames) {
console.log(EventNames)
}
// 没问题
handleEvent('scroll');
// 报错,event 不能为 'focus'
handleEvent('focus');
14. TypeScript—namespace
Namespaces are used to avoid variable naming conflicts, and TypeScript officially regards namespaces as "internal modules".
If you declare namespaces with the same name, the TypeScript compiler will combine them into one declaration.
Use namespace
the keyword to declare a namespace. The namespace of TypeScript can wrap the code, only expose the namespace object, and mount the variables in the namespace to the namespace object through export
keywords . A namespace is essentially an object, and its internal variables are organized into the properties of this object:
namespace Calculator {
const fn = (x: number, y: number) => x * y
export const add = (x: number, y:number) => x + y
}
Its compiled result:
"use strict";
var Calculator;
(function (Calculator) {
var fn = function (x, y) {
return x * y; };
Calculator.add = function (x, y) {
return x + y; };
})(Calculator || (Calculator = {
}));
Then, we can access the add property on the Calculator object:
Calculator.add(2, 3)
15. TypeScript—type declaration file
If we want to use the third-party library jQuery, a common way is to introduce jQuery through the <script> tag in HTML, and then use the global variable $ or jQuery. But in ts, the compiler doesn't know what $ or jQuery is.
Declaration file
When using a third-party library, we need to reference its declaration file in order to obtain the corresponding code completion, interface prompt and other functions. Usually we will put the declaration statement into a separate file (such as jQuery.d.ts), this is the declaration file, and the declaration file must .d.ts
be suffixed.
Generally speaking, ts will parse all *.ts files in the project, and of course it also includes files ending in .d.ts. So when we put jQuery.d.ts into the project, all other *.ts files can get the jQuery type definition.
Third-party declaration file
Of course, jQuery declaration file does not need us to define, JQuery has already defined it for us.
We can download and use it directly, but it is more recommended to use @types to uniformly manage the declaration files of third-party libraries.
The use of @types is very simple, just use npm to install the corresponding declaration module, take jQuery as an example:
npm install @types/jquery --save-dev
If you want to search for the declaration file you need, you can click this link: https://www.typescriptlang.org query.
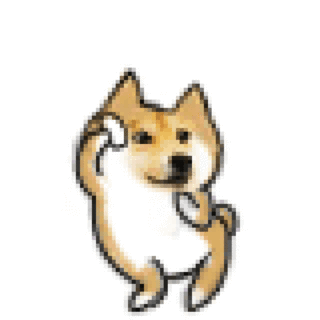
Mastering these grammars of TypeScript is like learning the art of building walls, but our purpose of learning TypeScript is not to build a "small hut", but to build "high-rise buildings", which is exactly what TypeScript's type system brings The advantages. In the next blog, I will learn and summarize the best practices of TypeScript engineering in detail.