Requirement: Reversely arrange the characters in the parameter string instead of printing them in reverse order.
1. Ideas
1. Think about how to change the string abcdef into fedcba?
eg.
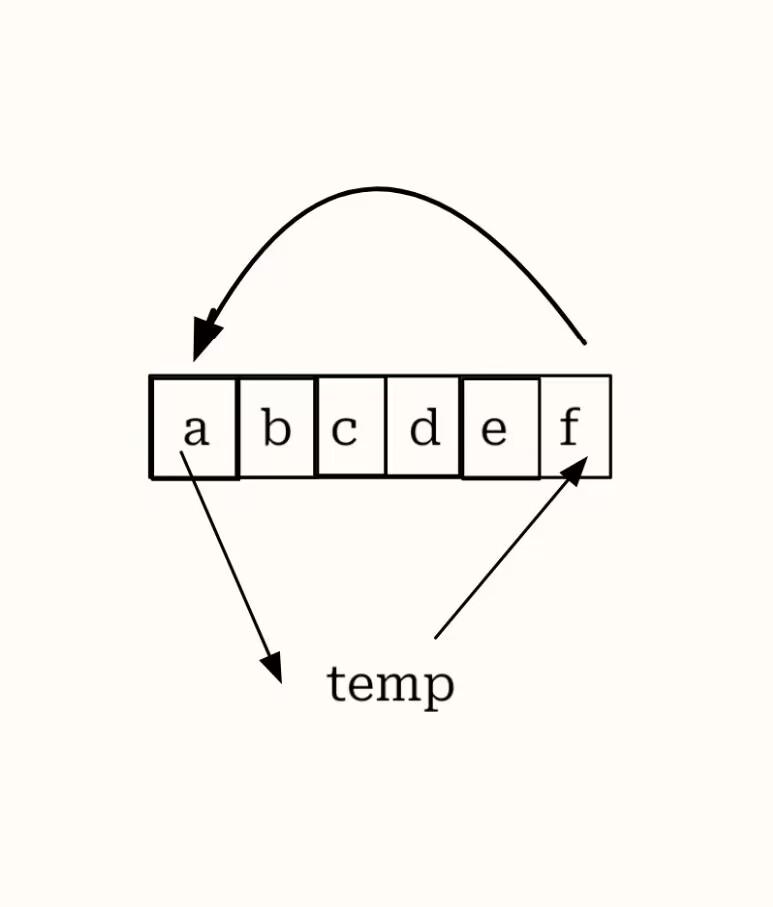
Use temp to exchange af
Then the code is as follows
#include<stdio.h>
#include<string.h>
void reverse_string(char* str)
{
int len = strlen(str);
char temp = *str;
*str = *(str + len - 1);
*(str + len - 1) = temp;
reverse_string(str + 1);
}
int main()
{
char arr[] = "abcdef";
reverse_string(arr);//设计了一个叫做reverse_string的函数用来实现字符串逆序
printf("%s\n", arr);
return 0;
}
When you exchange a and f, '\0' is behind f.
However, when you exchange b and e, '\0' is still behind f, which will cause b and a (the original position of f) to be exchanged.
The process is first green and then blue .
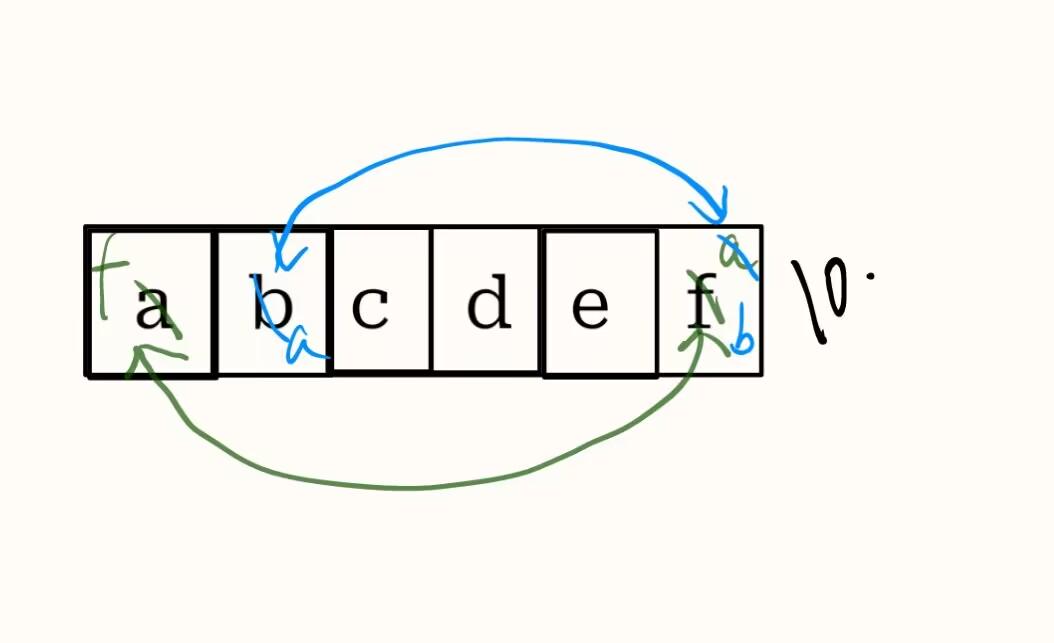
2. Think, what should I do then? -- advance '\0'
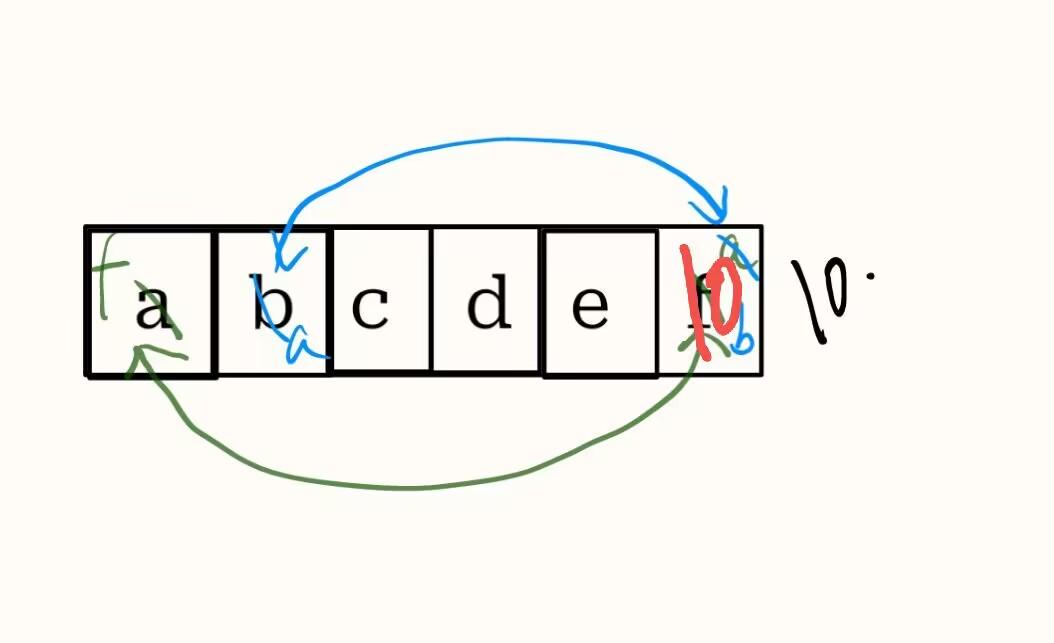
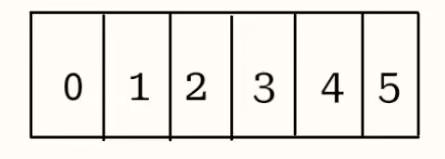
But if a has been placed in position 5, and you advance '\0', then a will disappear.
So you should mention '\0' first, and then move a.
That is, the code is as follows:
#include<stdio.h>
#include<string.h>
void reverse_string(char* str)
{
int len = strlen(str);
char temp = *str;
*str = *(str + len - 1);
*(str + len - 1) = '\0';
reverse_string(str + 1);
*(str + len - 1) = temp;
}
int main()
{
char arr[] = "abcdef";
reverse_string(arr);
printf("%s\n", arr);
return 0;
}
It is found that the above code will cause dead recursion,
3. Thinking, how to change, that is, how to limit?
It is necessary to reverse the order if it is greater than or equal to two characters
Two, the code
#include<stdio.h>
#include<string.h>
void reverse_string(char* str)
{
int len = strlen(str);
char temp = *str;
*str = *(str + len - 1);
*(str + len - 1) = '\0';
if(strlen(str+1)>=2)
reverse_string(str + 1);
*(str + len - 1) = temp;
}
int main()
{
char arr[] = "abcdef";
reverse_string(arr);
printf("%s\n", arr);
return 0;
}