1、vue-element-admin
Vue-element-admin is a background management system integration solution based on element-ui.
Function : https://panjiachen.github.io/vue-element-admin-site/zh/guide/#function
GitHub address: https://github.com/PanJiaChen/vue-element-admin
Project online preview: https://panjiachen.gitee.io/vue-element-admin
2、vue-admin-template
2.1. Introduction
vue-admin-template is a set of background management system basic templates (minimum simplified version) based on vue-element-admin, which can be used as templates for secondary development.
GitHub address: https://github.com/PanJiaChen/vue-admin-template
Suggestion: You can carry out secondary development on the basis of vue-admin-template, use vue-element-admin as a toolbox, and copy any functions or components you want from vue-element-admin.
2.2. Directory structure
|-dist 生产环境打包生成的打包项目
|-mock 使用mockjs来mock接口
|-public 包含会被自动打包到项目根路径的文件夹
|-index.html 唯一的页面
|-src
|-api 包含接口请求函数模块
|-table.js 表格列表mock数据接口的请求函数
|-user.js 用户登陆相关mock数据接口的请求函数
|-assets 组件中需要使用的公用资源
|-404_images 404页面的图片
|-components 非路由组件
|-SvgIcon svg图标组件
|-Breadcrumb 面包屑组件(头部水平方向的层级组件)
|-Hamburger 用来点击切换左侧菜单导航的图标组件
|-icons
|-svg 包含一些svg图片文件
|-index.js 全局注册SvgIcon组件,加载所有svg图片并暴露所有svg文件名的数组
|-layout
|-components 组成整体布局的一些子组件
|-mixin 组件中可复用的代码
|-index.vue 后台管理的整体界面布局组件
|-router
|-index.js 路由器
|-store
|-modules
|-app.js 管理应用相关数据
|-settings.js 管理设置相关数据
|-user.js 管理后台登陆用户相关数据
|-getters.js 提供子模块相关数据的getters计算属性
|-index.js vuex的store
|-styles
|-xxx.scss 项目组件需要使用的一些样式(使用scss)
|-utils 一些工具函数
|-auth.js 操作登陆用户的token cookie
|-get-page-title.js 得到要显示的网页title
|-request.js axios二次封装的模块
|-validate.js 检验相关工具函数
|-index.js 日期和请求参数处理相关工具函数
|-views 路由组件文件夹
|-dashboard 首页
|-login 登陆
|-App.vue 应用根组件
|-main.js 入口js
|-permission.js 使用全局守卫实现路由权限控制的模块
|-settings.js 包含应用设置信息的模块
|-.env.development 指定了开发环境的代理服务器前缀路径
|-.env.production 指定了生产环境的代理服务器前缀路径
|-.eslintignore eslint的忽略配置
|-.eslintrc.js eslint的检查配置
|-.gitignore git的忽略配置
|-.npmrc 指定npm的淘宝镜像和sass的下载地址
|-babel.config.js babel的配置
|-jsconfig.json 用于vscode引入路径提示的配置
|-package.json 当前项目包信息
|-package-lock.json 当前项目依赖的第三方包的精确信息
|-vue.config.js webpack相关配置(如: 代理服务器)
3. Secondary development
3.1. Project start
Here use vue-admin-template for secondary development. First clone the source code locally, or directly download the compressed package and decompress it locally.
Create a new folder (oa-system-front), put the source code file into it, open the folder with vscode, and add oa-system-front to the workspace
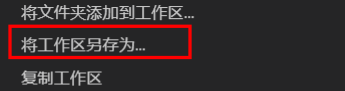
Save it under the open file! ! !
Download Node.js in advance, right-click the source code file, open it in the terminal, and use the npm install command to download related dependencies
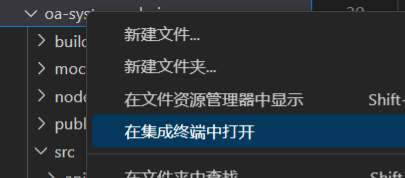
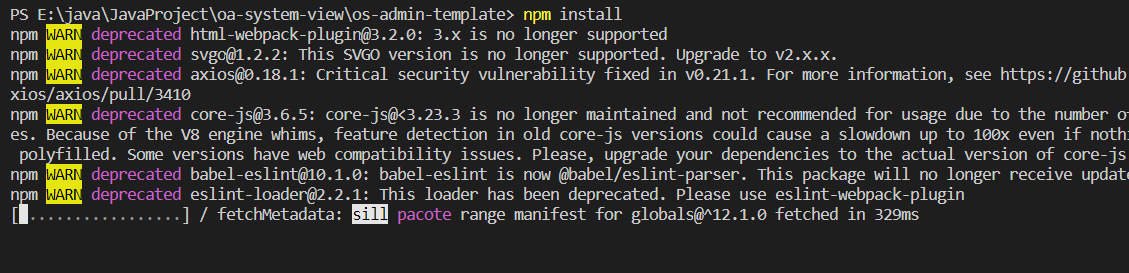
Start the project with the npm run dev command
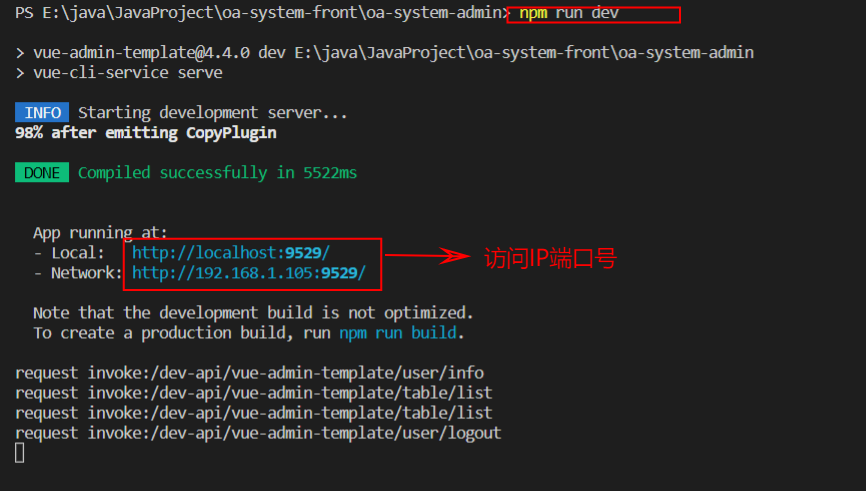
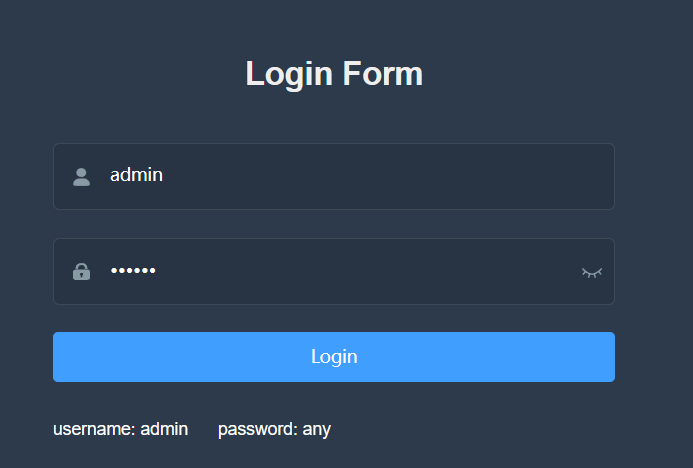
3.2, modify the relevant configuration
3.2.1. Set up a reverse proxy (forward the request to our backend)
Modification 1: Enter .env.development (cannot solve cross-domain problems)
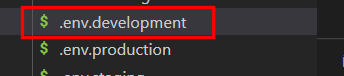

Modification 2: Modify the configuration in vue.config.js
Comment out the mock interface configuration
Configure the proxy to forward requests to the target interface
// before: require('./mock/mock-server.js')
proxy: {
'/dev-api': { // 匹配所有以 '/dev-api'开头的请求路径
target: 'http://localhost:8800',//类似于Nginx反向代理
changeOrigin: true, // 支持跨域
pathRewrite: { // 重写路径: 去掉路径中开头的'/dev-api'
'^/dev-api': ''
}
}
}
3.2.2. Write the back-end login interface
View the data of the login response

You can see that two requests have been sent! !
The response data of login and info requests are as follows


Write the corresponding interface
/**
* 返回结果状态码枚举
*/
@Getter
public enum ResultCodeEnum {
SUCCESS(200,"成功"),
FAIL(201, "失败");
private Integer code;
private String message;
private ResultCodeEnum(Integer code, String message) {
this.code = code;
this.message = message;
}
}
/**
* 全局返回同一结果封装类
*/
@Data
public class Result<T> {
private Integer code;
private String message;
private T data;
private Result(){}
public static <T> Result<T> build(T data){
Result<T> result=new Result();
if(data!=null){
result.setData(data);
}
return result;
}
public static <T> Result<T> build(T data,Integer code,String msg){
Result<T> build = build(data);
build.setCode(code);
build.setMessage(msg);
return build;
}
public static <T> Result<T> build(T data,ResultCodeEnum codeEnum){
Result<T> build = build(data);
build.setMessage(codeEnum.getMessage());
build.setCode(codeEnum.getCode());
return build;
}
//成功
public static <T> Result<T> success(){
return build(null,ResultCodeEnum.SUCCESS);
}
public static <T> Result<T> success(T data){
return build(data,ResultCodeEnum.SUCCESS);
}
//失败
public static <T> Result<T> fail(){
return build(null,ResultCodeEnum.FAIL);
}
public static <T> Result<T> fail(T data){
return build(data,ResultCodeEnum.FAIL);
}
public Result<T> message(String msg){
this.setMessage(msg);
return this;
}
public Result<T> code(Integer code){
this.setCode(code);
return this;
}
}
The interface is as follows:
@Api(tags = "后台登录管理")
@RestController
@RequestMapping("/admin/system/index")
public class IndexController {
@ApiOperation("登录接口")
@PostMapping("/login")
public Result login(){
Map<String,Object> map=new HashMap<>();
map.put("token","admin-token");
return Result.success(map);
}
@GetMapping("info")
public Result info() {
Map<String, Object> map = new HashMap<>();
map.put("roles","[admin]");
map.put("name","admin");
map.put("avatar","https://oss.aliyuncs.com/aliyun_id_photo_bucket/default_handsome.jpg");
return Result.success(map);
}
@PostMapping("logout")
public Result logout(){
return Result.success();
}
}
3.2.3. Modify request path and return status code interception
Modify the status code interceptor

It can be seen from this that you can only log in if the return status code is 20000 , but 200 in our unified result encapsulation class is success, so we can modify the front-end status code to intercept.
Modify in src/utils/request.js
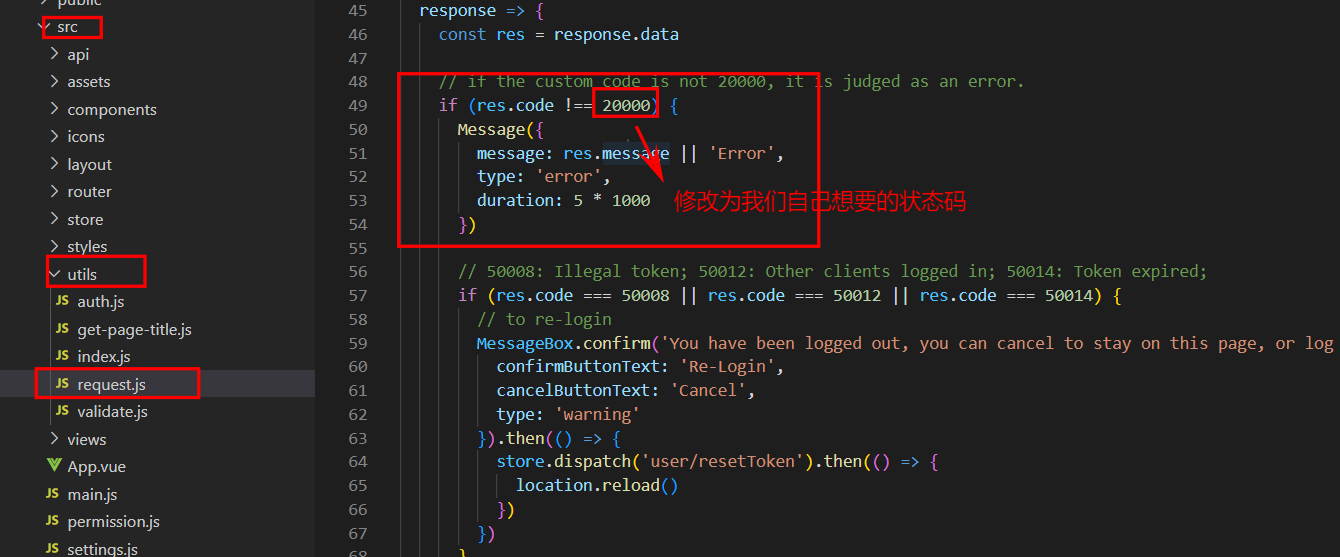
At this time, the status code I modified is 200, that is, when the status code is 200, you can log in to the system! !
Modify the login request path

The reverse proxy we configured above only solves the front part of the url, but the latter part has not been solved. At this time, the src/api/user.js file needs to be modified
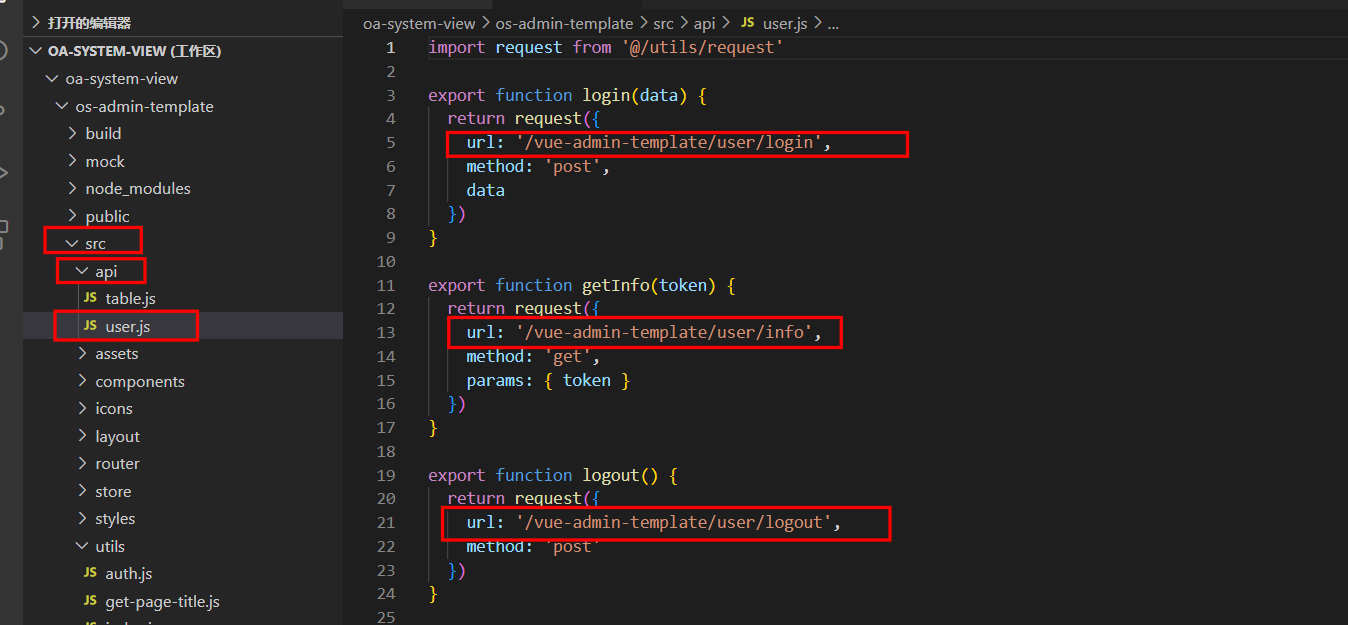
Just change the url to our backend interface, as follows:
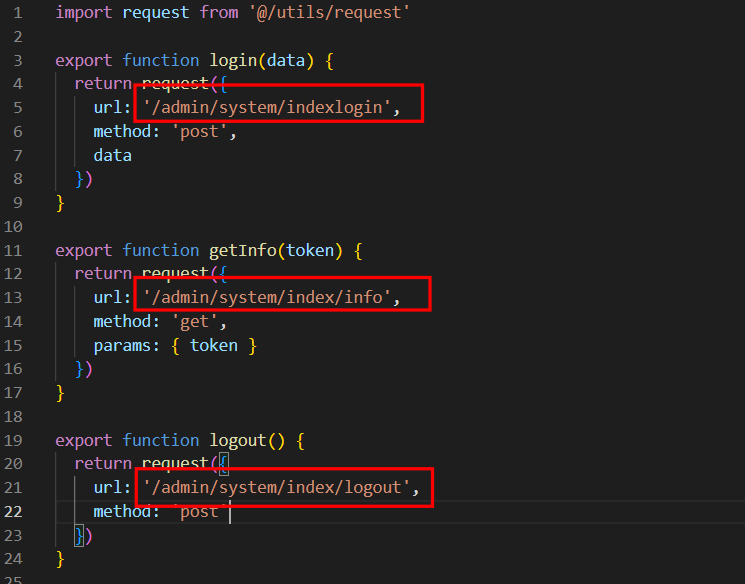
Start the backend project and frontend, log in and try it out! !


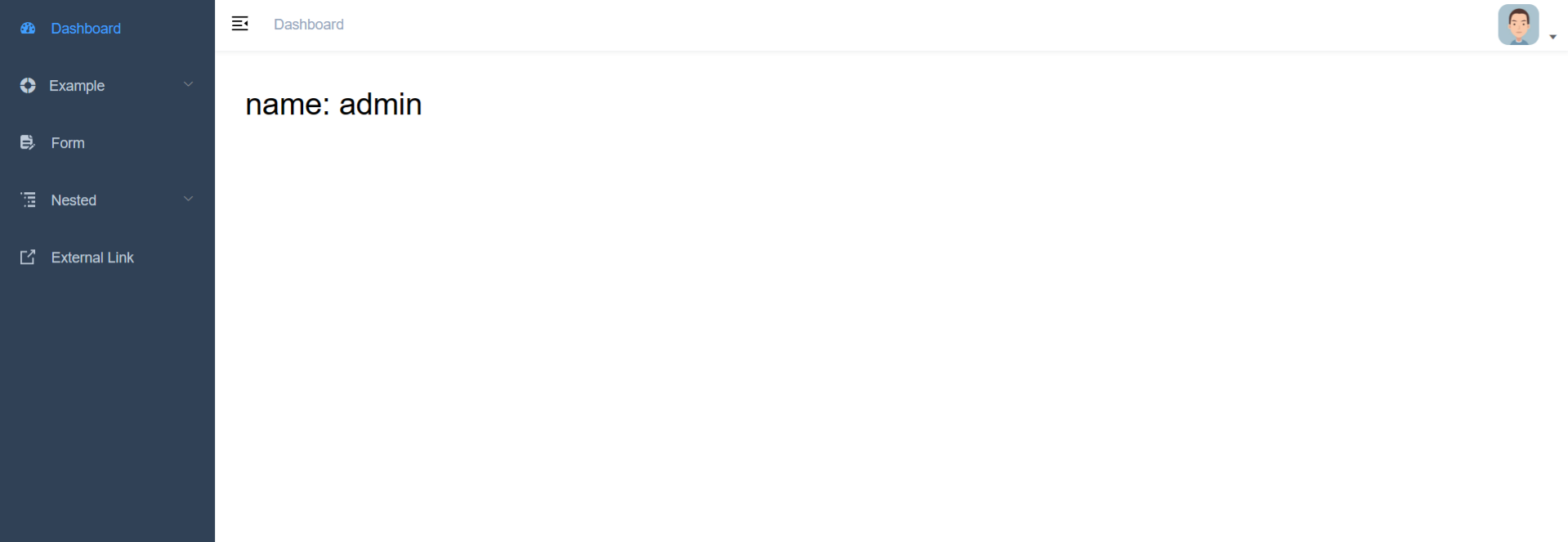
At this point, the login is successful! ! !
3.3. Page modification
3.3.1. Set page routing
Redefine constantRoutes in src/router/index.js
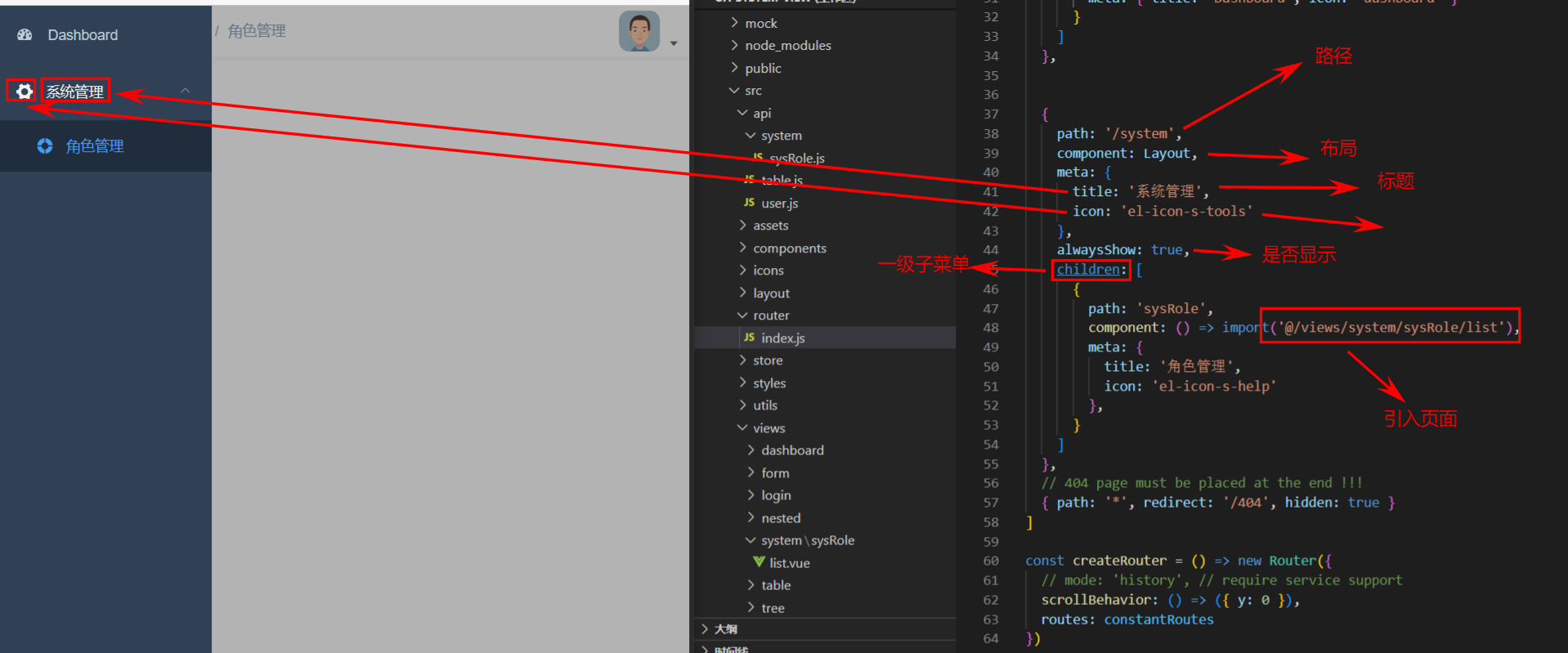
3.3.2. Create a js file (here sysRole.js) in the api folder to define interface information
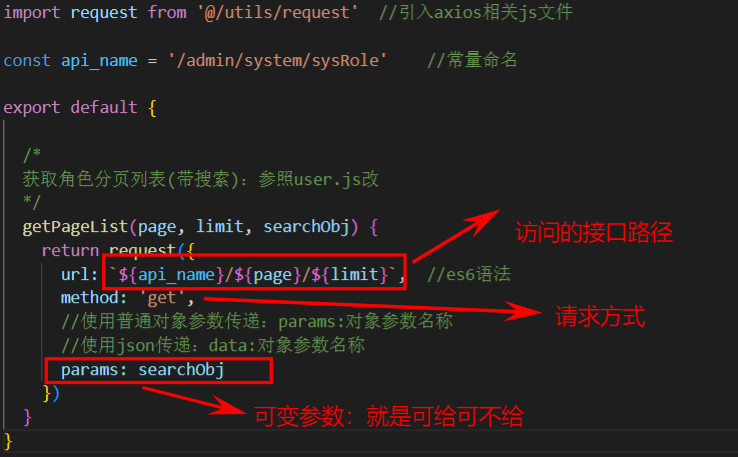
3.3.3. Create a page in the views folder, import the definition interface js file into the page, and call the interface to realize the function through axios
Refer to the page introduced above to create the corresponding folder and page (the suffix is vue)
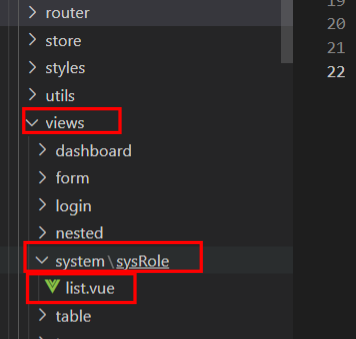
Initialize the page in the list page
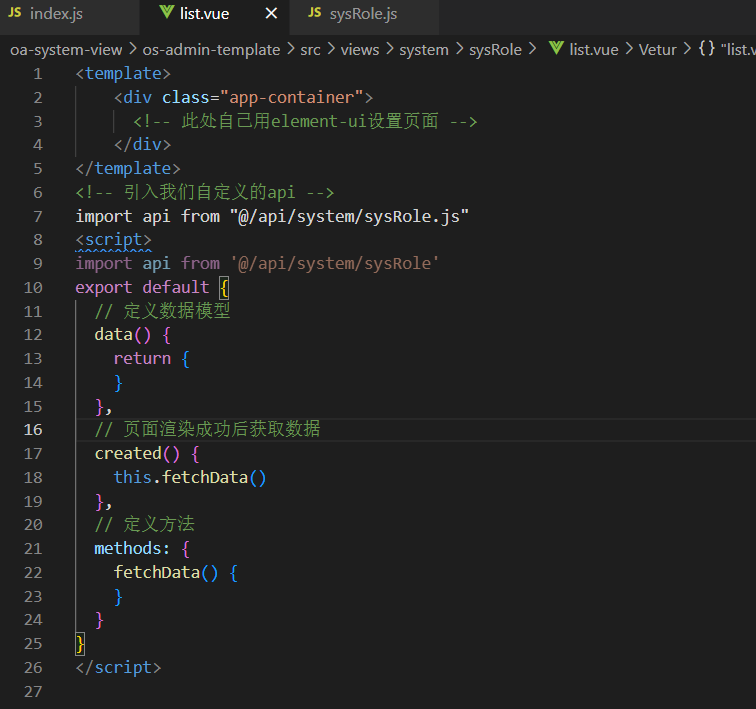
At this point, we can build our system by ourselves~~~~~~~~~~