Level 1: Say important things three times
The task of this level: the program accepts an input character, then outputs the character three times, and then outputs a !.
Add the code between Begin-End in the editor , the program receives an input character (the character will be given by the platform, you just need to get it), and then outputs the character three times and ends with ! .
// 包含标准输入输出函数库
#include <stdio.h>
// 定义main函数
int main()
{
// 请在下面编写将字符输出三遍的程序代码
/********** Begin *********/
int getchar(void);
char x = getchar();
putchar(x);
putchar(x);
putchar(x);
putchar('!');
/********** End **********/
return 0;
}
Level 2: Output format control of integer four arithmetic expressions
The task of this level: the user inputs two integers within four digits, please perform the four arithmetic operations on the two integers (in order to ensure that the division can be calculated correctly, the second integer input cannot be 0), and the output of the four arithmetic operations is required is perfectly aligned.
For example, given the input 1256 and 20, the output format of the four arithmetic operations that meet the above alignment requirements should be as follows (in order to make the space display more intuitive, the underscore _ is temporarily used here to represent the space ) :
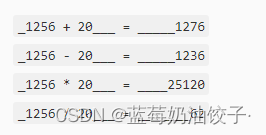
Add the code between Begin-End in the editor , for the two input non-negative integers (both less than 100000, the data is provided by the platform, and the two numbers are separated by a space, you can use it after you get it) Perform four arithmetic operations so that the output four arithmetic operations expressions are completely aligned. The specific output format requirements are as follows:
Each of the two integers occupies 5 characters. If the integer is less than 5 digits, the first integer is filled with spaces in front, and the second integer is filled with spaces in the back. Operators (+, -, *, / , = are both operators) occupy 1 character, and leave a space before and after the operator;
The output result occupies 10 characters, and if it is less than 10 characters, the front is filled with spaces.
//包含标准输入输出函数库
#include <stdio.h>
int main()
{
//声明两个整型变量,用于存储输入的两个整数
int x,y;
//请在Begin-End之间添加你的代码,按要求格式输出四则运算式子
/********** Begin *********/
scanf("%d%d",&x,&y);
int z1 = x + y;
int z2 = x - y;
int z3 = x * y;
int z4 = x / y;
printf("%5d",x);
printf(" + ");
printf("%-5d",y);
printf(" = ");
printf("%10d\n",z1);
printf("%5d",x);
printf(" - ");
printf("%-5d",y);
printf(" = ");
printf("%10d\n",z2);
printf("%5d",x);
printf(" * ");
printf("%-5d",y);
printf(" = ");
printf("%10d\n",z3);
printf("%5d",x);
printf(" / ");
printf("%-5d",y);
printf(" = ");
printf("%10d\n",z4);
/********** End **********/
return 0;
}
Level 3: Hello, birthday
Add the code between Begin-End in the editor on the right , the program receives your birthday input (the data is provided by the platform, and the input format is "year-month-day"), such as "1992 3 18", please output the greeting message" Hello! 3 18 1992" (output in "MMDDYYYY" format).
// 包含I/O流库iostream
#include <iostream>
// 加载名字空间std
using namespace std;
int main()
{
// 声明三个变量,分别用来存储年、月、日
int y, m, d;
// 请在Begin-End之间添加你的代码,输入你的生日,并按指定格式输出信息。
/********** Begin *********/
cin >> y >> m >> d;
cout<<"Hello!"<<" "<<m<<" "<<d<<" "<<y;
/********** End **********/
return 0;
}
Level 4: PI with different precision
Add the code between Begin-End in the editor on the right to realize the function of outputting PI with different precision . Specific requirements are as follows:
The input number is a non-negative integer n less than 15;
Output 5 PIs with different precisions , that is, keep n, n+1, n+2, n+3, and n+4 digits of PI after the decimal point , and each PI occupies one line.
#define PI 3.14159265358979323846
int main()
{
int n;
// 请在Begin-End之间添加你的代码,输入n,按不同的精度输出 PI。
/********** Begin *********/
cin >> n;
cout << setiosflags(ios::fixed) << setprecision(n) << PI << endl;
cout << setiosflags(ios::fixed) << setprecision(n+1) << PI << endl;
cout << setiosflags(ios::fixed) << setprecision(n+2) << PI << endl;
cout << setiosflags(ios::fixed) << setprecision(n+3) << PI << endl;
cout << setiosflags(ios::fixed) << setprecision(n+4) << PI << endl;
/********** End **********/
return 0;
}