Article directory
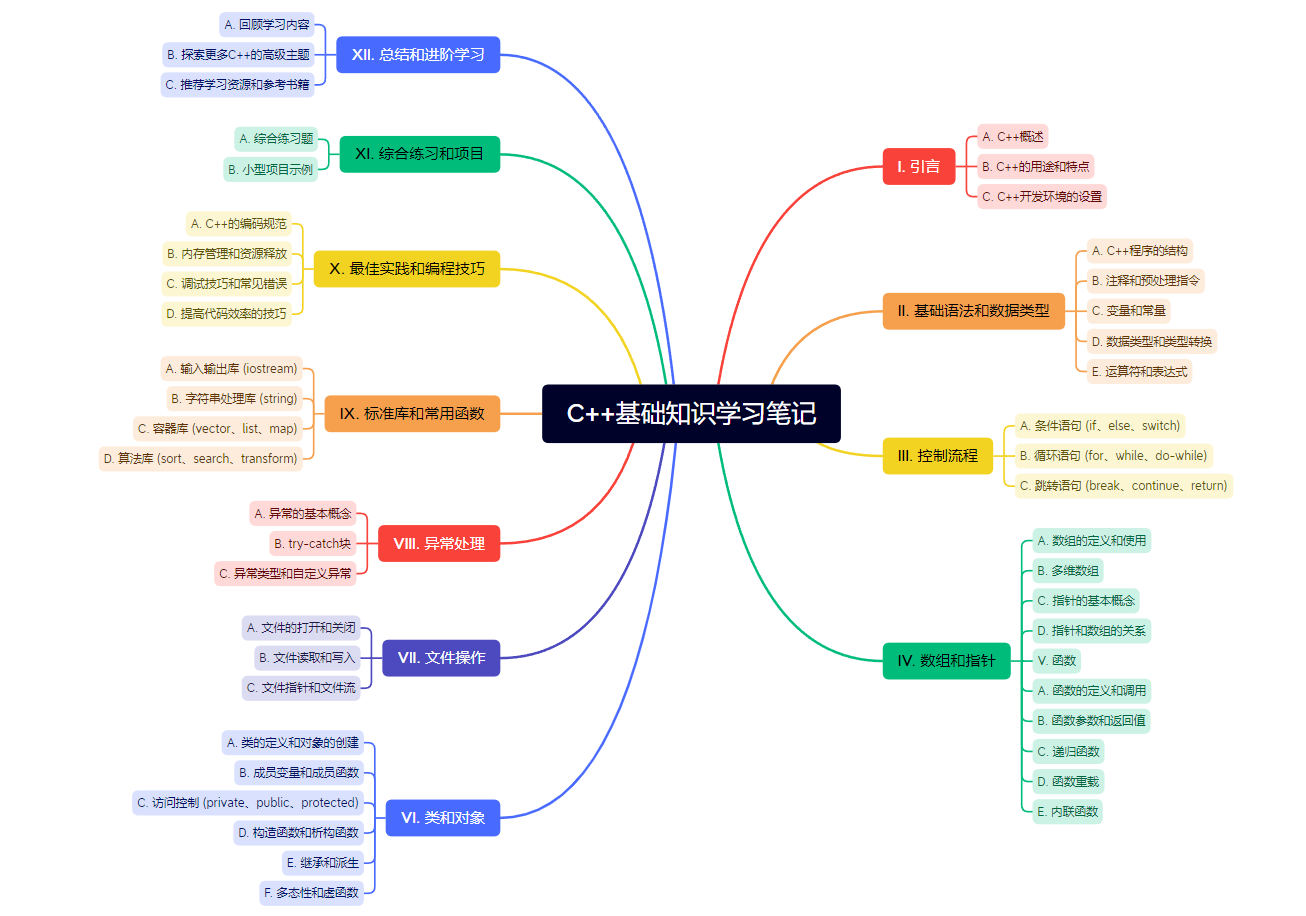
1️⃣ Input and output of C++ program
✨ input operation
C++ provides a variety of ways to perform input operations, including using cin
, , scanf
and so on.
♬ Use cin
to enter
#include <iostream>
int main() {
int number;
std::cout << "请输入一个整数: ";
std::cin >> number;
std::cout << "你输入的整数是: " << number << std::endl;
return 0;
}
std::cin
Used to receive input from the console.>>
Operators are used to read data from an input stream.
cin
It is an input stream object in the C++ standard library, and the >>
input value can be stored in the corresponding variable through the operator. In the above example, we used to cin
get an integer from the user input and store it in number
the variable.
♬ Use scanf
to enter
#include <cstdio>
int main() {
int number;
printf("请输入一个整数: ");
scanf("%d", &number);
printf("你输入的整数是: %d\n", number);
return 0;
}
scanf
Used to receive input from the console.%d
Is a format string that specifies that the data type of the input is an integer.&number
Is the address of the variable, used to store the input value into the variable.
scanf
It is an input function in the C standard library. It specifies the type of input through a format string, and uses the &
operator to obtain the address of the variable. In the above example, we used to scanf
get an integer from the user input and store it in number
the variable.
✨ output operations
C++ also provides a variety of ways to perform output operations, including using cout
, , printf
and so on.
♬ Use cout
for output
#include <iostream>
int main() {
int number = 10;
std::cout << "这是一个数字: " << number << std::endl;
return 0;
}
std::cout
Used to output information to the console.<<
Operators are used to insert data into an output stream.
cout
It is an output stream object in the C++ standard library, and <<
the operator can be used to output data to the console. In the above example, we used to cout
output a number to the console.
♬ Use printf
for output
#include <cstdio>
int main() {
int number = 10;
printf("这是一个数字: %d\n", number);
return 0;
}
-
printf
Used to format output messages to the console. -
%d
is a format string that specifies that the data type of the output is an integer.
printf
It is an output function in the C standard library, and the output type and format are specified by a format string. In the above example, we used to printf
output a number to the console.
✨ difference
cin
andcout
are the standard input and output streams of C++, andscanf
andprintf
are the input and output functions of the C language.cin
andcout
provide a more advanced and safer way of input and output, you can directly use the>>
and<<
operator for input and output.scanf
andprintf
need to use the format string to specify the data type of the input and output, and need to use the address of the variable to read and write the data.
In C++, it is recommended to use cin
and cout
for input and output operations, because they are more convenient, intuitive and safe.
2️⃣ Namespace and using declaration
In C++, namespaces are used to organize code and avoid name conflicts. A common namespace is std
that it contains many definitions of the standard library. To simplify coding, we can use using
declarations to avoid frequent use of full namespace qualifiers.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "请输入一个整数: ";
cin >> number;
cout << "你输入的整数是: " << number << endl;
return 0;
}
In the above example, we use using namespace std;
the declaration so that we can directly use cout
and cin
without writing std::cout
and std::cin
. However, it should be noted that in large projects, in order to avoid naming conflicts, it is best to avoid using using namespace
, and use namespace qualifiers to specify explicitly.
3️⃣ Variables, constants and data types
✨ variables
In C++, variables are used to store and manipulate data. Before using a variable, you need to declare the variable and specify its data type.
int main() {
int age = 30; // 整数类型变量
float weight = 68.5; // 浮点数类型变量
char letter = 'A'; // 字符类型变量
bool isTrue = true; // 布尔类型变量
return 0;
}
In the above example, we declared several variables of different types, including integer type ( int
), floating point type ( float
), character type ( char
), and boolean type ( bool
). Through assignment, we can store specific values into these variables.
✨ constant
Constants are unchangeable values used to store fixed data. In C++, const
constants can be defined using the keyword.
int main() {
const int MAX_VALUE = 100; // 整数常量
const float PI = 3.14159; // 浮点数常量
const char NEW_LINE = '\n'; // 字符常量
return 0;
}
In the above example, we const
defined several constants using the keyword. The value of a constant cannot be changed after it is defined, and it is used to store data that remains unchanged during program execution.
✨ data types
C++ provides rich data types for storing different types of data. Common data types include integer types, floating point types, character types, Boolean types, etc.
♬ integer type
C++ provides integer types of different sizes, including int
, short
, long
, long long
and so on.
int main() {
int age = 30; // 有符号整数类型
unsigned int count = 10; // 无符号整数类型
short distance = 1000; // 短整数类型
long population = 7000000000; // 长整数类型
return 0;
}
♬ Floating point type
C++ provides floating-point number types with different precisions, including float
, double
, long double
and so on.
int main() {
float weight = 68.5; // 单精度浮点数类型
double pi = 3.14159; // 双精度浮点数类型
return 0;
}
♬ character type
C++ uses the character type char
to represent individual characters.
int main() {
char letter = 'A'; // 字符类型
char name[] = "John"; // 字符串类型
return 0;
}
♬ Boolean type
C++ provides the boolean type bool
, which is used to represent the truth value, and the value is true
or false
.
int main() {
bool isTrue = true; // 布尔类型
return 0;
}
In the above examples, we have shown several common data types and how to use them. According to actual needs, choosing the appropriate data type can improve the efficiency and readability of the program.
This is a study note of input and output, variables, constants and data types in C++ basic grammar. In the next study, we will explore more features and syntax of C++ in depth. Remember to keep practicing and writing code to deepen your understanding and mastery of C++.