The length of the previous article is a bit too large, and it is not convenient to find things. We will continue to describe the newly opened content:
The next thing we are going to do is to follow the target, but the problem we have so far is that we don't know whether the object we want to follow is fixed, so we first use color recognition to replace it (the thing we are looking for is an orange object, and at the same time, We will use K210 to directly drive our servos to make the camera follow the object)
1. Object color recognition:
We use the code that finds the largest color patch to track our object
import sensor,lcd,time
import gc,sys
import ustruct
from machine import UART,Timer
from fpioa_manager import fm
#映射串口引脚
fm.register(6, fm.fpioa.UART1_RX, force=True)
fm.register(7, fm.fpioa.UART1_TX, force=True)
uart = UART(UART.UART1, 115200, read_buf_len=4096)
#摄像头初始化
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.set_vflip(1) #后置模式,所见即所得
sensor.set_auto_whitebal(False)#白平衡关闭
#lcd初始化
lcd.init()
# 颜色识别阈值 (L Min, L Max, A Min, A Max, B Min, B Max) LAB模型
# 此处识别为橙色,调整出的阈值,全部为红色
barries_red = (20, 100, -5, 106, 36, 123)
clock=time.clock()
#打包函数
def send_data_wx(x,a):
global uart;
data = ustruct.pack("<bbhhhhb",
0x2c,
0x12,
int(x),
int(a),
0x5B)
uart.write(data);
#找到最大色块函数
def find_max(blods):
max_size=0
for blob in blobs:
if blob.pixels() > max_size:
max_blob=blob
max_size=blob.pixels()
return max_blob
while True:
clock.tick()
img=sensor.snapshot()
#过滤
blods = img.find_blobs([barries_red],x_strid=50)
blods = img.find_blobs([barries_red],y_strid=50)
blods = img.find_blobs([barries_red],pixels_threshold=100)
blods = img.find_blobs([barries_red],area_threshold=60)
blobs = img.find_blobs([barries_red]) #找到阈值色块
cx=0;cy=0;
if blobs:
max_blob = find_max(blobs) #找到最大色块
cx=max_blob[5]
cy=max_blob[6]
cw=max_blob[2]
ch=max_blob[3]
img.draw_rectangle(max_blob[0:4])
img.draw_cross(max_blob[5],max_blob[6])
lcd.display(img) #LCD显示图片
print(max_blob[5],max_blob[6])
send_data_wx(max_blob[5],max_blob[6])
2. The driver code of the steering gear:
from machine import Timer,PWM
import time
#PWM 通过定时器配置,接到 IO17 引脚
tim = Timer(Timer.TIMER0, Timer.CHANNEL0, mode=Timer.MODE_PWM)
S1 = PWM(tim, freq=50, duty=0, pin=17)
'''
说明:舵机控制函数
功能:180 度舵机:angle:-90 至 90 表示相应的角度
360 连续旋转度舵机:angle:-90 至 90 旋转方向和速度值。
【duty】占空比值:0-100
'''
def Servo(servo,angle):
S1.duty((angle+90)/180*10+2.5)
while True:
#-45 度
Servo(S1,-45)
time.sleep(2)
#0 度
Servo(S1,0)
time.sleep(2)
#45 度
Servo(S1,45)
time.sleep(2)
Let's take a look at the documentation, how to use the 180° servo:
Servo drive, need 3 wires (GND VC++(5V) signal(P17))
The control of 180° steering gear generally requires a time base pulse of about 20ms. The high level part of the pulse is generally the angle control pulse part in the range of 0.5ms-2.5ms, and the total interval is 2ms . Taking the 180-degree angle servo as an example, the corresponding control relationship in MicroPython programming is from -90 ° to 90°.
tim = Timer(Timer.TIMER0, Timer.CHANNEL0, mode=Timer.MODE_PWM)#配置定时器
S1 = PWM(tim, freq=50, duty=0, pin=17) #开启S1口的PWM控制
Turn on S1 (Pin17 PWM, set the initial duty cycle to 0)
The PWM objects are under the machine module.
【tim】The PWM of K210 relies on the timer to generate waveforms, set
【freq】PWM frequency at the top
【duty】PWM duty cycle
【pin】PWM output pin
【enable】Whether to generate waveform immediately after constructing the object, the default is True.
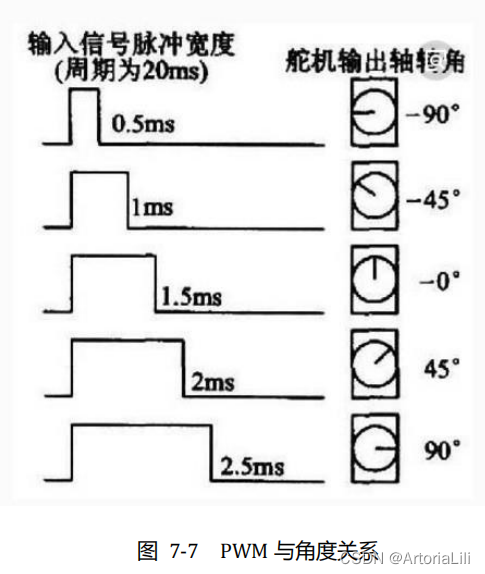
In the software, these angles have been packaged, we can directly drive the steering gear through PWM, the flow chart is as follows:
in:
def Servo(servo,angle):
S1.duty((angle+90)/180*10+2.5)
This code is for us to calculate the angle of rotation, call it, we can directly go to the angle we need, where 0 is our center position (servo is our position, angle is the angle of rotation)
=====>> to be continue...