We plan to brush different cells in the block map. I was interested in the function similar to the Warcraft editor before, so I realized it with the method of ray + object generation. The screenshot of the realization effect is as follows:
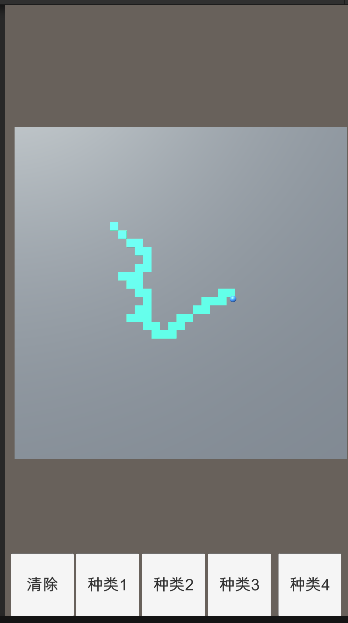
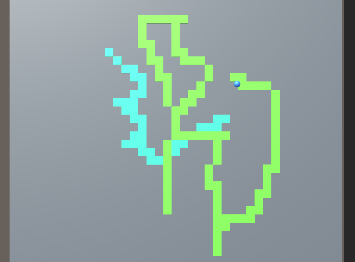
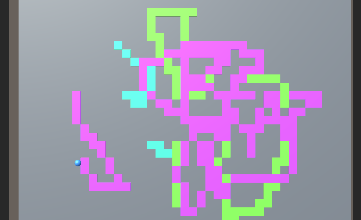
Clear effect:
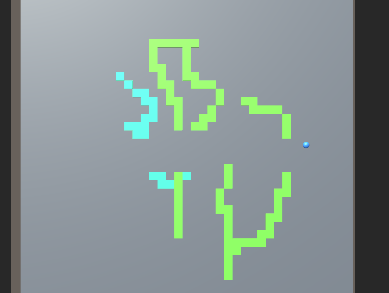
The implementation code is as follows:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class CreatMapUpdate : MonoBehaviour
{
public GameObject[] objs;
public Button[] btns;
public int creatID = 0;
public float length = 2.5f;
public float width = 2.5f;
public Dictionary<Vector2, GameObject> dic = new Dictionary<Vector2, GameObject>();
// Start is called before the first frame update
void Start()
{
for (var _i = 0; _i < btns.Length; _i++)
{
var _j = _i;
btns[_i].onClick.AddListener(delegate { Debug.LogError(_j); creatID = _j - 1; });
}
}
// Update is called once per frame
void Update()
{
if (Input.GetMouseButton(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition/*+new Vector3(15,0,-15)*/);
RaycastHit hit;
//判断是否碰撞到物体
bool isCollider = Physics.Raycast(ray, out hit) && hit.transform.tag == "TouchCreat";
if (isCollider)
{
var _creatPos = new Vector2((int)(hit.point.x / length), (int)(hit.point.z / width));
//Debug.Log(hit.point +" "+_creatPos);
GameObject.Find("指示球").transform.position = hit.point;
//以0,0点为中心,将地图切割为n个小地图块
if (dic.ContainsKey(_creatPos))
{
var _obj = dic[_creatPos];
if (creatID == -1)
{
dic.Remove(_creatPos);
Destroy(_obj);
}
else if (_obj.name != objs[creatID].name)
{
Destroy(_obj);
_obj = CreatObj(objs[creatID]);
dic[_creatPos] = _obj;
_obj.transform.position = new Vector3(_creatPos.x * length, 0, _creatPos.y * length);
_obj.transform.SetParent(transform);
}
}
else if (creatID >= 0)
{
var _obj = CreatObj(objs[creatID]);
dic.Add(_creatPos, _obj);
_obj.transform.localPosition= new Vector3(_creatPos.x * length, 0, _creatPos.y * length);
_obj.transform.SetParent(transform);
//Debug.Log(objs.Length + " " + creatID);
}
}
}
}
public GameObject CreatObj(GameObject _oo)
{
var _obj = GameObject.Instantiate(_oo) as GameObject;
_obj.name = _oo.name;
return _obj;
}
}
Engineering resource download address: map brush editor.unitypackage-C# document resource-CSDN library