In the following description of the characteristics of the JAVA language, the error is (D)
A.JAVA program has nothing to do with the platform, good portability
B.JAVA supports multithreading
C.JAVA supports distributed network applications
D.JAVA is a pure object-oriented programming language that supports single inheritance and multiple inheritance
The Java language has ten major characteristics, namely: simplicity, object-oriented, distribution, compilation and interpretation, robustness, security, portability, high performance, multi-threading, and dynamics.
Java does not support multiple inheritance
Which of the following identifiers is illegal in the Java language (C)
A.persons$
B.yourname
C.class
D._hello
Java identifier naming rules are as follows: 1. It can be composed of letters, numbers, underscore_ and dollar sign $; 2. It cannot start with a number; 3. It cannot conflict with keywords; 4. It cannot conflict with the class name of the java class library;
class is java keyword
Suppose the array Array is defined by the following statement (C) int[] Array=new int[10]; then the correct reference method of the last element of the array is:
A、Array[0]
B、Array[10]
C、Array[9]
D、Array
Java subscripts start from 0, so the subscript of the last element is 9.
Regarding the member variables modified by the access control character protected, the following statement is correct (B)
A.Can be referenced by the class itself, by other classes in the same package, or by other classes in other packages
B.Can be accessed and referenced by the class itself and all subclasses of the class
C.Can only be accessed and modified by the class itself
D.Can only be accessed by classes in the same package
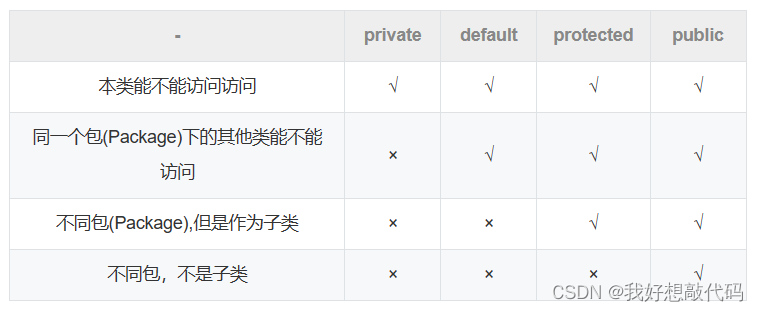
Which statement about constructors is correct? (C)
A.A class can have only one constructor
B.A class can have multiple constructors with different names
C.The constructor has the same name as the class
D.The constructor must be defined by itself, and the constructor of the parent class cannot be used
1. A constructor is also called a constructor, and the name of the constructor is the same as the name of the class.
2. The constructor cannot have a return value, nor can it be declared with void.
3. The constructor can have parameters or no parameters. In a class, multiple constructors can be defined.
4. The constructor is mainly to initialize the class and is called when new.
5. When any class object is generated, the constructor of the class must be called.
6. No matter how many constructors are defined in a class, only one of the constructors will be called when a class object is generated.
7. All classes have constructors. When defining a class, if you do not define a constructor, the system will generate an empty constructor by default. If you define a constructor yourself, the default constructor will be invalid.
8. Create a class object statement: A aa = new A(); In fact, A() after new is the constructor.
Which of the following statements about abstract methods is correct (C)
A.Abstract methods can have method bodies
B.Abstract methods can appear in non-abstract classes
C.An abstract method is a method without a method body
D.Methods in an abstract class are abstract methods
The characteristics of the abstract method:
An abstract method is an incomplete method without a method body.
Abstract methods must be abstract classes, abstract classes do not necessarily have abstract methods (methods in interfaces must be abstract methods!)
Abstract methods and classes must be modified by abstract
Abstract classes cannot be instantiated through new. To use abstract methods in abstract classes, subclasses must override all the abstract methods and create subclass objects to call. If the subclass only covers some abstract methods, then the subclass is still an abstract class.
Abstract methods can have ordinary methods
An abstract method must be an abstract class; there may be no abstract method in an abstract class
The abstract keyword and which keywords cannot coexist:
final: A class modified by final cannot have subclasses (cannot be inherited). The class modified by abstract must be a parent class (must be inherited).
private: The private abstract method in the abstract class cannot be overridden without being known by the subclass. The abstract method appears to need to be overridden.
static: If static can modify the abstract method, then even the object is saved, and the class name can be called directly. Then the abstract method is meaningless to run.
Which of the following statements about inheritance is correct (A)
A. Classes in Java only allow single inheritance
B.A class can only implement one interface in Java
C.A class cannot inherit a class and implement an interface at the same time in Java
D.Interfaces in Java only allow single inheritance
single inheritance, multiple interfaces
In Java a class can inherit a class and implement an interface at the same time
Class inheritance format: subclass extends parent class
Interfaces can only inherit interfaces, not classes, and interfaces support multiple inheritance (classes have a single inheritance relationship). The interface inheritance syntax is as follows:
[Modifier] interface interface name extends interface 1, interface 2
The inheritance relationship between classes is represented by the extends keyword, and the inheritance relationship between interfaces can only be represented by the extends keyword.
There can only be an implementation relationship between the interface and the implementing class, and the class implements the interface, which is represented by the implements keyword.
If there is a definition String tom="I am a good cat"; then the return value of tom.replace('a', 'b') is (C).
A."I am a good cat" B." I bm a good cat" C."I bm b good cbt" D."I am a good cbt"
The replace() method replaces all occurrences of the searchChar character in the string with the newChar character, and returns the replaced new string.
Syntax: replace(searchChar, newChar)
parameter:
searchChar -- the original character.
newChar -- the new character.
return value:
The new string generated after the substitution.
Subclasses of the OutputStream class or the Writer class are all (B)
A.input stream
B.output stream
C.input/output stream
D.Java communication classes
All output stream classes in Java are subclasses of the OutputStream abstract class (byte output stream) and the Writer abstract class (character output stream).
If you want to monitor keyboard events, the method you can use to add a monitor is (B)
A.addActionListener
B . addKeyListener
C.addItemListener
D.addTextListener
addActionListener: listener parent class, events that other listeners can listen to can be captured by it
addKeyListener: monitor the keyboard
addItemListener: Used to capture events generated by components with Item
addTextListener: text listening
ps: look at the meaning of the word
The correct command to compile HelloWorld.java is: (C)
A. java HelloWorld.class
B. java HelloWorld.java
C. javac HelloWorld.java
D. javac HelloWorld.class
The correct command to run HelloWorld.java correctly is: (A)
A. java HelloWorld
B. javac HelloWorld.java
C. javac HelloWorld.class
D. javac HelloWorld.class
The following are not Java reserved words: (C)
A. abstract
B. if
C. malloc
D. this
malloc is in C language
The following are illegal Java identifiers: (D)
A. abc_xyz
B. $abc
C. _abc_
D. abc-d
Java identifier naming rules are as follows: 1. It can be composed of letters, numbers, underscore_ and dollar sign $; 2. It cannot start with a number; 3. It cannot conflict with keywords; 4. It cannot conflict with the class name of the java class library;
In Java, the following description of the inheritance relationship of classes is correct (A).
A.A parent class can have multiple subclasses
B.A subclass can have multiple parent classes
C.Subclasses can use all properties and methods of parent class
D.Subclasses must have more member methods than parent classes
A subclass can have only one parent class
Subclasses can inherit all properties and methods of the parent class, but can only use non-private properties and methods
The following statement about the interface is correct (C).
A.A class can only implement one interface
B.There can be no inheritance relationship between interfaces
C.Implementing an interface must implement all methods of the interface
D.Interface and abstract class are the same thing
A class can implement multiple interfaces
There is an inheritance relationship between interfaces, and it is multiple inheritance
An abstract class is an abstraction of a thing, that is, an abstraction of a class, while an interface is an abstraction of behavior. An abstract class abstracts the entire class as a whole, including attributes and behaviors, but an interface abstracts the parts (behaviors) of the class. To give a simple example, airplanes and birds are different things, but they all have one thing in common, that is, they can fly.
Assuming String s="I love java", the result of s.indexOf('a', 4) is (A)
A.8
B.9
C.10
D.11
indexOf(ch, fromIndex): Returns the index of the first occurrence of the specified character in the string starting from the fromIndex position, or -1 if there is no such character in the string.
indexOf(ch): Returns the index of the first occurrence of the specified character in the string, or -1 if there is no such character in the string.
s.indexOf('a', 4): Start searching from the position where the subscript is 4, and the subscript position where a appears for the first time is 8
Note the spaces
Assuming double d=3.14, the value of Math.ceil(d) is (B).
A.3.0
B.4.0
C.3.1
D.3.2
math.cel(a)
The return value is double type, returning a minimum integer greater than or equal to the parameter a. That is, it returns an integer that is the smallest of all integers greater than or equal to a.
Math.floor(a)
The return value is double type, returning a maximum integer less than or equal to the parameter a. That is, it returns an integer that is the largest of all integers less than or equal to a.
Math.round(a)
Returns the integer closest to the parameter a. This method is equivalent to Math.floor(a + 0.5) and converts the result to long or int type, that is, rounds to the nearest integer.
In Java, when trying to operate on a null object, an exception of type (D) will be generated.
A.ArithmeticException
B.EOFException
C. IOException
D.NullPointerException
ArithmeticException is thrown when an abnormal operation condition occurs. For example, an instance of this class is thrown when an integer is "divided by zero".
EOFException: This exception is thrown when the end of the file or stream is unexpectedly reached during input.
IOException : input or output exception
NullPointerException: null pointer exception
If the content (data) in the file is to be read byte by byte, stream (D) should be used.
A.FileWriter
B.FileReader
C.FileOutputStream
D.FileInputStream
FileWriter : character output stream
FileReader: character input stream
FileOutputStream: byte output stream
FileInputStream: byte input stream