1. Understanding of database constraints
The concept of database constraints: database constraints are an important function of relational databases. "
Database constraints:
not null: cannot store null values
unique: guarantees that each row of a column must have a unique value
default: Specifies the default value when no value is assigned to the column
primary key: primary key, equivalent to the combination of not null and unique, the identity of each record
aoto_increment: automatically add the primary key value, you can also add it manually
foreign key: foreign key, the relationship between multiple tables, requires that a certain record must exist in another table
2. Not null constraint
Create a student table with two columns, namely: the id type is int, the constraint is not null, the name type is varchar, and the maximum storage is 20 characters
-- 如果已有student表,请先删除,因为同一个库里面不能存在相同名的表
create table student(id int not null,name varchar(20));
The constraint of id is not null, indicating that the id column cannot be empty
desc student;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | NO | | NULL | |
| name | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
Looking at the structure of the student table, you can find that the Null column of the id row is NO, which means that the id column cannot be NULL
3. Unique constraints
Create a student table with two columns, namely: id type is int constraint is unique, name type is varchar and can store up to 20 characters
-- 如果已有student表,请先删除,因为同一个库里面不能存在相同名的表
create table student(id int unique,name varchar(20));
The constraint of id is unique, which must be unique and cannot be repeated
desc student;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | UNI | NULL | |
| name | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
Looking at the structure of the student table, you can find that the key column of the id row is UNI, which means that the id must be unique and cannot be repeated
4. Default constraints
Create a student table with two columns, namely: id type is int, name type is varchar, the maximum storage is 20 characters, and the constraint is default
-- 如果已有student表,请先删除,因为同一个库里面不能存在相同名的表
create table student(id int,name varchar(20) default 'unkown');
The constraint of name is default, when no content is added to name, the default is unknown
desc student;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | varchar(20) | YES | | unkown | |
+-------+-------------+------+-----+---------+-------+
Looking at the structure of the student table, you can find that the default column of the name row is unknown, which means that when no content is added to the name, the default is unknown
5. primary key constraints
Create a student table with two columns, namely: id type is int constraint is primary, name type is varchar and can store up to 20 characters
-- 如果已有student表,请先删除,因为同一个库里面不能存在相同名的表
create table student(id int primary key,name varchar(20));
The constraint of id is primary key, indicating that id is the primary key, and the primary key is equivalent to the combination of not null and unique, which cannot be empty or repeated
desc student;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | NO | PRI | NULL | |
| name | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
2 rows in set (0.00 sec)
Looking at the structure of the student table, you can find that the Null column in the id row is NO, and the Key column in the id row is PRI, indicating that the id cannot be empty or repeated
When we create the student table, when the id is set to not null and unique, it is equal to the primary key
-- 如果已有student表,请先删除,因为同一个库里面不能存在相同名的表
create table student(id int not null unique,name varchar(20));
desc student;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | NO | PRI | NULL | |
| name | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
When setting the id constraint to not null and unique, when viewing the student table structure, it is found that it is the same as the structure constrained to the primary key
6. auto_increment constraint
For the primary key of integer type, it is often used with auto_increment. When the field corresponding to the inserted data does not give a value, use the maximum value +1
create table student(id int primary key auto_increment,name varchar(20));
desc student;
+-------+-------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+----------------+
| id | int(11) | NO | PRI | NULL | auto_increment |
| name | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+----------------+
When the constraint of id is set to primary key and auto_increment, when no content is added to id, the default is the maximum value +1
When we just add content to the name column in this table
create table student(id int primary key auto_increment,name varchar(20));
select * from student;
+----+------+
| id | name |
+----+------+
| 1 | 张三 |
| 2 | 李四 |
+----+------+
The id column will add a value by default, and it is the maximum value +1
7. Foreign key constraints
Foreign keys are used to associate primary keys and unique values of other tables to create constraints between the two tables. Syntax:
foreign key (字段名) references 主表(列)
First create a class class table:
create table class(id int primary key auto_increment,className varchar(20));
There are two columns in the class table, namely:
id column, type is int, constraint is primary key and auto increment
className column, the type is varchar and can store up to 20 characters
First create a student student table:
create table student(id int primary key auto_increment,
classId int ,
name varchar(20),
foreign key (classId) references class (id));
There are three columns in the student table, namely:
id column, type is int, constraint is primary key and auto increment
classId column, type is int
name column, the type is varchar and can store up to 20 characters
classId creates constraints for foreign keys and ids in the class table
The data in the student table depends on the data in the class table, and the data in the class table must be binding on the student table
The class table that acts as a constraint here is called the "parent table"; the constrained table is called the "child table"
Add some content to the class table:
insert into class (className) values('Java'),('C'),('python');
Add some content to the student table:
insert into student (classId,name) values(1,'张三'),(1,'李四'),(2,'王五'),(3,'赵六');
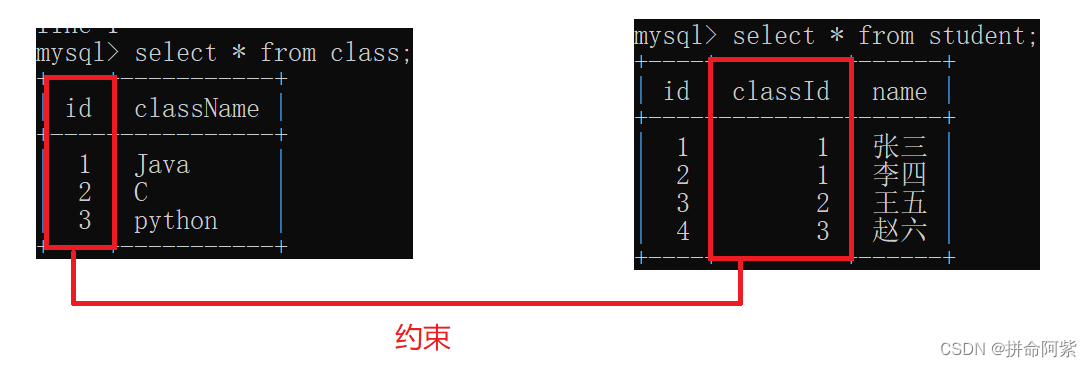