The apps we develop are more or less connected to the Internet. Or pull server-side data to update the UI, or push your own messages through the network, or delete your own photos on the mobile phone, or open the music player app to download your favorite songs. How to request, receive, process, and send data is what we will discuss in this section.
computer theory
1. Talk about the difference between GET and POST in HTTP
Keywords: #direction#type#argumentposition
In terms of direction, GET is to obtain information from the server, and POST is to send information to the server.
In terms of type, GET handles static and dynamic content, and POST only handles dynamic content.
From the perspective of parameter position, the parameters of GET are in its URI, and the parameters of POST are in its package body: From this perspective, POST is more secure and secretive than GET.
GET can be cached and stored in the browser history, and its content is theoretically limited in length; POST is just the opposite in these three points.
2. Talk about the concepts of Session, Token, and Cookie
Keywords: #UserAuthentication#Client#Server
Session is a data structure used by the server to authenticate and track users. It determines the user by judging the information sent by the client, and the unique identification of the user is the Session ID sent by the client.
Token is a string of strings generated by the server. It is the token for the client to request and the unique identifier for the server to determine the user. Session ID is often used as Token. The appearance of Token prevents the server from frequently querying user names and passwords, and reduces the query pressure on the database.
Cookie is a mechanism for client to save user information. The HTTP protocol for the first session will record a Session ID in the cookie, and then send the Session ID to the server each time.
Session is generally used for user authentication. It is stored in a file on the server by default, of course, it can also be stored in memory or database.
If the client disables cookies, the client will use URL rewriting technology, that is, add the Session ID at the end of the URL during the session and send it to the server.
3. In a website connected by HTTPS, after entering the account password and clicking login, what happened before the server returns the request?
Keywords: #lock#client#server
1) The client packs the request. Including url, port, your account password and so on. Account password login should use the Post method, so the relevant user information will be loaded into the body. This request should contain three aspects: network address, protocol, and resource path. Note that this is HTTPS, that is, HTTP + SSL / TLS, and a module for processing encrypted information is added to HTTP (equivalent to a lock). This process is equivalent to the client requesting the key.
2) The server accepts the request. Generally, the client's request will be sent to the DNS server first. The DNS server is responsible for resolving your network address into an IP address, which corresponds to a machine on the network. Among them, the problems of Hosts Hijack and ISP failure may occur. After passing the DNS level, the information reaches the server. At this time, the client will establish a socket connection with the port of the server. The socket usually parses the request in the form of a file descriptor. This process is equivalent to the server-side analyzing whether to send the key template to the client.
3) The server returns a digital certificate. There will be a set of digital certificates (equivalent to a key template) on the server side, and this certificate will be sent to the client first. This process is equivalent to sending the key template from the server to the client.
4) The client generates encrypted information. According to the received digital certificate (key template), the client will generate a key and lock the content, and the information has been encrypted at this time. This process is equivalent to the client generating a key and locking the request.
5) The client sends encrypted information. The server will receive the digital certificate lock information sent by itself. The key generated at this time is also sent to the server. This process is equivalent to the client sending a request.
6) The server side unlocks the encrypted information. After receiving the encrypted information, the server will decrypt it according to the obtained key, and symmetrically encrypt the data to be returned. This process is equivalent to the server-side unlock request, generation, and lock response information.
7) The server returns information to the client. The client receives the corresponding encrypted information. This process is equivalent to the server sending a response to the client.
8) The client unlocks and returns information. The client will use the newly generated key to decrypt and display the content on the browser.
The flowchart of the whole process is as follows:
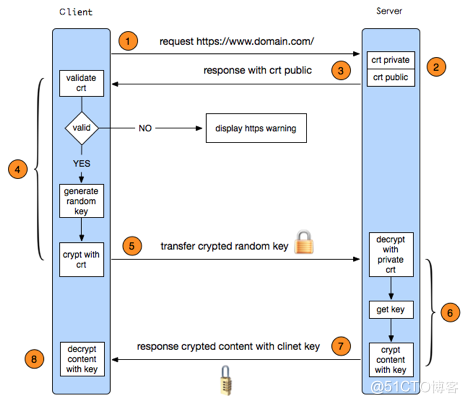
iOS network requests
4. Please describe and compare the following classes: URLSessionTask, URLSessionDataTask, URLSessionUploadTask, URLSessionDownloadTask
Keywords: #URLSession
URLSessionTask is an abstract class. By implementing it, any network transmission task can be instantiated, such as request, upload, and download tasks. Its suspend (cancel), resume (resume), and terminate (suspend) methods have default implementations
URLSessionDataTask is responsible for HTTP GET requests. It is a concrete implementation of URLSessionTask. It is generally used to obtain data from the server and store it in memory.
URLSessionUploadTask is responsible for HTTP Post/Put requests. It inherits URLSessionDataTask. Generally used for uploading data.
URLSessionDownloadTask is responsible for downloading data. It is a concrete implementation of URLSessionTask. It generally saves the downloaded data in a temporary file; after cancel, the data can be saved and the download can be continued later.
The relationship between them is as follows:
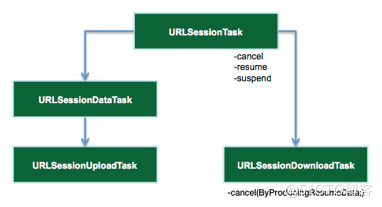
5. What is Completion Handler?
Keywords: #closure
Completion Handler is generally used to process the returned data after API request.
When the URLSessionTask ends, regardless of success or error, the Completion Handler generally accepts 3 parameters: Data, URLResponse, Error. Note that these 3 parameters are Optional.
In Swift, Completion Handlers must be marked with @escaping. Because it is always executed after the API request, that is to say, the Completion Handler will not be involved until the method has returned, which is a classic case of escaping closures.
6. Code combat: design a method, given the URL of the API, return user data
Keywords: #URLSessionDataTask
This question examines the basic usage of URLSessionDataTask. The following is the simplest and crudest way of writing:
func queryUser(url: String, completion: @escaping (_ user: User?, _ error : Error?) -> Void)) {
guard let url = URL(string: url) else {
return
}
URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error {
DispatchQueue.main.async {
completion(nil, error)
}
return
} else if let data = data, let response = response as? HTTPURLResponse {
DispatchQueue.main.async {
completion(convertDataToUser(data), nil)
}
} else {
DispatchQueue.main.async {
completion(nil, NSError(“invalid response”))
}
}
}.resume()
}
There are many problems with the above way of writing, the most important of which are:
Improper handling of url errors. An error message should be returned for future debugging, not return
Using URLSession's singleton is bad. It is a waste to create a dataTask for each request, and multiple requests in a short period of time will cause unnecessary pressure on the server. The correct way to deal with it should be to cancel the previous request for each request (whether it is completed or not).
Code duplication is redundant. The process of switching to the main thread and calling the closure is used many times in the code. We can actually extend the entire method into a class, and then use the return value in combination with member variables.
In addition to the above 3 points, we can further modify the code to enhance its readability and improve its logic. The modified code is as follows:
enum QueryError: String {
case InvaldURL = “Invalid URL”,
case InvalidResponse = “Invalid response”
}
class QueryService {
typealias QueryResult = (User?, String?) -> Void
var user: User?
var errorMessage: String?
let defaultSession = URLSession(configuration: .default)
var dataTask: URLSessionDataTask?
func queryUsers(url: String, completion: @escaping QueryResult) {
dataTask?.cancel()
guard let url = URL(string: url) else {
DispatchQueue.main.async {
completion(user, QueryError.InvalidURL)
}
return
}
dataTask = defaultSession.dataTask(with: url) { [weak self] data, response, error in
defer {
self?.dataTask = nil
}
if let error = error {
self?.errorMessage = error.localizedDescription
} else if let data = data,
let response = response as? HTTPURLResponse,
response.statusCode == 200 {
self?.user = convertDataToUser(data)
} else {
self?.errorMessage = QueryError.InvalidResponse
}
DispatchQueue.main.async {
completion(self?.user,self?.errorMessage)
}
}.resume()
}
}
The above modification method is mainly for some flaws. If the API is implemented with Swift's protocol-oriented programming, the entire code will be more flexible.
Information push
7. What is the process of local message notification in iOS development?
Keywords: #UserNotifications
The UserNotifications framework is Apple's framework for remote and local message notifications. The process is mainly divided into 4 steps:
1) Register. By calling the requestAuthorization method, the notification center will send a notification permission request to the user. Click Agree in the pop-up Alert to complete the registration.
2) Create. First set the information content UNMutableNotificationContent and the trigger mechanism UNNotificationTrigger; then use these two values to create UNNotificationRequest; finally add the request to the current notification center UNUserNotificationCenter.current().
3) Push. This step is the system or remote server push notification. Accompanied by a crisp sound (or customized sound), the process of displaying the corresponding UI to the mobile phone interface will be notified.
4) Response. When the user sees the notification, clicking it will have corresponding response options. The setting response options are UNNotificationAction and UNNotificationCategory.
Bonus answer:
The process of remote push is similar to that of local push, the difference is that in the second step of creation, the parameter content and message creation are all completed on the server side, not locally.
8. What is the principle of remote message push in iOS development?
Keywords: #APNs Server
The key to answering this question is to clarify the relationship between the iOS system, App, APNs server, and the client corresponding to the App. Specifically:
The app applies to the iOS system for remote message push permission. This is the same as the registration for local push notifications;
The iOS system requests the device token of the mobile phone from the APNs (Apple Push Notification Service) server, and tells the App to allow acceptance of push notifications;
The App transfers the device token of the mobile phone to the server corresponding to the App;
The remote message is generated by the server corresponding to the App, and it will first pass through APNs;
APNs pushes remote notifications to responding phones.
The specific flow chart is as follows:
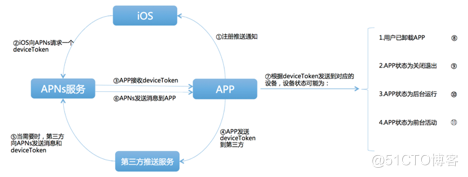
data processing
9. How to implement encoding and decoding in iOS development?
Keywords: #Encodable #Decodable
编码和解码在 Swift 4 中引入了 Encodable 和 Decodable 这两个协议,而 Codable 是 Encodable 和 Decodable 的合集。在 Swift 中,Enum,Struct,Class 都支持 Codable。一个最简单的使用如下:
enum Gender: String, Codable {
case Male = “Male”
case Female = “Female”
}
class User: Codable {
let name: String
let age: Int
let gender: Gender
init(name: String, age: Int, gender: Gender) {
(self.name, self.age, self.gender) = (name, age, gender)
}
}
这样定义完成之后,我们就可以轻易的在 User 及其对应 JSON 数据进行编码和解码,示范代码如下:
let userJsonString = """
{
"name": "Cook",
"age": 58,
"gender": "Male"
}
"""
// 从JSON解码到实例
if let userJSONData = userJsonString.data(using: .utf8) {
let userDecode = try? JSONDecoder().decode(User.self, from: userJSONData)
}
//从实例编码到JSON
let userEncode = User(name: "Cook", age: 58, gender: Gender.Male)
let userEncodedData = try? JSONEncoder().encode(userEncode)
追问:假如 JSON 的键值和对象的属性名不匹配该怎么办?
可以在对象中定义一个枚举(enum CodingKeys: String, CodingKey),然后将属性和 JSON 中的键值进行关联。
追问:假如 class 中某些属性不支持 Codable 该怎么办?
将支持 Codable 的属性抽离出来定义在父类中,然后在子类中配合枚举(enum CodingKeys),将不支持的 Codable 的属性单独处理。
10.谈谈 iOS 开发中数据持久化的方案
关键词: #plist #Preference #NSKeyedArchiver #CoreData
数据持久化就是将数据保存在硬盘中,这样无论是断网还是重启,我们都可以访问到之前保存的数据。iOS 开发中有以下几种方案:
plist。它是一个 XML 文件,会将某些固定类型的数据存放于其中,读写分别通过 contentsOfFile 和 writeToFile 来完成。一般用于保存 App 的基本参数。
Preference。它通过 UserDefaults 来完成 key-value 配对保存。如果需要立刻保存,需要调用 synchronize 方法。它会将相关数据保存在同一个 plist 文件下,同样是用于保存 App 的基本参数信息。
NSKeyedArchiver。遵循 NSCoding 协议的对象就就可以实现序列化。NSCoding 有两个必须要实现的方法,即父类的归档 initWithCoder 和解档 encodeWithCoder 方法。存储数据通过 NSKeyedArchiver 的工厂方法 archiveRootObject:toFile: 来实现;读取数据通过 NSKeyedUnarchiver 的工厂方法 unarchiveObjectwithFile:来实现。相比于前两者, NSKeyedArchiver 可以任意指定存储的位置和文件名。
CoreData。前面几种方法,都是覆盖存储。修改数据要读取整个文件,修改后再覆盖写入,十分不适合大量数据存储。CoreData 就是苹果官方推出的大规模数据持久化的方案。它的基本逻辑类似于 SQL 数据库,每个表为 Entity,然后我们可以添加、读取、修改、删除对象实例。它可以像 SQL 一样提供模糊搜索、过滤搜索、表关联等各种复杂操作。尽管功能强大,它的缺点是学习曲线高,操作复杂。
以上几种方法是 iOS 开发中最为常见的数据持久化方案。除了这些以外,针对大规模数据持久化,我们还可以用 SQLite3、FMDB、Realm 等方法。相比于 CoreData 和其他方案,Realm 以其简便的操作和丰富的功能广受很多开发者青睐。同时大公司诸如 Google 的 Firebase 也有离线数据库功能。其实没有最佳的方案,只有最合适的方案,应该根据实际开发的 App 来挑选合适的持久化方案。