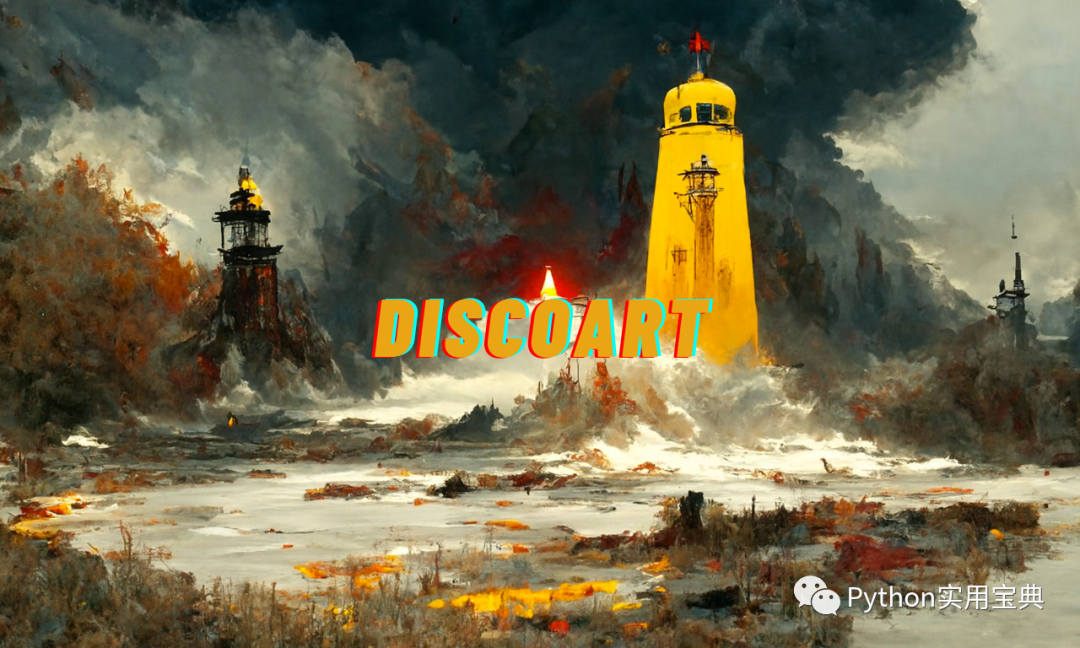
DiscoArt is a great open source module that can automatically paint according to the keywords you give.
The drawing process is fully visible, you can see the drawing process on the jupyter page:
1. Prepare
Before starting, you need to make sure that Python and pip have been successfully installed on your computer. If not, you can visit this article: Super detailed Python installation guide for installation.
(Optional 1) If you use Python for data analysis, you can install Anaconda directly: Anaconda, a good helper for Python data analysis and mining , has built-in Python and pip.
(Optional 2) In addition, it is recommended that you use the VSCode editor, which has many advantages: The best partner for Python programming—VSCode detailed guide .
Please choose one of the following ways to enter the command to install dependencies :
1. Open Cmd (Start-Run-CMD) in the Windows environment.
2. Open Terminal in the MacOS environment (command+space to enter Terminal).
3. If you are using VSCode editor or Pycharm, you can directly use the Terminal at the bottom of the interface.
pip install discoart
In order to run Discoart, you need Python 3.7+ and PyTorch with CUDA support.
2. Start using Discoart
You can run Discoart in Jupyter, which is convenient for showing the drawing process in real time:
from discoart import create
da = create()
This will create the image with the default text description and parameters:
Swipe up to see more codes
text_prompts:
- A beautiful painting of a singular lighthouse, shining its light across a tumultuous sea of blood by greg rutkowski and thomas kinkade, Trending on artstation.
- yellow color scheme
init_image:
width_height: [ 1280, 768 ]
skip_steps: 0
steps: 250
init_scale: 1000
clip_guidance_scale: 5000
tv_scale: 0
range_scale: 150
sat_scale: 0
cutn_batches: 4
diffusion_model: 512x512_diffusion_uncond_finetune_008100
use_secondary_model: True
diffusion_sampling_mode: ddim
perlin_init: False
perlin_mode: mixed
seed:
eta: 0.8
clamp_grad: True
clamp_max: 0.05
randomize_class: True
clip_denoised: False
rand_mag: 0.05
cut_overview: "[12]*400+[4]*600"
cut_innercut: "[4]*400+[12]*600"
cut_icgray_p: "[0.2]*400+[0]*600"
cut_ic_pow: 1.
save_rate: 20
gif_fps: 20
gif_size_ratio: 0.5
n_batches: 4
batch_size: 1
batch_name:
clip_models:
- ViT-B-32::openai
- ViT-B-16::openai
- RN50::openai
clip_models_schedules:
use_vertical_symmetry: False
use_horizontal_symmetry: False
transformation_percent: [0.09]
on_misspelled_token: ignore
diffusion_model_config:
cut_schedules_group:
name_docarray:
skip_event:
stop_event:
text_clip_on_cpu: False
truncate_overlength_prompt: False
image_output: True
visualize_cuts: False
display_rate: 1
This image is created:
All parameters supported by Create are as follows:
Swipe up to see more codes
text_prompts:
- A beautiful painting of a singular lighthouse, shining its light across a tumultuous sea of blood by greg rutkowski and thomas kinkade, Trending on artstation.
- yellow color scheme
init_image:
width_height: [ 1280, 768 ]
skip_steps: 0
steps: 250
init_scale: 1000
clip_guidance_scale: 5000
tv_scale: 0
range_scale: 150
sat_scale: 0
cutn_batches: 4
diffusion_model: 512x512_diffusion_uncond_finetune_008100
use_secondary_model: True
diffusion_sampling_mode: ddim
perlin_init: False
perlin_mode: mixed
seed:
eta: 0.8
clamp_grad: True
clamp_max: 0.05
randomize_class: True
clip_denoised: False
rand_mag: 0.05
cut_overview: "[12]*400+[4]*600"
cut_innercut: "[4]*400+[12]*600"
cut_icgray_p: "[0.2]*400+[0]*600"
cut_ic_pow: 1.
save_rate: 20
gif_fps: 20
gif_size_ratio: 0.5
n_batches: 4
batch_size: 1
batch_name:
clip_models:
- ViT-B-32::openai
- ViT-B-16::openai
- RN50::openai
clip_models_schedules:
use_vertical_symmetry: False
use_horizontal_symmetry: False
transformation_percent: [0.09]
on_misspelled_token: ignore
diffusion_model_config:
cut_schedules_group:
name_docarray:
skip_event:
stop_event:
text_clip_on_cpu: False
truncate_overlength_prompt: False
image_output: True
visualize_cuts: False
display_rate: 1
You can use parameters like this:
from discoart import create
da = create(
text_prompts='A painting of sea cliffs in a tumultuous storm, Trending on ArtStation.',
init_image='https://d2vyhzeko0lke5.cloudfront.net/2f4f6dfa5a05e078469ebe57e77b72f0.png',
skip_steps=100,
)
If you are not running with jupyter, you can also see the intermediate results, because both the final and intermediate results will be created in the current working directory, ie
./{name-docarray}/{i}-done.png
./{name-docarray}/{i}-step-{j}.png
./{name-docarray}/{i}-progress.png
./{name-docarray}/{i}-progress.gif
./{name-docarray}/da.protobuf.lz4
name-docarray
is a name defined at runtime, or randomly generated if not defined.i-*
The first batch.*-done-*
It is the final image after the current batch is completed.*-step-*
It is an intermediate image of a certain step, which is updated in real time.*-progress.png
are png images of all intermediate results so far, updated in real time.*-progress.gif
is an animated gif of all intermediate results so far, updated in real time.da.protobuf.lz4
is a compressed protobuf of all intermediate results so far, updated in real time.
3. Display/save/load configuration
If you want to know your current drawing configuration, there are three ways:
from discoart import show_config
show_config(da) # show the config of the first run
show_config(da[3]) # show the config of the fourth run
show_config(
'discoart-06030a0198843332edc554ffebfbf288'
) # show the config of the run with a known DocArray ID
To save the configuration of Document/DocumentArray:
from discoart import save_config
save_config(da, 'my.yml') # save the config of the first run
save_config(da[3], 'my.yml') # save the config of the fourth run
Import from configuration:
from discoart import create, load_config
config = load_config('my.yml')
create(**config)
In addition, you can directly export the configuration as an image
from discoart.config import save_config_svg
save_config_svg(da)
This is the end of our article. If you like today's Python practical tutorial, please continue to pay attention to Python Practical Collection.
If you have any questions, you can reply in the background of the official account: join the group , answer the corresponding red letter verification information , and enter the mutual assistance group to ask.
Originality is not easy, I hope you can give me a thumbs up below and watch to support me to continue creating, thank you!
Click below to read the original text for a better reading experience
Python Practical Collection (pythondict.com)
is not just a collection.
Welcome to pay attention to the official account: Python Practical Collection