1. OpenCV and OpenGL
OpenCV is Open Source Computer Vision Library, from image to data. OpenCV mainly provides basic algorithm libraries for image processing and video processing, and also involves some machine learning algorithms. For example, if you want to achieve video noise reduction, moving object tracking, and target (such as face) recognition, these are the fields of CV.
OpenGL is Open Graphics Library, from data to images. OpenGL focuses on Graphics, 3D graphics.
In fact, the difference between the two is the difference between the two disciplines of Computer Vision and Computer Graphics. The former focuses on obtaining information from the collected visual images and uses machines to understand images; the latter uses machines to draw appropriate visual images. for people to see.
2. Tutorial Notes
1. The process of creating a Windows window
Creating a window program mainly includes the following four processes:
(1) Registration window
(2) Monitor user operations, window message processing
(3) Create a window and display the window
(4) Ensure the duration of the window opening by looping
2. Code display
#include <windows.h>
//窗口消息处理函数
LRESULT CALLBACK GLWindowProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_CLOSE:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, msg, wParam, lParam); //系统默认消息处理函数
}
INT WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nShowCmd)
{
//注册窗口
WNDCLASSEX wndclass; //WNDCLASSEX结构用于注册窗口类
wndclass.cbClsExtra = 0; //指定紧跟在窗口类结构后的附加字节数。
wndclass.cbSize = sizeof(WNDCLASSEX); //WNDCLASSEX 的大小。我们可以用sizeof(WNDCLASSEX)来获得准确的值。
wndclass.cbWndExtra = 0; //指定紧跟在窗口实例的附加字节数
wndclass.hbrBackground = NULL; //背景画刷的句柄
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW); //光标的句柄
wndclass.hIcon = NULL; //图标的句柄
wndclass.hIconSm = NULL; //和窗口类关联的小图标。如果该值为NULL。则把hIcon中的图标转换成大小合适的小图标。
wndclass.hInstance = hInstance; //本模块的实例句柄
wndclass.lpfnWndProc = GLWindowProc; //窗口处理函数的指针
wndclass.lpszClassName = "GLWindow"; //指向类名称的指针
wndclass.lpszMenuName = NULL; //菜单名称
wndclass.style = CS_VREDRAW|CS_HREDRAW;//窗口重绘方式
ATOM atom = RegisterClassEx(&wndclass);
if (!atom)
{
MessageBox(NULL, "Register Fail", "Error", MB_OK);
return 0;
}
//窗口大小设置,使窗口实际大小为800*600
RECT rect;
rect.left = 0;
rect.right = 800;
rect.top = 0;
rect.bottom = 600;
AdjustWindowRect(&rect, WS_OVERLAPPEDWINDOW, NULL);
int windowWidth = rect.right - rect.left;
int windowHeight = rect.bottom - rect.top;
//创建窗口
HWND hwnd = CreateWindowEx(NULL, "GLWindow", "OpenGL Window", WS_OVERLAPPEDWINDOW,
100, 100, 800, 600,
NULL, NULL, hInstance, NULL);
//显示窗口
ShowWindow(hwnd, SW_SHOW);
UpdateWindow(hwnd);
//防止窗口一闪而过
MSG msg;
while (true)
{
if (PeekMessage(&msg, NULL, NULL, NULL, PM_REMOVE))
{
if (msg.message == WM_QUIT)
{
break;
}
TranslateMessage(&msg);
DispatchMessage(&msg);
}
}
return 0;
}
3. Solve problems
The following error is reported when the project is running, because the project is a console application when we create a new project, not a window application.

error message
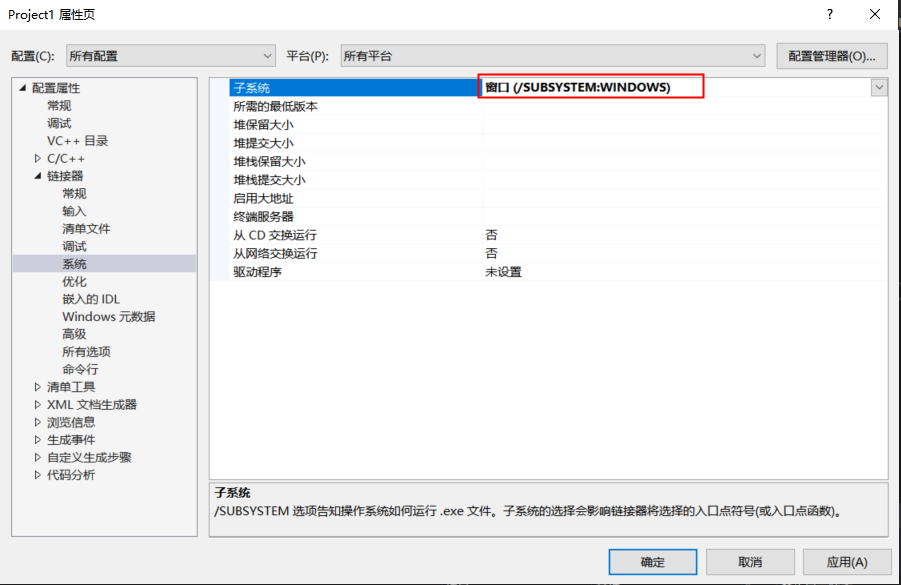
Solution