java design pattern type
Creational patterns: separate object creation from use
Structural pattern: how to compose classes and objects into a larger pattern according to a certain layout
Behavioral patterns: used to describe how classes or objects cooperate with each other to accomplish tasks that no single object can accomplish alone
Introduction to 23 Design Patterns
1. Singleton (Singleton) mode : a class can only generate one instance, which provides a global
An access point for external access to the instance, an extension of which is the finite-many-instance pattern.
2. Prototype mode : use an object as a prototype and clone it by copying it
Generate multiple new instances similar to the prototype.
3. Factory Method (Factory Method) mode : define an interface for creating products, by child
Classes determine what products are produced.
4. Abstract Factory (AbstractFactory) mode : Provide an interface to create a product family, each of which
Subclasses can produce a series of related products.
5. Builder (Builder) mode : decompose a complex object into multiple relatively simple parts, and then
Then create them separately according to different needs, and finally build this complex object.
6. Proxy mode : Provide a proxy for an object to control access to the object. Instant customer
The client indirectly accesses the object through the proxy, thereby restricting, enhancing or modifying some characteristics of the object.
7. Adapter (Adapter) mode : convert the interface of a class into another interface that the customer wants,
Enables classes that would otherwise not work together due to incompatible interfaces to work together.
8. Bridge mode : Separate abstraction from implementation so that they can vary independently. it is used group
It can be implemented by combining relationship instead of inheritance relationship, thus reducing the coupling degree of the two variable dimensions of abstraction and implementation.
9. Decorator mode : Dynamically add some responsibilities to the object, that is, add its additional functions
able.
10. Facade mode : Provide a consistent interface for multiple complex subsystems, making these subsystems
The system is easier to access.
11. Flyweight mode : use sharing technology to effectively support the complex of a large number of fine-grained objects
use.
12. Composite mode : Combine objects into a tree-like hierarchy, allowing users to
Consistent access with composite objects.
13. Template Method (TemplateMethod) mode : define the algorithm skeleton in an operation, and will calculate
Some steps of the method are deferred to subclasses, so that subclasses can be redefined without changing the structure of the algorithm
define some specific steps of the algorithm.
14. Strategy (Strategy) mode : defines a series of algorithms and encapsulates each algorithm so that it
They can be replaced with each other, and the change of the algorithm will not affect the customers who use the algorithm.
15. Command (Command) mode : encapsulate a request as an object, so that the responsibility for making the request
Separated from the responsibility for executing the request.
16. Chain of Responsibility (Chain of Responsibility) mode : pass the request from one object in the chain to the next
An object until the request is responded to. In this way the coupling between objects is removed.
17. State (State) mode : allows an object to change its behavior when its internal state changes
force.
18. Observer (Observer) mode : there is a one-to-many relationship between multiple objects, when an object changes
When the time changes, the change is notified to other multiple objects, thereby affecting the behavior of other objects.
19. Mediator mode : define an intermediary object to simplify the interaction between original objects
Relationship, reduce the coupling degree between objects in the system, so that the original objects do not need to understand each other.
20. Iterator mode : Provide a method to sequentially access a series of numbers in the aggregate object
data without exposing the internal representation of the aggregate object.
21. Visitor (Visitor) mode : without changing the elements of the collection, for each
Elements provide multiple access methods, that is, each element can be accessed by multiple visitor objects.
22. Memento mode : Acquire and save a pair without destroying encapsulation
internal state of the object so that it can be restored later.
23. Interpreter (Interpreter) mode : Provides how to define the grammar of the language, and the language sentence
Interpreter method, the interpreter
Commonly used design patterns:
Singleton mode :
Definition: In some systems, in order to save memory resources and ensure the consistency of data content, an instance is created for everyone to share, for example: Runtime class
Features :
Singleton has only one instance object
The singleton object must be created by the singleton class itself
Singleton; the class provides a public method to access
hungry man mode
Here, take window as an example to give you a demonstration
public class Window {
/*饿汉式单例:在类加载的时候的初始化实例(static)
* 因为创建的实例只有一个,所以不存在线程安全问题*/
private static Window window=new Window();
public Window getWindow(){
return window;
}
}
public class Test {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
new Thread(()->{
System.out.println(new Window().getWindow());//获得是同一个对象
}).start();
}
}
}
lazy mode
public class Window {
/*懒汉式单例:
* 在类加载的时候,不创建对象,在使用时进行创建
* 这时,生成对象的数量就需要我们自己来进行控制
* 但是懒汉式单例会出现线程安全问题:在多线程访问时,可能会有多个线程同时进行到if中,就会创建出多个对象
* 在并发的时候,会出现指令重排的情况,所以用volatile防止指令重排,避免造成错误*/
private static volatile Window window;
public Window getWindow(){
try {
//增加休眠增加并发的几率
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
//1.0版本给方法上锁,虽然可行,但是效率太低,每次只能运行一个线程
// if(windows==null){ 1.1版本 进入if之后上锁,这种上锁起不到多个线程公用一个对象实例的作用,一个执行完之后,另外一个线程进入重新创建一个实例对象
// synchronized (Windows.class){
// window=new Window()}
//}
//最终版本,双重判断,公用一个对象实例
if(window==null){
synchronized (Window.class){
if (window==null){
window=new Window();
}
}
}
return window;
}
}
public class Test {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
new Thread(()->{
System.out.println(new Window().getWindow());
}).start();
}
}
}
Factory pattern :
Simple factory : suitable for fewer product subcategories and does not conform to the principle of opening and closing
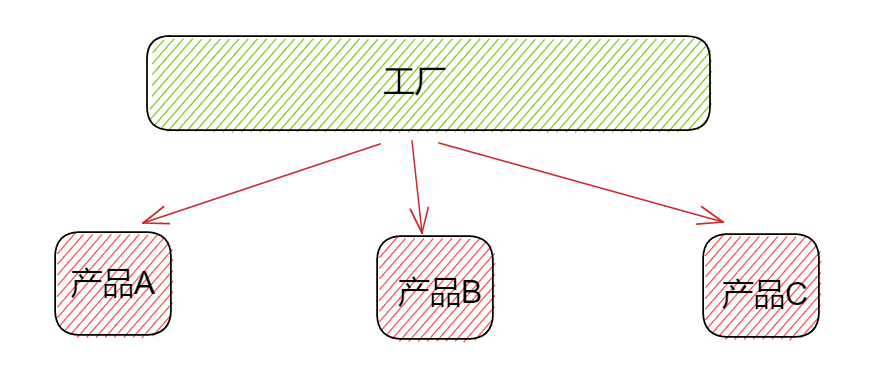
Roles and their responsibilities included in the pattern :
Factory : Responsible for logical operations and creating the required products
Abstract product : describe the interface or abstract class shared by all instances
Concrete product abstraction : is the goal of creating a simple factory
Code:
//Car接口
public interface Car {
void run();
}
//CarFactory工厂类
public class CarFactory{
public static Car createCar(String name){
if(name.equals("aodi")){
return new Aodi();
}
if(name.equals("bmw")){
return new Bmw();
}
return null;
}
}
//实现类
public class Aodi implements Car{
@Override
public void run() {
System.out.println("奥迪汽车行驶");
}
}
//实现类
public class Bmw implements Car{
@Override
public void run() {
System.out.println("宝马汽车行驶");
}
}
//客户端
public class Test {
public static void main(String[] args) {
//每次增加子类类型的时候,需要对代码进行修改,不符合开闭原则,适用于子类较少或者是不容易修改的项目中
//优点:客户端不负责对象的创建,由专门的工厂进行创建,客户端直需要对对象进行调用,实现创建和调用的分离,降低了代码的耦合度
//缺点:如果增加或减少子类,需要对代码进行修改,违背了设计原则中的开闭原则,如果子类过多,会导致工厂非常庞大,违背了高内聚原则,不利于后期的维护
Car bmw = CarFactory.createCar("bmw");
Car aodi = CarFactory.createCar("aodi");
bmw.run();
aodi.run();
}
}
Factory way pattern :
The factory is also abstracted, deferring the actual creation work to the subclass to complete
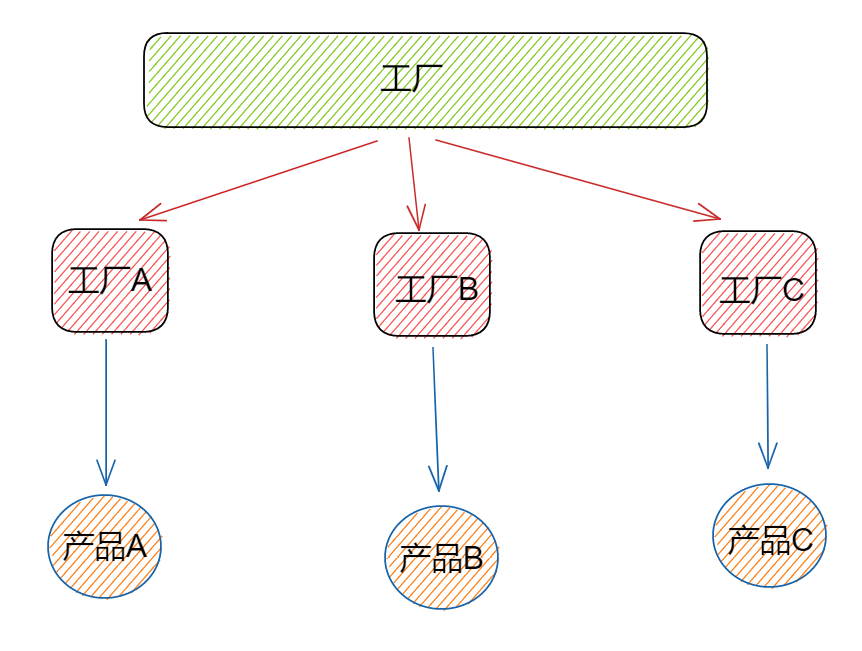
Advantages: The client is not responsible for the creation of the object, but is completed by a special factory class. The client is only responsible for the invocation of the object, which realizes the separation of creation and invocation, and reduces the difficulty of client code. There is no need to modify the factory, it is necessary to add product subcategories and factory subcategories, which conforms to the principle of opening and closing . Even if there are too many product subcategories, it will not lead to a huge factory category, which is conducive to later maintenance
Disadvantages : some extra code is required, which increases the workload
Abstract factory pattern :
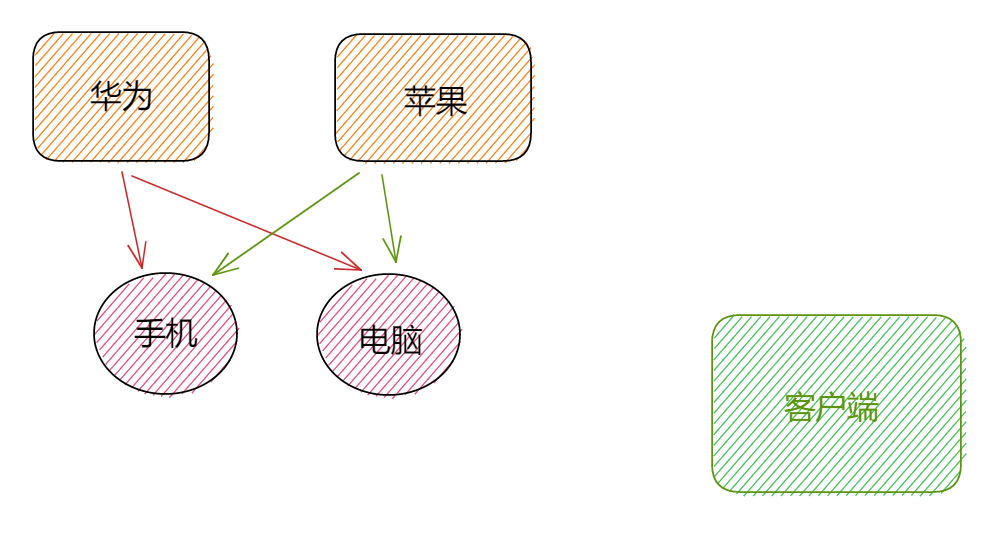
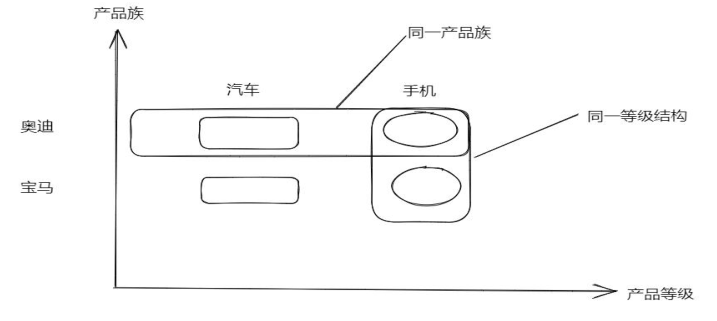
Putting items of the same brand together for production is like you can buy Lenovo mobile phones and Lenovo computers in a large production factory, instead of producing them separately, resulting in waste of resources
Advantages : To obtain a specific series of products, you only need to obtain them through a specific series of factories, and you don't care about the details of creation
Prototype mode:
In the project, we need many instances, but creating instance objects is very troublesome, so it is not a wise choice to directly create objects, so we often use clone when we need to create the same object multiple times , in order to increase the creation speed
Proxy mode:
The proxy mode is to provide a proxy for an object, and the proxy object controls the reference to the original object, so that the client cannot directly communicate with the real target object. The proxy object is the representative of the object, and the client operates on the actual object through the proxy object.
static proxy
Features : The proxy class implements an interface, and any object that implements the interface can be proxied through the proxy class, which increases the versatility
Advantages : It is possible to extend the function of the target object in accordance with the principle of opening and closing
缺点:代码量大,必须要有接口,再有代理
//Computer对象类
public class Compurter implements Huawei{
@Override
public void run() {
System.out.println("华为电脑跑步机器");
}
@Override
public void play() {
System.out.println("华为电脑在玩");
}
}
//customer客户端
public class customer {
public static void main(String[] args) {
Phone phone=new Phone();
Compurter compurter=new Compurter();
Proxy proxy1=new Proxy(phone);
Proxy proxy=new Proxy(compurter);
proxy.play();
proxy1.run();
}
}
//Huawei接口
public interface Huawei {
public void run();
public void play();
}
//Phone对象类
public class Phone implements Huawei{
@Override
public void run() {
System.out.println("华为手机在跑步");
}
@Override
public void play() {
System.out.println("华为手机在玩");
}
}
//Proxy代理对象
public class Proxy implements Huawei {
Huawei huawei;
public Proxy(Huawei huawei){
this.huawei=huawei;
}
@Override
public void run() {
try {
System.out.println("你是大坏蛋");
} catch (Exception e) {
} finally {
System.out.println("终于完了");
}
}
@Override
public void play() {
huawei.play();
}
}
动态代理
通过动态代理我们就不需要手动的创建代理类了,只需要编写一个动态处理就可以了,代理对象通过动态处理
jdk动态代理:
public class JdkProxy implements InvocationHandler {
Object object;//真实对象,接收任何的目标类对象
public JdkProxy(Object object){
this.object=object;
}
/* 在动态代理类中调用目标类中的具体方法,动态的将代理对象对象
* 目标类中要调用方法,以及方法中的参数传递过来
* Method method 动态获取的真正要执行的方法*/
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
method.invoke(object);
return proxy;
}
//真正意义上的运行时生成代理对象
public Object getProxy(){
return Proxy.newProxyInstance(object.getClass().getClassLoader(), object.getClass().getInterfaces(),this);
}
}
cg动态代理:
/*
* 动态代理类
*/
public class CGLibProxy implements MethodInterceptor {
private Enhancer enhancer = new Enhancer();
public Object getProxy(Class<?> clazz){
enhancer.setSuperclass(clazz);
enhancer.setCallback(this);
return enhancer.create();
}
/*
* 拦截所有目标类方法的调用
* 参数:
* obj 目标实例对象
* method 目标方法的反射对象
* args 方法的参数
* proxy 代理类的实例
*/
public Object intercept(Object obj, Method method, Object[] args,
MethodProxy proxy) throws Throwable {
//代理类调用父类的方法
System.out.println("开始事务");
Object obj1 = proxy.invokeSuper(obj, args);
System.out.println("关闭事务");
return obj1;
}
}