In Pygame, circles can be drawn through the circle() function under the draw module.
1 Completion of preparations
Before drawing a circle, you need to import the Pygame module, initialize the Pygame module, and create a Surface object.
import pygame
from pygame.locals import *
pygame.init()
screen = pygame.display.set_mode((600,500))
Among them, screen is the created Surface object.
2 Realization of circle drawing
2.1 usage of circle() function
The circle() function has two usages, one is the basic usage and the other is the extended usage.
2.1.1 Basic usage
The format of the basic usage of the circle() function is
circle(surface, color, center, radius)
Among them, the parameter surface refers to the Surface object to draw a circle; color specifies the color of the circle (the parameter width determines whether the color is the color of the line drawing the circle or the fill color), and the type of the parameter is the Color class or the color Tuple; center specifies the position of the center of the circle, and its type is a Vector2 class or a tuple or list representing the position; radius indicates the radius of the circle, and the type of this parameter is an integer or floating point type. If the value of this parameter is less than 1, Then the action of drawing a circle is not performed. The return value of the circle() function is a variable of type Rect, indicating the range of the drawn circle.
2.1.2 Extended Usage
The format of the extended usage of the circle() function is
circle(surface, color, center, radius, width=0, draw_top_right=None, draw_top_left=None, draw_bottom_left=None, draw_bottom_right=None)
The parameters surface, color, center and radius are used in the same way as the basic type; width indicates the thickness of the line for drawing a circle. When the value of width is 0, the parameter color indicates the fill color of the circle. When the value of width is greater than 0, the parameter color indicates the color of the line drawing the circle, and width indicates the thickness of the line. When the value of width is less than 0, the color and width parameters are ignored; the next four parameters specify whether to draw only the upper right, upper left, lower left and lower right of the circle respectively. Four parts, True means only draw the specified part.
2.2 Use of circle() function
2.2.1 Use of basic type
The basic code of the circle() function is as follows:
color = 255,0,0
center = 300,200
radius = 50
pygame.draw.circle(screen, color, center, radius)
pygame.display.update()
Among them, the color, center and radius variables respectively specify the color, center position and radius of the circle to be drawn; screen is the Surface object created in "1 Completion of preparations". The function of the pygame.display.update() function is to display the drawn circle on the screen. The running effect of the code is shown in Figure 1.
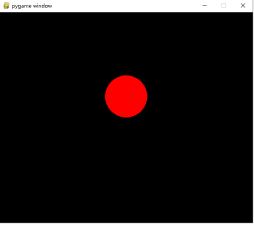
Figure 1 Basic circle drawing
2.2.2 Use of Extended Type
The code for the scalability of the circle() function is as follows:
pygame.draw.circle(screen, color, center, radius,0,True, False,True,False)
Among them, the value of the parameter width is 0; the values of draw_top_right and draw_bottom_left are True; the values of draw_top_left and draw_bottom_right are False, and the drawn effect is shown in Figure 2.
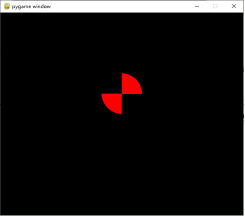
Figure 2 Extended circle drawing