Author: JD Retail Du Xingwen
Let's talk about the concept first. Web front-end automated testing is a method of automating the testing tasks of web applications by writing code. It is usually implemented using JavaScript and testing frameworks (such as Selenium, Appium, etc.).
The advantages of web front-end automated testing are that it can improve test efficiency, reduce test time and test cost, and ensure test quality. Here are some advantages of web front-end automated testing:
In practical applications, web front-end automated testing is usually used to test the interactive functions, performance, security and other aspects of web applications. For example, automated testing tools can be used to test web application functions such as login, registration, navigation, and form validation, or automated testing tools can be used to test web application performance, such as response speed, page load time, etc.
In short, web front-end automated testing is a method that can improve testing efficiency, reduce testing costs, and improve testing quality, and is suitable for various types of web applications.
This article talks about the solutions and thinking of front-end automated testing from entry to proficiency to expert level! Divided into the following regardless:
1. First, build a Selenium automated test case
The sample test requirement is very simple: visit the Baidu homepage, search for a certain keyword, and verify that the title of the search result page is "searched keyword" + "_ Baidu search". If the search keyword is "ChatGPT", then the title of the search results page should be "ChatGPT_ Baidu Search".
After understanding the test requirements, I strongly recommend that you perform the test manually first. The specific steps are: open the Chrome browser, enter Baidu's website "www.baidu.com"; enter the keyword "ChatGPT" in the search input box and press Enter; verify that the title of the search results page is "ChatGPT_百度搜索".
After clarifying the specific steps of GUI testing, we can use Java code to implement this test case based on Selenium. Here, I want to use the Chrome browser, so I need to download the Chrome Driver first and put it into the environment variable. Next, you can create an empty Maven project in a familiar way, and then add Selenium 2.0 dependencies in the POM file, as shown in Figure 1.
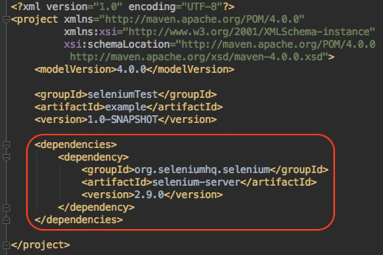
Figure 1 Adding Selenium 2.0 dependencies to the POM file
Then create a main method in Java, and copy the code shown in Figure 2 into your main method. Below is the sample code for Selenium based automated test case
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class BaiduSearch {
public static void main(String[] args) {
// 设置驱动路径
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
// 启动浏览器
WebDriver driver = new ChromeDriver();
// 访问百度首页
driver.get("https://www.baidu.com");
//获取百度搜索输入框元素,并自动写入搜索内容
driver.findElement(By.id("kw")).sendKeys("ChatGPT");
//线程睡眠1秒
Thread.sleep(1000);
//获取“百度一下”元素,并自动点击
driver.findElement(By.id("su")).click();
//线程睡眠3秒
Thread.sleep(3000);
Assert.assertEquals("ChatGPT _ 百度搜索",driver.getTitle());
//退出浏览器
driver.quit();
}
The above is the most simple and direct GUI automation test case established from 0 to 1. The implementation of this use case is very simple, but you can only really use it well if you really understand the principles of Selenium tools.
Second, after getting started, we have to show our talents in the efficiency of testing responsibilities, that is, the decoupling of scripts and data + Page Object model.
The essence of "test script and data decoupling" is to implement data-driven testing, so that tests with the same operation but different data can be implemented through the same set of automated test scripts, but different test input data is provided each time the test is executed.
In the test script, read a line of data from the CSV file through the data provider, assign it to the corresponding variable, and execute the test case. Then go to the CSV file to read the next line of data. After reading all the data, the test ends. There are several rows of data in the CSV file, and the test case will be executed several times. The specific process is shown in the figure below.
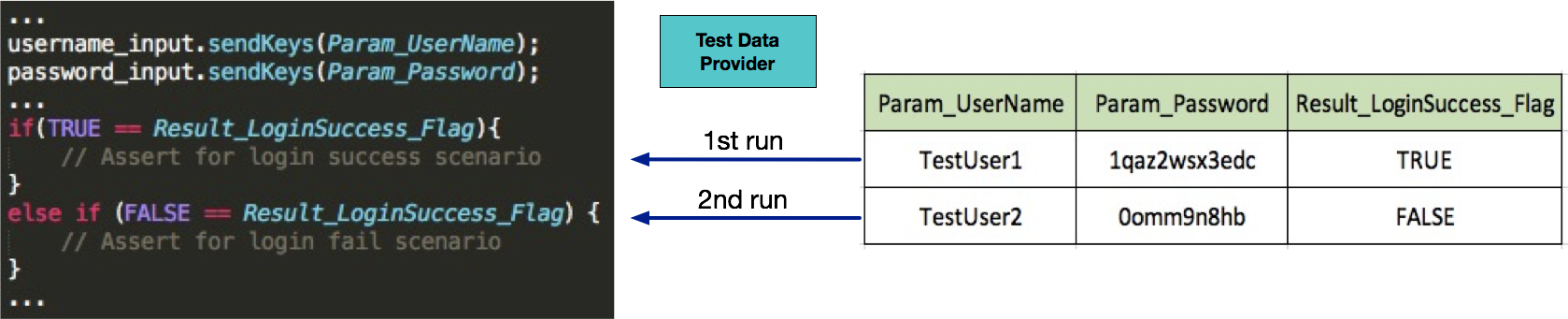
The core idea of the "page object model" is to encapsulate the controls on the page and some operations of the controls in units of pages. And the test case uses the page object to complete the specific interface operation.
The core idea of the page object model is to encapsulate the controls on the page and some operations of the controls in units of pages (Web Page or Native App Page). The test case, more precisely, the operation function, completes the specific interface operation based on the page package object, the most typical mode is "XXXPage.YYYComponent.ZZZOperation".
Based on this idea, the pseudocode of the above use case can be evolved into the structure shown in the figure below. The pseudocode of the login function is given here. It is recommended that you implement the code of search and logout by yourself according to this idea, so that you can better understand the changes brought about by the page object model.
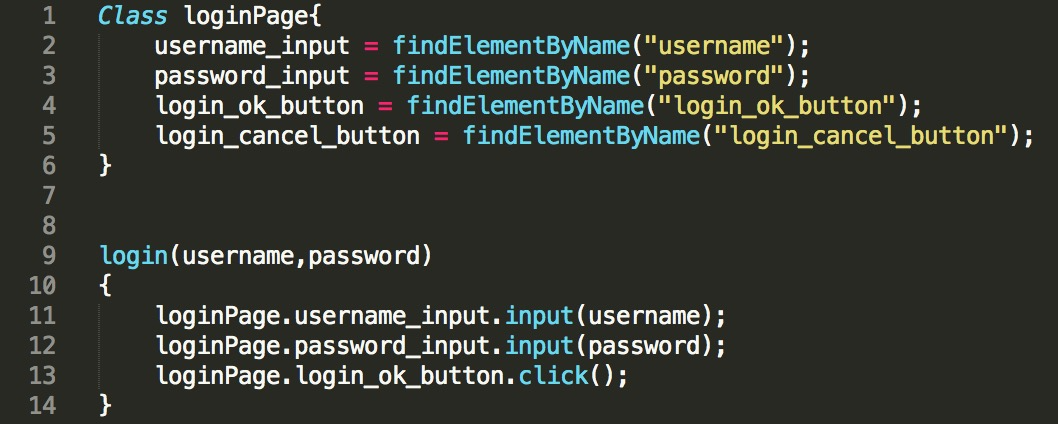
3. Let automated test scripts better describe the business
Business process abstraction is a higher-level abstraction method based on operation functions that is closer to the actual business. Test cases based on business process abstraction are often very flexible, and you can easily assemble various test cases.
Assume that a specific business process is: registered users log in to the e-commerce platform to purchase specified books. Then, the pseudo code of the test case based on business process abstraction is shown in the figure below.
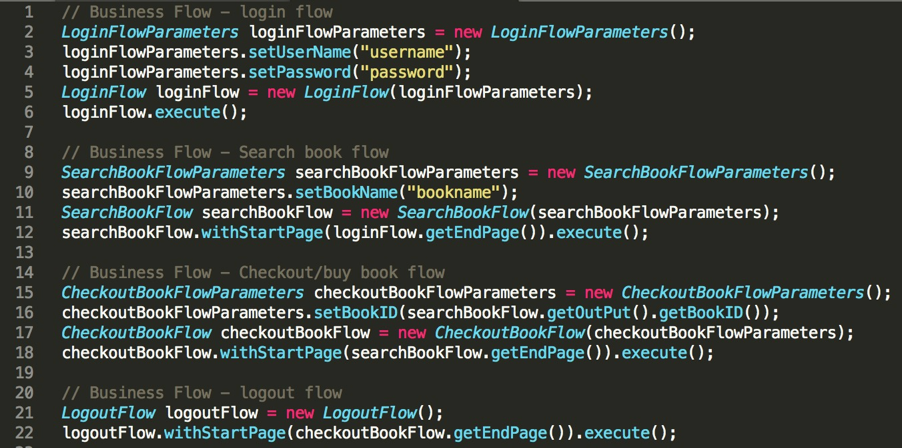
This pseudo-code contains a lot of information, but if you understand the design idea of this code, you will grasp the essence of business process abstraction.
From the perspective of the overall structure, the pseudocode calls four business processes in sequence, which are LoginFlow for user login, SearchBookFlow for book query, CheckoutBookFlow for book purchase, and LogoutFlow for user logout.
4. Test data of front-end GUI automation test
Test data for GUI automation testing refers to test data used to test the user interface (GUI) of an application. In automated testing, test data is usually obtained from test datasets, which contain different inputs and outputs of the application.
Here are some common GUI automation test data:
In GUI automation testing, the construction of the test data set is very important for the success of the test. The test dataset should cover as much as possible the different inputs and outputs of the application in order to identify potential problems and defects during testing.
Traditionally, data quality has been divided into six dimensions.
• Accuracy: To what extent does a piece of information reflect reality?
• Completeness: Does it meet your expectations for comprehensiveness?
• Coherence: Is the information stored in one place consistent with related data stored elsewhere?
• Timeliness: Is your information available when you need it?
• Availability: Is the information in a specific format, type or size? Does it follow business rules/best practices?
•Completeness: Can the disparate data sets be properly connected to reflect a larger picture? Are the relationships well defined and enforced?
These dimensions are defined when taking a broad view of designing a data warehouse. Consideration is given to all defined and collected data sets, their relationships, and their ability to properly serve the organization.
5. Key technologies to improve the stability of GUI automated testing
Theoretical points for improving the stability of GUI automated testing include the following:
The key technical points to improve the stability of GUI automated testing include the following points:
1. Basic HTML/CSS/JS skills: For a web front-end automation test engineer, basic HTML/CSS/JS skills are essential, which can help him better understand the page interaction and rendering mechanism.
2. Tool chain technology: For web front-end automated testing, tool chain technology is a necessary skill, such as Grunt and Gulp.
3. Language skills: Web automated testing requires the use of multiple programming languages, such as Java, Python, JavaScript, etc., and the ability to develop these languages is essential.
4. Basic testing technology: Web front-end automated testing engineers need to be familiar with the basic concepts and methods of testing, such as test plans, test cases, and test strategies.
5. API and interface testing: Web front-end automated testing engineers need to be familiar with how to test APIs and interfaces, which is very important to ensure the accuracy of application functions.
6. Automated testing framework technology: Web front-end automated testing engineers need to master at least one automated testing framework technology, such as Selenium, WebdriverIO, etc.
7. Debugging skills: Web front-end automation test engineers need to be proficient in using debugging skills to solve problems during the testing process, such as using Fiddler, Chrome developer tools, etc.
8. Database technology: Web front-end automation test engineers need to be familiar with basic database operations and SQL statements in order to perform data verification and data comparison during testing.
9. Script writing skills: By writing scripts such as JavaScript and Python, it can help testers realize automated testing and quickly generate test reports.
10. Efficient testing methods: Web front-end automated testing engineers need to be proficient in various testing methods and techniques in order to complete testing tasks more efficiently and comprehensively in their work.
In short, improving the stability of GUI automated testing requires comprehensive consideration of multiple factors such as test framework, test cases, test data, test environment, performance testing, and test maintenance, and through continuous optimization and upgrading, test efficiency and quality can be improved. It can be started from the following 5 aspects:
1. For the instability caused by unexpected pop-up dialog boxes, "abnormal scene recovery mode" can be introduced to solve it.
2. For instability caused by slight changes in page control properties, you can use "combined properties" to locate controls, and you can use "fuzzy matching technology" to improve the positioning recognition rate.
3. For the instability caused by A/B testing, it is necessary to do branch processing in the test case script, and the script needs to correctly identify different branches.
4. For instability caused by random page delays, a retry mechanism can be introduced. Retry can be at the step level, page level, or even business process level.
5. For the instability caused by the test data, I will not expand it in detail here, and leave it for a special introduction in the follow-up series of test data preparation articles.
6. Elegant automated test report
The early video-based GUI test report is not the best solution due to its large size and the inability to easily adapt to the log. The ideal GUI test report should be composed of a series of screenshots in chronological order, and the elements you operate can be highlighted on these screenshots, and a detailed description of the relevant operation steps is provided according to the execution sequence.
The GUI test reports of commercial GUI automation testing frameworks are already very mature, and usually do not require additional customization or development.
However, the GUI test report of the open source GUI automation test framework often needs to be developed by itself, which is mainly realized by extending the original operation function of Selenium and the Hook function.
Implementation Ideas of Test Report of Open Source GUI Test Framework
However, if you are using open source software, such as Selenium WebDriver, you need to implement the screenshot and highlight operation element functions yourself. The idea of implementation is usually: use the screenshot function of Selenium WebDriver to complete the interface screenshot function at some specific timing (for example, when the page jumps, when a certain control is operated on the page, or when the test fails, etc.).
Specific to the code implementation, there are usually two ways: 1. Extend the original operation function of Selenium; 2. Call the screenshot function in the related Hook operation.
First, extend the original operation function of Selenium to realize the function of taking screenshots and highlighting operation elements
Since Selenium's native click operation function does not have the function of taking screenshots and highlighting operation elements, let's implement a click function of our own. When the click function implemented by yourself is called:
First, use Javascript code to highlight the manipulated element. The highlighting method is to use JavaScript to render a 5-8 pixel edge on the border of the object;
Then, call the screenshot function to complete the screenshot before clicking;
Finally, call the native click function of Selenium to complete the real click operation.
Then, whenever you need to call the click function in the future, you will directly call this self-encapsulated click function, and directly get a screenshot of the interface with the object being operated highlighted.
Second, call the screenshot function in the relevant Hook operation to realize the functions of taking screenshots and highlighting operation elements
In fact, the method of using Hook is relatively simple and intuitive, but you must first understand what is Hook.
Hook means "hook" in Chinese. It is a bit difficult to understand what a "hook" is directly through the definition, so I will explain it to you through an example. When executing a certain function F, the system will implicitly execute an empty implemented function before executing function F, then when you need to do some extension or interception, you can add custom operations to this empty implemented function up. Then this empty implemented function is the so-called Hook function.
The third is the innovative design of the global GUI test report
The so-called globalization test means that the same business has its own website in every country in the world. For example, some large global e-commerce companies have their own sites in many countries, so the testing of these sites should not only focus on the basic functions and the unique functions of each country, but also verify the interface layout and translation in the context Is it appropriate.
Early on, local test engineers were hired to manually perform the main business scenario tests and verify that the relevant page layouts and translations fit in context. These test engineers specially hired locally are called LQA.
Obviously, the efficiency of hiring LQA is very low. The main reason is that all testing work is performed manually by LQA in the later stage of the project, and they need to be trained in business before execution; at the same time, we need to prepare very detailed test case documents, and LQA also needs to Put a lot of effort into taking screenshots and completing the final test report. In order to solve this inefficient mode, the best solution is to use GUI automated testing tools to generate screenshots of the complete test execution process.
In this way, LQA no longer needs to manually execute test cases, but directly analyzes the screenshots of the GUI interface during the business operation process in the test report, and then finds page layout problems or inappropriate translation problems.
This solution seems to be perfect, and the focus of LQA's work is clearer, but this is not the optimal solution. Because of these LQAs in actual work, there will be the following three more painful places:
It is necessary to frequently switch back and forth between test reports in multiple countries to compare page layouts;
It is necessary to frequently switch to the report of the US website (that is, the main website) to compare the matching degree between the translated content and the context;
After a defect is found, it is still necessary to copy the screenshot from the GUI test report and mark the problematic points with image software before opening the defect management system to submit the defect report.