source code at the bottom
Primer
Now ChatGPT is very popular, but the registered account must have a foreign mobile phone number, which stumps many people.
So many people encapsulated the ChatGPT API by themselves, made a simple forwarding, and got the answer to the question they wanted to ask by calling the ChatGPT API.
However, it seems that the answers obtained through the API are not as detailed as those obtained directly from the web page, and the reason for this is unknown.
Now such applications and websites are everywhere, but as a programmer with aspirations, how can you not implement it yourself?
Let's make the simplest page below, which only realizes the function of asking and answering:
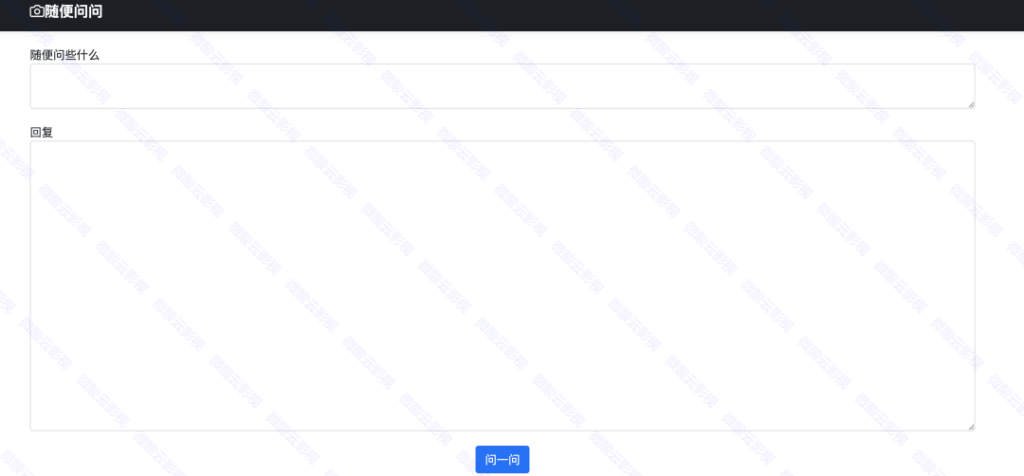
account and secret key
OK, here we go.
First of all, we need a ChatGPT account. There are many ways to register an account on the Internet. You can find it yourself, or you can directly buy a ready-made one.
After registering, click View API keys in the upper right corner, and then generate a secret key. This is the private key for calling the API. Be sure to keep it safe and not disclose it to others.
Take a look at the documentation, which contains API requests and returned data formats:
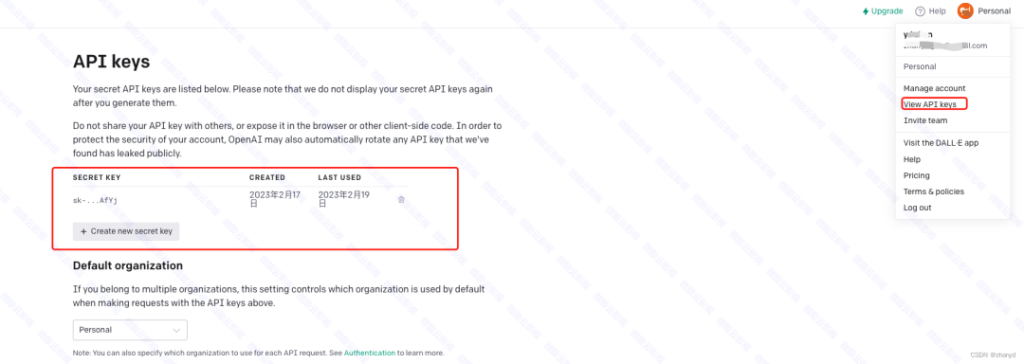
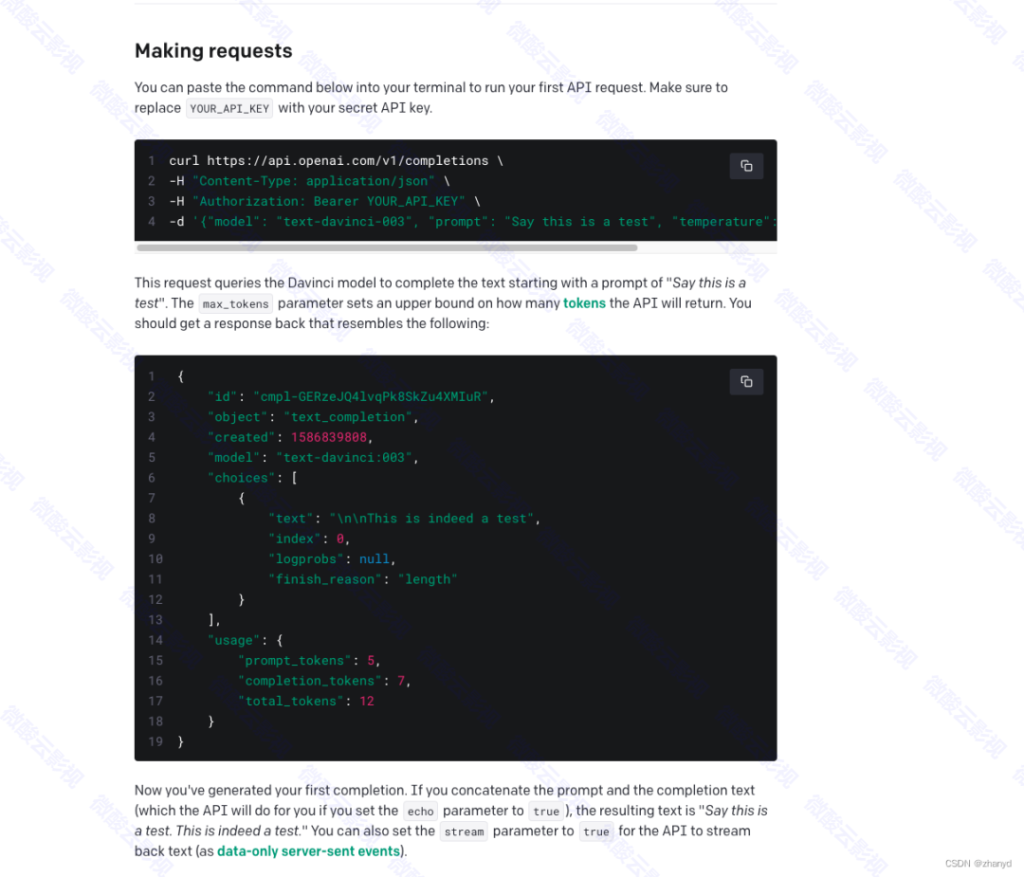
rear end
We start writing code, nothing more than calling an API interface, we can use any language, here I will use Java.
It is mainly the following code, and other codes are calls to this code. It is worth noting that it can only be called by post, not by get.
/**
* 调用ChatGPTAPI
* @param question
* @return
*/
public static String postChatGPT(String question) {
StringBuffer receive = new StringBuffer();
BufferedWriter dos = null;
BufferedReader rd = null;
HttpURLConnection URLConn = null;
try {
// API的地址
URL url = new URL("https://api.openai.com/v1/completions");
URLConn = (HttpURLConnection) url.openConnection();
URLConn.setReadTimeout(readTimeout);
URLConn.setConnectTimeout(connectTimeout);
URLConn.setDoOutput(true);
URLConn.setDoInput(true);
URLConn.setRequestMethod("POST");
URLConn.setUseCaches(false);
URLConn.setAllowUserInteraction(true);
URLConn.setInstanceFollowRedirects(true);
URLConn.setRequestProperty("Content-Type", "application/json");
// 这里填秘钥,最前面得加"Bearer "
URLConn.setRequestProperty("Authorization", "Bearer sk********");
JSONObject sendParam = new JSONObject();
// 语言模型
sendParam.put("model", "text-davinci-003");
// 要问的问题
sendParam.put("prompt", question);
// 温度,即随机性,0表示随机性最低,2表示随机性最高
sendParam.put("temperature", 0.8);
// 返回最大的字数
sendParam.put("max_tokens", 2048);
URLConn.setRequestProperty("Content-Length", String.valueOf(sendParam.toString().getBytes().length));
dos = new BufferedWriter(new OutputStreamWriter(URLConn.getOutputStream(), "UTF-8"));
dos.write(sendParam.toString());
dos.flush();
rd = new BufferedReader(new InputStreamReader(URLConn.getInputStream(), "UTF-8"));
String line;
while ((line = rd.readLine()) != null) {
receive.append(line);
}
} catch (IOException e) {
receive.append("访问产生了异常-->").append(e.getMessage());
e.printStackTrace();
} finally {
if (dos != null) {
try {
dos.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
if (rd != null) {
try {
rd.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
URLConn.disconnect();
}
String content = receive.toString();
LOGGER.info("content = "+content);
return content;
}
Controller
@RestController@EnableAutoConfiguration@RequestMapping("/api/ask")@ApiIgnorepublic class AskController { @Autowired AskService askService; /** * 获取chatGPT返回的数据 * @param question * @return */ @PostMapping("/chatGPT") public ApiResult<String> chatGPT(String question) { ApiResult<String> apiResult = new ApiResult<String>(); String result = askService.getResultFromChatGPT(question); return apiResult.success(result); }}
Service
@Servicepublic class AskService { private static final Logger LOGGER = LoggerFactory.getLogger(AskService.class); /** * 获取chatGPT返回的数据 * @param question * @return */ public String getResultFromChatGPT(String question) { // 调用API接口 String returnContent = HttpService.postChatGPT(question); LOGGER.info("returnContent = " + returnContent); // 解析返回的数据 JSONObject returnContentObject = JSONObject.parseObject(returnContent); JSONArray choicesArray = returnContentObject.getJSONArray("choices"); if(choicesArray != null && !choicesArray.isEmpty()) { JSONObject choicesObject = choicesArray.getJSONObject(0); if(choicesObject != null) { return choicesObject.getString("text"); } } return ""; }}
Ok, that's all for the backend code.
front end
The front-end page is very simple, just use HTML and jQuery directly, and add bootstrap5 for a more beautiful look.
<!DOCTYPE html>
<html lang="zh-CN">
<head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="keywords" content="chatGPT,你问我答">
<meta name="description" content="随便问点什么">
<meta name="author" content="[email protected]">
<link rel="icon" href="https://getbootstrap.com/docs/4.0/assets/img/favicons/favicon.ico">
<title>你问我答</title>
<link rel="canonical" href="https://getbootstrap.com/docs/4.0/examples/album/">
<link href="/assets/bootstrap-5.1.3-dist/css/bootstrap.css" rel="stylesheet">
<!-- jQuery 和 Bootstrap 集成包(集成了 Popper) -->
<script src="/assets/js/jquery-3.6.0.js"></script>
<!-- JavaScript Bundle with Popper -->
<script src="/assets/bootstrap-5.1.3-dist/js/bootstrap.bundle.js"></script>
<script src="/assets/js/index/index.js?2"></script>
<!-- Custom styles for this template -->
<link href="/assets/css/index/index.css" rel="stylesheet">
</head>
<body>
<header>
<div class="navbar navbar-dark bg-dark box-shadow">
<div class="container d-flex justify-content-between">
<a class="navbar-brand d-flex align-items-center">
<svg xmlns="http://www.w3.org/2000/svg" width="20" height="20" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="mr-2"><path d="M23 19a2 2 0 0 1-2 2H3a2 2 0 0 1-2-2V8a2 2 0 0 1 2-2h4l2-3h6l2 3h4a2 2 0 0 1 2 2z"></path><circle cx="12" cy="13" r="4"></circle></svg>
<strong>随便问问</strong>
</a>
</div>
</div>
</header>
<main role="main">
<div class="container">
<div class="form-group" style="margin-top: 20px;">
<label for="question">随便问些什么</label>
<textarea class="form-control" name="question" id="question" rows="2"></textarea>
</div>
<div class="form-group" style="margin-top: 20px;">
<label for="answer">回复</label>
<textarea class="form-control" name="answer" id="answer" rows="16"></textarea>
</div>
<div class="form-group" style="margin-top: 20px;display: flex;justify-content: center;">
<button class="btn btn-primary loading-btn" type="button" disabled style="display: none">
<span class="spinner-border spinner-border-sm" role="status" aria-hidden="true"></span>
请耐心等待...
</button>
<button class="btn btn-primary submit-btn">问一问</button>
</div>
</div>
</main>
<footer class="text-muted">
<div class="container">
<p>有问题请百度微酸云,关注微酸云影视公众号</p>
</div>
</footer>
</body>
</html>
js code
$(function(){ // 微信内置浏览器不支持onclick事件,在这里绑定 $(".submit-btn").attr('onclick','getResultFromChatGPT()')})// 新增用户访问数据function getResultFromChatGPT() { if($("#question").val() === '') { alert('问题不能为空') return } $(".loading-btn").show() $(".submit-btn").hide() $("#answer").text('') //获取商机状态列表 $.ajax({ type:"post", url:"/api/ask/chatGPT", data:{ question: $("#question").val() }, success:function(result){ console.log(result) $("#answer").text(result.data) $(".loading-btn").hide() $(".submit-btn").show() } })}
One pitfall is that if you open this webpage directly in WeChat, the browser that comes with WeChat cannot recognize the onclick method of html, and the click event can only be bound in js.
$(".submit-btn").attr('onclick','getResultFromChatGPT()')
Also, it may take a long time to call the API, so you must make a reminder to wait patiently, otherwise the user will think that the system has not responded.
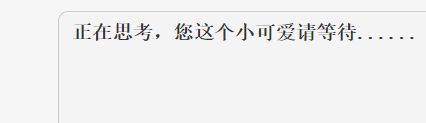
run
Ok, the code is finished, let's run the program to see the effect:
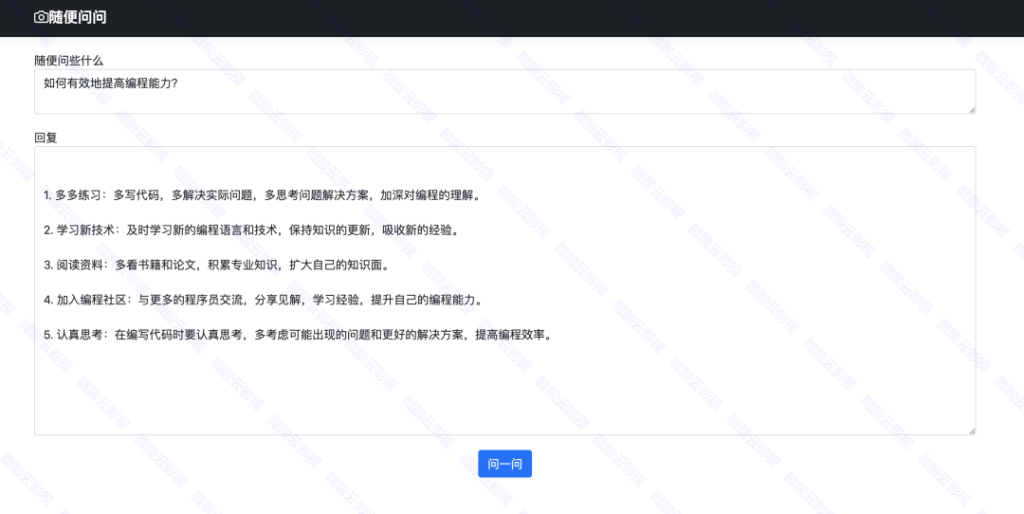
Well, that's a good suggestion.
If you open it, please pay attention to the official account of "Slightly Sour Cloud Film and Television"
Download an app that I run, pure and ad-free (aggregate film and television comic novels, chatgpt, and other various tools):
Direct link: myapp.weiyunyingshi.apk
https://wwxm.lanzouo.com/i83vG0qje00f
密码:3062