Let's take a look at an example to see how call by value and call by reference are going on.
I will talk in detail why the two numbers in the main function cannot be exchanged through the call by value of the function, and how we can solve this problem.
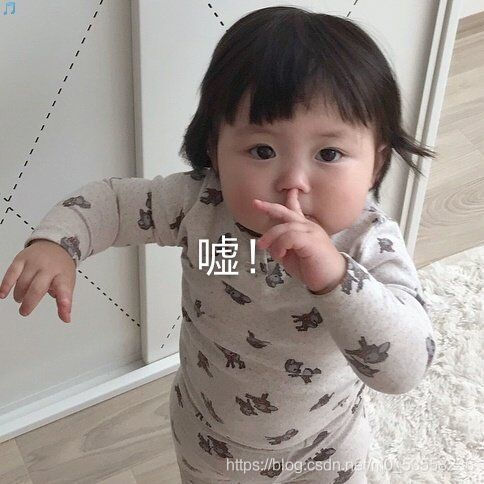
Quietly tell you: I used to only know that the two numbers in the main function cannot be exchanged through the call by value of the function, but I still don’t know why it doesn’t work. If you still have this doubt now, you might as well set up a small bench, sit down, and listen to me explain the principle to you in detail.
Table of contents
Example: Swap two variables
example:
"Write a function that swaps the contents of two integer variables"
1. Do not exchange by writing external functions
First of all, if you don't exchange the value of two numbers by writing an external function, it's like this:
#include<stdio.h>
int main()
{
int a = 10;
int b = 20;
int c = 0;
printf("交换前:a=%d b=%d\n", a, b);
c = a;
a = b;
b = c;
printf("交换后:a=%d b=%d\n", a, b);
return 0;
}
output
Before exchange: a=10 b=20
After exchange: a=20 b=10
But now, we need to swap a and b by writing an external function,
2. Try to exchange two numbers by calling the function by value
According to the above ideas, we wrote the following code:
#include<stdio.h>
void Swap(int x, int y)
{
int z = 0;
z = x;
x = y;
y = z;
}
int main()
{
int a = 10;
int b = 20;
printf("交换前:a=%d b=%d\n", a, b);
//函数
Swap(a, b);
printf("交换后:a=%d b=%d\n", a, b);
return 0;
}
But let's run it
and find that the result of the operation is like this:
Before exchange: a=10 b=20
After exchange: a=10 b=20
The values of a and b have not changed.
Why is this?
The principle of call by value
In fact,
when the actual parameters a and b are passed to the formal parameters x and y, the formal parameters will be a temporary copy of the actual parameters.
Changing the formal parameter variables x and y will not affect the actual parameters a and b. (The concept and summary of actual parameters and formal parameters are placed at the end of the article. If necessary, you can read it first.) For
details, let’s take a look. The principle is this:
we created an a and a b, and there are values in a 10, a value of 20 is placed in b;
then pass parameters through the function Swap to create variable x and variable y, get 10 of a in x, and 20 of b in y.
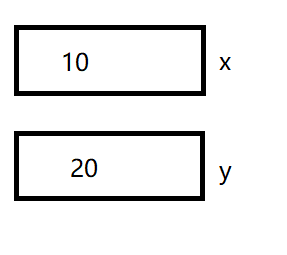
Then, through the variable z, to exchange the values of x and y.
We need to know that x and y are an independent memory space , and changes in the values of x and y will not affect a and b at all.
So the values of a and b are not swapped at all.
#So how do we solve the above problems?
3. Exchange two numbers by calling the function by reference (positive solution)
We can see from the above example that from the perspective of space, there is no connection between the actual parameter and the formal parameter, and the formal parameter cannot change the actual parameter in the above way . So next we need to connect them.
We obtain the address of a through a pointer variable pa.
int a = 10;
int* pa = &a;
Change the value of a by pa
*pa = 20;//Change the value in the address pointed to by pa (that is, the value stored in a) to 20
In this way, the address of a is stored in pa, so that pa and a are connected. The address of a can be found through pa, and then the value of a can be changed.
#include<stdio.h>
int main()
{
int a = 10;
int* pa = &a;
*pa = 20;
printf("%d\n", a);
return 0;
}
Run it and get
20
It can be seen that the value of a has indeed changed. According to this idea, we pass the addresses of a and b to the Swap function
Swap(&a, &b);
Then the parameter of our external Swap function should be a pointer variable, because Swap(&a, &b) is passed an address, and the address should be placed in the pointer variable.
Swap(int* px, int* py)
{
}
The address of a is stored in the pointer px, and the address of b is stored in py.
The value in the address pointed to by px (that is, the value of a) and the value in the address pointed to by py (that is, the value of b) are exchanged through the variable z.
void Swap(int* px, int* py)
{
int z = 0;
z = *px;//*px相当于是a
*px = *py;//*py相当于是b
*py = z;
}
The whole code looks like this
#include<stdio.h>
void Swap(int* px, int* py)
{
int z = 0;
z = *px;
*px = *py;
*py = z;
}
int main()
{
int a = 10;
int b = 20;
printf("交换前:a=%d b=%d\n", a, b);
//函数
Swap(&a, &b);
printf("交换后:a=%d b=%d\n", a, b);
return 0;
}
If you don’t understand it yet, don’t panic, let’s draw a picture to see how it works:
Principle of call by address
At the beginning, we created a variable a and b, their values are 10 and 20 respectively.
a and b open up space in the memory, assuming that the starting address of the space of a is 0x0012ff40 the
starting address of the space of b is 0x0012ff48;
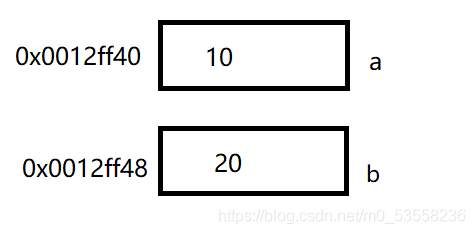
Then we pass the parameters through the Swap function, the address of a (0x0012ff40) is passed to px, and the address of b (0x0012ff48) is passed to py. At this time, the address of a is placed in px and the addresses of a and b are found through px and py, so
that Swaps the values of a and b.
Put call by value and call by reference together.
The concept of function parameters
Let's take a look at the concept of function parameters:
function parameters
1 Actual parameter (actual parameter):
The parameters actually passed to the function are called actual parameters. Actual parameters can be: constants, variables, expressions, functions, etc.
No matter what type of quantity the actual parameters are, they must have definite values when the function is called, so that these values can be transferred to the formal parameters.
2 formal parameters (parameters):
Formal parameters refer to the variables in parentheses after the function name, because formal parameters are only instantiated (allocated memory units) when the function is called, so they are called formal parameters.
Formal parameters are automatically destroyed when the function call completes. Therefore formal parameters are only valid within the function.
The parameters x, y, px, py in the Swap1 and Swap2 functions above are all formal parameters. The a, b passed to Swap1 in the main function and the &a, &b passed to the Swap2 function are the actual parameters.
Due to my limited ability, please correct me if I make any mistakes!
If this article is helpful to you, remember to like and pay attention !
I am summarizing related content recently, you can follow me and view more of my articles.
After all, we are going to learn together and make progress together!