Vue.js 2.0 study notes (2) Getting to know Vue for the first time
Article directory
- Vue.js 2.0 study notes (2) Getting to know Vue for the first time
- 1. Getting to know Vue for the first time
- 2. Basic grammar and instructions
- Three, ES6 object enhanced writing
- 4. The life cycle of Vue
- 5. Filter (Vue 3.x is no longer used)
- 6. Listener
- Seven, computed properties
- Eight, axios
- Nine, Vue-cli
1. Getting to know Vue for the first time
- Vue is a progressive framework, what is progressive
- Progressive means that Vue can be embedded as a part of the application, and the interactive experience is richer
- If you want to use Vue to implement more business logic, Vue's core library and its ecological environment such as: Core+Vue-router+Vuex can also meet various needs
- Features of Vue and common advanced features in web development
-
Decoupling Views and Data
-
reusable components
-
Front-end routing technology
-
state management
-
Virtual DOM
-
- Vue is declarative programming
- Vue characteristics:
- Data-driven view (one-way data binding): When the page data changes, the page will be reloaded
- Two-way data binding : When filling out the form , this feature can assist developers to automatically synchronize the content filled by the user to the data source without manipulating the DOM (form is responsible for collecting data, and Ajax is responsible for submitting data)
1.1 MVX pattern
1.1.1 MVC
1. MVC definition
The MVC pattern stands for the Model-View-Controller (Model-View-Controller) pattern. This pattern is used for layered development of applications .
-
Model: Represents the core of the application (such as a database)
-
View: A view represents a visualization of the data contained by the model
-
Controller (controller): The controller acts on the model and view. It controls the flow of data to model objects and updates views when data changes. It separates the view from the model
2. MVC design pattern
MVC is a combination of multiple design patterns. The three patterns that make up MVC are combination pattern, observer pattern, and strategy pattern . The power of MVC in software development is ultimately inseparable from the tacit cooperation of these three patterns.
-
Composite mode: Composite mode is only active in the view layer , and the class hierarchy of the composite mode is tree-like . For example: when we do Web, the view layer is an html page, and the structure of html is tree-like.
-
Observer mode: The observer mode consists of two parts, the observed object and the observer, and the observer is also called the listener. Corresponding to MVC, the Model is the object to be observed, and the View is the observer . Once the Model layer changes, the View layer will be notified to update.
-
Strategy mode: Strategy mode is the relationship between View and Controller, Controller is a strategy of View , and Controller is replaceable for View.
The design pattern corresponding to the relationship between the layers of MVC:
-
View layer: implements combination mode alone
-
Model layer and View layer : implements the observer mode
-
View layer and Controller layer : implement the strategic pattern
1.1.2 MVP
Model-View-Presenter; MVP is evolved from the classic pattern MVC
There is an important difference between MVP and MVC: in MVP, View does not directly access Model, and the communication between them is carried out through Presenter. All interactions occur inside Presenter. Presenter completely separates view and model . The Presenter is not directly related to the specific view, but interacts through the defined interface, so that the Presenter can be kept unchanged when the view is changed
1.1.3 MVVM
Vue.js is the representative framework of MVVM.
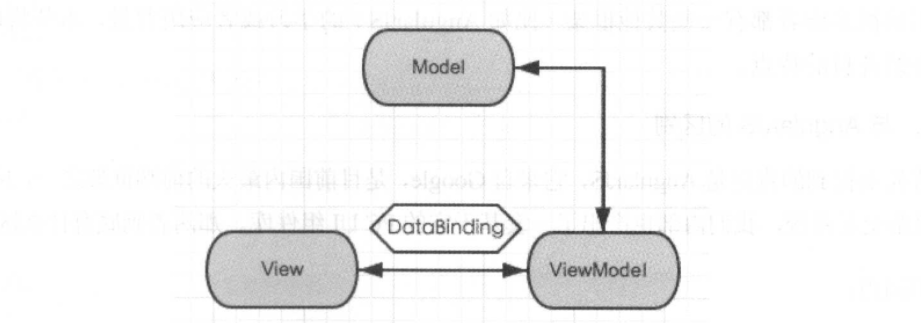
Changes in the View will be automatically updated to the viewModel, and changes in the viewModel will also be automatically synchronized to the view for display.
(ViewModel) A ViewModel is an abstraction of a View that exposes common properties and commands. MVVM does not have a controller in the MVC pattern, nor a presenter in the MVP pattern, but some are a binder. In the view model, the binder communicates between the view and the data binder. Its functions: First, convert [Model] into [View], that is, convert the data passed by the backend into the page you see. The way to achieve it is: data binding. The second is to convert [view] into [model], that is, to convert the viewed page into back-end data. The way to achieve it is: DOM event monitoring. Both directions are implemented, which we call two-way binding of data.
1. MVVM in Vue
ViewModel is an instance of vue
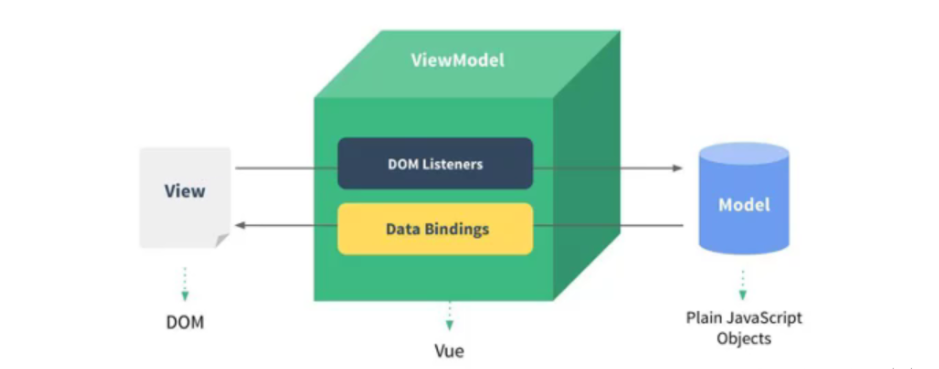
2. Correspondence between code in Vue and MVVM
2. Basic grammar and instructions
2.1 Interpolation operation
Can only be used in content nodes, not attribute nodes
2.1.1 Mustache syntax
Variables can be written directly in Mustache syntax, and simple expressions can also be written :
<div id="app">
<h2>Message:{
{message}}</h2>
<h2>{
{firstName + '' + lastName}}</h2>
<h2>{
{firstName}} {
{lastName}}</h2>
</div>
Vue allows adding filters after expressions, and multiple filters can be connected in series
{
{example | filterA | filterB}}
Notice:
- Mustache syntax cannot be used on HTML attributes, in this case you should use
v-bind
Sometimes you only need to render the data once, which can be achieved by "*" :
<span>text: {
{*msg}}</span>
If it is an HTML fragment:
<div>logo: {
{
{logo}}}</div>
logo:'<<span>hhh</span>>'
2.1.2 v-once directive
<span v-once>这个将不会改变: {
{ msg }}</span>
Notice:
- No expression is required after this directive
- One-time interpolation can also be performed with the v-once directive. But this instruction indicates that elements and components are only rendered once and will not change as the data changes
2.1.3 v-html directive
Background: In some cases, the data requested from the server itself is HTML. If it is output through { {}}, the output is the HTML source code and will not be parsed
- v-html directive followed by a string
- The instruction will parse and render the html page of string
<h2 v-html='link'></h2>
// link :'<a> href = 'http://www.baidu.com'</a>'
2.1.4 v-text directive
- Similar to mustache: both are used to display data in the interface
- v-text receives a string type
<h2 v-text='message'></h2>
2.1.5 v-pre directive
- v-pre: Used to skip the compilation process of this element and its sub-elements, used to display the original mustache syntax, that is, its content will not be parsed
<p>{
{message}}</p> //hello world!
<p v-pre>{
{message}}</p> //{
{message}}
2.1.6 v-cloak directive
When the network is slow and the webpage is still loading Vue.js, causing Vue to render too late, the page will directly display the uncompiled Vue source code
<div id="app" v-cloak>
{
{context}}
</div>
<script>
var app = new Vue({
el: '#app',
data: {
context:'互联网头部玩家钟爱的健身项目'
}
});
</script>
[v-cloak]{
display: none;
}
2.2 v-bind dynamic binding properties
- Role: dynamically bind one or more properties, or pass props value to another component
- Syntactic sugar - :
- Case: such as binding the src of the picture, the href of the website link, dynamically binding some classes and styles
<div id="app">
<a v-bind:href="">hhh</a>
<img v-bind:src="" alt="">
</div>
<script>
let app = new Vue({
el:"#app",
data: {
logoURL:'https://vuejs.org/images/logo.png',
link:'https://vuejs.org'
}
})
</script>
2.2.1 v-bind binding class
1. Binding method
- object syntax
用法一:直接通过{}绑定一个类
<h2 :class="{
'active': isActive}">Hello World</h2>
用法二:通过判断,传入多个值
<h2 :class="{
'active': isActive, 'line': isLine}">Hello World</h2>
用法三:和普通的类同时存在,并不冲突
<h2 class="title" :class="{
'active': isActive, 'line': isLine}">Hello World</h2>
用法四:若过于复杂,可以放在一个methods或computed中
注意:classes是计算属性
<h2 class="title" :class="classes"></h2>
-
Array syntax (less used)
: class followed by an array
用法一:直接通过{}绑定一个类
<h2 :class="['active']}">Hello World</h2>
用法二:通过判断,传入多个值
<h2 :class="['active','line']">Hello World</h2>
用法三:和普通的类同时存在,并不冲突
<h2 class="title" :class="['active','line']">Hello World</h2>
用法四:若过于复杂,可以放在一个methods或computed中
注意:classes是计算属性
<h2 class="title" :class="classes"></h2>
2.2.2 v-bind binding style
-
Use v-bind:style to bind some inline styles
-
Pay attention when writing attribute names, such as font-size:
- CamelCase can be used: fontSize
- Separated by dashes, remember to enclose them in single quotes : 'font-size'
-
Binding method:
- object syntax
语法: :style ="{color:currentColor, fontSize:fontSize + "px"}"
style is followed by an object type:
- The key of the object is the CSS property name
- The value of the object is a specific assigned value , and the value can come from the attributes in data
<h2 :style="{fontSize:'50px'}">Hello World</h2>
Note: The above 50px must be added with single quotes. Without single quotes, it will be considered as a variable , and if the variable name cannot start with a number, an error will be reported. Adding single quotes is a string, and vue will remove the single quotes when parsing
- Array syntax (less used)
:style="[baseStyles,overrideingStyles]"
style is followed by an array type
2.3 Condition judgment
2.3.1 v-if v-else-if v-else
- Render or destroy elements or components in the DOM according to the value of the expression. If the condition is false, the corresponding element and its child elements will not be rendered, and the corresponding label will not appear in the DOM
- The logic is called complex, it is best to use computed properties
Case: Login switch
Requirement: When the user logs in again, they can switch to log in with the user account or email address
<div id="app">
<span v-if="type === 'username'">
<label for="">用户账号:</label>
<input placeholder="用户账号">
</span>
<span v-else>
<label for="">邮箱地址:</label>
<input placeholder="邮箱地址">
</span>
<button @click="handleToggle">切换类型</button>
</div>
<script>
let app = new Vue({
el:"#app",
data: {
type:'username'
},
methods: {
handleToggle() {
this.type = this.type === 'email' ? 'email' : 'username';
}
}
})
</script>
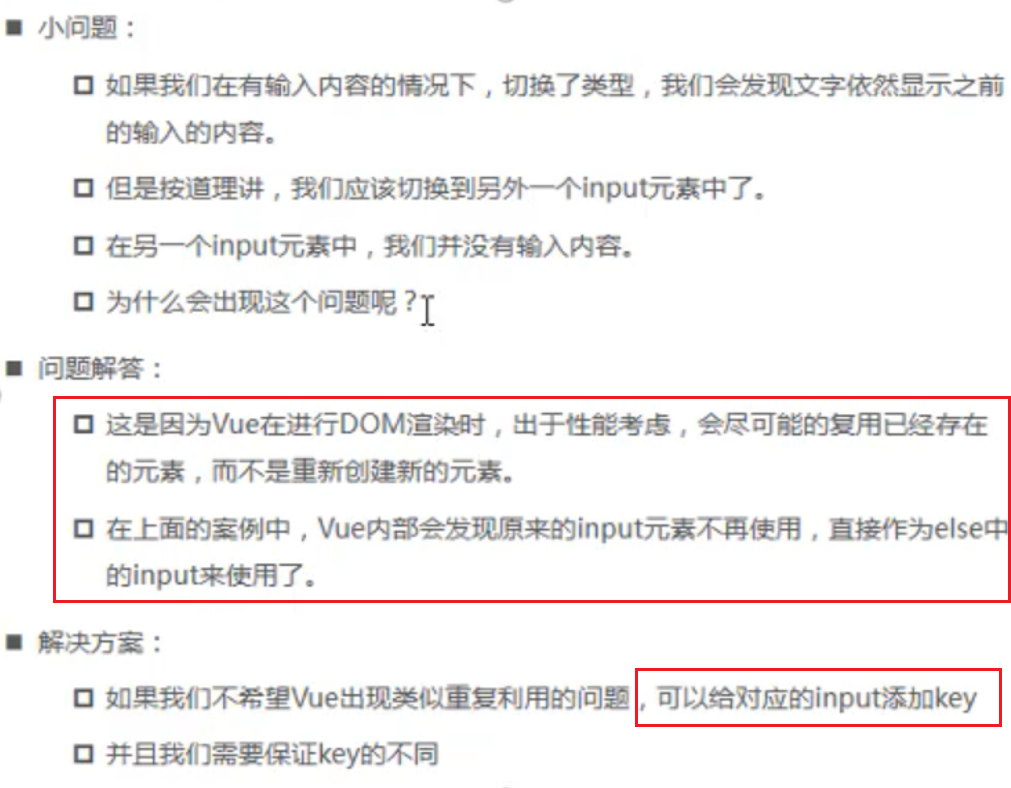
<span v-if="isUser">
<label for="username">用户账号:</label>
<input type='text' id='username' placeholder="用户账号" key='username'>
</span>
<span v-else>
<label for="">邮箱地址:</label>
<input type='text' id='email' placeholder="邮箱地址" key='email'>
</span>
<button @click="isUser = !isUser">切换类型</button>
Key points: Adding inputs with different keys will not cause repeated use
2.3.2 v-show
Also used to determine whether an element is rendered
Comparison of v-if and v-show
- v-if When the condition is false, there will be no corresponding element in the DOM at all
- v-show simply sets the element's display property to none when the condition is false . That is, the DOM element is created, but hidden
How to choose v-if and v-show in development
- Use v-show when you need to switch between showing and hiding frequently
- When there are only a few switches, use v-if
2.4 Loop Traversal
2.4.1 v-for traverse array or object
Iterate over the array:
<ul>
<li v-for="item in names">{
{item}}</li>
</ul>
// 遍历过程中获取索引值
<ul>
<li v-for="(item, index) in names">
{
{index+1}}.{
{item}}
</li>
</ul>
<ul>
<li v-for="item in names">
{
{$index}}-{
{item}}
</li>
</ul>
Iterate over objects:
<ul>
<li v-for="(value,key) in info">{
{value}}-{
{key}}</li>
</ul>
<ul>
<li v-for="(value,key,index) in info">{
{value}}-{
{key}}-{
{index}}</li>
</ul>
Main points:
- When getting key and value, value comes first, format: (value, key)
- When getting key, value and index, format: (value, key, index)
- Regardless of traversing objects or arrays, when you want to get the index, the index is always placed at the end , and the index is not described in the for, you can directly **use { {$index}} ** in the content to get the index
2.4.2 v-for binding and non-binding key attributes
<ul>
<li v-for="item in letters" :key="item">{
{value}}-{
{key}} </li>
</ul>
It is officially recommended that when using v-for, add a key attribute to the corresponding element or component, and make a unique identification for each node. The function:
- The Diff algorithm can correctly identify this node
- Efficiently update the virtual DOM
2.4.3 Reactive methods in arrays
- Because Vue is responsive, when the data changes, Vue will automatically monitor the data changes, and the view will be updated accordingly
- Vue includes a set of methods for observing array compilation, using them to change the array will also trigger the update of the view (responsive):
- push()
- pop()
- shift()
- unshift()
- splice()
- sort()
- reverse()
//注意:通过索引值修改数组中的元素
this.letters[0] = 'hhhh'; // 非响应式
this.letters.splice(0, 1, 'hhhh'); //响应式
Vue.set(this.letters,0,'hhhh'); //响应式
2.4.4 Functional programming (higher-order functions)
Higher order functions: filter/reduce/map
(1) filter (filter)
Main points:
1. The callback function in filter must return a Boolean value . When true is returned, the function will automatically add the value n of this callback to a new array; when it is false, the function will filter the value n of this time
2. The number of times the callback function is executed is the number of array elements
3. The new array is automatically generated, only one variable is needed to receive
const nums = [10,20,304,304,1204,221,45];
let newNums = nums.filter(function(n) {
return n < 100;
})
console.log(newNums);
(2) map
let newNums2 = nums.map(function(n) {
return n * 2;
})
(3) reduce
Summarize all the contents of the array: add all or multiply all
let total = nums.filter(n => n < 100).map(n => n*2).reduce((pre,n) => pre + n);
2.5 Event monitoring v-on
- Role: used to bind event listeners
- Syntactic sugar: @
- Parameters: event
<div id="app">
<h2>点击次数:{
{counter}}</h2>
<button v-on:click="counter++">按钮1</button>
<button @click="btnClick">按钮2</button>
</div>
<script>
let app = new Vue({
el:"#app",
data: {
counter:0
},
methods: {
btnClick() {
this.counter++
}
}
})
</script>
2.5.1 v-on parameter
When defining methods in methods for @click calls, you need to pay attention to parameter issues:
-
Situation 1: If the method does not require additional parameters, then the () after the method may not be added. If the method itself has a parameter, the native event event parameter will be passed in by default.
-
Case 2: If you need to pass in a certain parameter and event at the same time, you can pass in the event through $event
<div id="app">
<h2>点击次数:{
{counter}}</h2>
<button @click="handleAdd"></button>
<button @click="handleAddTen(10,$event)"></button>
</div>
<script>
let app = new Vue({
el:"#app",
data: {
counter:0
},
methods: {
handleAdd(event) {
this.counter++;
},
handleAddTen(count,event) {
this.counter += count;
if(count % 2 == 0) {
event.targer.style.background-color = 'red'
}
}
}
})
</script>
2.5.2 Modifiers for v-on
Vue provides modifiers to help us handle some events:
- .stop : calls event.stopPropagation()
- .prevent : call event.preventDefault()
- .{keyCode | keyAlias} : the callback will only be fired when the event is fired from a specific key
- .native : Listen to the native event of the root element of the component
- .once : trigger the callback only once
<!-- 停止冒泡 -->
<button @click.stop="btnClick"></button>
<!-- 阻止默认行为 -->
<button @click.prevent="doThis"></button>
<!-- 阻止默认行为,无表达式 -->
<form @click.prevent></form>
<!-- 串联修饰符 -->
<button @click.stop.prevent="doThis"></button>
<!-- 键修饰符,键别名 -->
<input @keyup.enter="onEnter">
<!-- 键修饰符,键代码 -->
<input @keyup.13="onEnter">
<!-- 点击回调只会触发一次 -->
<button @click.once="doThis"></button>
2.6 form binding v-model
2.6.1 Basic usage of v-model
-
Role: realize the two-way binding of form elements and data
-
v-model is actually syntactic sugar, behind it contains two operations:
- v-bind: bind value value
- v-on: Bind events to the current element
<div id="app">
<input type="text" v-model="message">
<h2>{
{message}}</h2>
</div>
<script>
let app = new Vue({
el:"#app",
data: {
message:''
}
})
</script>
When we enter content in the input box, because the v-model in the input is bound to the message, the input content will be passed to the message in real time, and the message will change. And use the Mustache syntax to bind the value of the message to the DOM, so the DOM will change accordingly
2.6.2 v-model + radio
[External link picture transfer failed, the source site may have an anti-leeching mechanism, it is recommended to save the picture and upload it directly (img-ANB7UAkR-1635481971630)(C:\Users\Xiaomeng\Desktop\image-20211021143537209.png)]
Key points: If v-models are bound to the same variable, they can also be mutually exclusive after deleting the name
2.6.3 v-model+checkbox
1. checkbox radio button
<div id="app">
<label for="agree">
<input type="checkbox" id="agree" v-model="isAgree">同意协议
</label>
<h2>您的选择是:{
{isAgree}}</h2>
<button :disaled="!isAgree">下一步</button>
</div>
2. checkbox multi-selection box
<input type="checkbox" value="篮球" v-model="hobbies">篮球
<input type="checkbox" value="足球" v-model="hobbies">足球
<input type="checkbox" value="乒乓球" v-model="hobbies">乒乓球
<input type="checkbox" value="羽毛球" v-model="hobbies">羽毛球
<h2>您的爱好是:{
{hobbies}}</h2>
<script>
let app = new Vue({
el:"#app",
data: {
message: '你好啊',
isAgree: false, //单选框
hobbies: [] //多选框
}
})
</script>
Key points: value is the value that will be returned after clicking
2.6.4 v-model + select
radio
- v-model is bound to a value
- When we select an option, its corresponding value will be assigned to select (fruit)
multiple choice
- v-model is bound to an array
- When multiple values are selected, the value corresponding to the selected option will be added to the array selects (fruits)
<div id="app">
<!-- 选择一个 -->
<select name="abc" v-model="fruit">
<option value="苹果">苹果</option>
<option value="香蕉">香蕉</option>
<option value="西瓜">西瓜</option>
<option value="梨子">梨子</option>
</select>
<h2>您选择的水果是:{
{fruit}}</h2>
<!-- 选择多个 -->
<select name="abc" v-model="fruits" multiple>
<option value="苹果">苹果</option>
<option value="香蕉">香蕉</option>
<option value="西瓜">西瓜</option>
<option value="梨子">梨子</option>
</select>
<h2>您选择的水果是:{
{fruits}}</h2>
</div>
<script>
let app = new Vue({
el:"#app",
data: {
message: '你好啊',
fruit:'香蕉',
fruits:[]
}
})
</script>
2.6.5 input binding
<div id="app">
<label v-for="item in originHobbies" :for="item">
<input type="checkbox" :value="item" :id="item" v-model="hobbies">{
{item}}
</label>
</div>
<script>
let app = new Vue({
el:"#app",
data: {
message: '你好啊',
hobbies:[],
originHobbies:['篮球','足球','乒乓球','羽毛球','台球']
}
})
</script>
2.6.6 Modifiers of v-model
-
lazy modifier
- Function: In order to avoid frequent data updates, the lazy modifier allows the data to be updated only when the focus is lost or entered, rather than frequently updated during the input process
- By default, v-model synchronizes the data in the input box in the input event, that is, once the data occurs, the data in the corresponding data of the century will automatically change
-
number modifier
-
Function: Allows the content entered in the input box to be automatically converted to a digital type
-
By default, whether we enter numbers or letters in the input box, it will be treated as a string type. When we want to deal with digital types, it is best to treat the content directly as numbers
-
-
trim modifier
- Function: filter the spaces on the left and right sides of the content
- There may be many spaces at the beginning and end of the input content, and it is usually desirable to remove them
<input type="text" v-model.lazy="message">
<h2>{
{message}}</h2>
<input type="number" v-model.number="age">
<h2>{
{age}}</h2>
2.7 Summary
- Content Rendering Directive: Renders the text content of a DOM element
- v-text
- { { }}
- v-html
- Attribute binding instructions: dynamically bind attribute values for the attributes of elements
- v-bind
- Event Binding Directive
- v-on
- Two-way binding instructions: Quickly get form data without manipulating DOM
- v-model
- Conditional Rendering Directives
- v-if
- v-show
- List rendering instructions
- v-for
Three, ES6 object enhanced writing
In ES6, many enhancements have been made to object literals.
3.1 Shorthand for attribute initialization
// ES6之前
let name = 'why';
let age = 18;
let obj1 = {
name: name,
age: age
}
console.log(obj1);
// ES6之后
let obj2 = {
name, age
}
console.log(obj2);
3.2 Shorthand for methods
// ES6之前
let obj1 = {
test: funtion() {
console.log("obj1");
}
};
obj1.test();
// ES6之后
let obj1 = {
test() {
console.log("obj2");
}
};
obj2.test();
4. The life cycle of Vue
4.1 The concept of life cycle
Each component of Vue is independent, and each component has a life cycle that belongs to it. From a component creation (new), data initialization, mounting, updating, and destruction , this is the so-called life cycle of a component. At the same time, some functions called life cycle hooks will also be run during this process , which gives users the opportunity to add their own code at different stages.
4.2 Lifecycle hooks (functions)
-
beforeCreate()
At this time, the option object of the component has not been created, and el and data have not been initialized, so methods and data on methods, data, computed, etc. cannot be accessed.
-
create()
Invoked after the instance has been created. In this step, the instance has completed the following configurations: data observer, operation of attributes and methods, and watch/event event callback . However, the mount phase has not yet started and the $el property is not currently visible . You can call the methods in methods to change the data in data; get the calculated attributes in computed , and often make network requests in this hook
-
beforeMount()
Called before the mount starts: the relevant render function is called for the first time (virtual DOM). The instance has completed the following configurations: Compile the template, generate html from the data in the data and the template, and complete the initialization of el and data. Note that the html has not been mounted on the page yet .
-
mounted()
**Mounting is complete, that is, the HTML in the template is rendered to the HTML page. **At this time, you can generally do some ajax operations, and mounted will only be executed once
-
beforeUpdate()
Called before the data is updated, before the virtual DOM is re-rendered and patched , the state can be further changed in this hook, and no additional re-rendering process will be triggered
-
update()
Called after the virtual DOM has been re-rendered and patched due to data changes. When called, the component DOM has been updated, so operations that depend on the DOM can be performed. In most cases, changes during this period should be avoided state, as this could cause an infinite loop of updates, this hook is not called during server-side rendering
-
beforeDestroy()
Called before the instance is destroyed, the instance is still fully available
- In this step, you can also use this to get the instance ,
- Generally, some reset operations are done in this step, such as clearing the timer in the component and the dom event listened to
-
destroyed()
Called after the instance is destroyed. After calling, all event listeners will be removed, and all sub-instances will also be destroyed. This hook is not called during server-side rendering
NOTE: Do not use arrow functions such as created: () => console.log(this.a)
or on options properties or callbacksvm.$watch('a', newValue => this.myMethod())
. because arrow functions don'tthis
5. Filter (Vue 3.x is no longer used)
5.1 Basic use of filters
Filters are often used for formatting text. Filters can be used in two places: interpolation expressions and v-bind attribute bindings
The filter is a function , which should be added at the end of the JS expression and called with **"pipe character"**, for example:
<!-- 在双括号中通过管道符调用 captalize 过滤器,对 message 的值进行格式化 -->
<!-- message作为参数传给capitalize函数,最后在message位置看到的是capitalize函数的返回值-->
<p>{
{message | capitalize}}</p>
<!-- 在v-bind 中通过“管道符”调用 formatID 过滤器,对 rawID 的值进行格式化 -->
<div v-bind:id='rawID | foematID'></div>
<div id="app">
<p>message的值: {
{message | capitalize}}</p>
</div>
<script src="./vue.js"></script>
<script>
const vm = new Vue({
el:'#app',
data: {
message: 'hello vue'
},
// 过滤器函数
filters: {
//注意:过滤器函数中的形参val永远都是管道符前面的那个值
capitalize(val) {
//强调:过滤器中一定要有返回值
const first = val.charAt(0).toUpperCase();
const other = val.slice(1);
return first + other;
}
}
})
</script>
Notice:
- The formal parameter val in the filter function is always the value before the pipe character
- There must be a return value in the filter
- The filter itself is a function
5.2 Private and global filters
-
Private filter: The filter defined under the filters node can only be used in the el area controlled by the current vm instance
-
Global filter: Filters can be shared between multiple vue instances
Format for defining global filters:
// 第一个参数:全局过滤器的名字, 第二个参数:全局过滤器的处理器
Vue.filter('capitalize',(str) => {
return str.charAt(0).toUpperCase() + str.slice(1);
})
Note: If the name of the private filter is the same as that of the global filter, according to the "nearest principle", the private filter is called
Case: You can use the global filter to format the time, and use dayjs() to quickly format
5.3 Continuously calling multiple filters
{
{ message | filterA | filterB}}
Parse:
- Send the value of message to filterA for processing
- Pass the value processed by filterA to filterB for processing
- Finally return the result processed by filterB as the final value
5.4 Filter parameters passing
<p>{
{message | filterA(arg1,arg2)}}</p>
<script>
//第二个参数中的第一个参数永远是管道符前面待处理的值
Vue.filter('filterA',(message,arg1,arg2) => {
...
})
</script>
6. Listener
6.1 The concept of watch listener
The watch listener allows developers to monitor data changes and perform specific operations on data changes . The syntax is as follows:
const vm = new Vue({
el:'#app',
data: {
username: ''
},
watch: {
// 监听username值的变化
// newVal是变化后的新值,oldVal是变化前的旧值
username(newVal, oldVal) {
console.log(newVal,oldVal);
}
}
})
Notice:
- The formal parameters in the monitoring function are "new before old"
- To listen to whoever is in the watch node as the function name
6.2 Business Scenario——Judge whether the username is occupied
<div id="app">
<input type="text" v-model='username'>
</div>
<script>
const vm = new Vue({
el:'#app',
data: {
usename: 'hello vue'
},
watch: {
username(newVal, oldVal){
if(newVal === '') return;
//1. 调用jQuery中的Ajax发起请求,判断 newVal是否被占用
$.get('http://www/escook.cn/api/finduser/' + newVal, function(result) {
console.log(result);
});
}
}
})
</script>
6.3 Options for listeners
6.3.1 Listener format
-
listener for method format
shortcoming:
- Cannot be triggered automatically when just entering the page
- If the listener is an object, if a property in the object changes, the listener will not be triggered
-
object format listener
advantage:
- The listener can be automatically triggered by the immediate option
- You can use the deep option to let the listener deeply monitor the changes of each property in the object
6.3.2 immediate attribute
<script>
const vm = new Vue({
el:'#app',
data: {
usename: 'hello vue'
},
watch: {
username:{
handler(newVal, oldVal) {
console.log(newVal,oldVal);
},
//immediate默认选项是false,作用是:控制侦听器是否自动触发一次
immediate: true
}
}
})
</script>
6.3.3 deep attribute (deep listening)
<script>
const vm = new Vue({
el: '#app',
data: {
info: {
usename: 'admin'
}
},
watch: {
info: {
handler(newVal) {
console.log(newVal);
},
deep: true
}
}
})
</script>
Seven, computed properties
7.1 Computed properties
Calculated properties are calculated after a series of calculations, and finally get an attribute value
<div id="app">
<h2>总价格:{
{totalPrice}}</h2>
</div>
<script src="./vue.js"></script>
<script>
let app = new Vue({
el:"#app",
data: {
books:[
{
id: 110, name: 'hhh', price: 119},
{
id: 111, name: 'xxx', price: 105},
{
id: 112, name: 'sss', price: 98},
{
id: 113, name: 'jjj', price: 87}
]
},
computed: {
totalPrice: function() {
let res = 0;
for(let i=0; i < this.books.length; i++) {
res += this.books[i].price;
}
return res;
//其他的for写法
for(let i in this.books){
this.books[i];
}
for(let book of this.books){
}
}
}
})
</script>
Notice:
-
totalPrice without brackets, treat it as an attribute
-
Calculated properties should be defined as method formats when declared, and used as properties when used
benefit:
- Implemented code reuse
- Computed properties are automatically re-evaluated as long as the dependent data source in the calculated property changes
7.2 Setters and getters for computed properties
Computed properties generally do not have a set method and are read-only properties
7.3 Comparison of computed properties and methods
<div id="app">
<!-- 1.直接拼接 -->
<h2>{
{firstName}} {
{lastName}}</h2>
<!-- 2.通过定义methods -->
<h2>{
{getFullName()}}</h2>
<!-- 3.通过computed -->
<h2>{
{fullName}}</h2>
</div>
Key points: The computed attribute is cached, no matter how many { {fullName}} need to be called, it is only calculated once
Eight, axios
Axios is a library focusing on network requests and data requests
8.1 Basic usage of axios
The basic syntax is as follows:
axios({
//请求方式
method: '请求的类型',
//请求地址
url: '请求的url',
//url中的查询参数(按需可选,一般GET用)
params:{
},
//请求体参数(按需可选,一般post用)
data:{
}
}).then((result) => {
//.then用来指定请求成功后的回调函数
//形参中的 result 是请求成功之后的结果
})
Method analysis:
- The return value obtained after calling the axios method is a Promise object
- Promise objects can use the .then method
8.1.1 Initiating a GET request
/*
get请求传递两个参数
参数一表示请求地址,参数二表示配置信息
配置信息中:
params:表示传递到服务器端的数据以url参数的形式拼接到请求地址后面
headers:表示请求头
例如:请求地址为:https://www.github.com/martians
params中 {page:1,per:3}
最终生成的url为:https://www.github.com/martians?page=1&per=3
*/
axios({
//请求方式
method: 'GET',
//请求地址
url: 'http://www.hhh.com/api/books',
//url中的查询参数(按需可选)
params:{
id:1
},
}).then((result) => {
console.log(result);
})
8.1.2 Initiating a POST request
axios({
method: 'POST',
url: 'http://www.hhh.com/api/books',
data:{
name:'zs',
age:20
},
}).then((result) => {
console.log(result);
})
8.1.3 Combine async and await to call axios
Main points:
- If the return value of calling a method is a Promise instance, await can be added in front
- await can only be placed in methods modified by async
document.querySelector('#btnPost').addEventListener('click', async function() {
const result = await axios({
method: 'POST',
url: 'http://www.hhh.com/api/books',
data: {
name: 'zs',
age: 20
}
})
})
8.1.4 Using destructuring assignment
Only get the data you care about
document.querySelector('#btnPost').addEventListener('click', async function() {
//解构复制的时候,使用:进行重命名
const {
data : res } = await axios({
method: 'POST',
url: 'http://www.hhh.com/api/books',
data: {
name: 'zs',
age: 20
}
})
console.log(res.data);
})
To summarize the steps to use axios:
- After calling axios, use async+await to simplify
- Use destructuring assignment to destructure the data attribute from the large object encapsulated by axios
- Rename the deconstructed data attribute with a colon, usually as { data: res }
8.2 Initiate requests based on axios.get and axios.post
8.2.1 axios.get
Grammar format:
axios.get('url地址', {
//GET参数
params: {
}
})
document.querySelector('#btnPost').addEventListener('click', async function() {
//解构复制的时候,使用:进行重命名
const {
data : res } = await axios.get('http://www.hhh.com/api/books',{
params:{
id: 1 }
})
console.log(res);
})
8.2.2 axios.post
Grammar format:
axios.post('url',{
/*post 请求体数据*/})
document.querySelector('#btnPost').addEventListener('click', async function() {
//解构复制的时候,使用:进行重命名
const {
data : res } = await axios.post('http://www.hhh.com/api/books',{
name: 'zs',
age: 20
})
console.log(res);
})
Nine, Vue-cli
9.1 Single Page Application
It means that there is only one HTML page in a web site, and all functions and interactions are completed in this one and only page.
9.2 vue-cli (scaffolding)
Vue-cli is a standard tool for Vue.js development, which simplifies the creation of engineering Vue projects based on webpack for programmers. It has already configured webpack for us, just use it directly
9.3 Installation and use
installed command
npm install -g @vue/cli
Create a project with the specified name:
vue create 项目的名字
9.4 Composition of src directory in vue project
- assets: store static resource files used by the project, such as: css style sheets, image resources
- components: reusable components encapsulated by programmers
- main.js: is the entry file of the project. Corresponding to the entry in webpack, the operation of the entire project must first execute main.js
- App.vue: the root component of the project
9.5 Running process of vue project
In the factory project, the task of vue: render App.vue to the designated area of index.html through main.js
in:
- App.vue: used to write the template structure to be rendered
- index.html: need to reserve an el area
- main.js: render App.vue to the area reserved by index.html
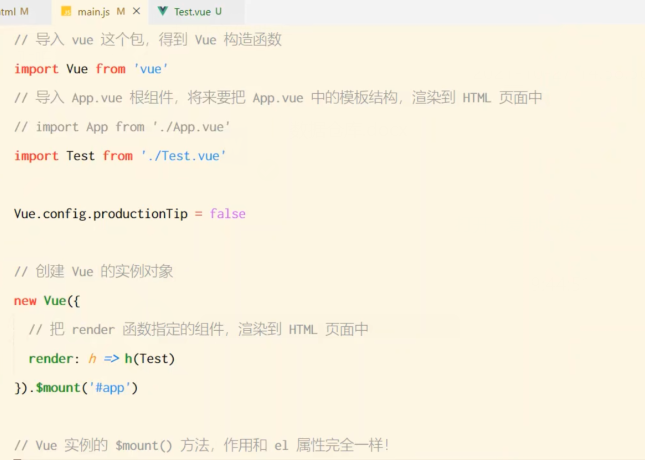