This article summarizes some knowledge points of C#, which is convenient for quick browsing and mastering some basic concepts of C# language.
This article is not well structured and organized. I copied a lot, wrote a lot, and wrote whatever came to mind.
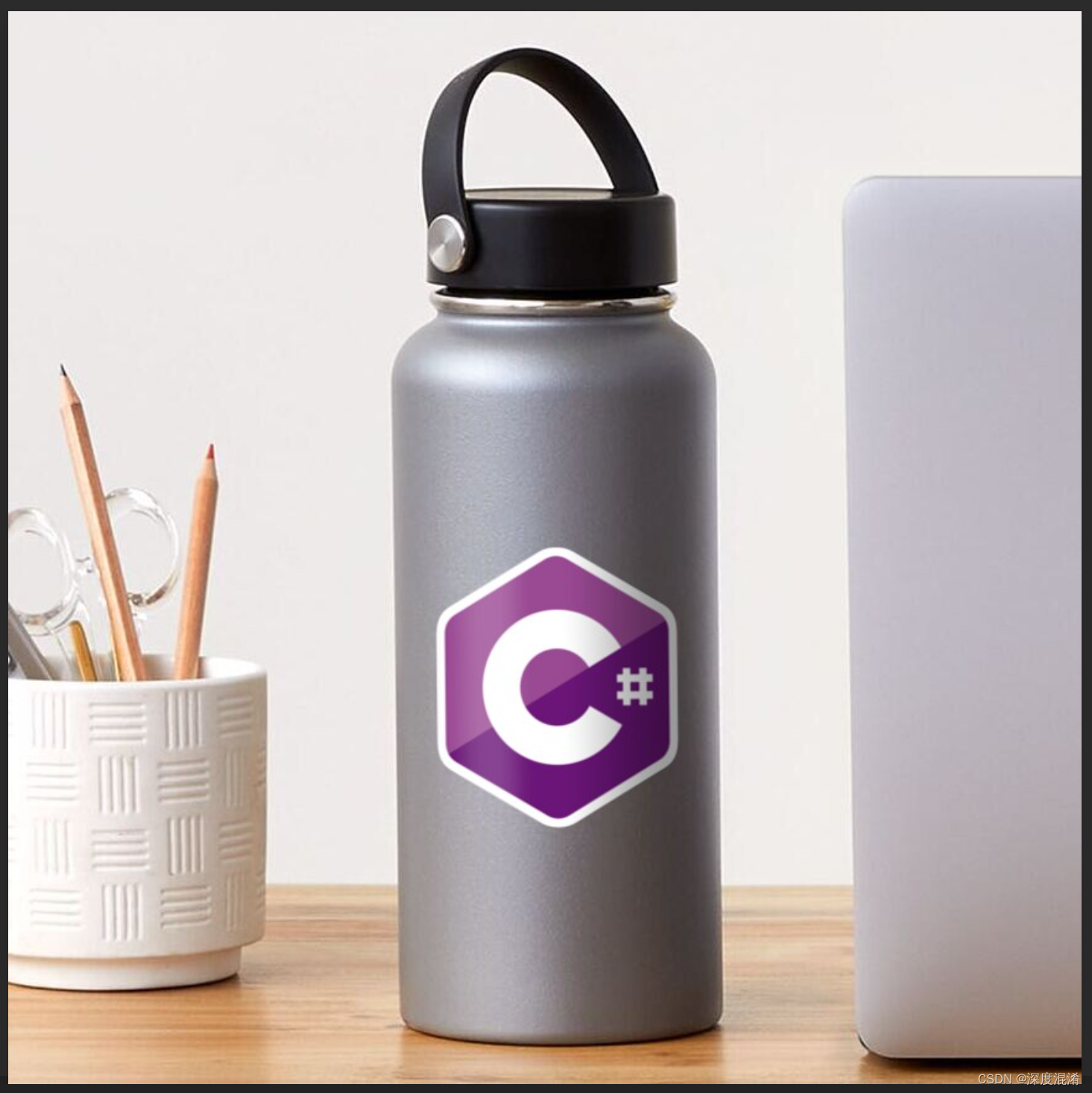
01 class class
Classes are the basic elements of Object-oriented programming languages (OOP) such as C#.
Class is the most important invention in the history of software development, which greatly improves the efficiency and reliability of software development. It is precisely because of classes that there is explosive growth of software.
A class is a user-defined reference data type, also known as a "class type". Each class contains a description of the data and a set of functions for manipulating the data or passing messages. Instances of classes are called objects. A class is an abstraction of a class of things with common characteristics in real life. If the data types provided in a program have a direct correspondence with the concepts in the application, the program will be easier to understand and easier to modify. A well-chosen set of user-defined classes can make programs more concise. Additionally, it makes various forms of code analysis easier to perform. In particular, it also makes it possible for the compiler to check for illegal uses of objects.
The inside of the class encapsulates properties and methods for manipulating its own members. A class is a definition of a certain object, with behavior (behavior), which describes what an object can do and the method (method), which are programs and processes that can operate on this object. It contains information about how an object behaves, including its name, properties, methods, and events. The composition of a class includes member attributes and member methods (data members and member functions). The data member corresponds to the attribute of the class, and the data member of the class is also a data type, which does not need to allocate memory. Member functions are used to operate various attributes of the class, which is a unique operation of a class. For example, "students" can "attend class", but "fruit" cannot. The operation that the class interacts with the outside world is called interface interface or proxy delegate.
Three properties of a class
(1) Encapsulation encapsulates data and operations into an organic whole. Since the private members in the class are hidden and only provide limited interfaces to the outside, it can ensure high internal cohesion and low coupling with the outside . Users do not need to understand the specific implementation details, but only use the members of the class with specific access rights through the external interface, which can enhance security and simplify programming.
(2) Inheritance is more in line with cognitive laws, making programs easier to understand and saving unnecessary repetitive code.
(3) Polymorphism means that the same operation acts on different objects, which can have different interpretations and produce different execution results. At runtime, methods in subclasses (derived classes) can be called through pointers to parent classes (base classes).
A class can be understood as a house in a building, or a part.
Equivalent to class class are structure struct, enumeration enum, interface interface and proxy delegate.
Class members include attribute attribute, method method (or called function function).
The class definition can include the class class, the structure struct, the enumeration enum, the interface interface and the proxy delegate (yes! I am not mistaken, you are not mistaken! These can be defined in the class definition!!!).
You can understand a class within a class as a tent in a room.
public class PragmaNode
{
/// <summary>
/// 定位令牌
/// </summary>
public Token Token { get; set; } = null;
/// <summary>
/// 提取有效的 Pragma 标志
/// </summary>
/// <param name="pragma"></param>
/// <returns></returns>
private void Pragma_Segments(out List<Token> segments, out List<string> pragma)
{
// ...
}
}
02 Namespace namespace
A namespace is a collection of classes.
A namespace can be understood as a building, or a component composed of many parts.
If you need to use your own or others' components, you need to use using to import the corresponding namespace.
using System;
using System.IO;
using System.Text;
using System.Collections;
using System.Collections.Generic;
using Newtonsoft.Json;
The Visual Studio menu [Project] [Manage NuGet Package (N)...] can easily obtain a large number of available basic components.
03 permission permission
Permissions are at the heart of all management software, and at the heart of programming languages.
From the perspective of software commercialization, there is no more important non-algorithmic factor than rights management.
If you can't understand and apply permissions proficiently, your programming level is still at the door.
"Permissions" in computer language refers to the accessibility conventions of members (referring to classes, class properties and class methods, variables).
The C# language specifies the permissions of members in two ways: (1) Access modifiers. (2) Braces.
03.01 Access modifiers
(1) public, public, class and class member modifiers, there is no level restriction on accessing members;
(2) private, private, class member modifier, can only be accessed inside the class;
(3) protected, protected, class member modifier, accessed inside the class or in a derived class, regardless of whether the class and the derived class are in the same assembly;
(4) internal, internal, class and class member modifiers, can only be accessed in the same assembly (Assembly);
(5) protected internal, protected internal: if it is an inheritance relationship, it can be accessed regardless of whether it is in the same assembly; if it is not an inheritance relationship, it can only be accessed in the same assembly.
03.02 Braces
A pair of curly braces restricts the scope of access to local variables.
Braces are storage boxes for packing things in the house. The right amount of storage boxes make the room tidy and reasonable.
Many software bugs appear in the conflict between local variables and global variables. Using curly braces can greatly reduce the occurrence of such errors.
In C#, brackets "{" and "}" are scope marks, a way to organize code, and are used to identify the beginning and end of a logically closely related piece of code in an application.
Braces can be nested to indicate different levels in the application.
switch(a)
{
case 1:
{
int b = a * 10;
break;
}
case 2:
{
int b = a / 10;
break;
}
}
or:
// 顶层逻辑
{
// 二层逻辑
{
}
}
In other words, { } is not necessarily used in blocks such as for. A pair of braces is a good embodiment of program logic.
Languages such as python and FORTRAN 77 do not have curly braces, making the maintainability of the program extremely poor. If python is used for large-scale software development, its subsequent maintenance and support will be expensive or even bottomless.
04 reserved words (keywords, reserved words)
A reserved word in C# code is a string with specific meaning to C#.
All computer languages have reserved words.
The following words are the main reserved words of C#.
abstract,as,base,bool,break,byte,case,catch,char,checked,
class,const,continue,decimal,default,delegate,do,double,else,
enum,event,explicit,extern,false,finally,fixed,
float,for,foreach,get,goto,if,implicit,int,
interface,internal,lock,long,namespace,new,
null,object,operator,out,override,partial,params,private,
protected,public,readonly,ref,return,sbyte,
sealed,set,short,sizeof,static,string,
struct,switch,T,this,throw,true,try,typeof,uint,
ulong,unchecked,unsafe,ushort,using,var,value,virtual,
void,volatile,while,yield,
from,in,group,orderby,where,select,let,into,join,equals,
dynamic,async,await,nameof,when,_,is,
stackalloc,global,nint,unint,and,with,init,not,or,record,
using,for,foreach,if,while,catch,switch,lock,fixed,
else,do,try,finally,unsafe,checked,unchecked,case,default,
double,System.Double,Double,
float,System.Single,Single,
decimal,System.Decimal,Decimal,
sbyte,System.SByte,SByte,
byte,System.Byte,Byte,
short,System.Int16,Int16,
ushort,System.UInt16,UInt16,
int,System.Int32,Int32,
uint,System.UInt32,UInt32,
long,System.Int64,Int64,
ulong,System.UInt64,UInt64,
nint,System.IntPtr,IntPtr,
unint,System.UIntPtr,UIntPtr,
bool,System.Boolean,Boolean,
string,System.String,String,
char,System.Char,Char,
object,System.Object,Object,
var,void,dynamic,
,async,await,as,event,explicit,implicit,
is,new,out,override,params,
ref,return,volatile,
Reserved words cannot be used in custom properties, variables and methods (except functions, operator overloading).
Therefore reserved words should be avoided.
Of course, you don't need to memorize these reserved words. If you make a mistake, Visual Studio will automatically prompt! ! !
05 sentence sentence
A sentence that performs an operation in a program can be called a statement, and ends with a semicolon ";".
For example:
a = b + c;
Multiple statements can be written on one line, or one statement can be written on multiple lines.
06 Program segment (block)
The function (method) of the class class is composed of a series of program segments (paragraphs), and the program segment contains several statements.
According to the difference of execution mode, there are many kinds of program segments. Example conditional block:
if(...) { ... } else { ... }
Others include:
for(...) { ... continue; }
foreach(... in ...) { ... continue; }
do { ... } while(...);
while(...) { }
switch(...) { case ...: break; default: ... }
using(...) { ... }
fixed(...) { ... }
lock(...) { ... }
unsafe { ... }
checked { ... }
unchecked { ... }
try { ... } catch(...) { ... } finally { ... }
07 Indentation and spaces
Indentation is used to indicate the structural level of the code, but indentation can clearly indicate the structural level of the program, and the code should be written in a uniform indentation format in program design.
Spaces have two functions, one is grammatical requirements and must be followed, and the other is to keep the statement from being too crowded.
For example:
int a = 4;
Luckily: Visual Studio has automatic typesetting.
You only need to delete the last curly brace, and then re-enter the curly brace to automatically typeset.
08 letter case
Uppercase "X" and lowercase "x" are two different characters for C#.
int X = 10;
int x = X + 10;
09 Notes
The C# language supports a variety of annotations.
(1) A single-line comment starts with a double slash "//" and cannot wrap.
// 单行注释
(2) Multi-line comments start with "/*" and end with "*/", and can be wrapped.
/*
多行注释
*/
(3) /// Annotations, specially used for annotations of class attributes and methods. This annotation has great benefits and applications. Where the attribute or method is referenced, the mouse can display the content of the comment, and understand the description and usage of the attribute and method.
/// <summary>
/// PRAGMA类型
/// </summary>
public PragmaType Type { get; set; } = PragmaType.UNDEFINED;
/// <summary>
/// 提取有效的 Pragma 标志
/// 同时返回 #define 预定义信息
/// </summary>
/// <param name="pragma"></param>
/// <returns></returns>
private void Pragma_Segments(out List<Token> segments, out List<string> pragma)
{
}
(4) #region and #endregion can be block comments with descriptions.
#region 多谱系图像合成算法
...
#endregion
(5) Preprocessing like #if __UNUSED__ can also be regarded as a comment.
#if DEBUG
// 内测版本代码
#else
// 发行版本代码
#endif
10 primitive numeric types
10.01 integer
Signed integers include sbyte (signed byte type), short (short integer type), int (integer type), long (long integer type).
Unsigned integers include byte (byte type), ushort (unsigned short integer), uint (unsigned integer), ulong (unsigned long integer).
10.02 Floating point numbers (real numbers)
Floating point types include float (single precision), double (double precision), and decimal (financial industry, decimal type).
The boolean type also belongs to the numerical value, which will be discussed later.
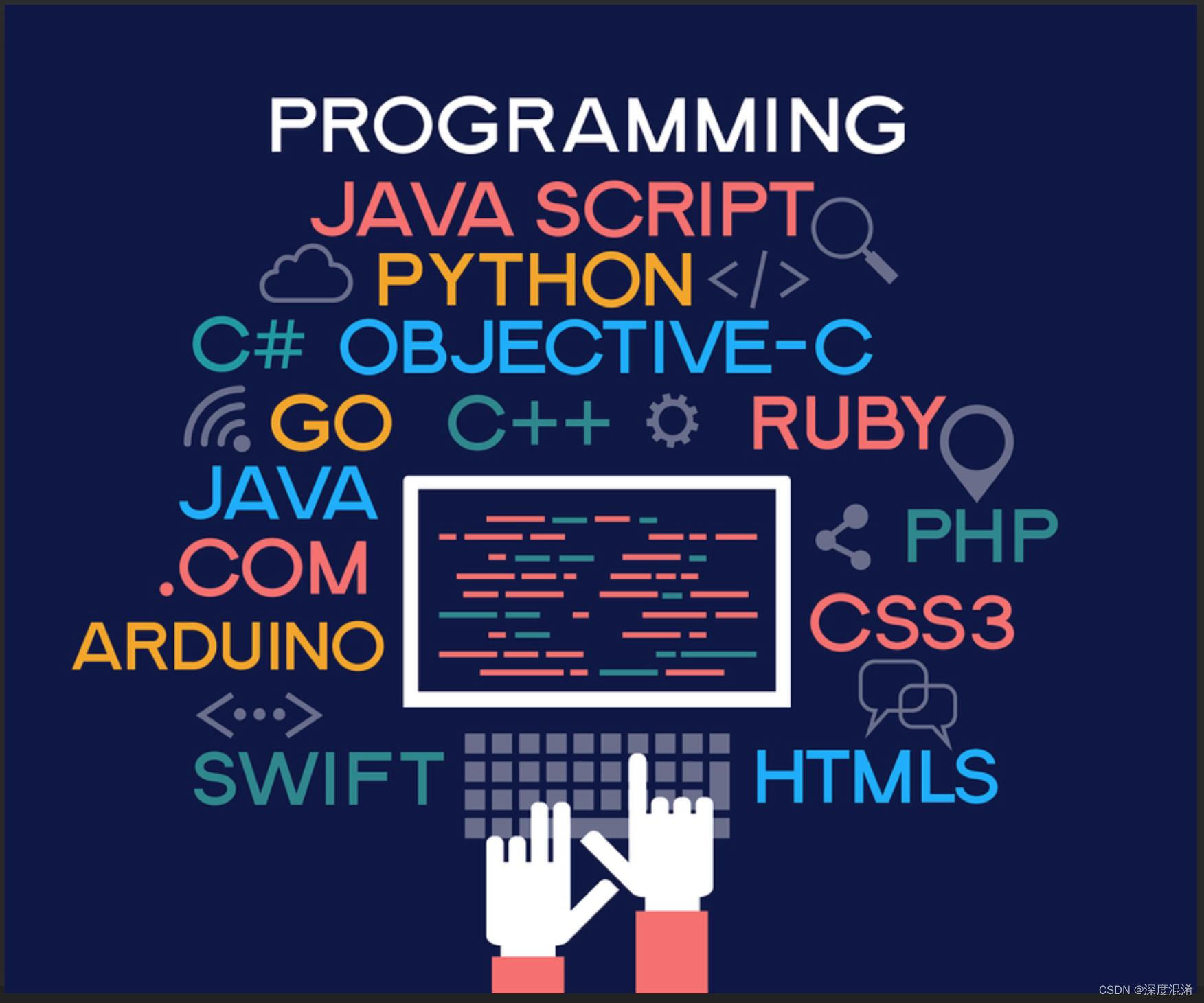
11 Characters and Strings
11.01 Unicode Character Set
C# supports the Unicode character set.
It is recommended that your code and data fully support Unicode, especially the UTF-8 character set.
11.02 char (character type)
char (character type): The data range is a single character in the Unicode character set between 0 and 65535, occupying 2 bytes.
char (character type) represents an unsigned 16-bit integer, and the possible value set of char (character type) corresponds to the Unicode character set.
11.03 string (string type)
String is the same as string in C#.
string(字符串型):指任意长度的Unicode字符序列,占用字节根据字符多少而定。
string(字符串型)表示包括数字与空格在内的若干个字符序列,允许只包含一个字符的字符串,甚至可以是不包含字符的空字符串。
12 布尔类型和对象类型
12.01 bool(布尔型)
C# 中 bool 与 boolean 相同。bool 看起来更简洁。
bool表示布尔逻辑量。bool(布尔型)数据范围是“true”(真)和“false”(假)。
bool(布尔型)名义上占用一个字节,事实并不如此。
bool(布尔型)和布尔值 true (真)、 false 都是保留字。
12.02 object(对象型)
object可以表示任何类型的值,其占用字节视具体表示的数据类型而定。
object(对象型)是所有其他类型的最终基类。
C#中的每种类型都是直接或间接从object类型派生的。
12.03 var 推断类型(其实也就是弱化类型的定义)
var可代替任何类型,编译器会根据上下文来判断你到底是想用什么类型,类似 object,但是效率比object高点。
我们的建议是,非不得已不用 var 与 object 。
13 再论 var
var 可能是从java系列语言获得的IP。
使用var定义变量时有以下四个特点:
(1) 必须在定义时初始化。也就是必须是:
var s = "abcd";
形式,而不能是如下形式:
var a;
a = "abcd";
(2)一但初始化完成,就不能再给变量赋与初始化值类型不同的值了。
(3)var要求是局部变量。
(4)使用var定义变量和object不同,它在效率上和使用强类型方式定义变量完全一样。
优点:
简单。
缺点:
利己不利他。大大增加了别人(或者是未来的自己)阅读代码、维护代码的难度。
喜欢使用 var 的程序员在团队中不受欢迎!
建议:
最好不用!最好不用!最好不用!
14 变量
变量是某种数值、数据类型的一个或一组实例。
14.01 变量声明(定义)
变量声明的格式为:
(1)数据类型名称 变量名列表;
(2)数据类型名称 变量名列表 = 初值;
例如:
// 声明一个整型变量
int a;
// 声明一个整型变量,带初值
int b = 2;
// 声明一个布尔型变量
bool c;
// 声明一个十进制变量
decimal d;
14.02 多个变量
虽然可以一次声明多个变量,例如:
// 声明两个有符号字节型变量
sbyte a, b;
一次声明多个变量,变量名之间用逗号分隔。
但不鼓励这样做!这会大大降低程序的可读性、可维护性、拓展性。
建议使用逐个定义,并给出初值。
sbyte a = 0;
sbyte b = 1;
14.03 变量赋值
C#规定,变量必须赋值后才能引用。为变量赋值需使用赋值号“=”。例如:
// 为变量赋值32
int a;
a = 32;
也可以使用变量为变量赋值,例如:
bool b;
// 为变量赋值true(假设open为已声明的bool型变量,其值为true)
b = open;
15 常数
15.01 整型常数
整型常数即整数,整型常数有三种形式:
(1)十进制形式,即通常意义上的整数,如:123,48910等。
(2)十六进制形式,输入十六进制整型常数,需要在数字前面加“0x”或“0X”,如:0x123,0X48910等
(3)八进制形式,输入八进制整型常数,需要在数字前面加“0”,如:0123,038等。
(4)二进制形式,如:0b010101010。
15.02 浮点数(实型)常数
实型常数即带小数的数值,实型常数有两种表示形式:
(1)小数形式,即人们通常的书写形式,如:0.123,12.3,.123等等。
(2)指数形式,也叫科学记数,由底数加大写的E或小写的e加指数组成。
如:123e5或123E5都表示123×10^5。
15.03 字符常数
字符常数表示单个的Unicode字符集中的一个字符,通常包括数字、各种字母、标点、符号和汉字等。
字符常数用一对英文单引号界定,如,'X','x','+','国' 等等。
特殊字符的处理
在C#中,有些字符不能直接放在单引号中作为字符常数,这时需要使用转义符来表示这些字符常数,转义符由反斜杠“\”加字符组成,如 '\n' 。
15.04 字符串常数
字符串常数是由一对双引号界定的字符序列,例如:
"欢迎使用C#!"
"I am a student."
需要注意的是,即使由双引号界定的一个字符,也是字符串常数,不能当做字符常数看待,例如,'A'与"A",前者是字符常数,后者是字符串常数。
16 const 与 readonly 常量
容易混淆的两种常量(不是常数!)
静态常量 const 是指编译器在编译时候会对常量进行解析,并将常量的值替换成初始化的那个值。
动态常量 readonly 的值则是在运行的那一刻才获得的。编译器编译期间将其标示为只读常量,而不用常量的值代替,这样动态常量不必在声明的时候就初始化,而可以延迟到构造函数中初始化。
17 类型转换
17.01 隐式转换
隐式转换是系统自动执行的数据类型转换。隐式转换的基本原则是允许数值范围小的类型向数值范围大的类型转换,允许无符号整数类型向有符号整数类型转换。
17.02 显式转换
显式转换也叫强制转换,是在代码中明确指示将某一类型的数据转换为另一种类型。显式转换的一般格式为:
(数据类型名称)数据
例如:
int x = 600;
short z = (short)x;
显式转换中可能导致数据的丢失,例如:
decimal d = 234.55M;
int x = (int)d;
17.03 类型转换方法
(1) Parse方法
Parse方法可以将特定格式的字符串转换为数值。Parse方法的使用格式为:
数值类型名称.Parse(字符串型表达式)
例如:
int x = int.Parse("123");
(2) ToString方法
ToString方法可将其他数据类型的变量值转换为字符串类型。ToString方法的使用格式为:
变量名称.ToString( )
例如:
int x = 123;
string s = x.ToString();
string t = x + "";
18 运算符与表达式
① 一元运算符:-(取负)、+(取正)、++(增量)、--(减量)。
② 二元运算符:+(加)、-(减)、*(乘)、/(除)、%(求余)。
18.01 字符串运算符与字符串表达式
字符串运算符只有一个,即“+”运算符,表示将两个字符串连接起来。例如:
string connected = "abcd" + "ef";
// connec的值为“abcdef”
“+”运算符还可以将字符型数据与字符串型数据或多个字符型数据连接在一起,例如:
string connec="abcd" + 'e' + 'f';
// connec的值为“abcdef”
18.02 关系运算符与关系表达式
依次为大于,小于,大于等于,小于等于,等于,不等于。
用于字符串的关系运算符只有相等“==”与不等“!=”运算符。
>,<,>=,<=,==,!=。
18.03 逻辑运算符与逻辑表达式
在C#中,最常用的逻辑运算符是!(非)、&&与、||(或)。
例如:
// b1的值为false
bool b1 = !true;
// b2的值为false
bool b2 = (5>3) && (1>2);
// b3的值为true
bool b3 = (5>3) || (1>2);
18.04 条件运算符与条件表达式
条件运算符是C#中唯一的三元运算符,条件运算符由符号“?”与“:”组成,通过操作三个操作数完成运算,其一般格式为:
布尔类型表达式?表达式1:表达式2
例如:
int a = (b>10) ? 0 : 1;
18.05 赋值运算符与赋值表达式
在赋值表达式中,赋值运算符左边的操作数叫左操作数,赋值运算符右边的操作数叫右操作数。左操作数通常是一个变量。
复合赋值运算符,如“*=”、“/=”、“%=”、“+=”、“-=”等。
例如:
a /= 10;
19 静态成员和非静态成员的区别
静态变量使用 static 修饰符进行声明,在类被实例化时创建,通过类进行访问。
不带有 static 修饰符声明的变量称做非静态变量,在对象被实例化时创建,通过对象进行访问;
定义:
public class KPoint
{
public static int DIM = 3;
public int[] XYZ = new int[3];
}
使用:
KPoint kx = new KPoint();
for(int i=0; i<KPoint.DIM; i++)
{
k[i] = 0;
}
一个类的所有实例的同一静态变量都是同一个值,同一个类的不同实例的同一非静态变量可以是不同的值;
静态函数的实现里不能使用非静态成员,如非静态变量、非静态函数等。
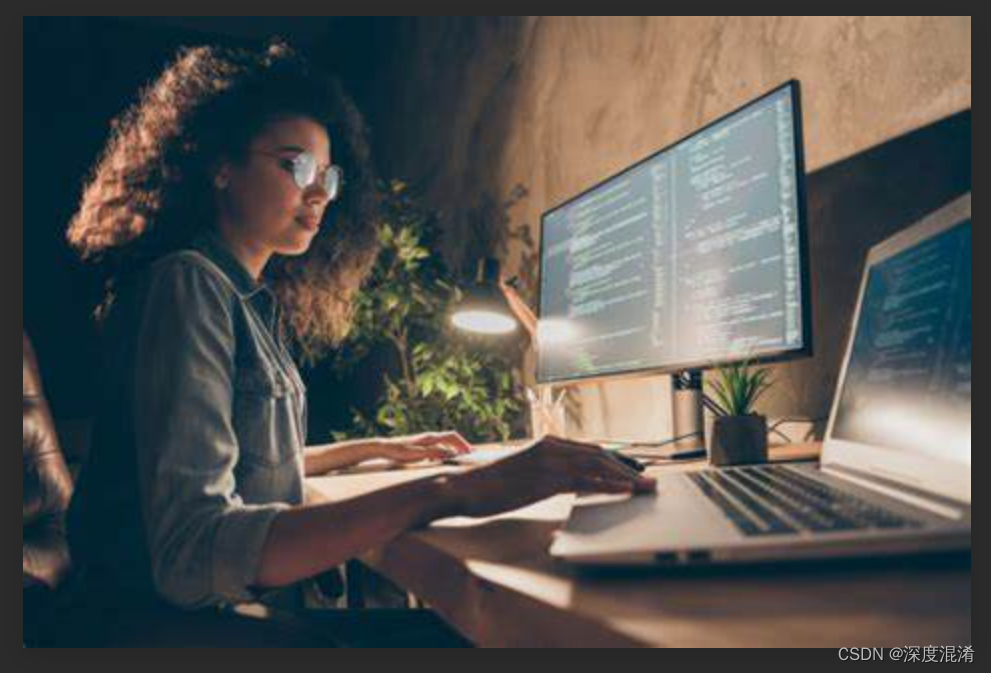
20 extern 外援
extern 修饰符用于声明由程序集外部实现的成员函数。
经常用于系统API函数的调用(通过 DllImport )。注意,和DllImport一起使用时要加上 static 修饰符。extern也可以用于对于同一程序集不同版本组件的调用(用 extern 声明别名)。不能与 abstract 修饰符同时使用。
21 abstract 抽!像!
abstract 修饰符可以用于类、方法、属性、事件和索引指示器(indexer),表示其为抽象成员。abstract 不可以和 static 、virtual 一起使用。声明为 abstract 成员可以不包括实现代码,但只要类中还有未实现的抽象成员(即抽象类),那么它的对象就不能被实例化,通常用于强制继承类必须实现某一成员。
22 internal 特供
internal 修饰符可以用于类型或成员,使用该修饰符声明的类型或成员只能在同一程集内访问接口的成员,不能使用 internal 修饰符。值得注意的是,如果为 internal 成员加上了 protected 修饰符,这时的访问级别为internal 或 protected。只是看字面意思容易弄错,许多人认为 internal protected应该是“只有同一个程序集中的子类可以访问”,但其实它表示“同一个程序集中的所有类,以及所有程序集中的子类都可以访问”。
23 sealed 胶带密封
sealed 修饰符表示密封用于类时,表示该类不能再被继承,不能和 abstract 同时使用,因为这两个修饰符在含义上互相排斥,用于方法和属性时,表示该方法或属性不能再被继承,必须和 override 关键字一起使用,因为使用 sealed 修饰符的方法或属性肯定是基类中相应的虚成员,通常用于实现第三方类库时不想被客户端继承,或用于没有必要再继承的类以防止滥用继承造成层次结构体系混乱恰当的利用 sealed 修饰符也可以提高一定的运行效率,因为不用考虑继承类会重写该成员。
24 override 和 overload
override(重写,覆盖) (1)方法名、参数、返回值相同。 (2)子类方法不能缩小父类方法的访问权限。 (3)子类方法不能抛出比父类方法更多的异常(但子类方法可以不抛出异常)。 (4)存在于父类和子类之间。 (5)方法被定义为final不能被重写。 (6)被覆盖的方法不能为private,否则在其子类中只是新定义了一个方法,并没有对其进行覆盖。
overload(重载,过载) (1)参数类型、个数、顺序至少有一个不相同。 (2)不能重载只有返回值不同的方法名。 (3)针对于一个类而言。 (4)不能通过访问权限、返回类型、抛出的异常进行重载; (5)方法的异常类型和数目不会对重载造成影响;
25 索引器 indexer
实现索引指示器(indexer)的类可以象数组那样使用其实例后的对象,但与数组不同的是索引指示器的参数类型不仅限于int简单来说,其本质就是一个含参数属性。
public class Team
{
private string[] Members = new string[] { "Messi", "CR7", "MP" };
public string this[int idx]
{
get
{
if (idx >= 0 && idx < Members.Length)
return Members[idx];
else
return string.Empty;
}
set
{
if (idx >= 0 && idx < Members.Length)
Members[idx]= value;
}
}
使用:
Team tx = new Team;
string m = tx[0];
26 new 修饰符与 new 操作符
new 修饰符与 new 操作符是两个概念。new 修饰符用于声明类或类的成员,表示隐藏了基类中同名的成员。而new 操作符用于实例化一个类型new 修饰符只能用于继承类,一般用于弥补基类设计的不足。new 修饰符和 override 修饰符不可同时用在一个成员上,因为这两个修饰符在含义上互相排斥
27 this 自闭
this 是一个保留字,仅限于构造函数和方法成员中使用在类的构造函数中出现表示对正在构造的对象本身的引用,在类的方法中出现表示对调用该方法的对象的引用,在结构的构造上函数中出现表示对正在构造的结构的引用,在结构的方法中出现表示对调用该方法的结果的引用this 保留字不能用于静态成员的实现里,因为这时对象或结构并未实例化在 C# 系统中,this 实际上是一个常量,所以不能使用 this++ 这样的运算this 保留字一般用于限定同名的隐藏成员、将对象本身做为参数、声明索引访问器、判断传入参数的对象是否为本身
28 属性访问 get & set
属性访问器(Property Accessor),包括 get 访问器和 set 访问器分别用于字段的读写操作其设计目的主要是为了实现面向对象(OO)中的封装思想。根据该思想,字段最好设为private,一个精巧的类最好不要直接把字段设为公有提供给客户调用端直接访问另外要注意属性本身并不一定和字段相联系
29 接口 interface
接口可以包含属性、方法、索引指示器和事件,但不能包含常量、域、操作符、构造函数和析构函数,而且也不能包含任何静态成员。
30 抽象类abstract和接口interface
抽象类(abstract class)可以包含功能定义和实现,接口(interface)只能包含功能定义抽象类是从一系列相关对象中抽象出来的概念, 因此反映的是事物的内部共性;接口是为了满足外部调用而定义的一个功能约定, 因此反映的是事物的外部特性分析对象,提炼内部共性形成抽象类,用以表示对象本质,即“是什么”为外部提供调用或功能需要扩充时优先使用接口
31 释放内存
C#在内存管理方面提供了GC(Garbage Collection),负责自动释放托管资源和内存回收的工作。但在以下两种情况需要我们手工进行资源释放:一、由于它无法对非托管资源进行释放,所以我们必须自己提供方法来释放对象内分配的非托管资源,例如你在对象的实现代码中使用了一个COM对象;二、你的类在运行是会产生大量实例(象GIS 中的Geometry),必须自己手工释放这些资源以提高程序的运行效率最理想的办法是通过实现一个接口显式的提供给客户调用端手工释放对象,System 命名空间内有一个 IDisposable 接口,拿来做这事非常合适,省得我们自己再声明一个接口了。
32 StringBuilder 夹子
String 在进行运算时(如赋值、拼接等)会产生一个新的实例,而 StringBuilder 则不会。所以在大量字符串拼接或频繁对某一字符串进行操作时最好使用 StringBuilder,不要使用 String
另外,对于 String 我们不得不多说几句:
(1) 它是引用类型,在堆上分配内存
(2) 运算时会产生一个新的实例
(3) String 对象一旦生成不可改变(Immutable)
33 explicit 和 implicit
explicit 和 implicit 属于转换运算符,如用这两者可以让我们自定义的类型支持相互交换explicti 表示显式转换,如从 A -> B 必须进行强制类型转换(B = (B)A)implicit 表示隐式转换,如从 B -> A 只需直接赋值(A = B)隐式转换可以让我们的代码看上去更漂亮、更简洁易懂,所以最好多使用 implicit 运算符。不过!如果对象本身在转换时会损失一些信息(如精度),那么我们只能使用explicit 运算符,以便在编译期就能警告客户调用端。
34 params 核酸排队,永远的记忆
params 关键字在方法成员的参数列表中使用,为该方法提供了参数个数可变的能力它在只能出现一次并且不能在其后再有参数定义,之前可以。
35 Reflection 是反射不是发射
反射,Reflection,通过它我们可以在运行时获得各种信息,如程序集、模块、类型、字段、属性、方法和事件通过对类型动态实例化后,还可以对其执行操作简单来说就是用string可以在runtime为所欲为的东西,实际上就是一个.net framework内建的万能工厂一般用于插件式框架程序和设计模式的实现,当然反射是一种手段可以充分发挥其能量来完成你想做的任何事情(前面好象见过一位高人用反射调用一个官方类库中未说明的函数。。。)
未完不续。