1. vue-cli
1. What is vue-cli
Vue-cli (commonly known as: vue scaffolding ) is a tool officially provided by vue to quickly generate vue engineering projects .
Features:
① out of the box
②Based on webpack
③ Rich in functions and easy to expand
④Support the creation of vue2 and vue3 projects
The homepage of the Chinese official website of vue-cli: https://cli.vuejs.org/zh/
2. Install vue-cli
Vue-cli is a tool developed based on Node.js, so you need to use npm to install it as a globally available tool:
#全局安装vue-cli
npm install -g @vue/cli
#查看vue-cli 的版本,检验vue-cli 是否安装成功
vue --version
2.1 Solve the problem that Windows PowerShell does not recognize vue commands
By default, executing the vue --version command in PowerShell will prompt the following error message:
The solution is as follows:
①Run PowerShell as an administrator
② Execute the set-ExecutionPolicy RemoteSigned command
③Input the character Y and press Enter
3. Create a project
vue-cli provides two ways to create projects :
#基于【命令行】的方式创建vue项目
vue create 项目名称
#OR
#基于【可视化面板】创建vue项目
vue ui
4. Create a vue project based on vue ui
Step 1: Run the vue ui command under the terminal to automatically open the visual panel of the created project in the browser :
Step 2: Fill in the project name on the details page :
Step 3: Select manual configuration items on the preset page :
Step 4: Check the functions to be installed on the function page ( Choose Vue Version, Babel, CSS preprocessor, use configuration files ):
Step 5: Check the version of v ue and the required preprocessor on the configuration page :
Step 6: Save all the configurations just now as presets (templates), so that you can directly reuse the previous configurations when creating a project next time :
Step 7: Create a project and automatically install dependencies:
The essence of vue ui : After collecting the user's configuration information through the visual panel , the project is automatically initialized in the background based on the command line :
After the project is created, it will automatically enter the project dashboard :
5. Create a vue project based on the command line
Step 1: Run the vue create demo2 command in the terminal to create a Vue project based on the interactive command line :
Step 2: Select the features to install:
Step 3: Use the up and down arrows to select the version of vue, and use the enter key to confirm the selection:
Step 4: Use the up and down arrows to select the css preprocessor to use and confirm the selection with the enter key :
Step 5: Use the up and down arrows to select how to store the plugin's configuration information , and use the Enter key to confirm the selection:
Step 6: Whether to save the configuration just now as a preset:
Step 7: Choose how to install the dependencies in the project:
Step 8: Start creating a project and automatically install dependencies:
Step 9: The project is created:
6. Sort out the basic structure of the vue2 project
Main files:
src -> app.vue
src -> main.js
//1.导入 Vue的构造函数
import Vue from 'vue '
// 2.导入 App 根组件
import App from '.lApp. vue'
//屏蔽浏览器console面板的提示消息
Vue.config.productionTip = false
// 3.创建vue实例对象
new Vue( {
render: h => h(App), // 3.1使用render函数渲染App根组件
}).$mount( ' #app') // 3.2把 App 根组件渲染到$mount函数指定的el 区域中
8. Use routing in vue2 projects
In the vue2 project, only the 3.x version of vue-router can be installed and used.
The main difference between version 3 and version 4 routing : the way of creating routing modules is different !
8.1 Recap: How Routing in Version 4.x Creates Routing Modules
import { createRouter , createWebHashHistory } from 'vue-router' // 1.按需导入需要的方法
import MyHome from ' . /Home . vue
//2.导入需要使用路由进行切换的组件
import MyMovie from ' . /Movie . vue
const router = createRouter ({
// 3.创建路由对象
history: createWebHashHistory(), // 3.1指定通过hash管理路由的切换
routes: [
// 3.2创建路由规则
{ path: ' /home',component: MyHome },
{ path: ' /movie ',component: MyMovie },
],
})
export default router //4.向外共享路由对象
8.2 Learning: How to create a routing module for routing in version 3.x
npm i vue- [email protected] -S
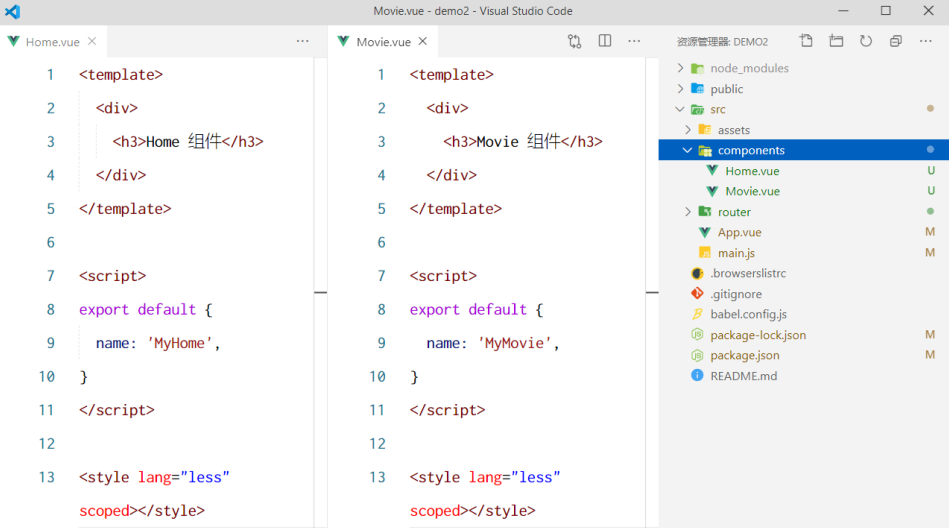
Step 3: Create router -> index.js routing module in the src directory:
import Vue from 'vue '
// 1.导入Vue2的构造函数
import VueRouter from 'vue- router '
//2.导入3.x路由的构造函数
import Home from '@/ components/Home.vue' // 3.导入需要使用路由切换的组件
import Movie from ' @/ components/Movie . vue
Vue .use(VueRouter)
1/ 4.调用Vue.use() 函数,把路由配置为Vue的插件
const router = new VueRouter({
//5.创建路由对象
routes: [
//5.1声明路由规则
{ path: '/', redirect: ' /home' },
{ path: '/home', component: Home },
{ path: ' /movie', component: Movie }
],
})
export default router //6.向外共享路由对象
Step 4: Import the routing module in main.js and mount it through the router attribute:
import Vue from 'vue'
import App from ' . /App. vue'
// 1.导入路由模块
import router from ' ./router
Vue . config. productionTip = false
new
Vue({
render: h => h(App),
// 2.挂载路由模块
// router: router,
router,
}).$mount('#app' )
Step 5: In the App.vue root component, declare a placeholder for the route using <router-view> :
<template>
<div>
<h1>App根组件</h1>
<hr/>
<!--声明路由的占位符-->
<router -view></ router -vi ew>
</div>
</ template>
2. Component library
1. What is a vue component library
In actual development, front-end developers can organize , package, and publish their encapsulated .vue components as npm packages for others to download and use. This ready-made component that can be directly downloaded and used in the project is called the vue component library .
2. The difference between vue component library and bootstrap
There is an essential difference between the two:
- Bootstrap only provides pure raw materials (css style, HTML structure and JS special effects), which need to be further assembled and modified by developers
- The vue component library is a highly customized off-the-shelf component that follows the vue syntax and works out of the box
3. The most commonly used vue component library
① PC terminal
- Element UI(https://element.eleme.cn/#/zh-CN)
- View UI(http://v1.iviewui.com/)
②Mobile terminal
- Mint UI(http://mint-ui.github.io/#!/zh-cn)
- Vant(https://vant-contrib.gitee.io/vant/#/zh-CN/)
4. Element UI
Element UI is a set of PC- side Vue component libraries open sourced by the front-end team of Ele.me. Support for use in vue2 and vue3 projects:
- The vue2 project uses the old version of Element UI (https://element.eleme.cn/#/zh-CN)
- The vue3 project uses the new version of Element Plus (https://element-plus.gitee.io/#/zh-CN)
4.1 Install element-ui in the vue2 project
Run the following terminal command:
npm i element-ui -S
4.2 Introducing element-ui
Developers can import all element-ui components at once , or only import element-ui components as required:
- Complete import: The operation is simple, but some additional components that are not used will be introduced , resulting in an excessively large project size
- Import on demand: The operation is relatively complicated, but only the components used will be imported , which can optimize the project volume
4.3 Complete introduction
Write the following in main.js :
import Vue from 'vue
import App from ' . /App. vue
// 1.完整引入element ui的组件
import El ementUI from ' element-ui'
// 2.导入element ui组件的样式
import ' e lement-ui/ lib/ theme-chalk/ index.css'
// 3.把ElementUI 注册为vue的插件[注册之后,即可在每个组件中直接使用每一个element ui的组件]
Vue . use( ElementUI)
new Vue({
render: h => h(App),
}).$mount('#app')
4.4 Import on demand
With the help of babel-plugin-component , we can only introduce the required components to achieve the purpose of reducing the size of the project.
Step 1 , install babel-plugin-component:
npm install babel-plugin-component -D
Step 2 , modify the babel.config.js configuration file in the root directory , and add the plugins node as follows:
module.exports = {
presets: [ ' @vue/cli-plugin-babel/preset'],
//↓↓↓
plugins: [
component
libr aryName: ' element-ui',
styleL ibraryName: ' theme-chalk' ,
},
],
],
//↑↑↑
}
Step 3 , if you only want to introduce some components , such as Button, then you need to write the following content in main.js:
import Vue from'vue'
import App from'./App. vue'
// 1.按需导入element ui的组件
import { Button } from ' element-ui '
//2.注册需要的组件
Vue . component( Button . name,But ton)
/*或写为
* Vue. use(Button)
*/
new Vue( {
render: h => h(App),
}). $mount( ' #app' )
4.5 Encapsulate the import and registration of components as independent modules
Create a new element-ui/index.js module in the src directory , and declare the following code:
//→模块路径/src/element-ui/ index. js
import Vue from ' vue
//按需导入element ui的组件
import { Button, Input } from ' e lement -ui '
//注册需要的组件
Vue . use(Button)
Vue . use( Input )
//→在main.js中导入
import ' ./element-ui '
3. Axios interceptor
1. Review: globally configure axios in the vue3 project
import { createApp } from 'vue
import App from ' . /App. vue
// 1.导入axios
import axios from ' axios '
const app = createApp(App )
// 2.配置请求根路径
ax ios . defaults. baseURL = ' https: / /www. escook. cn'
// 3.全局配置axios
app . config. globalProperties.$http = axios
app . mount(,#app' )
2. Configure axios globally in the vue2 project
Axios needs to be configured globally through the prototype prototype object of the Vue constructor in the main.js entry file:
import Vue from 'vue "
import App from ' ./App . vue '
// 1.导入axios
import axios from ' axios'
// 2.配置请求根路径
axios . defaults.baseURL = 'https:/ /www. escook. cn'
// 3.通过Vue构造函数的原型对象,全局配置axios
Vue . prototype . $http =
axios
new Vue( {
render: h => h(App),
}). $mount( ' #app' )
3. What is an interceptor
Interceptors (English: Interceptors) will be triggered automatically every time an ajax request is initiated and a response is received .
Application scenario:
①Token authentication
②Loading effect
③etc…
4. Configure the request interceptor
The request interceptor can be configured through axios.interceptors.request.use ( successful callback , failed callback). The sample code is as follows:
axios . interceptors . request . use( function (config) {
// DO something before request is sent
return config;
}, function (error) {
// DO something with request error
return Promise. reject(error );
})
Note: the failure callback function can be omitted !
4.1 Request Interceptor – Token Authentication
import axios from ' axios'
ax ios . defaults. baseURL = 'https://www.escook.cn'
//配置请求的拦截器
axios . interceptors. request .use(config => {
//为当前请求配置Token 认证字段
config . headers . Authorization = 'Bearer xXX '
return config
})
Vue . prototype . $http = axios
4.2 Request interceptor – show Loading effect
With the help of the Loading effect component (https://element.eleme.cn/#/zh-CN/component/loading) provided by element ui, the display of the Loading effect can be easily realized:
// 1.按需导入Loading 效果组件
import { Loading } from'element-ui'
// 2.声明变量,用来存储Loading组件的实例对象
let loadingInstance = null
//配置请求的拦截器
axios . interceptors . request . use(config => {
// 3.调用Loading 组件的service() 方法,创建Loading 组件的实例,并全屏展示loading 效果
loadingInstance = Loading. service({ fullscreen: true })
return config
})
5. Configure the response interceptor
Response interceptors can be configured through axios.interceptors.response.use ( success callback , failure callback). The sample code is as follows:
// Add a response interceptor
axios . interceptors. response . use( function (response) {
// Any status code that lie within the range of 2xx cause this function to tr igger
// DO something with response data
return response;
}, function (error) {
// Any status codes that falls outside the range of 2xx cause this function to trigger
// DO something with response error
return Promise . reject(error );
});
Note: the failure callback function can be omitted!
5.1 Response interceptor – turn off Loading effect
Call the close() method provided by the Loading instance to close the Loading effect . The sample code is as follows:
//响应拦截器
axios.interceptors.response.use( response => {
//调用Loading 实例的close方法即可关闭loading效果
loadingInstance.close( )
return response
})
Four, proxy cross-domain proxy
1. Review: cross-domain problems of interfaces
The address where the vue project runs: http://localhost:8080/
The address where the API interface runs: https://www.escook.cn/api/users
Since the current API interface does not enable CORS cross-domain resource sharing, by default, the above interface cannot request success !
2. Solve the cross-domain problem of the interface through the proxy
When a project created through vue-cli encounters an interface cross-domain problem, it can be solved by proxy :
①Set the request root path of axios to the running address of the vue project (interface requests are no longer cross-domain)
②The vue project finds that the requested interface does not exist, and forwards the request to the proxy proxy
③The proxy replaces the request root path with the value of the devServer.proxy attribute, and initiates a real data request
④The agent forwards the requested data to axios
3. Configure the proxy proxy in the project
Step 1. In the main.js entry file, change the request root path of axios to the root path of the current web project :
// axios.defaults.baseURL = 'https://www.escook.cn'
//配置请求根路径
axios.defaults.baseURL = 'http://localhost:8080'
Step 2 , create a vue.config.js configuration file in the project root directory , and declare the following configuration:
module.exports = {
devServer: {
//当前项目在开发调试阶段,
//会将任何未知请求(没有匹配到静态文件的请求)代理到https: / /www. escook. cn
proxy: 'https://www.escook.cn'
},
}
Notice:
① The proxy function provided by devServer.proxy only takes effect during the development and debugging phase
②When the project is released online, the API interface server still needs to enable CORS cross-domain resource sharing
video:
This article is a self-summary of my learning video, please forgive me if there is any infringement. Infringement must be deleted.