Partial
/**
* Make all properties in T optional
*/
type Partial<T> = {
[P in keyof T]?: T[P];
};
The effect is to make all attributes in the incoming type optional
Example of use
export interface Student {
name: string;
age: number;
}
const student1: Student = {}
const student2: Partial<Student> = {}
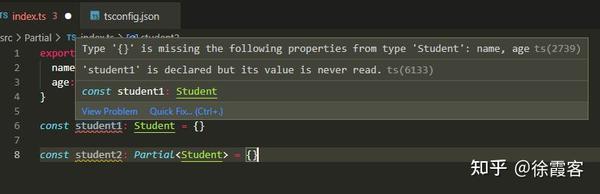
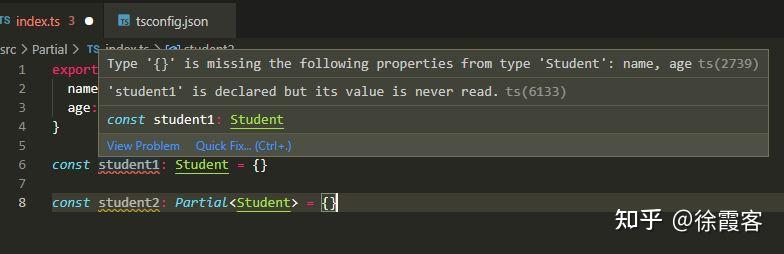
The type of the variable student1 is Student. By default, all attributes of Student cannot be empty, and all will report an error, but student2 will not
Required
/**
- Make all properties in T required
*/
type Required<T> = {
[P in keyof T]-?: T[P];
};
The role of Partial is the opposite, making all attributes in the incoming type mandatory
Example of use
export interface Student {
name?: string;
age?: number;
}
const student1: Student = {}
const student2: Required<Student> = {}
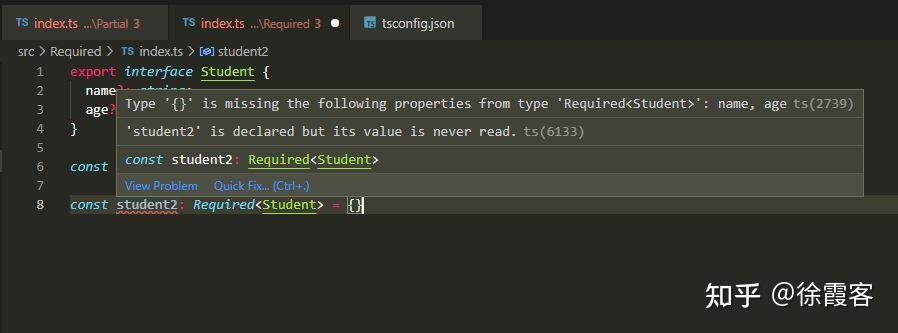
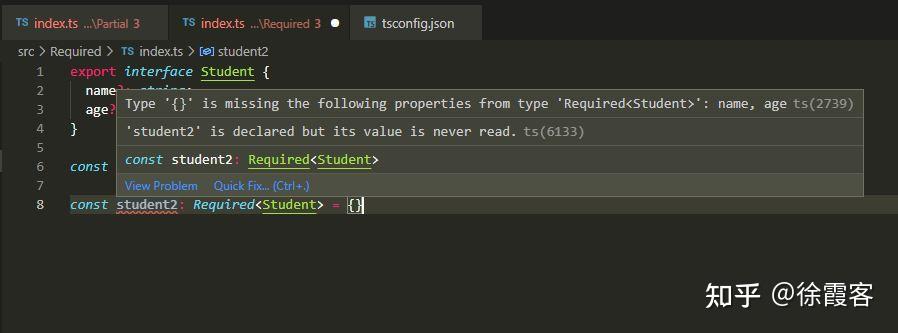
The type of the variable student1 is Student. By default, all attributes of Student can be empty, and all will not report an error, but student2 will report an error.
Readonly (read-only)
/**
- Make all properties in T readonly
*/
type Readonly<T> = {
readonly [P in keyof T]: T[P];
};
The effect is to make all properties in the incoming type read-only (the properties cannot be modified)
Example of use
export interface Student {
name: string;
age: number;
}
const student1: Student = {
name: ‘张三’,
age: 20
}
student1.age = 21
const student2: Readonly<Student> = {
name: ‘李四’,
age: 20
}
student2.age = 21
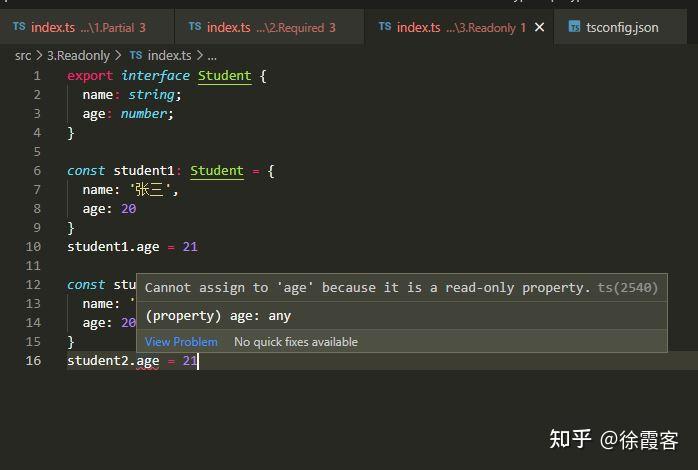
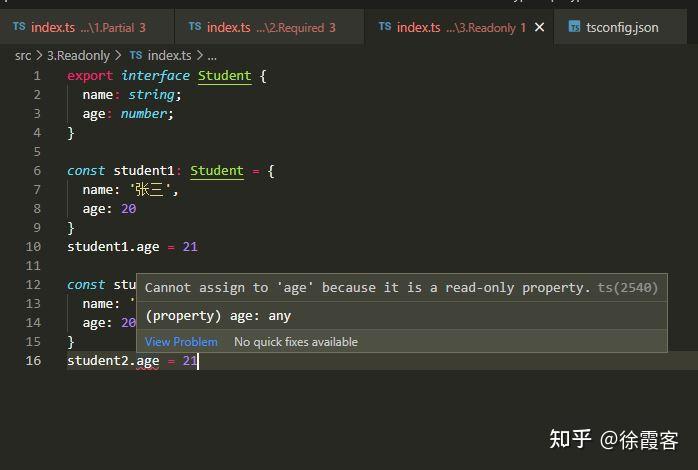
Reassigning the attribute age of student1 will not report an error, but reassigning the attribute age of student2 will report an error, because all attributes of student2 are read-only
Pick (choose)
/**
- From T, pick a set of properties whose keys are in the union K
*/
type Pick<T, K extends keyof T> = {
[P in K]: T[P];
};
The function is to select some attributes in the incoming type to form a new type
Example of use
export interface Student {
name: string;
age: number;
}
const student1: Student = { name: 'Zhang San', age: 20 }
const student2: Pick<Student, ‘name’> = {
name: ‘李四’
}
const student3: Pick<Student, ‘name’> = {
name: ‘王五’,
age: 20
}
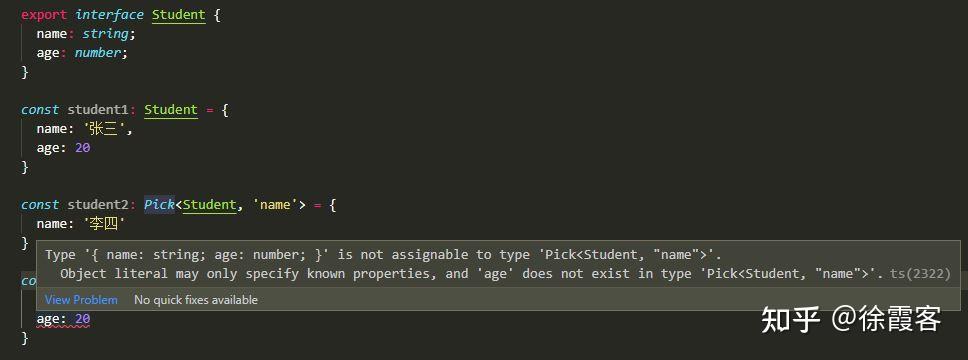
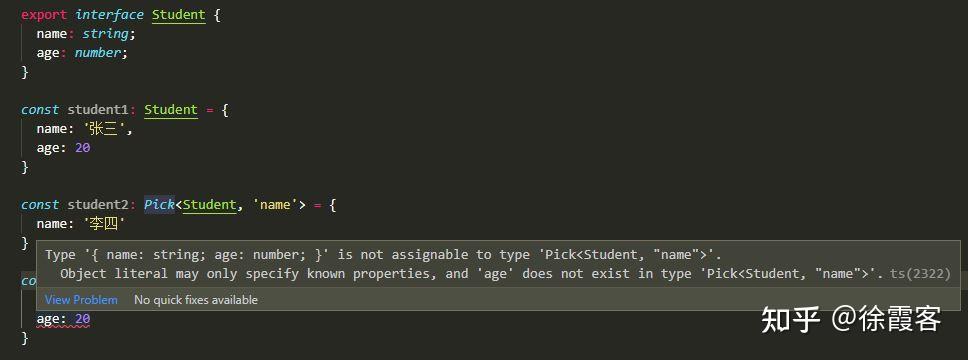
The variable student1 can have all the attributes name and age, the variable student2 can only have the attribute name, and the variable student3 plus the attribute age will report an error
Record
/**
- Construct a type with a set of properties K of type T
/
type Record<K extends keyof any, T> = {
[P in K]: T;
};
The role is to build a type that describes an object whose properties all have the same type
Example of use
export const student1: Record<string, any> = {
name: ‘张三’,
age: 20
}
Record should be a built-in type with high frequency of daily use. It is mainly used to describe objects. It is generally recommended not to use Object to describe objects, but to use Record instead. Record<string, any> can almost be said to be a panacea.
Exclude
/*
- Exclude from T those types that are assignable to U
*/
type Exclude<T, U> = T extends U ? never : T;
For the joint type (interface is useless), in human terms, exclude the same and leave the different
Example of use
export type PersonAttr = ‘name’ | ‘age’
export type StudentAttr = ‘name’ | ‘age’ | ‘class’ | ‘school’
const student1: Exclude<StudentAttr, PersonAttr>
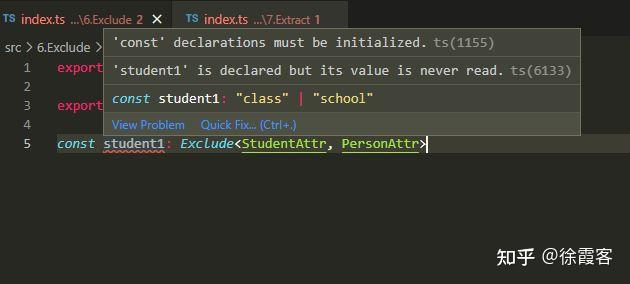
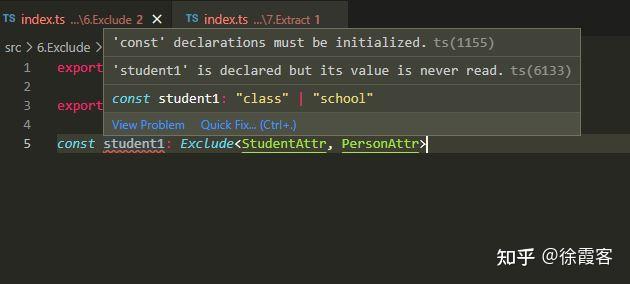
student1 can only be assigned as 'class' or 'school'
Extract
/**
- Extract from T those types that are assignable to U
*/
type Extract<T, U> = T extends U ? T : never;
Contrary to Exclude, for joint types, exclude different ones and take out the same ones
Example of use
export type PersonAttr = ‘name’ | ‘age’
export type StudentAttr = ‘name’ | ‘age’ | ‘class’ | ‘school’
const student1: Extract<StudentAttr, PersonAttr>
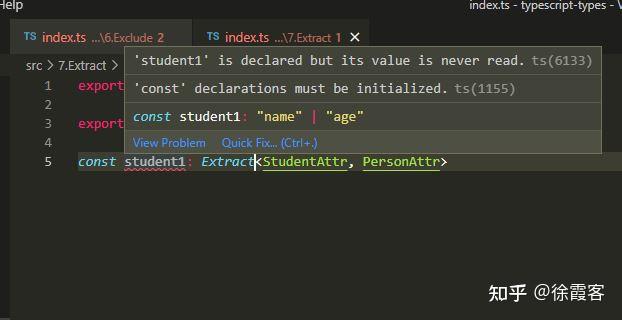
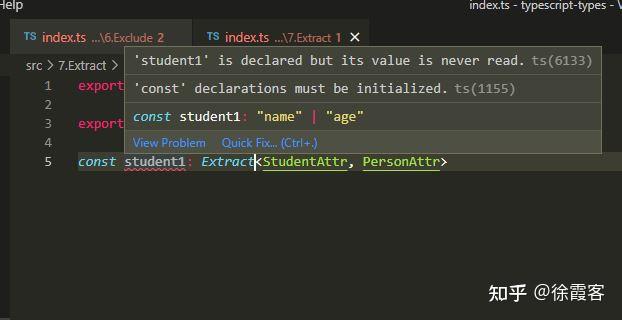
student1 can only be assigned as 'name' or 'age'
Omit (omitted)
/**
- Construct a type with the properties of T except for those in type K.
*/
type Omit<T, K extends keyof any> = Pick<T, Exclude<keyof T, K>>;
Pass in a type, and several attributes of this type, omit the incoming attributes to form a new type
Example of use
export interface Student {
name: string;
age: number;
class: string;
school: string;
}
export type PersonAttr = ‘name’ | ‘age’
export type StudentAttr = ‘name’ | ‘age’ | ‘class’ | ‘school’
const student1: Omit<Student, PersonAttr> = {}
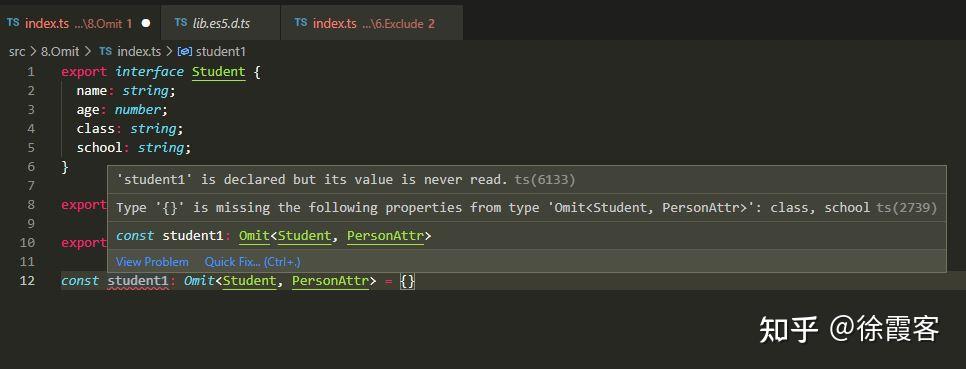
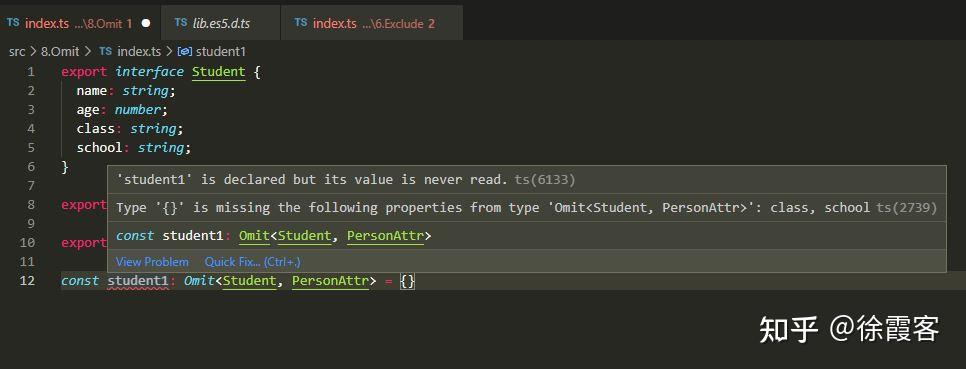
student1 reported an error, suggesting that there is no attribute 'name', 'age'
NonNullable (cannot be null)
/**
- Exclude null and undefined from T
*/
type NonNullable<T> = T extends null | undefined ? never : T;
Literally, cannot be empty
Example of use
export interface Student {
name: string;
age: number;
}
const student1: NonNullable<Student | undefined | null> = null
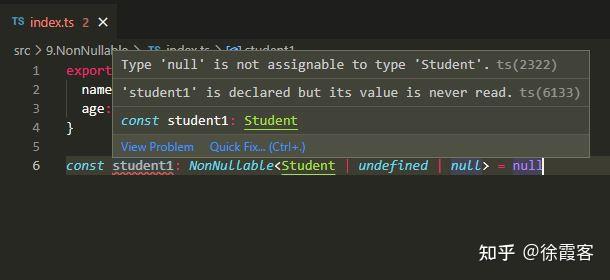
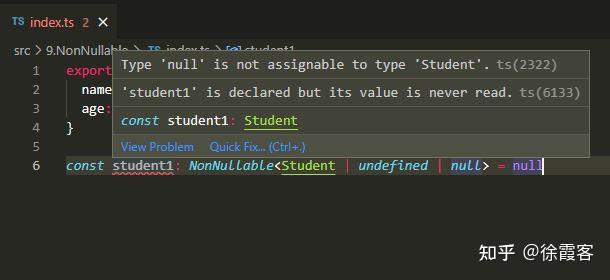
An error will be reported if student1 is assigned a value of null (enable type checking in the tsconfig.json configuration file, “skipLibCheck”: false
)
Parameters
/**
- Obtain the parameters of a function type in a tuple
*/
type Parameters<T extends (…args: any) => any> = T extends (…args: infer P) => any ? P : never;
Get the type of the parameters passed into the function
Example of use
export interface Student {
name: string;
age: number;
}
export interface StudentFunc {
(name: string, age: number): Student
}
const student1: Parameters<StudentFunc>
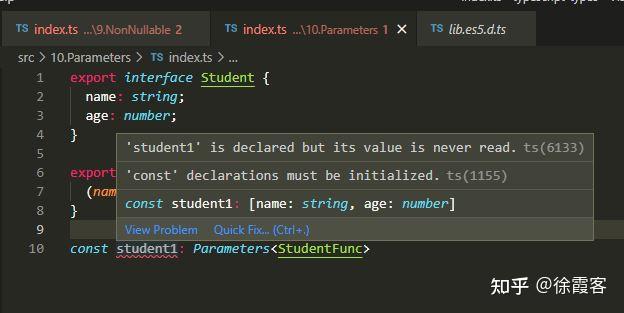
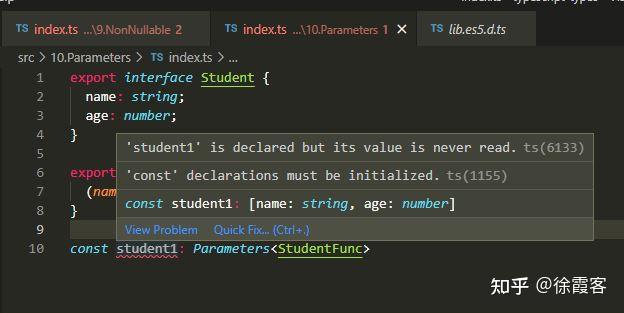
student1 is of type[name: string, age: number]
ConstructorParameters (constructor parameters)
/**
- Obtain the parameters of a constructor function type in a tuple
*/
type ConstructorParameters<T extends abstract new (…args: any) => any> = T extends abstract new (…args: infer P) => any ? P : never;
Get the type of parameters passed to the constructor
Example of use
export interface Student {
name: string;
age: number;
}
export interface StudentConstructor {
new (name: string, age: number): Student
}
const student1: ConstructorParameters<StudentConstructor>
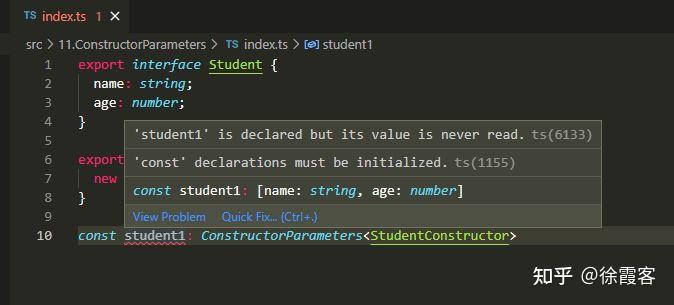
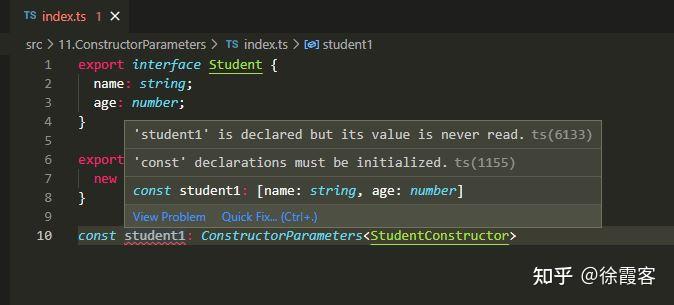
student1 is of type[name: string, age: number]
ReturnType (return type)
/**
- Obtain the return type of a function type
*/
type ReturnType<T extends (…args: any) => any> = T extends (…args: any) => infer R ? R : any;
Get the return type of the passed function
Example of use
export interface Student {
name: string;
age: number;
}
export interface StudentFunc {
(name: string, age: number): Student
}
const student1: ReturnType<StudentFunc> = {}
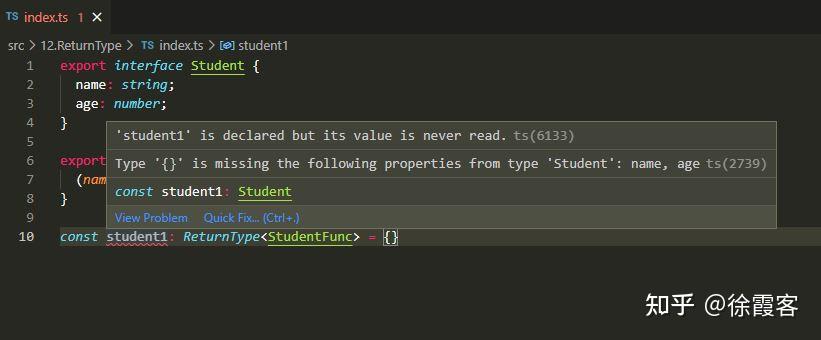
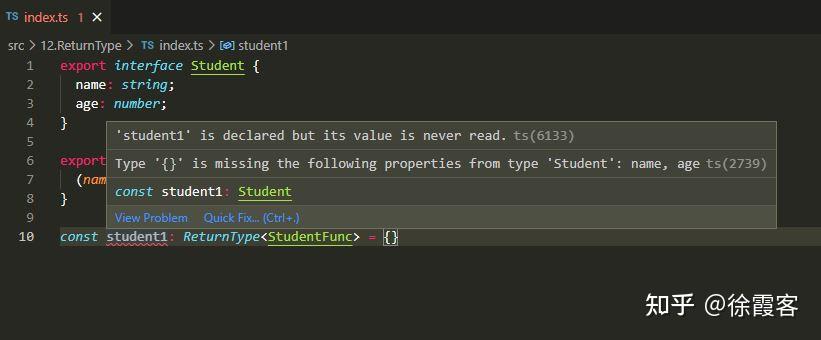
student1 is of typeStudent
InstanceType (construct return type, instance type)
/**
- Obtain the return type of a constructor function type
*/
type InstanceType<T extends abstract new (…args: any) => any> = T extends abstract new (…args: any) => infer R ? R : any;
Get the return type of the passed constructor
Example of use
const Student = class {
name: string;
age: number;
constructor (name: string, age: number) {
this.name = name
this.age = age
}
showInfo () {
console.log('name: ', this.name, 'age: ', this.age);
}
}
const student1: InstanceType<typeof Student> = new Student(‘张三’, 20)
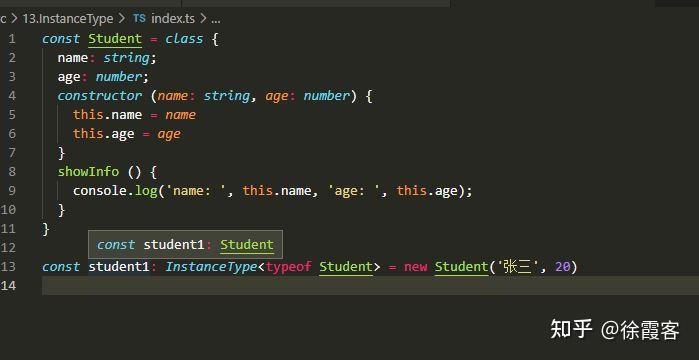
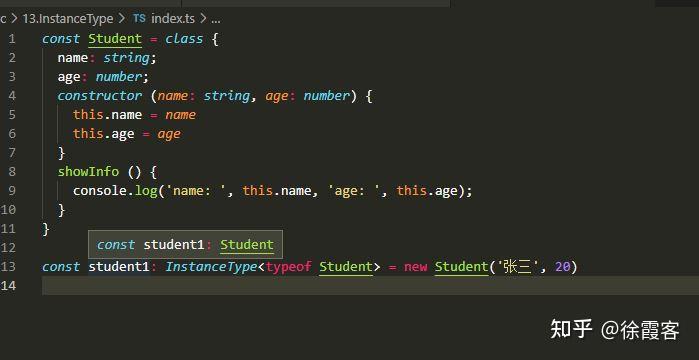
Personally think that this is a very useful built-in type. Currently, class is used more and more in front-end projects. In TS, class can actually be used as a type declaration space to describe object types, but Generally speaking, it seems that this is rarely used, and interface or type are mostly used
export class Student {
name: string;
age: number;
}
So generally, class is directly used as a variable declaration space, but how to describe its type for an instance created by class new, as mentioned above, it const student1: Student
will definitely report an error directly, because Student is used as a variable declaration space, which is useless Make a type declaration space (sounds so convoluted), then you can use InstanceType to solve the problem perfectly
Uppercase
/**
- Convert string literal type to uppercase
*/
type Uppercase<S extends string> = intrinsic;
capitalize
Example of use
export type StudentSexType = ‘male’ | ‘female’
const studentSex: Uppercase<StudentSexType> = ‘MALE’
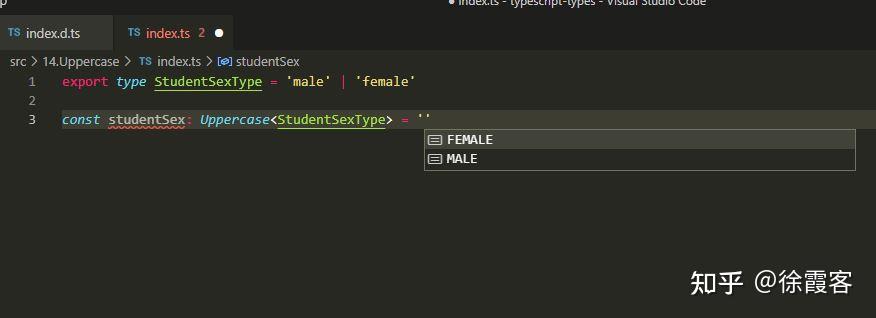
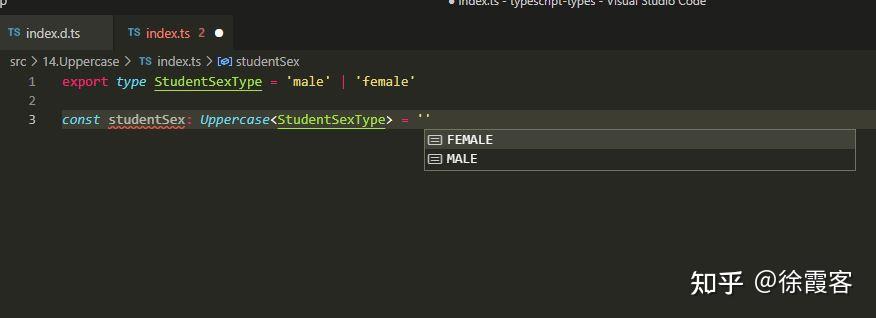
Lowercase (lowercase)
/**
- Convert string literal type to lowercase
*/
type Lowercase<S extends string> = intrinsic;
Lowercase
Example of use
export type StudentSexType = ‘MALE’ | ‘FEMALE’
const studentSex: Lowercase<StudentSexType> = ‘’
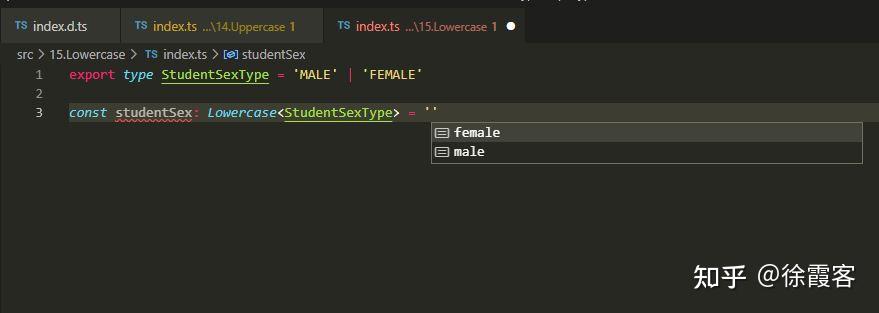
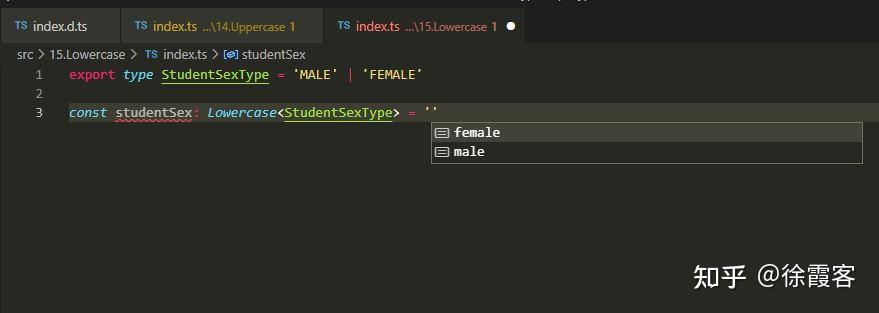
Capitalize (capitalize the first letter)
/**
- Convert first character of string literal type to uppercase
*/
type Capitalize<S extends string> = intrinsic;
Capitalize the first letter
Example of use
export type StudentSexType = ‘male’ | ‘female’
const studentSex: Capitalize<StudentSexType> = ‘’
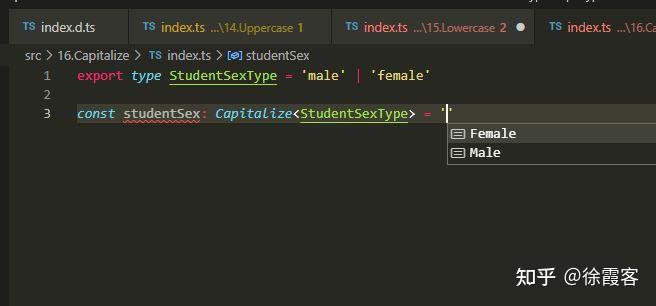
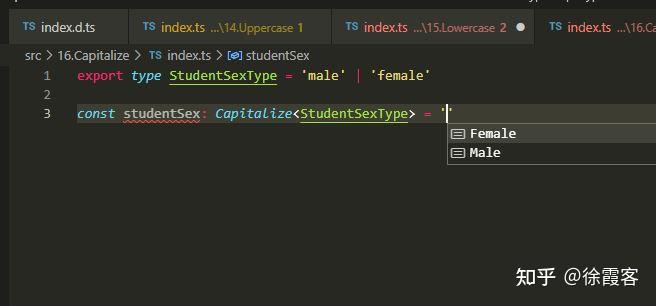
Uncapitalize (lowercase first letter)
/**
- Convert first character of string literal type to lowercase
*/
type Uncapitalize<S extends string> = intrinsic;
Lowercase the first letter
Example of use
export type StudentSexType = ‘MALE’ | ‘FEMALE’
const studentSex: Uncapitalize<StudentSexType> = ‘’
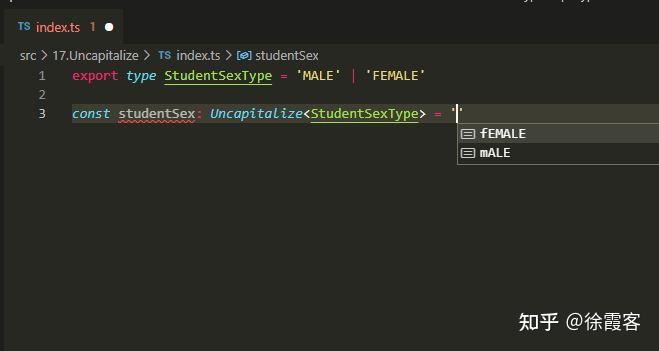
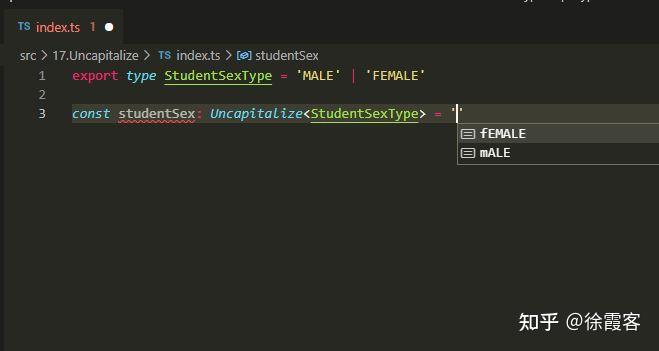