Reprinted: https://www.cnblogs.com/ysw-go/p/5384516.html
Iterator pattern definition
The Iterator pattern provides a way to sequentially access the various elements of an aggregate object without exposing the object's internal representation.
The role composition of the iterator pattern
(1) Iterator role (Iterator): Define the methods needed to traverse elements. Generally speaking, there are three methods: the method next() to get the next element, the method hasNext() to judge whether the traversal is over), move out The method remove() of the current object,
(2) Concrete Iterator: Implement the methods defined in the iterator interface to complete the iteration of the collection.
(3) Container role (Aggregate): generally an interface that provides an iterator() method, such as Collection interface, List interface, Set interface, etc. in java
(4) Concrete Aggregate: It is the concrete implementation class of the abstract container, such as the ordered list of the List interface implements ArrayList, the linked list of the List interface implements LinkList, and the hash list of the Set interface implements HashSet, etc.
Scenarios and Significance of Iterator Pattern Application
(1) Access the contents of an aggregate object without exposing its internal representation(2) Supports multiple traversal of aggregated objects
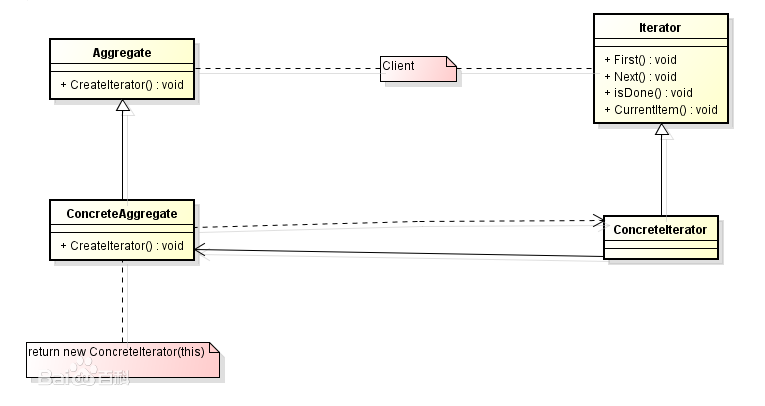
1 public interface Iterator { 2 3 public boolean hasNext(); 4 public Object next(); 5 }
Defining a Concrete Iterator
1 package patten.design; 2 3 import patten.design.List;; 4 5 public class ConcreteIterator implements Iterator { 6 private List list = null; 7 private int index; 8 9 public ConcreteIterator(List list) { 10 super(); 11 this.list = list; 12 } 13 14 @Override 15 public boolean hasNext() { 16 if (index >= list.getSize()) { 17 return false; 18 } else { 19 return true; 20 } 21 } 22 23 @Override 24 public Object next() { 25 Object object = list.get(index); 26 index++; 27 return object; 28 } 29 30 }
定义容器角色(Aggregate)
1 package patten.design; 2 3 //定义集合可以进行的操作 4 public interface List { 5 6 public void add(Object obj); 7 public Object get(int index); 8 public Iterator iterator(); 9 public int getSize(); 10 }
定义具体容器角色(ConcreteAggregate)
1 package patten.design; 2 3 public class ConcreteAggregate implements List{ 4 5 private Object[] list; 6 private int size=0; 7 private int index=0; 8 public ConcreteAggregate(){ 9 index=0; 10 size=0; 11 list=new Object[100]; 12 } 13 @Override 14 public void add(Object obj) { 15 list[index++]=obj; 16 size++; 17 } 18 19 @Override 20 public Iterator iterator() { 21 22 return new ConcreteIterator(this); 23 } 24 @Override 25 public Object get(int index) { 26 27 return list[index]; 28 } 29 @Override 30 public int getSize() { 31 32 return size; 33 } 34 35 }
代码测试
1 package patten.design; 2 3 public class IteratorTest { 4 5 /** 6 * @param args 7 */ 8 public static void main(String[] args) { 9 10 List list=new ConcreteAggregate(); 11 list.add("a"); 12 list.add("b"); 13 list.add("c"); 14 list.add("d"); 15 Iterator it=list.iterator(); 16 while(it.hasNext()){ 17 System.out.println(it.next()); 18 } 19 } 20 21 }
迭代器模式的优缺点:
迭代器模式的优点有:
- 简化了遍历方式,对于对象集合的遍历,还是比较麻烦的,对于数组或者有序列表,我们尚可以通过游标来取得,但用户需要在对集合了解很清楚的前提下,自行遍历对象,但是对于hash表来说,用户遍历起来就比较麻烦了。而引入了迭代器方法后,用户用起来就简单的多了。
- 可以提供多种遍历方式,比如说对有序列表,我们可以根据需要提供正序遍历,倒序遍历两种迭代器,用户用起来只需要得到我们实现好的迭代器,就可以方便的对集合进行遍历了。
- 封装性良好,用户只需要得到迭代器就可以遍历,而对于遍历算法则不用去关心。
迭代器模式的缺点:
- 对于比较简单的遍历(像数组或者有序列表),使用迭代器方式遍历较为繁琐,大家可能都有感觉,像ArrayList,我们宁可愿意使用for循环和get方法来遍历集合。
总的来说: 迭代器模式是与集合共生共死的,一般来说,我们只要实现一个集合,就需要同时提供这个集合的迭代器,就像java中的Collection,List、Set、Map等,这些集合都有自己的迭代器。假如我们要实现一个这样的新的容器,当然也需要引入迭代器模式,给我们的容器实现一个迭代器。